ZOD Library: Mastering Input Validation
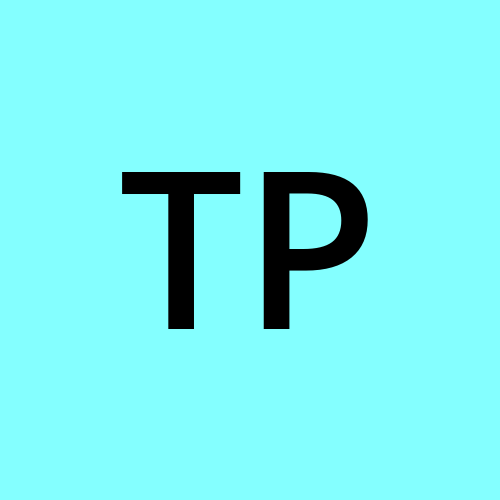
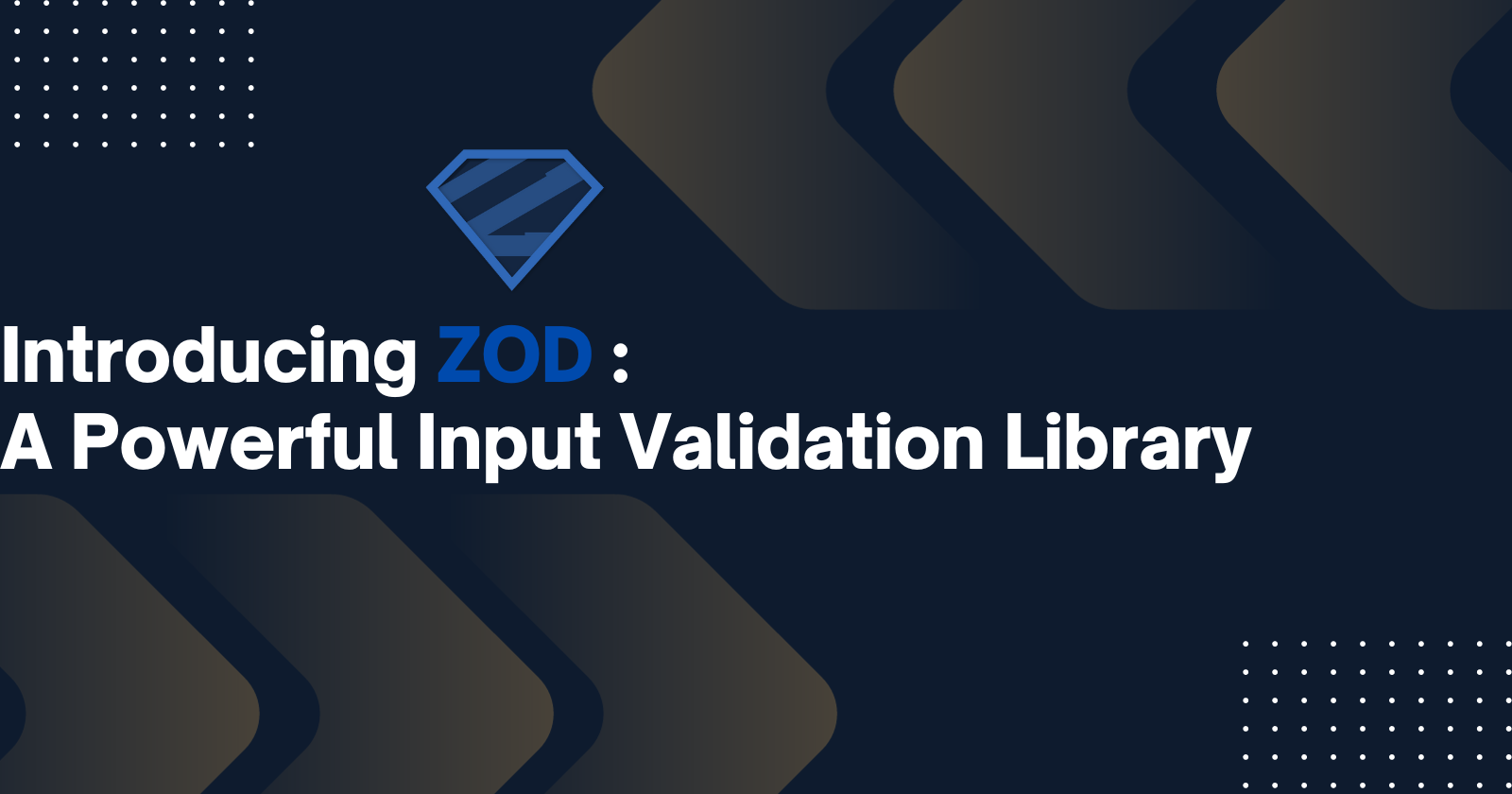
Before delving into ZOD, it's essential to understand the concepts of an input validator and schema.
Input Validator: The tool that ensures the data provided meets specified criteria or rules, helping maintain accuracy and integrity.
Schema: A schema is a blueprint or definition for the data structure and types.
