How to make a To-Do List App in JavaScript
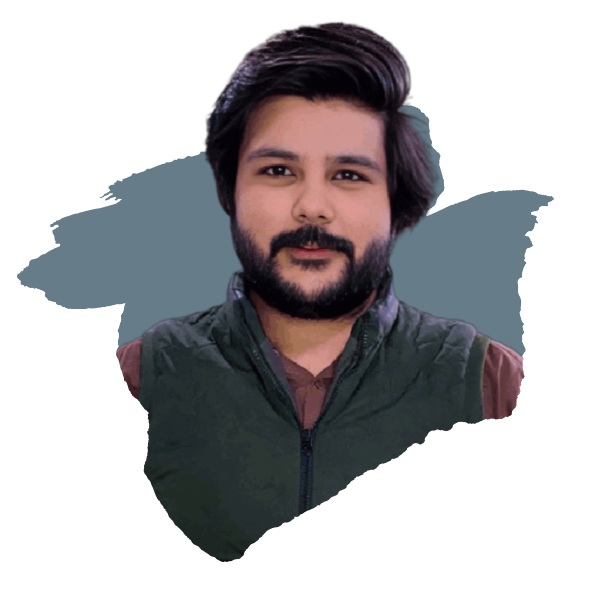
Table of contents
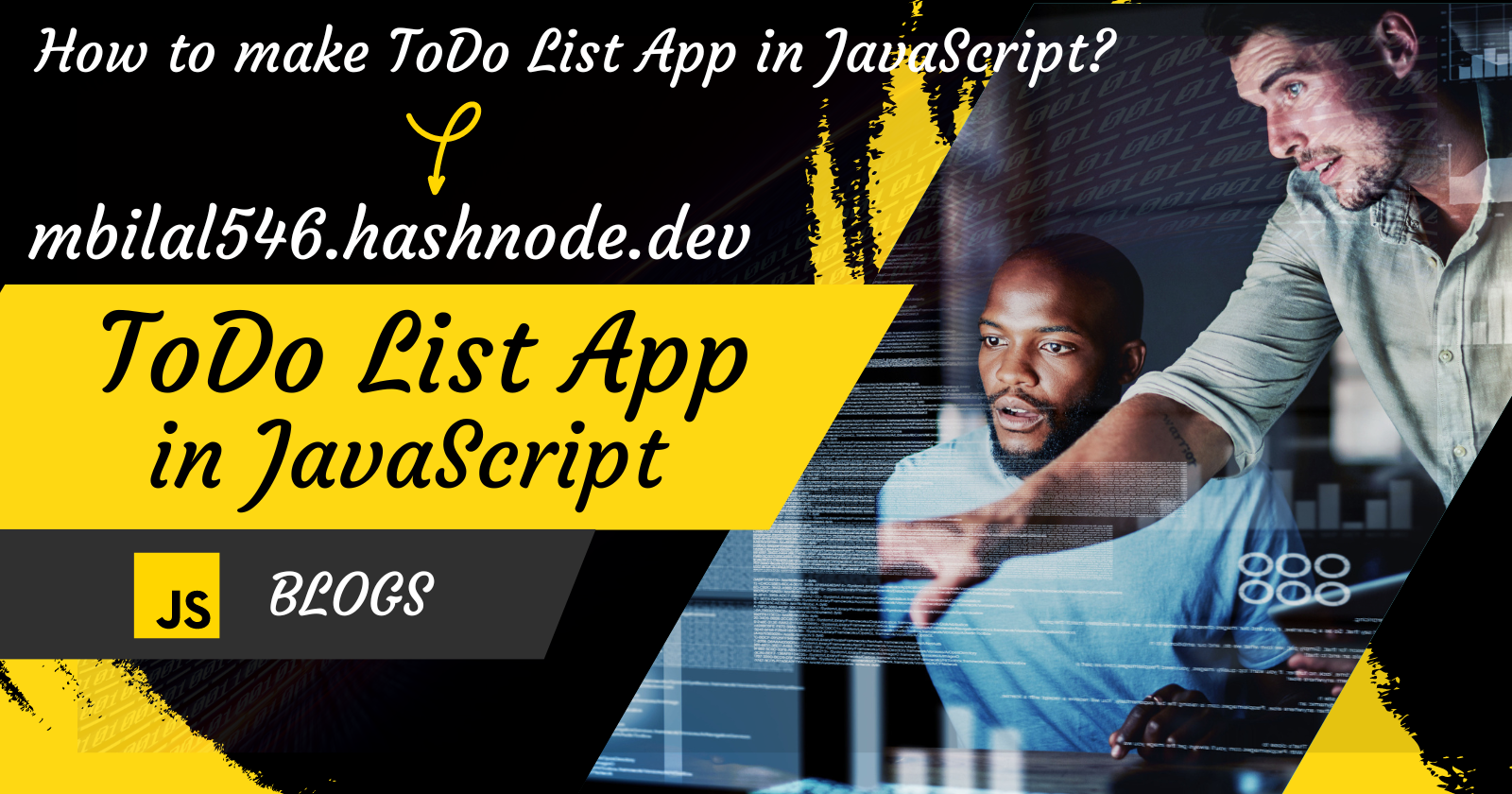
OUTPUT of the given code
Use the following code:
HTML code.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>TODO List App</title>
// Use this if you use seprate css
<link rel="stylesheet" href="style.css">
</head>
<body>
<h1>TODO LIST</h1>
<section class="todo-section">
<h1>Your Plan for today ?</h1>
<div class="todo-div">
<input type="text" id="todo-input" placeholder="Add Your Task Of Today">
<input type="button" value="Add To List" class="btn">
</div>
<ul class="todo-list"></ul>
</section>
// Use this if you use seprate JS file
<script src="script.js"></script>
</body>
</html>
CSS code.
