setTimeout and clearTimeout function

Table of contents
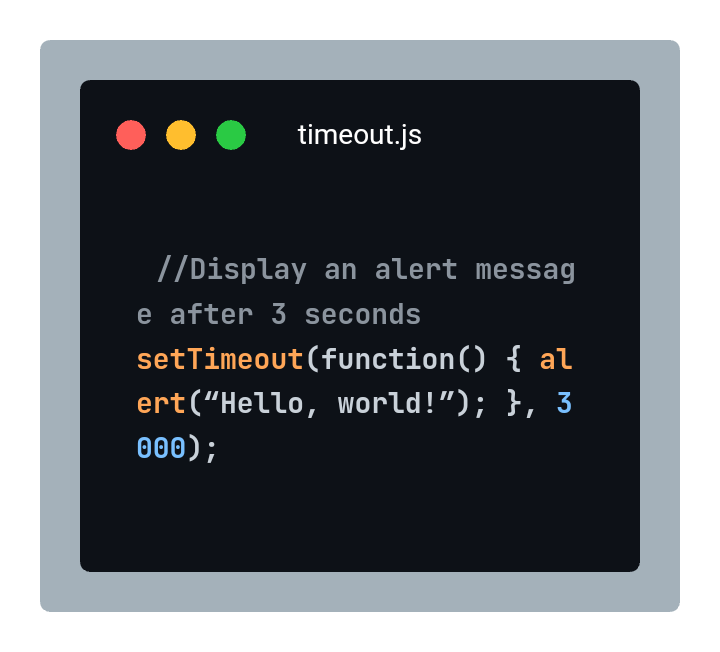
what are they?
The setTimeout function runs a function or a piece of code after waiting for a certain amount of time in milliseconds. It has three parameters: the function to run, the time to wait, and an optional callback function to run when the timeout is cleared. The setTimeout function gives an ID that can be used to stop the timeout later.
The clearTimeout function is used to cancel a timeout that was previously created by the setTimeout function. It takes one parameter: the ID of the timeout that you want to clear. If you don’t provide a valid ID, nothing will happen.
Here are some examples of how to use setTimeout and clearTimeout in JavaScript:
Example 1
//Display an alert message after 3 seconds
setTimeout(function() { alert(“Hello, world!”); }, 3000);
Example 2
//Display an alert message after 5 seconds and then display another message after another 2 seconds
setTimeout(function() { alert(“This is the first message.”); }, 5000);
setTimeout(function() { alert(“This is the second message.”); }, 10000);
Example 3
//Cancel a timeout that was created by
setTimeout var timerID = setTimeout(function() { console.log(“Hello, world!”); }, 2000); clearTimeout(timerID);
Example 4
//Cancel a timeout that was created by setTimeout with no callback
function var timerID = setTimeout(function() { console.log(“Hello, world!”);
},1000);
clearTimeout(timerID);
👍Thanks for reading.
Subscribe to my newsletter
Read articles from Babatunde Akintola directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Babatunde Akintola
Babatunde Akintola
I am a frontend Developer who just started out with JavaScript and wants to develop consistency through practice and sharing of knowledge and at the same time enhancing technical writing skills. Thank you.