Array Methods (map, filter, reduce, forEach)
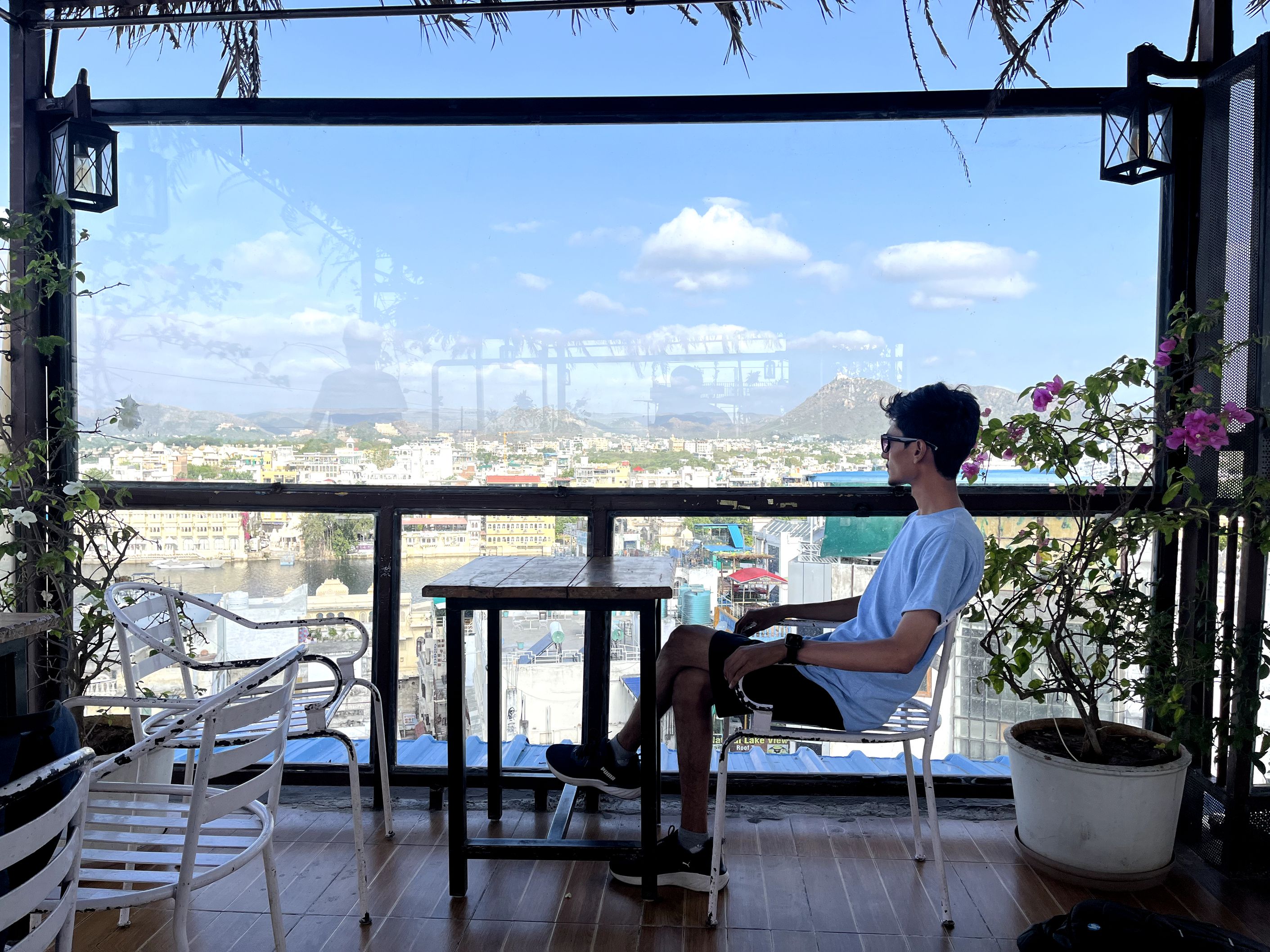
Expert-Level Explanation
Array methods in JavaScript are functions that can be used to perform operations on arrays. These include map
, filter
, reduce
, and forEach
, each serving a different purpose.
Creative Explanation
Think of array methods as different kitchen tools used to prepare a meal:
map
is like a knife that modifies each ingredient (element).filter
is like a sieve, separating wanted ingredients from unwanted ones.reduce
is like a blender, combining all ingredients into one final product.forEach
is like tasting each ingredient separately while cooking.
