Exploring the Power of FlatList in React Native: Features, Use Cases and Implementation
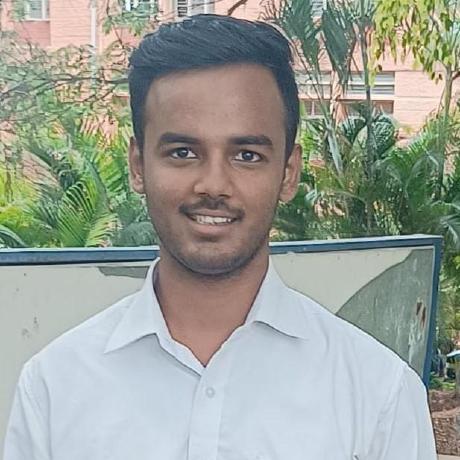
Table of contents
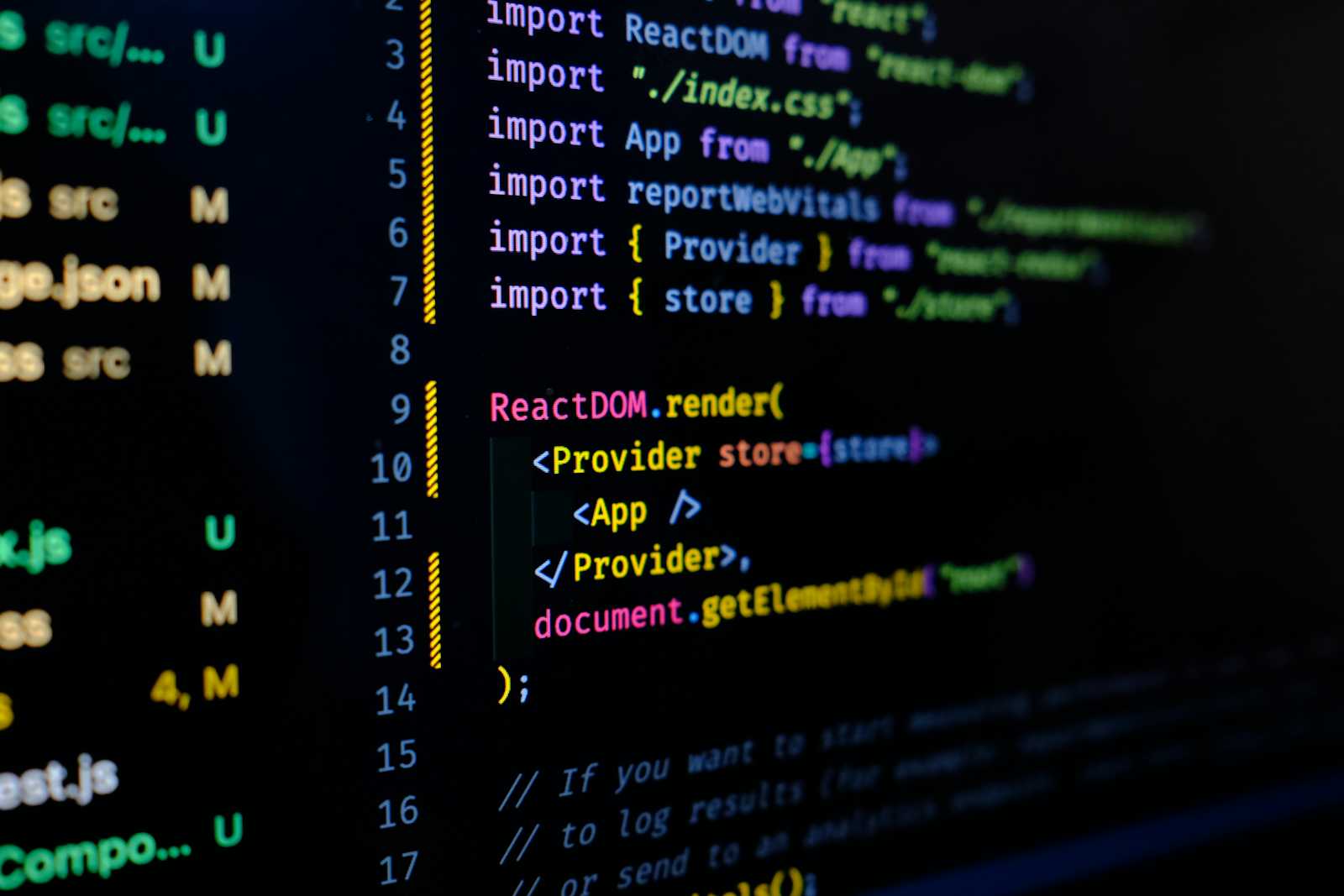
When efficiently displaying dynamic lists in React Native, FlatList emerges as a powerful and versatile component. Its ability to handle large datasets while maintaining optimal performance has made it a developer's go-to choice. In this blog post, we'll dive deep into the features that make FlatList indispensable and explore its various use cases.
