"Mastering Java Collections: Unleashing Sets and Queues for Dynamic Coding"
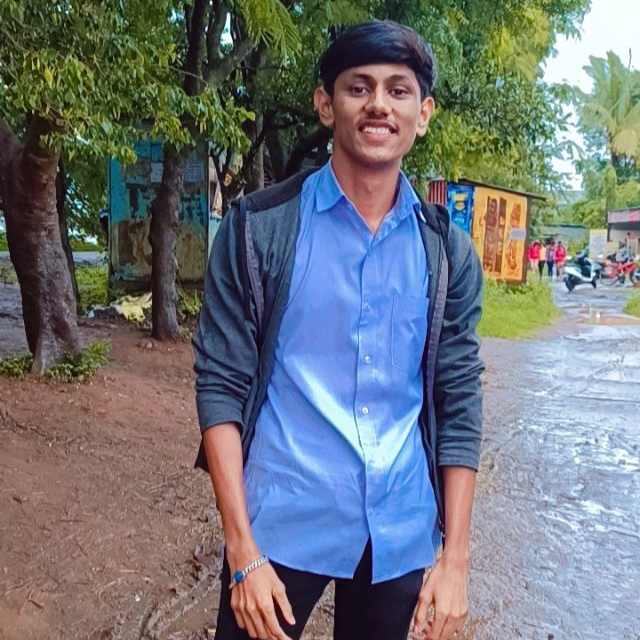
Table of contents
- #CodeMagicLaughs🦸♂️
- Introduction:
- 1. Set Interface:
- Definition:
- Purpose:
- Interface Hierarchy:
- Methods:
- Ordering and Duplication:
- Common Implementing Classes:
- Additional Concepts:
- Use Cases:
- 2. Queue Interface:
- Definition:
- Purpose:
- Interface Hierarchy:
- Methods:
- Order Processing:
- Common Implementing Classes:
- Additional Concepts:
- Use cases:
- Conclusion:
- Happy coding! 🚀✨
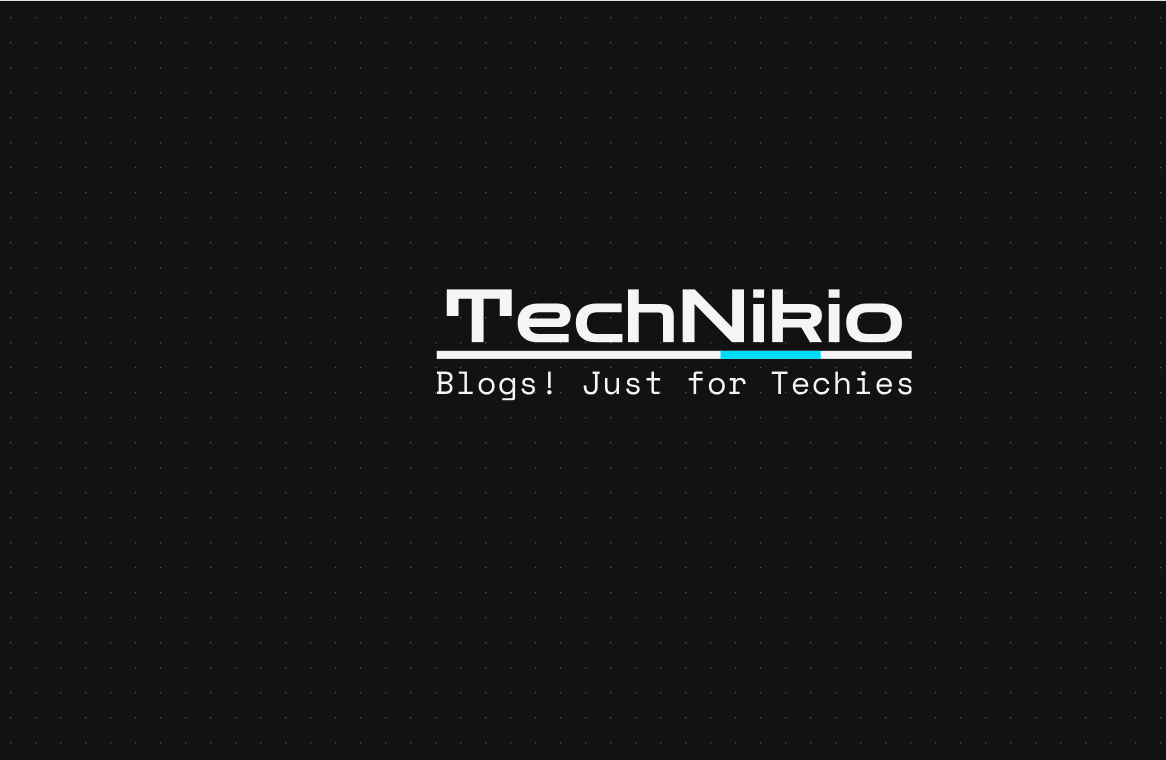
Welcome, fellow coders, to a thrilling exploration of Java Collections—a powerful framework that elevates data manipulation in the world of Java programming. In this chapter, we turn our focus to the Set interface & Queue interface, unraveling its capabilities and uncovering the magic of storing and manipulating ordered data. 🚀📚💻 #JavaCollections #ListInterfaceMagic
#CodeMagicLaughs🦸♂️
Introduction:
Java Collections form the backbone of data manipulation in Java programming. In this blog, we'll embark on a journey through two pivotal interfaces:
Set
andQueue
. Let's unravel their essence, exploring definitions, key implementations, purposes, interface hierarchy, methods, common implementing interfaces, and culminating with real-world use cases.
