Optimizing React Applications with Dynamic Imports and Virtualization
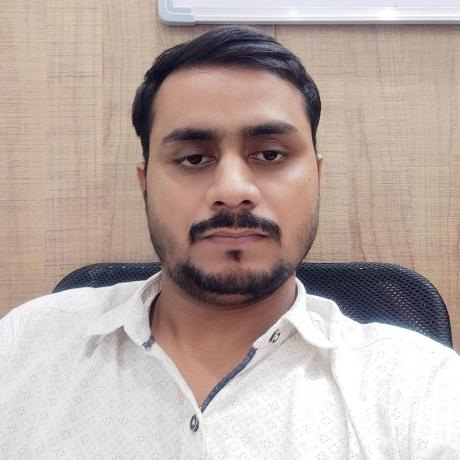
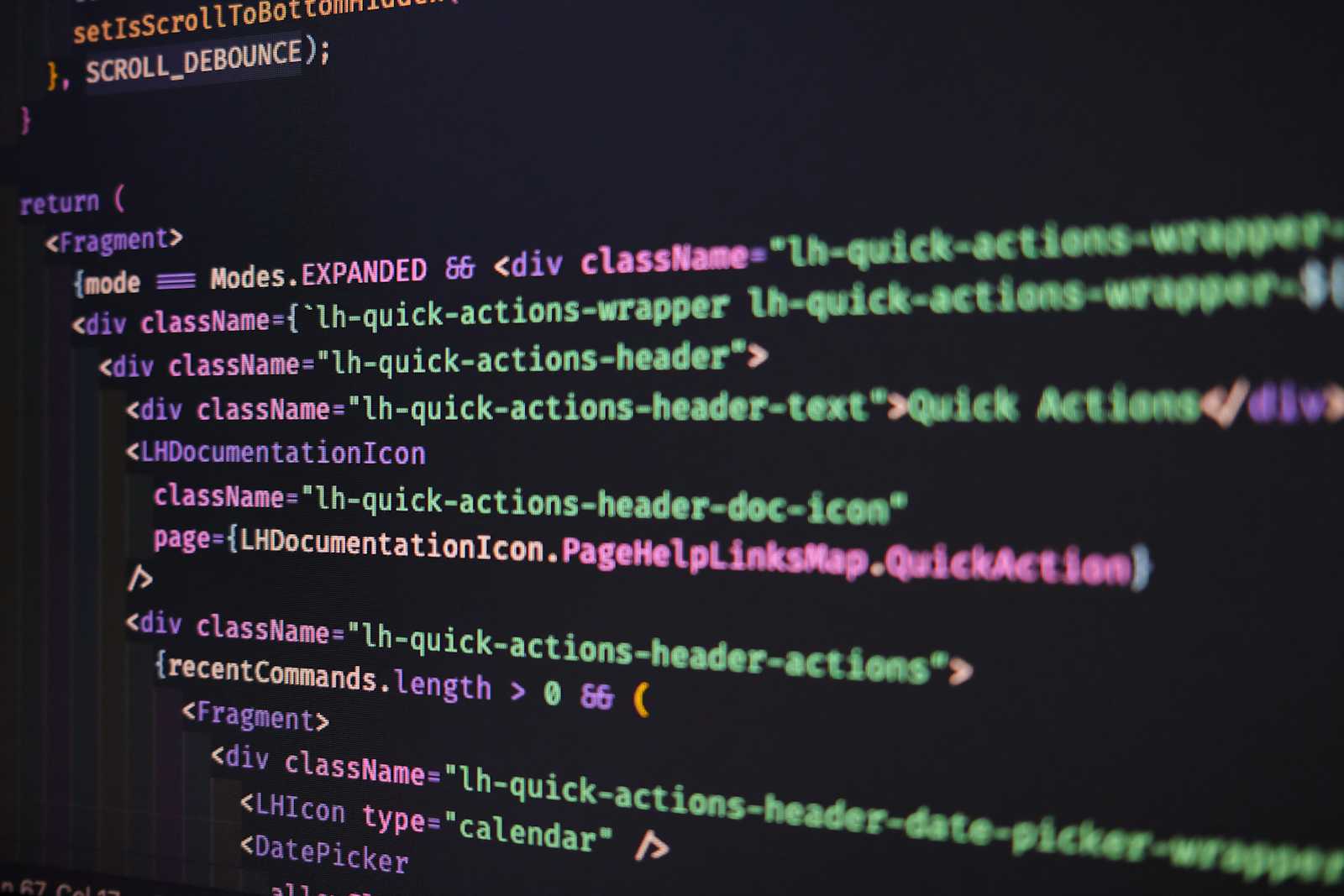
In web development, enhancing performance and user experience is key. React developers can achieve significant optimization by employing two techniques: dynamic imports and virtualization. These methods are particularly effective in applications that handle complex components or large datasets. Let's explore how to implement these techniques with a practical example.