Introduction to FlatList
React Native FlatList is a component that allows you to render lists with minimal hassle and minimal code. It is a performant way to render several highly customisable pieces of data. FlatList only shows the items that are currently visible on the screen since it uses a virtualized list. It facilitates enhanced performance.
FlatList is very easy to use and provides several helpful props and methods. This is an ideal choice for both novice and expert developers. There are several React Native benefits and real-life use case examples we will see in this post along with implementing it in a React Native application.
Top React Native FlatList Benefits:
Performance: FlatList optimizes rendering by only displaying the items that are currently visible on the screen. This virtualized rendering ensures smooth performance, even with extensive lists.
Dynamic Loading: Lazy loading and dynamic item loading enable FlatList to efficiently handle large datasets. It loads items on-demand, improving the app's responsiveness and reducing initial loading times.
Infinite Scrolling: Implementing infinite scrolling is seamless with FlatList. It dynamically loads more items as the user scrolls down, ensuring a continuous and engaging user experience without overwhelming the device's resources.
Network Requests and API Integration: FlatList seamlessly integrates with network requests and API calls. It simplifies the process of fetching and displaying data from external sources, making it an ideal choice for applications that rely on dynamic content.
Customization Options: The
renderItem
function in FlatList allows developers to customize the appearance of each item. This flexibility enables the creation of diverse and visually appealing list layouts tailored to the specific needs of the application.Responsive Design: FlatList adapts well to different screen sizes and orientations, ensuring a consistent and user-friendly experience across various devices. This responsiveness is crucial for creating versatile and adaptable mobile applications.
React Native Flatlist Use Cases
Now, let's see React Native FlatList use cases:
Displaying Lists of Items: The most fundamental use case for FlatList is displaying lists of items. Whether it's a list of products, messages, or any other type of data, FlatList efficiently handles rendering and scrolling through large datasets.
Infinite Scrolling: Implementing infinite scrolling is a common requirement in many apps. FlatList simplifies this process, allowing you to dynamically load more items as the user scrolls down, providing a seamless and continuous user experience.
News Feeds and Social Media Timelines: Applications with news feeds or social media timelines often require the efficient rendering of dynamic content. FlatList excels in this scenario by rendering only the visible items, optimizing performance for a smooth scrolling experience.
Grids and Multi-Column Layouts: FlatList is versatile and can be used to create grids and multi-column layouts. This is particularly useful when presenting images, products, or any other data that benefits from a grid-based arrangement.
Horizontal Scrolling Carousels: Creating horizontal scrolling carousels or sliders for showcasing featured content is another effective use case for FlatList. It allows users to swipe through a series of items in a visually appealing manner.
Dynamic Data from API Calls: When fetching data from an external API, FlatList streamlines the process of displaying dynamic content. It seamlessly integrates with network requests, making it an excellent choice for applications that rely on real-time or frequently updated data.
Implementation of FlatList in React Native
1) Prerequisites:
Before getting started, make sure you have the following prerequisites:
Node.js installed on your machine.
npm (Node Package Manager) or yarn installed.
A code editor of your choice (e.g., Visual Studio Code).