Let's go and explore them and move forward with our collection framework series.....🚀📚💻
1. Set Interface:
In the vast landscape of Java Collections, Sets stand out as champions of uniqueness. A Set does not allow duplicate elements, making it ideal for scenarios where distinct values matter. Key features include:
Uniqueness:
- Sets ensure that each element is unique.
No Specific Order:
- Elements in a Set have no defined order.
Dynamic Resizing:
- Sets dynamically adjust to accommodate varying elements.
Now, let's delve into the intricacies of Set interfaces with a real-world example.
Definition:
Extends the Collection Interface.
Represents a collection of unique elements where duplicate values are not allowed.
Does not maintain any order amongs its elements.
Purpose:
It a collection of Unique elements where same element can not be store again & again.
Ensures that no duplicates elements can be added to the set.
It does not preserve the insertion order of elements.
Interface Hierarchy:
Extends the Collection interface, providing collectoin behaviour along with set-specific operations.
sub interfaces include SortedSet and NavigableSet.
Set | Collection | Iterable
Methods:
Boolean add(E e)
Boolean remove(Object o)
Boolean contains(Object o)
boolean isEmpty()
int size()
void clear()
Ordering and Duplication:
Set do not maintain any order among their elements.
It uses a Hashtable as a underlying data structures.
It also does not show output in insertion order. It will sort the elements based on hashcode.
Common Implementing Classes:
- HashSet:
Implements the set interfaces using hash table for storage.
Offers fast access and efficient operations, but does not maintain order.
- Treeset:
Implements the SortedSet interface using Red Black Tree
Elements are stored in Sorted Order based on their natural ordering or a custom comparator.
- LinkedHashSet:
Maintains a doubly LinkedList along with a HashTable.
Provides predictable interation order, which is the order in which elements were inserted.
- EnumSet:
Specialised implementation for sets of enum elements.
Provides efficient storage and retrieval operations.
Additional Concepts:
- SortedSet Interface:
Extends the Set Interfaces to handle SortedSet.
Provides additional methods to deal witht he ordering of elements.
- NavigableSet Interface:
- Extends lower, floor, ceiling, higher & related methods for elements retrieval based on the ordering.
Use Cases:
Sets are widely used for tasks involving uniqueness of elements, such as storing a collection of unique user IDs, unique keys in databases.
Example:
import java.util.HashSet;
import java.util.Set;
public class SetExample {
public static void main(String[] args) {
// Creating a Set
Set<String> programmingLanguages = new HashSet<>();
// Adding elements
programmingLanguages.add("Java");
programmingLanguages.add("Python");
programmingLanguages.add("JavaScript");
programmingLanguages.add("Java"); // Ignored due to uniqueness
// Accessing elements
System.out.println("Programming Languages: " + programmingLanguages);
}
}
2. Queue Interface:
Queues introduce order and sequence into the Java Collections arena. Elements are processed in a first-in, first-out (FIFO) manner, mimicking a real-world queue. Key features include:
FIFO Processing:
- Elements are processed in the order they are added.
Dynamic Sizing:
- Queues dynamically adjust to varying elements.
Versatility in Implementations:
- Java offers various Queue implementations for diverse needs.
Let's witness the power of Queues in action with a hands-on example.
Definition:
The Queue interface is another gem in the Java Collections Framework, representing a collection designed for holding elements prior to processing.
Supports Operations like enqueue (add) & dequeue (remove) for elemens in a specific order. E.g. FIFO (First in First out).
