Extent Reports & Screenshot Integration with TestNG Listeners
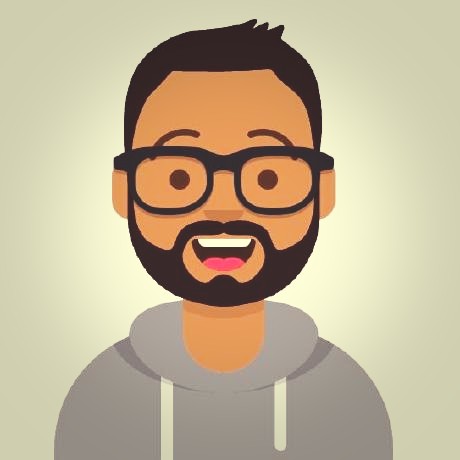
What are Extent Reports?
Extent Reports is an open-source reporting library for Java, designed to create interactive and detailed HTML-based reports for test executions. It is widely used in the Selenium WebDriver community to generate comprehensive and visually appealing reports. Extent Reports simplifies the process of tracking and analyzing test results, making it an essential tool for testers.
Key Features of Extent Reports
Interactive Dashboards
Detailed Test Logs
Screenshots and Media Attachments
Parallel Test Execution Support
Implementing Extent Reports
Step 1: Add Extent Reports Dependency
Make sure you have the Extent Reports dependency in your project's pom.xml
file:
<dependency>
<groupId>com.aventstack</groupId>
<artifactId>extentreports</artifactId>
<version>5.0.9</version>
</dependency>
Step 2: Create an EventListener Class for Screenshots and Logs
Create an EventListener class and extend it to the BaseClass (assuming your driver is present here) and implement the ITestListener interface which contains all the listener methods.
import java.io.File;
import java.io.IOException;
import org.apache.commons.io.FileUtils;
import org.openqa.selenium.OutputType;
import org.openqa.selenium.TakesScreenshot;
import org.openqa.selenium.WebDriver;
import org.testng.ITestContext;
import org.testng.ITestListener;
import org.testng.ITestResult;
import com.aventstack.extentreports.ExtentReports;
import com.aventstack.extentreports.ExtentTest;
import com.aventstack.extentreports.Status;
import com.aventstack.extentreports.markuputils.ExtentColor;
import com.aventstack.extentreports.markuputils.MarkupHelper;
import com.aventstack.extentreports.reporter.ExtentSparkReporter;
import com.aventstack.extentreports.reporter.configuration.Theme;
import com.mystore.base.BaseClass;
public class EventListener extends BaseClass implements ITestListener {
ExtentSparkReporter htmlReporter;
ExtentReports reports;
ExtentTest test;
public void configureReport() {
htmlReporter = new ExtentSparkReporter("Report.html");
reports = new ExtentReports();
reports.attachReporter(htmlReporter);
htmlReporter.config().setDocumentTitle("Demo Report");
htmlReporter.config().setReportName("First Name : DemoName");
htmlReporter.config().setTheme(Theme.DARK);
}
// ScreenShot Logic
public static void captureScreenshot(WebDriver driver, String screenshotName) {
// Convert WebDriver object to TakesScreenshot
TakesScreenshot ts = (TakesScreenshot) driver;
// Capture screenshot as File
File source = ts.getScreenshotAs(OutputType.FILE);
// Define destination for the screenshot
String destination = System.getProperty("user.dir") + "\\ScreenShot\\" + screenshotName + ".png";
File target = new File(destination);
try {
// Copy file to the destination
FileUtils.copyFile(source, target);
} catch (IOException e) {
e.printStackTrace();
}
}
@Override
public void onTestStart(ITestResult result) {
configureReport();
System.out.println("Test Started: " + result.getName());
}
@Override
public void onTestSuccess(ITestResult result) {
System.out.println("Test Passed: " + result.getName());
}
@Override
public void onTestFailure(ITestResult result) {
test = reports.createTest(result.getName());
test.log(Status.FAIL, MarkupHelper.createLabel("Name of failed test" + result.getName(), ExtentColor.RED));
captureScreenshot(driver, result.getName());
test.addScreenCaptureFromPath("File path of screenshot" + result.getName() + ".png", result.getName());
System.out.println("Test Failed: " + result.getName());
}
@Override
public void onTestSkipped(ITestResult result) {
System.out.println("Test Skipped: " + result.getName());
}
@Override
public void onStart(ITestContext context) {
System.out.println("Test Suite Started: " + context.getName());
}
@Override
public void onFinish(ITestContext context) {
System.out.println("Test Suite Finished: " + context.getName());
reports.flush();
}
}
Step 3: Configure the testing.xml file
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE suite SYSTEM "https://testng.org/testng-1.0.dtd">
<suite name="Suite">
<listeners>
<listener class-name="EventListner"></listener>
</listeners>
<test name="Test">
<classes>
<class name="com.mystore.testcases.LoginTest"/>
</classes>
</test> <!-- Test -->
</suite> <!-- Suite -->
Step 4: Run the testing.xml file
Right-click on the testing.xml file and Click on "Run As" the select "TestNG Suite".
If you want to check out the entire source code of the project click here.
Conclusion
Integrating Extent Reports and screenshots enriches TestNG reporting, fostering better collaboration and providing a holistic view for quicker issue resolution. Upgrade your testing suite for more informative and actionable results.
Subscribe to my newsletter
Read articles from Rinaldo Badigar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
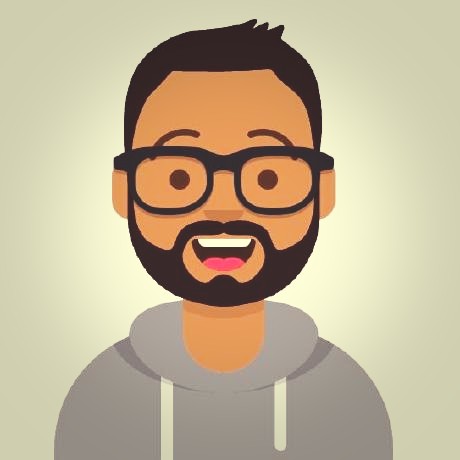
Rinaldo Badigar
Rinaldo Badigar
Software Engineer with 3+ years of experience in Automation, with a strong foundation in Manual, API, and Performance testing, ensuring high-quality software delivery for Ecommerce and Banking applications.