What is ZOD?
Zod is a TypeScript-first schema declaration and validation library. By defining a const schema, you can easily parse and validate data, with Zod automatically inferring the static TypeScript type from the schema. The library supports various schema types, from basic strings and numbers to complex structures such as objects, arrays, and tuples. This versatility makes Zod a powerful tool for validating intricate data structures and generating form validation schemas.
While libraries like JOI and YUP are renowned, ZOD's unique combination of static type inference, expressive schema definition, custom error messages, and composable schemas positions it as a powerful choice for data validation.
Key Features are :
Static Type Inference: Zod automatically infers TypeScript types, enhancing code readability and maintainability.
Flexibility: Supports various data types and validation rules for diverse data structures.
Expressive Schema Definition: Enables concise and expressive schema definitions, facilitating the creation of complex schemas and reuse across code.
Custom Validation Rules: Allows the definition of custom validation rules for tailored data validation.
Error Messages: Provides the flexibility to customize error messages, enhancing the user experience with clear and contextual guidance.
Composable Schemas: Schemas can be composed to create more intricate structures, promoting easy reuse and customization for specific needs.
Installation
Just like any other library, installing ZOD is very easy the prerequisites are :
Node.JS & TypeScript 4.5+.
If the prerequisites are present :
npm install zod # npm
yarn add zod # yarn
bun add zod # bun
pnpm add zod # pnpm
If Node is not available we can use Deno. Deno relies on direct URL imports instead of a package manager like NPM
import { z as zod } from "https://deno.land/x/zod/mod.ts";
You can also specify a particular version:
import { z as zod } from "https://deno.land/x/zod@v3.16.1/mod.ts";
Parsing Data
.parse(_)
It throws an error if validation fails.
Suitable when you want to handle errors with try-catch blocks or other error-handling mechanisms.
safeParse(_)
Returns
ZodParseResult
object.Suitable when you prefer to check for errors without using try-catch, allowing for more control over error handling.
Both perform parsing and validation. Example :
const zod = require('zod');
const number = zod.number();
const result1 = number.parse(10); // 10
const result1 = number.parse("0"); // throws ZodError
const result2 = number.safeParse(10); // { success: true, data: 10 }
const result2 = number.safeParse("a"); // { success: false, error: [Getter] }
Zod Primitives || Dynamic Schemas
Number - Schema that validates numbers.
const zod = require('zod'); const number = zod.number(); const result = number.safeParse(10); // { success: true, data: 10 } const result2 = number.safeParse(); // { success: false, error: [Getter] }
String - Schema that validates strings.
const str = zod.string(); const result1 = str.safeParse("string"); // success: true, data: 'string' const result2 = str.safeParse(10); // success: false, error: [Getter]
Boolean - Schema that validates booleans.
const isBool = zod.boolean(); const result1 = isBool.safeParse(true); // success: true, data: true const result2 = isBool.safeParse("John");// success: false, error: [Getter]
Array - Schema that validates arrays.
const arr = zod.array(zod.string()); const result1 = arr.safeParse(["Name", "Name2"]); // success: true, data: [ 'Name', 'Name2' ] const result2 = arr.safeParse("Name");// success: false, error: [Getter]
Undefined - Schema that validates undefined.
const undef = zod.undefined(); const result1 = undef.safeParse(undefined); // success: true, data: undefined const result2 = undef.safeParse("Name");// success: false, error: [Getter]
Object -
Creates a schema for validating objects, specifying the structure with nested schemas for each property.
const obj = zod.object({
name: zod.string(),
age: zod.number(),
});
const result1 = obj.safeParse({ // success: true, data: { name: 'Name', age: 10 }
name: "Name",
age: 10,
});
const result2 = obj.safeParse("Name");// success: false, error: [Getter]
Conclusion
In summary, Zod emerges as a powerful and user-friendly schema validation library, offering developers an efficient and streamlined experience. With its simplicity, excellent error messages, rapid execution, and minimal bundle size, Zod presents a compelling alternative to established validation libraries like Joi and Yup. Whether you're a seasoned developer or a beginner, Zod stands out for its ease of use and performance, positioning it as a noteworthy choice for seamlessly integrating schema validation into your projects.
Subscribe to my newsletter
Read articles from T Prayag directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
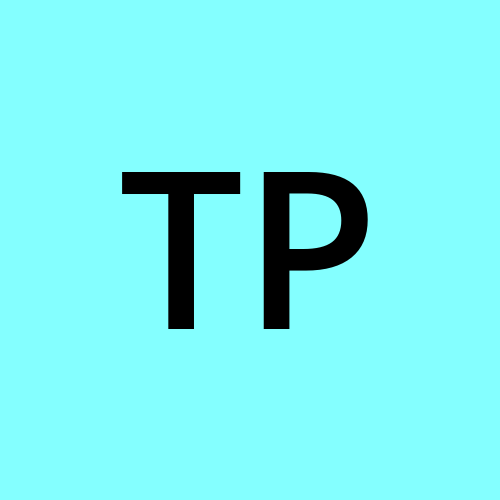