Getting Started with Backend Development in Go: A Beginner's Guide
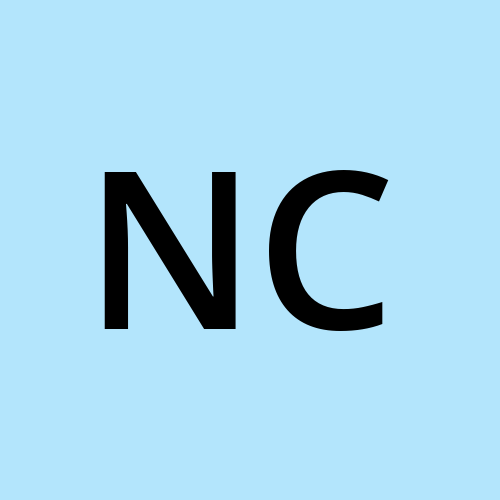
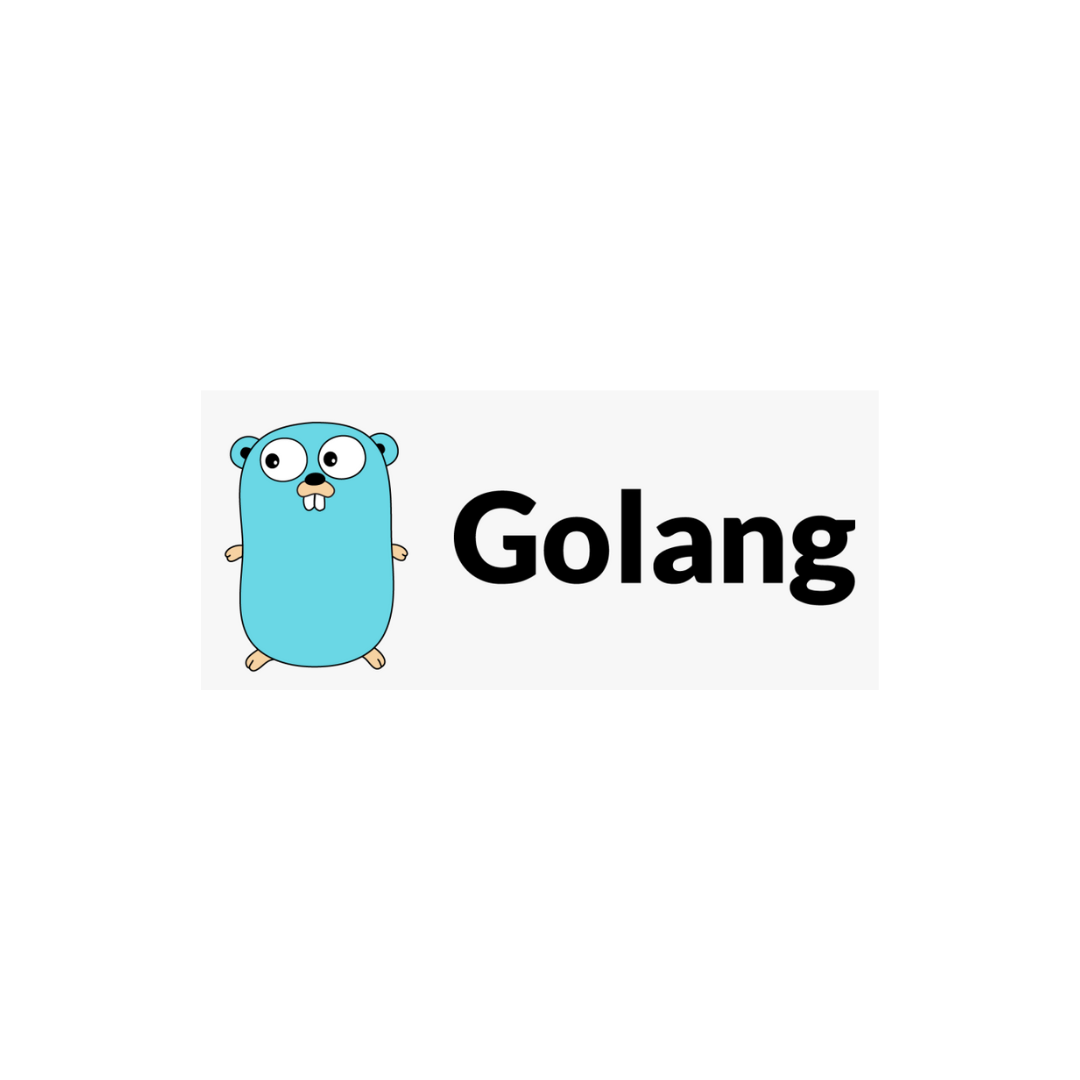
Introduction
Welcome to this beginner-friendly guide on creating a backend using Go lang! Go is a powerful programming language known for its simplicity, efficiency, and concurrency features. In this blog, we will explore the basics of setting up a Go backend and demonstrate some code examples along the way. So, let's dive in!
