How Many Ways to Iterate Over an Object?
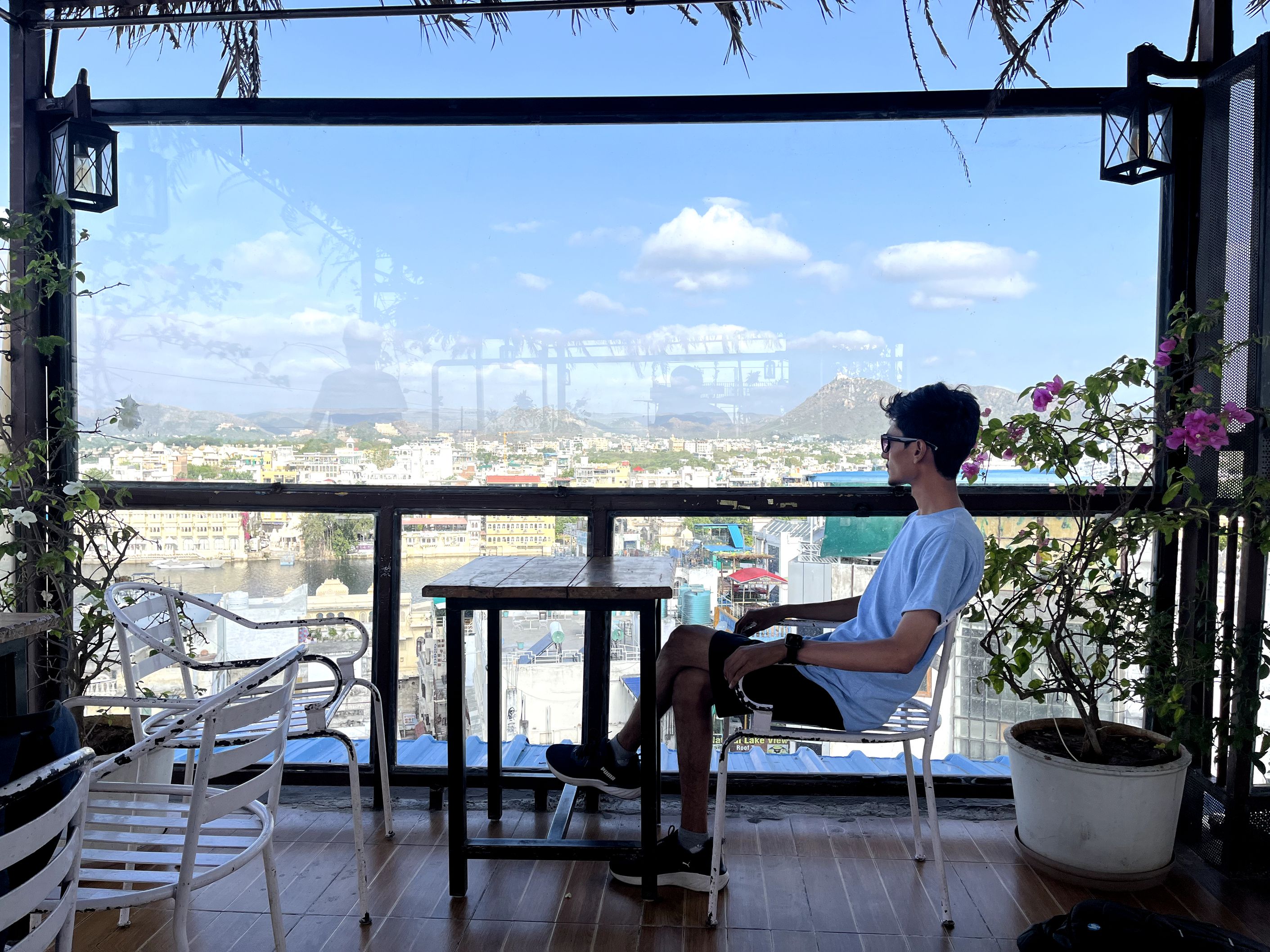
Expert-Level Explanation
In JavaScript, there are several ways to iterate over the properties of an object. These include:
for...in loop: Iterates over all enumerable properties of an object.
Object.keys(): Creates an array of an object's own enumerable property names and then iterates using array methods.
Object.values(): Similar to
Object.keys()
, but creates an array of values.Object.entries(): Provides an array of
[key, value]
pairs and can be used with array iteration methods.forEach with Object.keys/values/entries: Use
forEach
to iterate over the array returned by these methods.for...of with Object.keys/values/entries: Requires a conversion to an array format, then iterates over elements.
Creative Explanation
Think of iterating over an object like exploring a house.
for...in loop: walking through every room and noting details (properties).
Object.keys(): Making a list of room names and then visiting each.
Object.values(): listing what's in each room and then checking each item.
Object.entries(): Creating pairs of room names and contents, then reviewing each pair.
forEach with Object.keys/values/entries: Similar to above, but like following a tour guide who points out each item.
for...of with Object.keys/values/entries: turning the house exploration into a linear path and walking through it.