body{
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: Arial, Helvetica, sans-serif;
text-align: center;
color: white;
background-color: black;
display: flex;
flex-direction: column;
align-items: center;
}
.todo-section{
border: 2px solid yellow;
width: 60vw;
min-height: 100vh;
text-align: center;
border-radius: 10px;
}
#todo-input,.btn,.todo-btn{
height: 50px;
border-radius: 5px;
outline: none;
border: none;
font-size: 16px;
font-weight: bold;
}
#todo-input{
width: 80%;
padding-left: 10px;
}
.btn,.todo-btn{
width: 120px;
color: white;
background-color: rgba(255, 0, 0, 0.521);
cursor: pointer;
}
.todo-list li{
width: 95%;
list-style: none;
display: flex;
justify-content: space-between;
font-weight: bold;
font-size: 18px;
padding: 5px;
align-items: center;
}
.todo-btn{
width: 100px;
}
.green{
background-color: green;
}
JavaScript code.
let addBtn = document.querySelector('.btn');
let todoInput = document.querySelector('#todo-input');
let todoList = document.querySelector('.todo-list');
addBtn.addEventListener('click', (e)=>{
e.preventDefault();
const newTodoText = todoInput.value;
const newLi = document.createElement('li');
newLi.innerHTML = `
<h3 class="todo-text">${newTodoText}</h3>
<div class="todo-buttons">
<button class="todo-btn green">Done</button>
<button class="todo-btn red">Remove</button>
</div>
`;
if (todoInput.value === '') {
alert('Please enter some task here !')
}
else{
todoList.append(newLi);
}
todoInput.value = '';
});
todoList.addEventListener('click', (e)=>{
if (e.target.classList.contains('green')) {
let lineTrough = e.target.parentNode.previousElementSibling;
lineTrough.style.textDecoration = 'line-through';
lineTrough.style.color = 'green';
}
if (e.target.classList.contains('red')) {
let removeElem = e.target.parentNode.parentNode;
removeElem.remove();
}
});
Subscribe to my newsletter
Read articles from Muhammad Bilal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
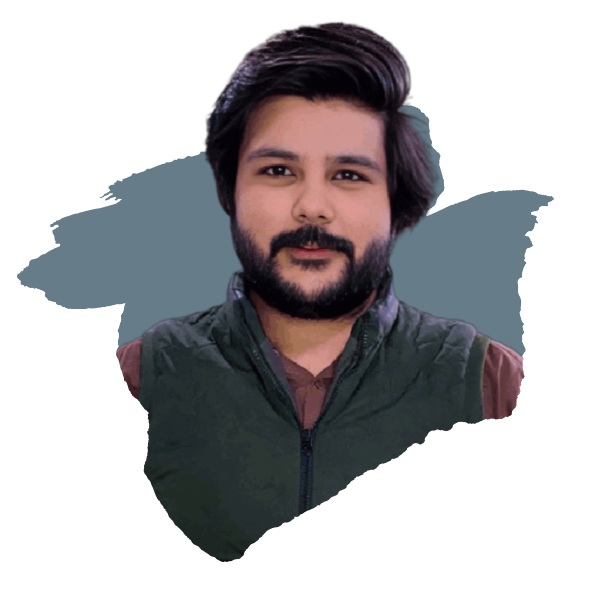
Muhammad Bilal
Muhammad Bilal
With a passion for creating seamless and visually appealing web experiences, I am Muhammad Bilal, a dedicated front-end developer committed to transforming innovative ideas into user-friendly digital solutions. With a solid foundation in HTML, CSS, and JavaScript, I specialize in crafting responsive and intuitive websites that captivate users and elevate brands. ๐ Professional Experience: In my two years of experience in front-end development, I have had the privilege of collaborating with diverse teams and clients, translating their visions into engaging online platforms. ๐ Skills: Technologies: HTML5, CSS3, JavaScript, WordPress Responsive Design: Bootstrap, Tailwind CSS, Flexbox, and CSS Grid Version Control: Git, GitHub ๐ฅ Projects: I have successfully contributed to projects where my focus on performance optimization and attention to detail played a crucial role in delivering high-quality user interfaces. โจ Continuous Learning: In the fast-paced world of technology, I am committed to staying ahead of the curve. Regularly exploring new tools and methodologies, I am dedicated to enhancing my skills and embracing emerging trends to provide the best possible user experience. ๐ Education: I hold a BSc degree in Software Engineering from Virtual University, where I gained both technical skills and knowledge. ๐ฅ Let's Connect: I am always open to new opportunities, collaborations, and discussions about the exciting possibilities in front-end development. Feel free to reach out via LinkedIn, Instagram, Facebook, Twitter, or professional email.