Dynamic Imports in React
Understanding Dynamic Imports
Dynamic imports in React are a way to load components or modules only when they're needed. This approach is essential for reducing the initial load time of your application, which is crucial for both user engagement and SEO.
How Dynamic Imports Work
In React, dynamic imports are implemented using the import()
syntax. This instructs your bundler, typically Webpack, to split your code into separate chunks that are loaded asynchronously. It's particularly useful for larger components or libraries that aren't required immediately when the application loads.
Example Code:
Here's an example of how dynamic imports can be used in a React application:
import React, { useState } from 'react';
const App = () => {
const [DynamicComponent, setDynamicComponent] = useState(null);
const loadDynamicComponent = async () => {
const module = await import('./DynamicComponent');
setDynamicComponent(() => module.default);
};
return (
<div>
<h1>React Dynamic Import Example</h1>
<button onClick={loadDynamicComponent}>Load Dynamic Component</button>
{DynamicComponent && <DynamicComponent />}
</div>
);
};
export default App;
In this App
component, DynamicComponent
is not loaded until the user clicks the button. This is a practical use of dynamic imports, where the component is fetched and rendered on demand, thus optimizing the initial loading time.
Virtualization in React
What is Virtualization?
Virtualization is a technique to efficiently render only the visible items in a large list or dataset. This method is essential for preventing performance issues that arise from rendering a massive number of DOM nodes at once.
Implementing Virtualization
Virtualization can be implemented using libraries like react-window
. These libraries render items that fit in the user's viewport and dynamically load/unload items during scrolling, significantly reducing memory usage and improving performance.
Example Code:
Imagine you have a LargeListComponent
that needs to display a long list of items:
import { FixedSizeList as List } from 'react-window';
const LargeListComponent = ({ items }) => (
<List
height={500}
itemCount={items.length}
itemSize={35}
width={300}
>
{({ index, style }) => <div style={style}>{items[index]}</div>}
</List>
);
In this example, react-window
creates a list where only the items in view are rendered.
Integrating Dynamic Imports with Virtualization
Combining dynamic imports with virtualization can greatly enhance application performance. In this approach, the LargeListComponent
could be dynamically imported into the main application:
const loadLargeList = async () => {
const module = await import('./LargeListComponent');
setLargeList(() => module.default);
};
By doing this, the application initially loads faster because the heavy LargeListComponent
is not loaded until it's required. When it does load, it uses virtualization to efficiently render its large dataset.
Conclusion
Dynamic imports and virtualization are powerful tools in a React developer's arsenal for optimizing application performance. Dynamic imports reduce initial load times by loading components only when necessary, while virtualization ensures smooth rendering of large datasets. By integrating these techniques, developers can build faster, more efficient, and user-friendly web applications.
Subscribe to my newsletter
Read articles from Abhishek Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
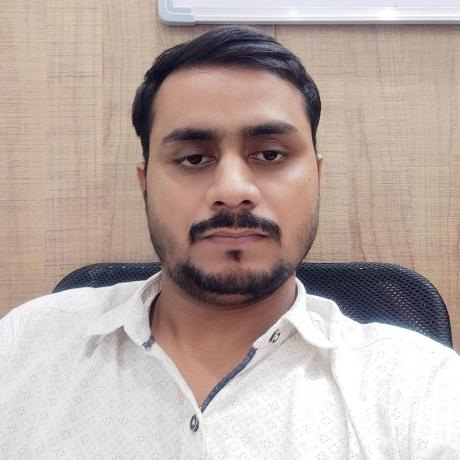