Practical Explanation with Code
const person = { name: 'Alice', age: 30 };
// for...in Loop
for (const key in person) {
console.log(key, person[key]);
}
// Object.keys()
Object.keys(person).forEach(key => console.log(key, person[key]));
// Object.values()
Object.values(person).forEach(value => console.log(value));
// Object.entries()
for (const [key, value] of Object.entries(person)) {
console.log(key, value);
}
Real-world Example
Iterating over an object in different ways is like conducting an inventory check in a store. Each method is a different approach to reviewing what items (properties) are in stock and their details (values).
Subscribe to my newsletter
Read articles from Akash Thoriya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
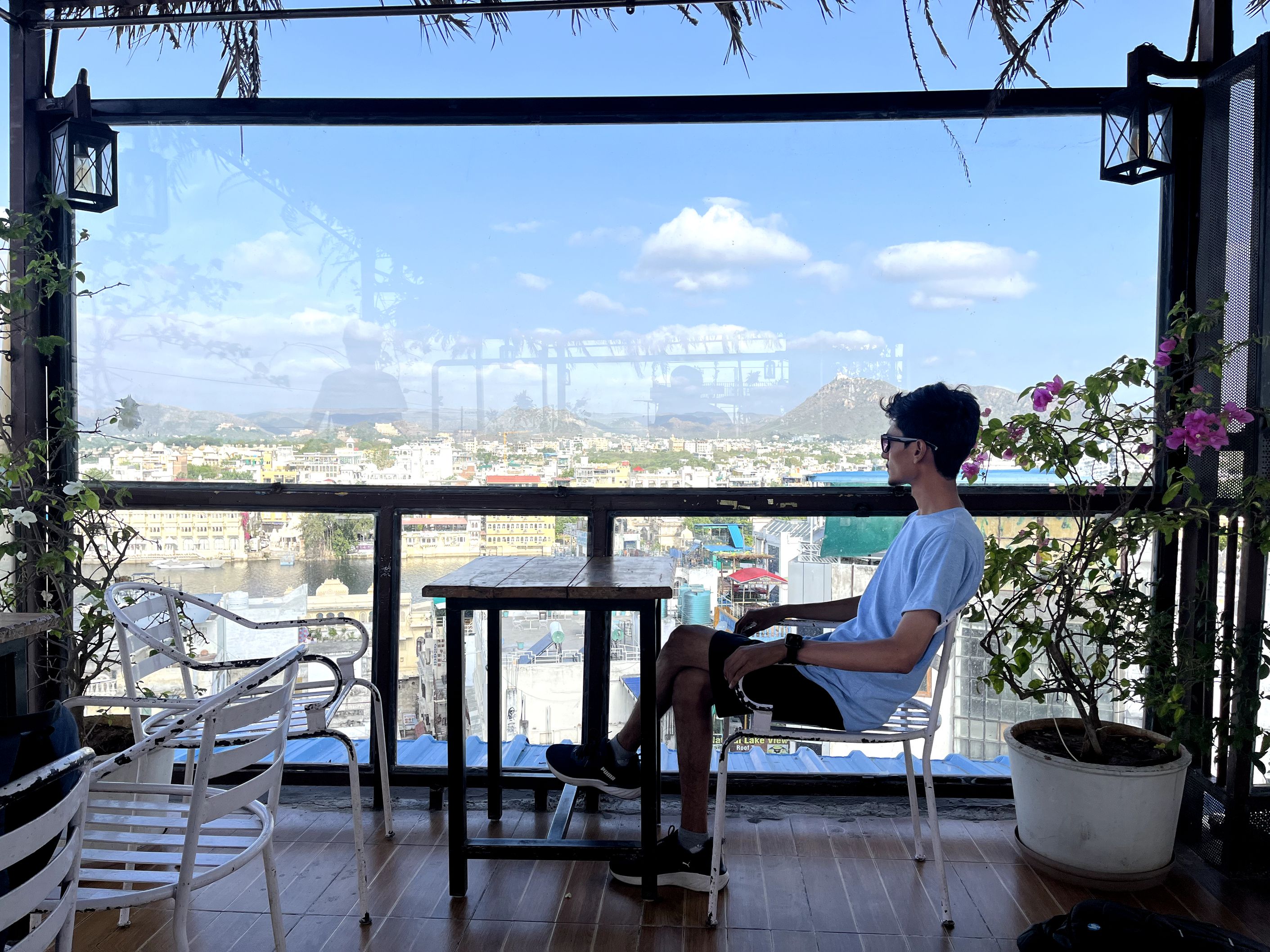
Akash Thoriya
Akash Thoriya
As a senior full-stack developer, I am passionate about creating efficient and scalable web applications that enhance the user experience. My expertise in React, Redux, NodeJS, and Laravel has enabled me to lead cross-functional teams and deliver projects that consistently exceed client expectations.