Understanding TestNG Listeners
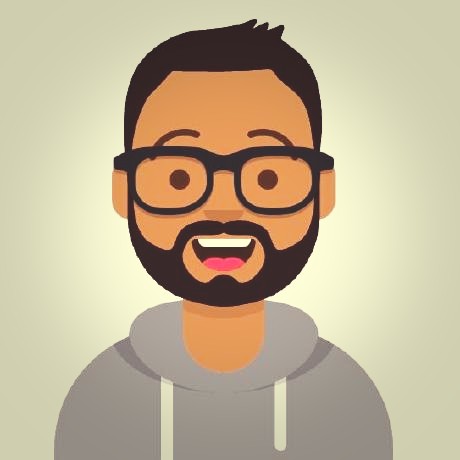
Introduction
TestNG, a widely-used testing framework for Java, provides a powerful mechanism known as "listeners" that allows customization and control over the test execution lifecycle. This blog post delves into the world of TestNG listeners, exploring their types, and use cases, and providing real-world examples to illustrate their practical applications.
What Are TestNG Listeners?
TestNG listeners are event-driven classes designed to intercept and respond to various events during the execution of test cases. They play a crucial role in enhancing test automation by providing a means to perform custom actions or validations at specific points in the test lifecycle.
Types of TestNG Listeners:
IInvokedMethodListener:
- Performs actions before and after each test method is invoked.
ITestListener:
- Tracks the overall test execution lifecycle, enabling actions before and after the entire test suite and each test method.
ISuiteListener:
- Performs actions before and after the entire suite is executed, enabling suite-level customization.
IReporter:
- Customizes HTML reporting of test results, allowing the generation of customized reports.
IAnnotationTransformer:
- Modifies annotations dynamically at runtime, enabling parameterization and flexibility in test execution.
IRetryAnalyzer:
- Implements custom logic for test retry mechanisms, allowing a test to be rerun based on specified conditions.
IConfigurationListener:
- Monitors configuration methods, providing hooks for actions before and after configuration methods are invoked.
IExecutionListener:
- Offers methods for executing tasks before and after the entire TestNG suite, providing a broader scope than suite-level listeners.
Real-World Examples:
1. Logging Test Start and End Times:
import org.testng.ITestContext;
import org.testng.ITestListener;
import org.testng.ITestResult;
public class TimeTrackerListener implements ITestListener {
@Override
public void onTestStart(ITestResult result) {
System.out.println("Test Started: " + result.getName());
}
@Override
public void onTestSuccess(ITestResult result) {
System.out.println("Test Passed: " + result.getName());
}
@Override
public void onTestFailure(ITestResult result) {
System.out.println("Test Failed: " + result.getName());
}
@Override
public void onTestSkipped(ITestResult result) {
System.out.println("Test Skipped: " + result.getName());
}
@Override
public void onStart(ITestContext context) {
System.out.println("Test Suite Started: " + context.getName());
}
@Override
public void onFinish(ITestContext context) {
System.out.println("Test Suite Finished: " + context.getName());
}
}
2. Retry Failed Tests:
import org.testng.IRetryAnalyzer;
import org.testng.ITestResult;
public class RetryAnalyzer implements IRetryAnalyzer {
private int maxRetryCount = 3;
private int retryCount = 0;
@Override
public boolean retry(ITestResult result) {
if (retryCount < maxRetryCount) {
System.out.println("Retrying test: " + result.getName());
retryCount++;
return true;
}
return false;
}
}
Configuring TestNG Listeners:
To use TestNG listeners, configure them in your test suite XML file or programmatically in your test classes. Here's an example XML configuration:
<!DOCTYPE suite SYSTEM "http://testng.org/testng-1.0.dtd">
<suite name="MyTestSuite">
<listeners>
<listener class-name="com.example.TimeTrackerListener"/>
<listener class-name="com.example.RetryAnalyzer"/>
</listeners>
<test name="MyTest">
<classes>
<class name="com.example.MyTestClass"/>
</classes>
</test>
</suite>
Conclusion
TestNG listeners empower developers to exert greater control over test automation processes, enabling custom actions, reporting, and retry mechanisms. By understanding the different types of listeners and their use cases, testers can tailor automation frameworks to meet specific project requirements. Happy Testing..!!
Subscribe to my newsletter
Read articles from Rinaldo Badigar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
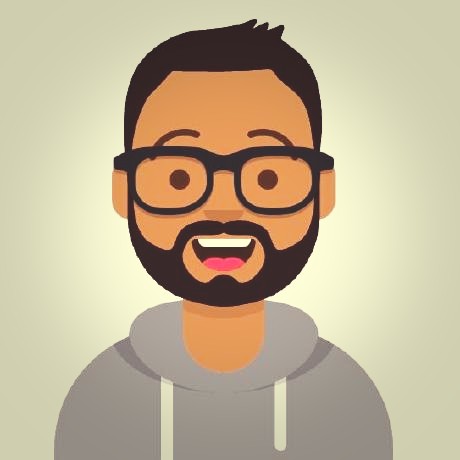
Rinaldo Badigar
Rinaldo Badigar
Software Engineer with 3+ years of experience in Automation, with a strong foundation in Manual, API, and Performance testing, ensuring high-quality software delivery for Ecommerce and Banking applications.