Understanding Creational Design Patterns in Java: A Deep Dive into Factory and Abstract Factory Patterns
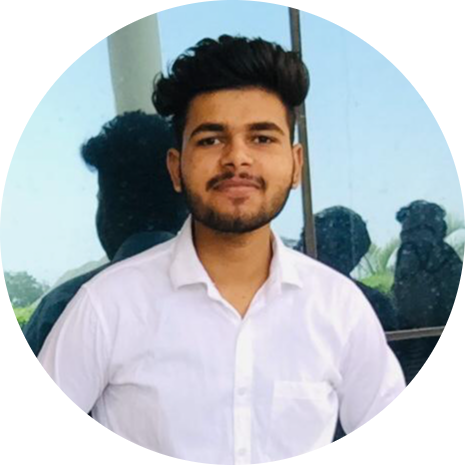
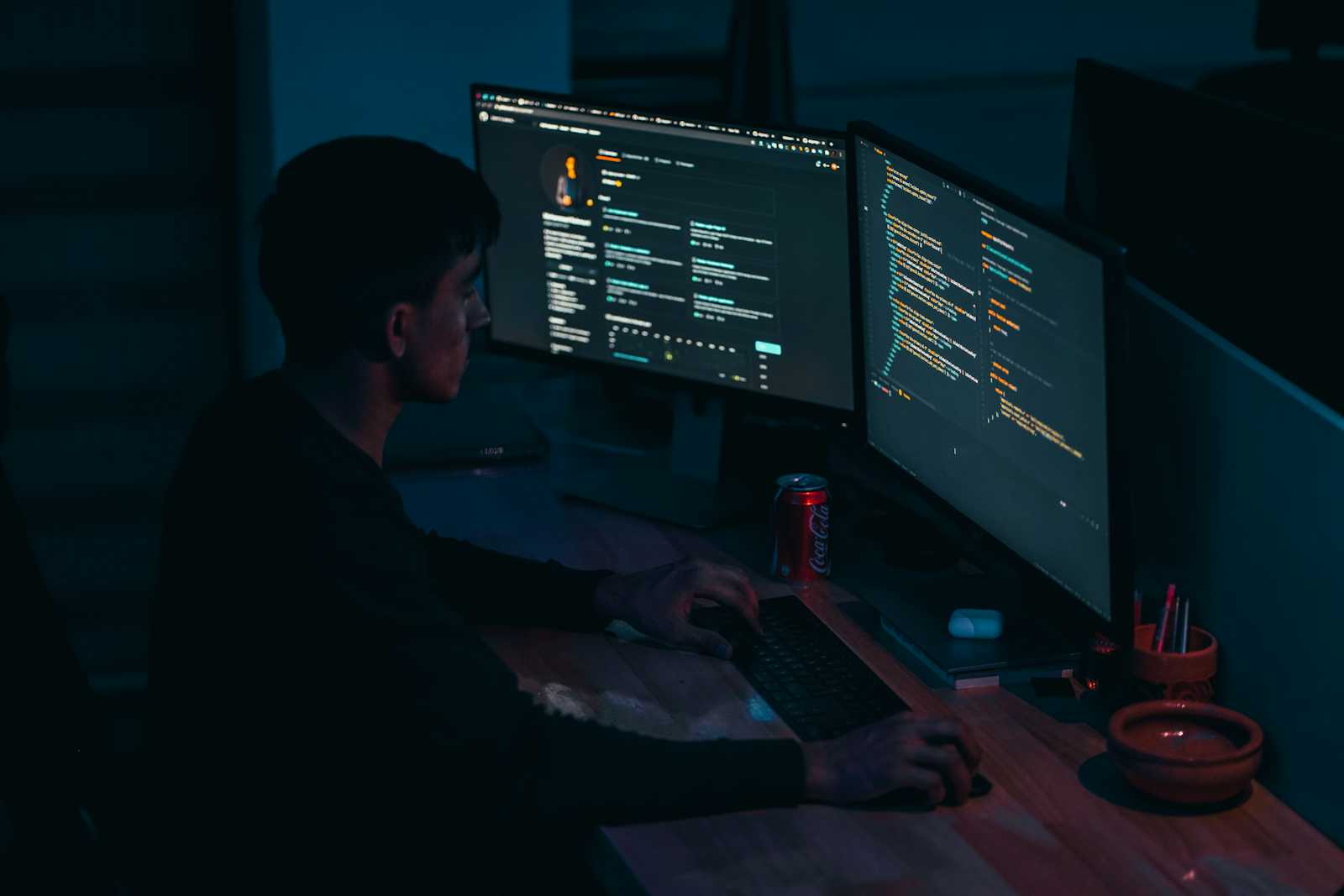
Certainly! Let's expand on the blog, providing more details and explanations about creational design patterns, specifically focusing on the Factory and Abstract Factory patterns.
Creational Design Patterns
Introduction
Creational design patterns are a subset of design patterns that deal with object creation mechanisms, trying to create objects in a manner suitable to the situation. They often involve the use of a dedicated class or method for creating objects, providing a way to decouple the client code from the actual instantiation of objects. In Java, creational design patterns are crucial for promoting flexibility, reusability, and maintainability in software development.
Factory Design Pattern
Overview
The Factory Design Pattern falls under the creational category and is used to define an interface for creating an object but leaves the choice of its type to the subclasses. This pattern provides one of the best ways to create an object. In the Factory pattern, we delegate the responsibility of instantiating the object to its subclasses, allowing a class to delegate the responsibility of instantiating its instances to its subclasses.