Basic knowledge of React Native and TypeScript.
Expo Go application installed in your mobile device
2) Setting Up Create React Native Expo App:
Why Am I choosing expo here? Because it is easy to get started with and also since we are just interested in learning about FlatList and not necessarily building an application.
To initiate a new React Native Expo app, follow these steps:
# Install Expo CLI globally
npm install -g expo-cli
# Create a new Expo project
expo init Flatlist-demo-app
After executing these two commands, it gives you prompt to select the template to choose from:
In my case, I am choosing the blank(Typescript) template (it is highlighted in bluish green). After you press enter, it starts downloading the starter template code and all the React Native dependencies required to setup the project. Be advised, it downloads all the dependencies in yarn package manager instead of NPM. After the downloading part, it gives you this prompt:
Your project is ready!
To run your project, navigate to the directory and run one of the following yarn commands.
- cd Flatlist-demo-app
- yarn start # you can open iOS, Android, or web from here, or run them directly with the commands below.
- yarn android
- yarn ios # requires an iOS device or macOS for access to an iOS simulator
- yarn web
As just following the instructions, to open the project in Text editor we use:
# navigate to react native application folder
cd Flatlist-demo-app
# Open up the application in you favorite Text Editor
code .
# The above command will open the application in text editor,
# to run the application, use the command:
yarn start
The execution of these commands will run the metro bundler and give you a scanner to open the application in your mobile device. To open the application in your mobile scan the QR Code through your expo application. Upon scanning the QR Code, the application will start running on your mobile device.
3) Fetching Data through an external API:
Now, let's fetch data from the Fake Store API. To get started, first clean up you app.tsx file:
App.tsx
import { StatusBar } from "expo-status-bar";
import { StyleSheet, Text, View } from "react-native";
import FlatlistDemo from "./components/FlatlistDemo";
export default function App() {
return (
<View style={styles.container}>
<FlatlistDemo />
</View>
);
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: "#fff",
alignItems: "center",
justifyContent: "center",
},
});
Create a folder named components
and create a file named FlatListDemo.tsx
and create a boilerplate code in that component:
FlatListDemo.tsx
import { StyleSheet, Text, View } from "react-native";
import React from "react";
const FlatlistDemo = () => {
return (
<View>
<Text>FlatlistDemo</Text>
</View>
);
};
export default FlatlistDemo;
const styles = StyleSheet.create({});
So now, when you run the application by using yarn start
it will show a 'FlatListDemo' at the center of the screen.
Now, let's proceed with the data fetching part in FlatListDemo.tsx
FlatListDemo.tsx
import { StyleSheet, Text, View } from "react-native";
import React, { useEffect, useState } from "react";
const FlatlistDemo = () => {
const [data, setData] = useState([]);
const fetchData = async () => {
const res = await fetch("https://fakestoreapi.com/products?limit=5");
const apiResData = await res.json();
setData(apiResData);
};
console.log("data", data);
useEffect(() => {
fetchData();
}, []);
return (
<View>
<Text>FlatlistDemo</Text>
</View>
);
};
export default FlatlistDemo;
const styles = StyleSheet.create({});
After copying this code, when you refresh the builder (ctrl+c
and yarn start
to restart the server). You should be able to data in you text editor's terminal suggesting the data has been fetched successfully.
4) Implementation of FlatList in React Native:
Now let's proceed with showing the data in a FlatList, the most important part of this blog.