Prerequisites
Before we begin, make sure you have Go installed on your system. You can download the latest version of Go from the official website (https://golang.org) and follow the installation instructions based on your operating system.
Setting up the Project
To get started, let's create a new directory for our project. Open your terminal and navigate to the desired location.
$ mkdir backend-project
$ cd backend-project
Next, we need to initialize our project as a Go module. Run the following command:
$ go mod init github.com/your-username/backend-project
This command initializes a new Go module with a unique module path. Replace your-username
with your GitHub username or any other desired module path.
Creating the Server
Now that our project is set up, we can start writing our backend code. Create a new file called server.go
and open it in your favorite text editor.
package main
import (
"fmt"
"log"
"net/http"
)
func main() {
http.HandleFunc("/", handler)
log.Fatal(http.ListenAndServe(":8080", nil))
}
func handler(w http.ResponseWriter, r *http.Request) {
fmt.Fprint(w, "Hello, World!")
}
In the code above, we import the necessary packages, define a main function, and set up a basic HTTP server. The handler
function is responsible for handling incoming requests and writing a response to the client.
Running the Server
To run the server, open your terminal, navigate to the project directory, and execute the following command:
$ go run server.go
You should see the server start running on localhost
at port 8080
. Open your web browser and visit http://localhost:8080
. You should see the message "Hello, World!" displayed on the page.
Congratulations! You have successfully created your first Go backend server.
Building RESTful APIs
Now that we have a basic server set up, let's create a simple RESTful API using Go's net/http
package and the popular Gorilla Mux router.
First, install the Gorilla Mux package by running the following command:
$ go get -u github.com/gorilla/mux
Next, update the server.go
file with the following code:
package main
import (
"encoding/json"
"log"
"net/http"
"github.com/gorilla/mux"
)
type User struct {
ID string `json:"id"`
Username string `json:"username"`
Email string `json:"email"`
}
var users []User
func main() {
router := mux.NewRouter()
router.HandleFunc("/users", getUsers).Methods("GET")
router.HandleFunc("/users", createUser).Methods("POST")
log.Fatal(http.ListenAndServe(":8080", router))
}
func getUsers(w http.ResponseWriter, r *http.Request) {
json.NewEncoder(w).Encode(users)
}
func createUser(w http.ResponseWriter, r *http.Request) {
var newUser User
_ = json.NewDecoder(r.Body).Decode(&newUser)
users = append(users, newUser)
json.NewEncoder(w).Encode(newUser)
}
In the updated code, we define a User struct and create two API endpoints: /users
(GET) and /users
(POST). The getUsers
function returns the list of users in JSON format, and the createUser
function adds a new user to the users
slice.
Conclusion
In this beginner-friendly guide, we learned how to create a backend using Go lang. We covered setting up the project, creating a basic server, and building RESTful APIs. Go's simplicity and powerful standard library make it an excellent choice for backend development. Now that you have the basics down, feel free to explore more advanced topics and build exciting projects with Go!
Happy coding!
Subscribe to my newsletter
Read articles from Nirpendra Chaudhary directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
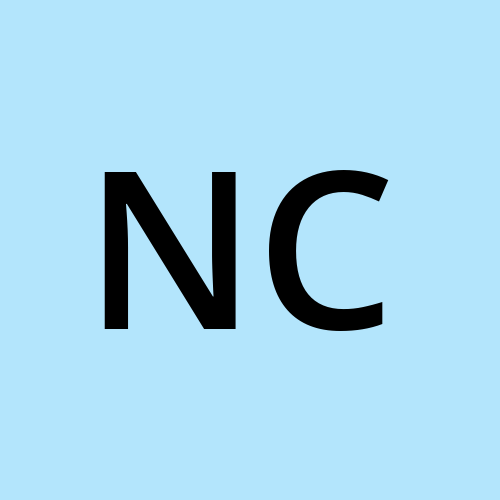