Implementation in Java
Let's consider a simple example to illustrate the Factory Design Pattern in Java:
// Product interface
interface Shape {
void draw();
}
// Concrete product: Circle
class Circle implements Shape {
@Override
public void draw() {
System.out.println("Drawing a Circle");
}
}
// Concrete product: Rectangle
class Rectangle implements Shape {
@Override
public void draw() {
System.out.println("Drawing a Rectangle");
}
}
// Factory interface
interface ShapeFactory {
Shape createShape();
}
// Concrete factory: CircleFactory
class CircleFactory implements ShapeFactory {
@Override
public Shape createShape() {
return new Circle();
}
}
// Concrete factory: RectangleFactory
class RectangleFactory implements ShapeFactory {
@Override
public Shape createShape() {
return new Rectangle();
}
}
// Client code
public class ShapeClient {
public static void main(String[] args) {
ShapeFactory circleFactory = new CircleFactory();
Shape circle = circleFactory.createShape();
circle.draw();
ShapeFactory rectangleFactory = new RectangleFactory();
Shape rectangle = rectangleFactory.createShape();
rectangle.draw();
}
}
In this example, the ConcreteFactory
class is responsible for creating a ConcreteProduct
. The client code uses the factory to create the product without knowing the specific implementation details of the product.
Benefits
Flexibility: The client code is not tied to the specific classes it instantiates, allowing for easy changes or additions of new product types.
Encapsulation: The process of creating an object is encapsulated within the factory, reducing the client's knowledge about the creation details.
Code Reusability: The factory can be reused to create different products, promoting code reusability.
Abstract Factory Design Pattern
Overview
The Abstract Factory Design Pattern extends the Factory pattern by providing an interface for creating families of related or dependent objects without specifying their concrete classes. It is particularly useful when the system needs to be independent of how its objects are created, composed, and represented.
Implementation in Java
Let's delve into a more complex example to illustrate the Abstract Factory Design Pattern:
// Abstract product A
interface ProductA {
void createA();
}
// Concrete product A1
class ConcreteProductA1 implements ProductA {
@Override
public void createA() {
System.out.println("Concrete Product A1 created");
}
}
// Concrete product A2
class ConcreteProductA2 implements ProductA {
@Override
public void createA() {
System.out.println("Concrete Product A2 created");
}
}
// Abstract product B
interface ProductB {
void createB();
}
// Concrete product B1
class ConcreteProductB1 implements ProductB {
@Override
public void createB() {
System.out.println("Concrete Product B1 created");
}
}
// Concrete product B2
class ConcreteProductB2 implements ProductB {
@Override
public void createB() {
System.out.println("Concrete Product B2 created");
}
}
// Abstract factory
interface AbstractFactory {
ProductA createProductA();
ProductB createProductB();
}
// Concrete factory 1
class ConcreteFactory1 implements AbstractFactory {
@Override
public ProductA createProductA() {
return new ConcreteProductA1();
}
@Override
public ProductB createProductB() {
return new ConcreteProductB1();
}
}
// Concrete factory 2
class ConcreteFactory2 implements AbstractFactory {
@Override
public ProductA createProductA() {
return new ConcreteProductA2();
}
@Override
public ProductB createProductB() {
return new ConcreteProductB2();
}
}
// Client code
public class Client {
public static void main(String[] args) {
AbstractFactory factory1 = new ConcreteFactory1();
ProductA productA1 = factory1.createProductA();
ProductB productB1 = factory1.createProductB();
AbstractFactory factory2 = new ConcreteFactory2();
ProductA productA2 = factory2.createProductA();
ProductB productB2 = factory2.createProductB();
productA1.createA();
productB1.createB();
productA2.createA();
productB2.createB();
}
}
In this example, we have two families of products (A and B), each with two variations. The client can create products from a particular family without worrying about the concrete classes involved.
Benefits
Abstracted Object Creation: The client code is shielded from the complexities of object creation, promoting a high level of abstraction.
Consistent Product Families: Abstract Factory ensures that the created objects are compatible and consistent, as they belong to the same family.
Easy Extensibility: Adding new product families or variations is easier, as you can simply create a new set of concrete classes that implement the abstract product interfaces.
Conclusion
Creational design patterns play a crucial role in shaping the way objects are instantiated in a software system. The Factory and Abstract Factory patterns, in particular, provide elegant solutions to object creation challenges. By encapsulating the instantiation process, these patterns enhance code flexibility, encapsulation, and reusability.
For Further Reference:
For a more in-depth exploration of creational design patterns and other design patterns, you can refer to Refactoring Guru's Creational Patterns insights into various design patterns.
In your Java projects, consider the context in which these patterns may be beneficial and leverage their power to create maintainable and extensible software solutions. Happy coding❤️!
Subscribe to my newsletter
Read articles from Mukul kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
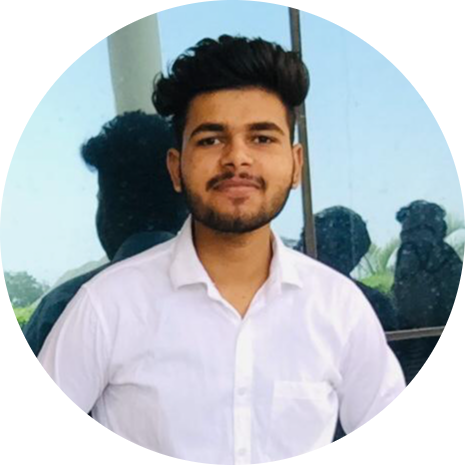
Mukul kumar
Mukul kumar
Hey! I am a software Engineer who is skilled in Java backend and has a good understanding of Spring Boot and Quarkus Frameworks