Understanding The Fundamentals Of Javascript.
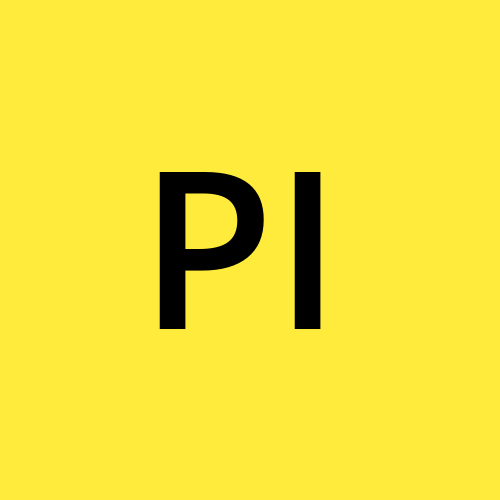
FUNDAMENTALS OF JAVASCRIPT; VARIABLES, OPERATORS, AND DATA TYPES.
In the vast realm of web development, JavaScript has become known as the cornerstone upon which websites are transformed into dynamic, engaging experiences. imagine a website being just static with no interactions. That is exactly what a website would look like without JavaScript. It is safe to say that it breathes life into a web. Now all these functionalities are possible through the fundamentals of javascript; variables, data types, and operators, which are the keys to unlocking the full potential of web development.
This article will unravel the fundamentals of JavaScript; variables, data types, operators, and the knowledge needed to master these fundamentals.
Variables In Javascript variables mean varieties just as the name implies. Variables act as containers that can be used in storing and manipulating data values. They enable the management of information during the execution of a script. They are declared using var
, let
, or const
, each with unique characteristics in terms of scope and mutability.
// Declaration using var
var x = 10;
// Declaration using let
let y = 5;
// Declaration using const
const pi = 3.14;
Here are some certain rules that should be noted while declaring a variable:
Any variable name must start with a letter (a to z or A to Z), underscore( _ ), or dollar( $ ) sign.
after the first letter, we can use digits (0 to 9), for example- abc1
javascript variables are case sensitive(x and X are different variables)
Understanding their differences in terms of scope and mutability is vital for writing effective and maintainable code.
var:
Variables declared
var
are function-scoped, meaning their scope is limited to the function in which they are declared.They are hoisted to the top of their function scope during the execution phase.
var
variables can be re-declared within the same scope without causing an error.
function exampleVar() {
if (true) {
var x = 10;
}
console.log(x); // Outputs 10
}
let:
let
provides block-scoping, confining the variable to the block, statement, or expression where it is defined.let
variables are not hoisted to the top of their scope.They cannot be re-declared within the same scope.
function exampleLet() {
if (true) {
let y = 5;
}
console.log(y); // ReferenceError: y is not defined
}
const:
Similar to
let
,const
is block-scoped and not hoisted.const
variables, however, are immutable, meaning their value cannot be reassigned after declaration.They must be initialized at the time of declaration.
function exampleConst() {
const pi = 3.14;
pi = 4; // TypeError: Assignment to constant variable.
}
Examples of Variable Declaration, Initialization, and Naming Conventions
Let's explore examples that illustrate the process of declaring variables, initializing them with values, and adhering to naming conventions:
// Declaration and initialization
let age = 23;
// Variable reassignment
age = 24;
// Naming conventions (camelCase)
let myVariable = 'example';
// Meaningful variable names
let totalAmount = 100;
let isUserLoggedIn = true;
Adhering to naming conventions and choosing appropriate variable names enhances code readability and maintainability, contributing to effective collaboration and understanding of the codebase. Understanding these subtle differences ensures the usage of the right tool for the job promoting best practices in js programming.
Operators in JavaScript
Operators are fundamental elements that perform operations on variables and values. They are necessary for manipulating data, and calculations, and making decisions by utilizing actions like arithmetic calculations, logical comparisons, and more.
Arithmetic Operators:
Arithmetic operators perform basic mathematical operations.
Examples:
let a = 4;
let b = 3;
let sum = a + b; // Addition
let difference = a - b; // Subtraction
let product = a * b; // Multiplication
let quotient = a / b; // Division
let remainder = a % b; // Modulus (remainder)
Comparison Operators:
Comparison operators are used to compare values, resulting in a boolean (true/false) outcome.
Examples:
let a = 4;
let b = 3;
console.log(a > b); // Greater than
console.log(a < b); // Less than
console.log(a === b); // Equal to (strict equality)
console.log(a !== b); // Not equal to
Logical Operators:
Logical operators perform logical operations and return a boolean result.
