Day 63: Understanding Terraform Variables and Data Types

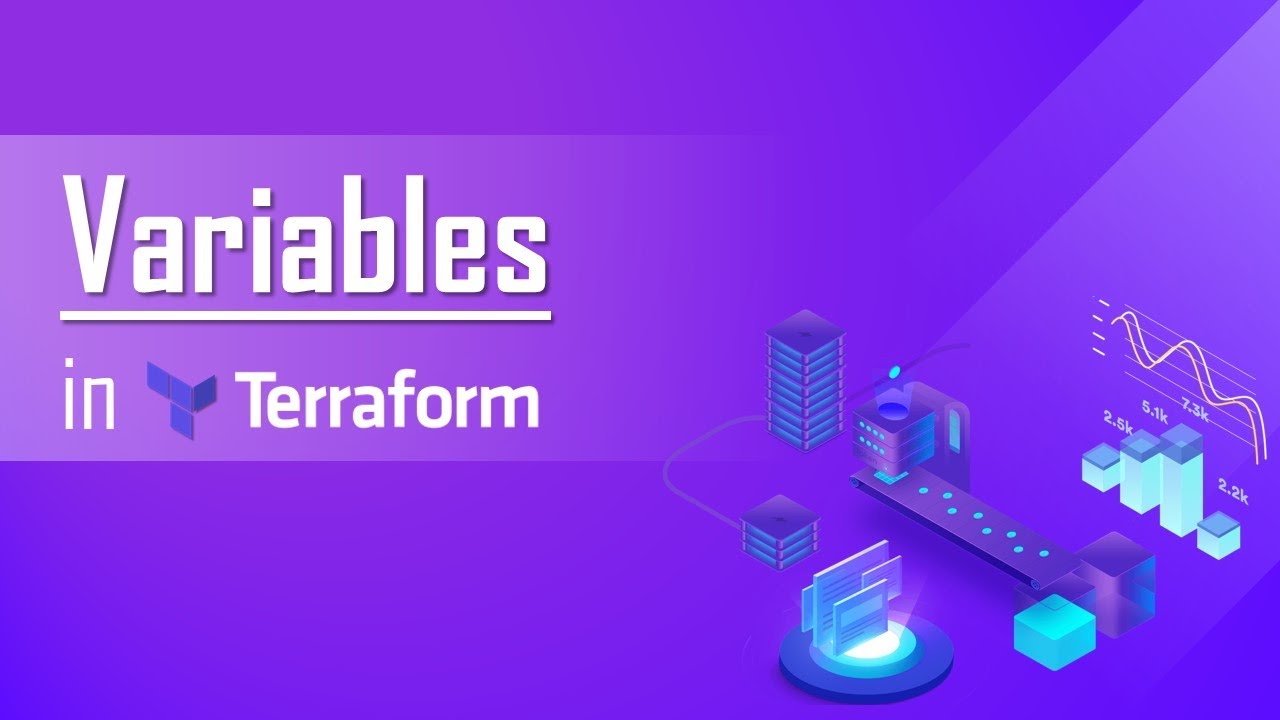
Terraform's efficacy lies in its ability to represent infrastructure as code (IaC) through declarative configurations. Central to this paradigm are Terraform variables and data types, which provide a means to parameterize and structure these configurations effectively.
Terraform Variables
Variables in Terraform serve as placeholders for dynamic values, facilitating configuration flexibility and reusability. They enable the decoupling of configuration details from the main Terraform code, allowing for customization without modifying the underlying infrastructure definitions.
Key Characteristics of Terraform Variables:
Parameterization: Variables allow users to parameterize Terraform configurations, enabling the definition of values outside the configuration files.
Scoping: Variables can be scoped at different levels within a Terraform project, including global, module, and local scopes, providing granular control over their usage.
Type Constraints: Terraform supports various data types for variables, ensuring type safety and validation during configuration execution.
Terraform Data Types
Terraform offers a range of data types to represent different kinds of values within configurations. Understanding these data types is crucial for structuring and organizing configuration data effectively.
Common Terraform Data Types Include:
Primitive Types: These include string, number, boolean, and null, providing fundamental data representations within Terraform configurations.
Complex Types:
Maps: Maps are collections of key-value pairs, allowing for the organization of structured data. They are particularly useful for configuring resources with multiple attributes.
Lists: Lists represent ordered collections of values, enabling the definition of sequences of items within configurations. They are often used for configuring arrays of resources or properties.
Sets: Sets are unordered collections of unique values, ensuring that each element appears only once within the set. They are beneficial for defining distinct sets of values within configurations.
Objects: Objects encapsulate multiple attributes into a single entity, providing a cohesive structure for representing complex data. They facilitate the modeling of hierarchical or composite configurations.
Practical Implementation
To solidify the theoretical understanding, let's embark on practical tasks to demonstrate the application of Terraform variables and data types.
Task 1: Utilizing Map Data Type
Define Map Variables:
variable "file_contents" { type = map default = { "statement1" = "this is cool" "statement2" = "this is cooler" } }
Create Local File Resources:
resource "local_file" "devops" { for_each = var.file_contents filename = "/path/to/${each.key}.txt" content = each.value }
Run Terraform Commands:
terraform init terraform apply
Review Output: Verify the creation of local files with dynamic content based on the map variables.
Task 2: Demonstrating List, Set, and Object Data Types
Define Variables:
variable "list_example" { type = list(string) default = ["item1", "item2", "item3"] } variable "set_example" { type = set(string) default = ["item1", "item2", "item3"] } variable "object_example" { type = object({ key1 = string key2 = number }) default = { key1 = "value1" key2 = 123 } }
Use Variables in Terraform Configuration:
output "list_output" { value = var.list_example } output "set_output" { value = var.set_example } output "object_output" { value = var.object_example }
Run Terraform Commands:
terraform init terraform apply
Review Output: Examine the outputs showcasing the usage of list, set, and object data types within Terraform configurations.
Conclusion
Terraform variables and data types are indispensable tools for crafting flexible, maintainable, and scalable infrastructure configurations. By leveraging these constructs effectively, users can abstract configuration details, enhance reusability, and streamline infrastructure management processes within Terraform projects.
Subscribe to my newsletter
Read articles from Vedant Thavkar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vedant Thavkar
Vedant Thavkar
"DevOps enthusiast and aspiring engineer. Currently honing skills in streamlining development workflows and automating infrastructure. Learning AWS, Docker, Kubernetes, Python, and Ansible. Eager to contribute and grow within the DevOps community."