FlatListDemo.tsx
import { StyleSheet, Text, View, FlatList, SafeAreaView } from "react-native";
import React, { useEffect, useState } from "react";
import { productType } from "../types";
const FlatlistDemo = () => {
const [data, setData] = useState<productType[]>([]);
const fetchData = async () => {
const res = await fetch("https://fakestoreapi.com/products");
const apiResData = await res.json();
setData(apiResData);
};
useEffect(() => {
fetchData();
}, []);
const renderItem = ({ item }: { item: productType }) => (
<Text style={styles.item}>{item.title}</Text>
);
return (
<SafeAreaView>
<View style={styles.container}>
<FlatList
numColumns={1}
data={data}
renderItem={renderItem}
keyExtractor={(item) => item.id}
/>
</View>
</SafeAreaView>
);
};
export default FlatlistDemo;
const styles = StyleSheet.create({
container: {
flex: 1,
marginTop: 40,
backgroundColor: "#fff",
alignItems: "center",
justifyContent: "center",
},
item: {
padding: 20,
marginVertical: 8,
marginHorizontal: 16,
backgroundColor: "#eee",
},
});
After copying this code, typescript compiler wont be happy and will start throwing error at unidentified types. So let's create a types file and write some types for our data. In last step, when we console logged the data, we could see what the data consisted of and based on that we create types for our data.
types.ts
export type productType = {
id: string;
title: string;
price: string;
description: string;
category: string;
image: string;
};
Since, this blog concentrates only on the FlatList implementation. I wont cover the styling of the card or the data. But I will explain the different pieces of the Flatlist component. Lets go:
<FlatList
numColumns={1}
data={data}
renderItem={renderItem}
keyExtractor={(item) => item.id}
/>
<FlatList />
Tag: It is a native component which is present in the corereact-native
package. So to use it, we need to import it from the package.numColumns={1}
: It is one of the many props provided by the FlatList to us, the functionality of this prop is to communicate to FlatList, the number of columns that it needs to render in one column. In our case, it is one.data
: Thedata
prop is where you provide the array of data that you want to render in the FlatList. In the example, it's the array of products fetched from the Fake Store API.keyExtractor
: ThekeyExtractor
prop is a function used to extract a unique key for each item in thedata
array. It helps React efficiently update and re-render the components. In this case, we use theid
property of each item as a key.keyExtractor={(item) => item.id}
renderItem
: TherenderItem
prop is a function that takes an item from thedata
array and returns a React element responsible for rendering the item. It receives an object with a structure{ item, index, separators }
const renderItem = ({ item }: { item: productType }) => ( <Text style={styles.item}>{item.title}</Text> );
In our case, we have keep it every simple. We are just taking the individual item from the products array and rendering the title on the screen.
Conclusion
In conclusion, FlatList in React Native emerges as a powerhouse for rendering dynamic lists efficiently. Its ability to handle large datasets, implement infinite scrolling, and optimize memory usage makes it a developer's favourite. With automatic key extraction, customization options, and smooth animations, FlatList offers flexibility and ease of use. To read more about FlatList, please click here
In our practical implementation, we fetched data from the Fake Store API, showcasing how to create an interactive list. As you delve into React Native development, consider FlatList's versatility for crafting responsive and engaging user interfaces. Its efficiency and features make it an invaluable asset for creating performant mobile applications. Explore, customize, and leverage FlatList to elevate your React Native projects.
For any query, you can get in touch with me via LinkedIn and twitter. Below you can find the code in GitHub Repository. Leave all your comments and suggestions in the comment section. Let's connect and share more ideas.
Happy coding!
Subscribe to my newsletter
Read articles from Gautham R Vanjre directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
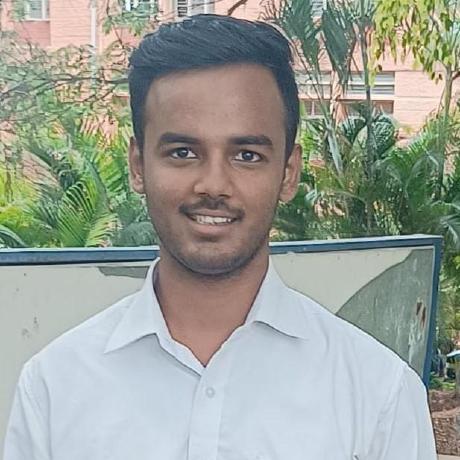
Gautham R Vanjre
Gautham R Vanjre
SDE-1 @HashedIn by Deloitte | Passionate about Web and Mobile Development | ๐ Turning ideas into digital solutions | #JavaScript #React #NodeJS #WebDev #Typescript #MobileDev ๐