Practical Explanation with Code
let numbers = [1, 2, 3, 4, 5];
let doubled = numbers.map(number => number * 2); // [2, 4, 6, 8, 10]
let evens = numbers.filter(number => number % 2 === 0); // [2, 4]
let sum = numbers.reduce((total, number) => total + number, 0); // 15
numbers.forEach(number => console.log(number)); // 1 2 3 4 5
Real-world Example
Array methods are like different staff members in a warehouse:
map
is like a worker who repackages items.filter
is quality control, removing defective items.reduce
is the accountant, summarising all items' values.forEach
is like inspecting each item on the conveyor belt.
Subscribe to my newsletter
Read articles from Akash Thoriya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
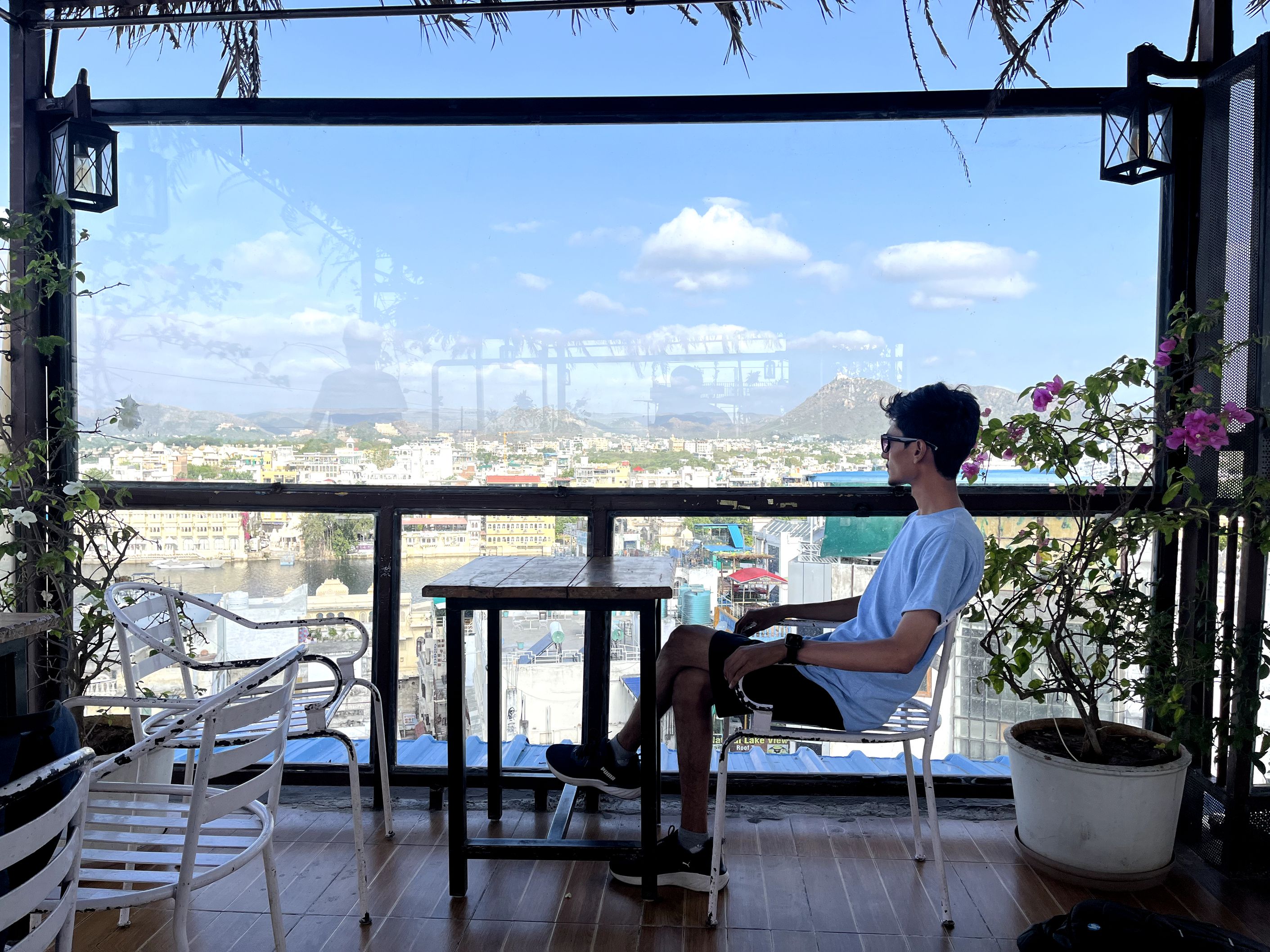
Akash Thoriya
Akash Thoriya
As a senior full-stack developer, I am passionate about creating efficient and scalable web applications that enhance the user experience. My expertise in React, Redux, NodeJS, and Laravel has enabled me to lead cross-functional teams and deliver projects that consistently exceed client expectations.