Purpose:
Represents a collection for holding elements prior to processing.
Typically follows a First-in First-out (FIFO) order, where the element added first is the first to be processed.
Interface Hierarchy:
Queue interfaces extends the collection interface, providing additional methods specific to queues.
Core interfcaes include queue, Deque and Blocking Queue.
Queue | Collection | Iterable
Methods:
Boolean add(E e)
Boolean offer()
E remove()
E poll()
E element()
E peek()
Order Processing:
Queues follows a FIFO order for element processing.
Elements are added at the rear and removed from the front of the queue.
Common Implementing Classes:
- LinkedList:
Implements the Queue, Deque and List interface using a doubly LinkedList.
Supports efficient insertion and deletion operations.
- PriorityQueue:
Implementing the queue interface using a priority heap(Binary Heap).
Processes the elements based on their priority.
- ArrayDeque:
Implements the Deque and Queue using an array.
Provides efficient insertion and deletion at both ends of the queue.
- LinkedBlockingQueue:
An implementation of the BlockingQueue interface, which is designed for user in multithreaded environments.
Supports blocking operations, ensuring thread safety.
Additional Concepts:
- Deque Interface (Doubly Ended Queue):
Extends the Queue interface, allowing addition and removal of elements at both ends.
Provides methos for push,pop,peek and related operations.
Use cases:
Queues are essential for tasks that require managing and processing elements in a specific order, such as task scheduling, managing work items in a thred pool, and handling asynchronous requests.
Understanding the queue interfaces and their various implementing is crucal when dealing with ordered data and when maintaining the sequance of elements is vital to the functionality of your program.
Example:
import java.util.LinkedList;
import java.util.Queue;
public class QueueExample {
public static void main(String[] args) {
// Creating a Queue
Queue<String> tasks = new LinkedList<>();
// Enqueueing tasks
tasks.add("Task 1");
tasks.add("Task 2");
tasks.add("Task 3");
// Dequeueing tasks
while (!tasks.isEmpty()) {
System.out.println("Processing: " + tasks.poll());
}
}
}
Conclusion:
As we bid adieu to the riveting journey through the realms of Set and Queue interfaces, it's clear that these Java Collection superheroes bring order and uniqueness to the chaotic world of data management. From eliminating duplicates with finesse to orchestrating elements in a disciplined FIFO dance, Sets and Queues have proven their mettle.
The countdown begins—don't miss the thrill! 🚀💻 #JavaCollectionMagic #NextChapterUnleashed 🌈✨
#CodeMagicLaughs🦸♂️
Happy coding! 🚀✨
Subscribe to my newsletter
Read articles from Nikhil Abhiman Jadhav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
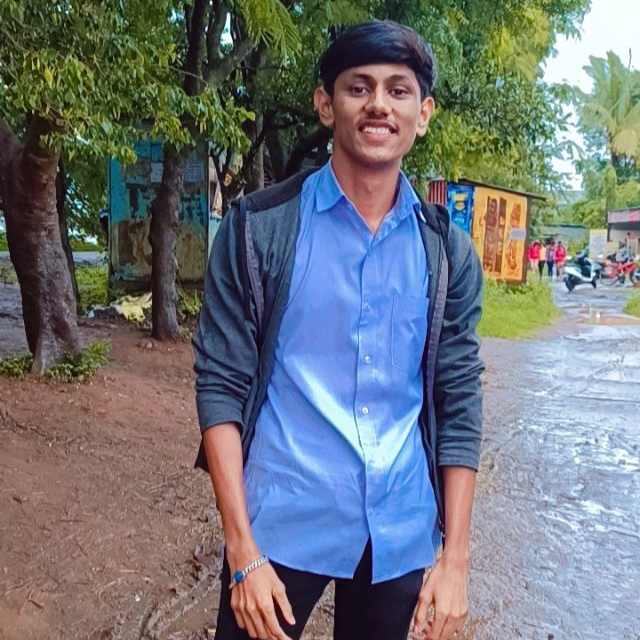
Nikhil Abhiman Jadhav
Nikhil Abhiman Jadhav
Hey there 👋🏻, I'm Nikhil Jadhav, a passionate tech enthusiast and aspiring writer on a mission to demystify the complexities of coding and technology. By day, I'm immersed in lines of code, and by night, I'm weaving words to make tech more accessible and enjoyable for everyone. 📬 Get in touch Twitter: https://twitter.com/Technikio LinkedIn:linkedin.com/in/nikhil-7571nik GitHub:github.com/jadhavnikhil2624