Examples:
let l = true;
let w = false;
console.log(l && w); // Logical AND
console.log(l || w); // Logical OR
console.log(!l); // Logical NOT
Assignment Operators:
Assignment operators are used to assign values to variables.
Examples:
let num = 10;
num += 5; // Equivalent to num = num + 5
num -= 3; // Equivalent to num = num - 3
num *= 2; // Equivalent to num = num * 2
Other Operators:
JavaScript includes various other operators, such as:
Unary operators: Operate on a single operand.
Ternary operator (Conditional operator): A shorthand for an if-else statement.
// Unary operator
let value = 5;
console.log(-value); // Unary negation
// Ternary operator
let isEven = (value % 2 === 0) ? 'Even' : 'Odd';
Understanding and mastering these operators is crucial for writing efficient and expressive JavaScript code. They form the foundation for performing diverse tasks.
Data Types
This helps specify the type of data to be stored or manipulated within a program. mainly, there are two types of data types:
Primitive.
Non-Primitive.
Primitive Data Type: they hold data one at a time. they include;
Strings:
Strings are ideal for representing textual data, such as names, messages, or any sequence of characters and are enclosed in single (' ') or double (" ") quotes.
Examples:
let message = 'Good, Morning!';
let name = "Precious";
Numbers:
Numbers are Used for numeric calculations, measurements, or any quantitative data and they can be integers or floating-point numbers.
Examples:
let age = 23;
let pi = 3.14;
Booleans:
Booleans represent true or false values and are often used for logical operations.
Examples:
let isTrue = true;
let isFalse = false;
null:
null
Explicitly denotes the absence of a value or object.It is often used to signify that a variable should have no value or that a function deliberately returns no value.
Example:
let emptyValue = null;
undefined:
undefined
is a primitive value that is automatically assigned to variables that have been declared but not initialized.It is also the default return value of functions that do not explicitly return anything.
Example:
let notDefined;
console.log(notDefined); // Outputs: undefined
Non-Primitive Data Type: They can hold collections of values and even complex entities. It includes;
- Object: These are collections of key-value pairs, where keys are strings or symbols. Example:
let person = {
name: 'Precious',
age: 23,
};
- Array: They are ordered list of values, accessible by index. Example:
let numbers = [1, 2, 3, 4, 5];
- Function: These are reusable blocks of code that can be invoked with a set of parameters. Example:
function add(a, b) {
return a + b;
};
Understanding the nuances of these data types is foundational to writing robust and efficient JavaScript code. Whether manipulating text, performing calculations, or making logical decisions, selecting the appropriate data type is crucial for effective and accurate programming.
Conclusion
A comprehensive grasp of JavaScript's foundational elements—variables, operators, and data types—is essential for web developers aiming to create dynamic and engaging websites. The meticulous understanding of variable distinctions (var, let, const) and their scope, coupled with mastery over operators for diverse data manipulations, ensure code that is not only functional but also expressive and maintainable. Additionally, the strategic selection of appropriate data types, whether primitive or non-primitive, is pivotal in crafting accurate and robust code, allowing developers to manipulate data with precision. In essence, these fundamentals serve as the cornerstone of effective web development, providing the tools and knowledge necessary for creating innovative and seamless online experiences.
As web development continually evolves, a strong foundation in JavaScript basics becomes indispensable for developers navigating the ever-changing landscape. Proficiency in these fundamental aspects empowers developers to go beyond mere functionality, enabling them to create websites that captivate and engage users in the dynamic realm of modern web development.
Subscribe to my newsletter
Read articles from precious ikegbo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
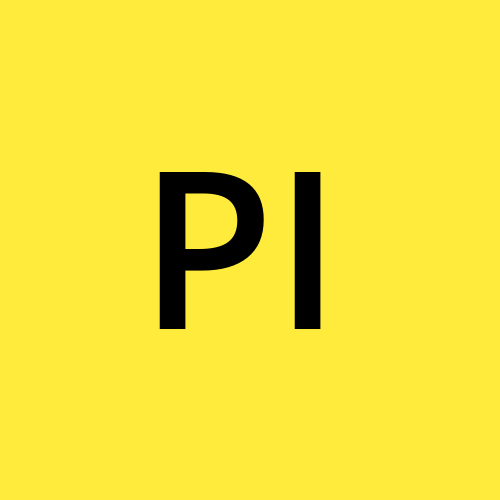