Ref API and Forwarding Refs in React
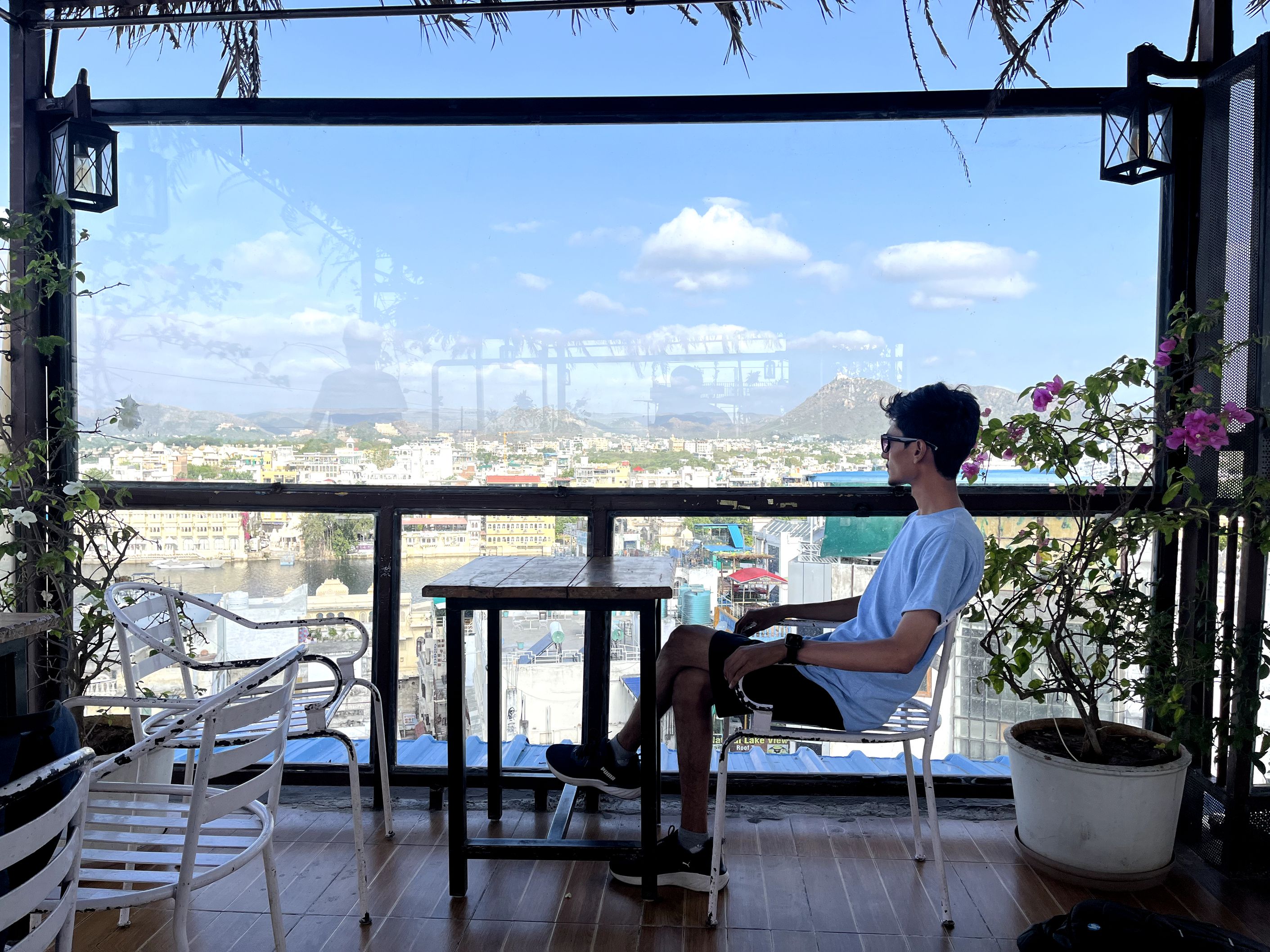
Expert-Level Explanation
The Ref API in React provides a way to access DOM nodes or React elements created in the render method. A ref is used for more imperative actions in your components, like directly managing focus, media playback, or triggering animations. It bypasses the normal data flow and allows you to modify a child component without using props. Forwarding refs is a technique that lets you pass refs down to child components, which is particularly useful in higher-order components or when you need to access a child component's DOM node from a parent component.
Creative Explanation
Think of refs in React as a direct line of communication to a component or DOM element, like a phone line to a specific office in a large building. Normally, messages (data) travel through a complex mail system (props), but sometimes you need to bypass this and speak directly to a specific department (DOM node or component). Forwarding refs is like an operator who can connect your call (ref) directly to the right office (child component) inside the building (component tree).
Practical Explanation with Code
createRef
To create a ref:
import React, { createRef, Component } from 'react';
class MyComponent extends Component {
constructor(props) {
super(props);
this.myRef = createRef();
}
componentDidMount() {
this.myRef.current.focus();
}
render() {
return <input type="text" ref={this.myRef} />;
}
}
In this example, createRef
creates a ref (this.myRef
) which is attached to an input element. When the component mounts, focus is set directly to this input.
forwardRef
UsingforwardRef
to forward a reference to a child component:
import React, { forwardRef } from 'react';
const FancyInput = forwardRef((props, ref) => (
<input ref={ref} type="text" style={{ borderColor: 'blue' }} />
));
class ParentComponent extends React.Component {
inputRef = React.createRef();
componentDidMount() {
this.inputRef.current.focus();
}
render() {
return <FancyInput ref={this.inputRef} />;
}
}
forwardRef
is used to pass a reference from ParentComponent
to FancyInput
, allowing the parent to directly interact with the DOM element in the child.
Real-World Example
Imagine a large-scale application like an online text editor. In this application, you might have a complex component structure where a parent component needs direct access to a DOM element in a deeply nested child component, such as a specific text input field. Using createRef
andforwardRef
, you can manage focus, select text, or perform other imperative actions on that input field from the parent component, even though it is deeply nested within the application's component tree.
For example, when opening a document, the application can automatically set focus to the text input field using references, allowing the user to start typing immediately without needing to click into the field first.
Subscribe to my newsletter
Read articles from Akash Thoriya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
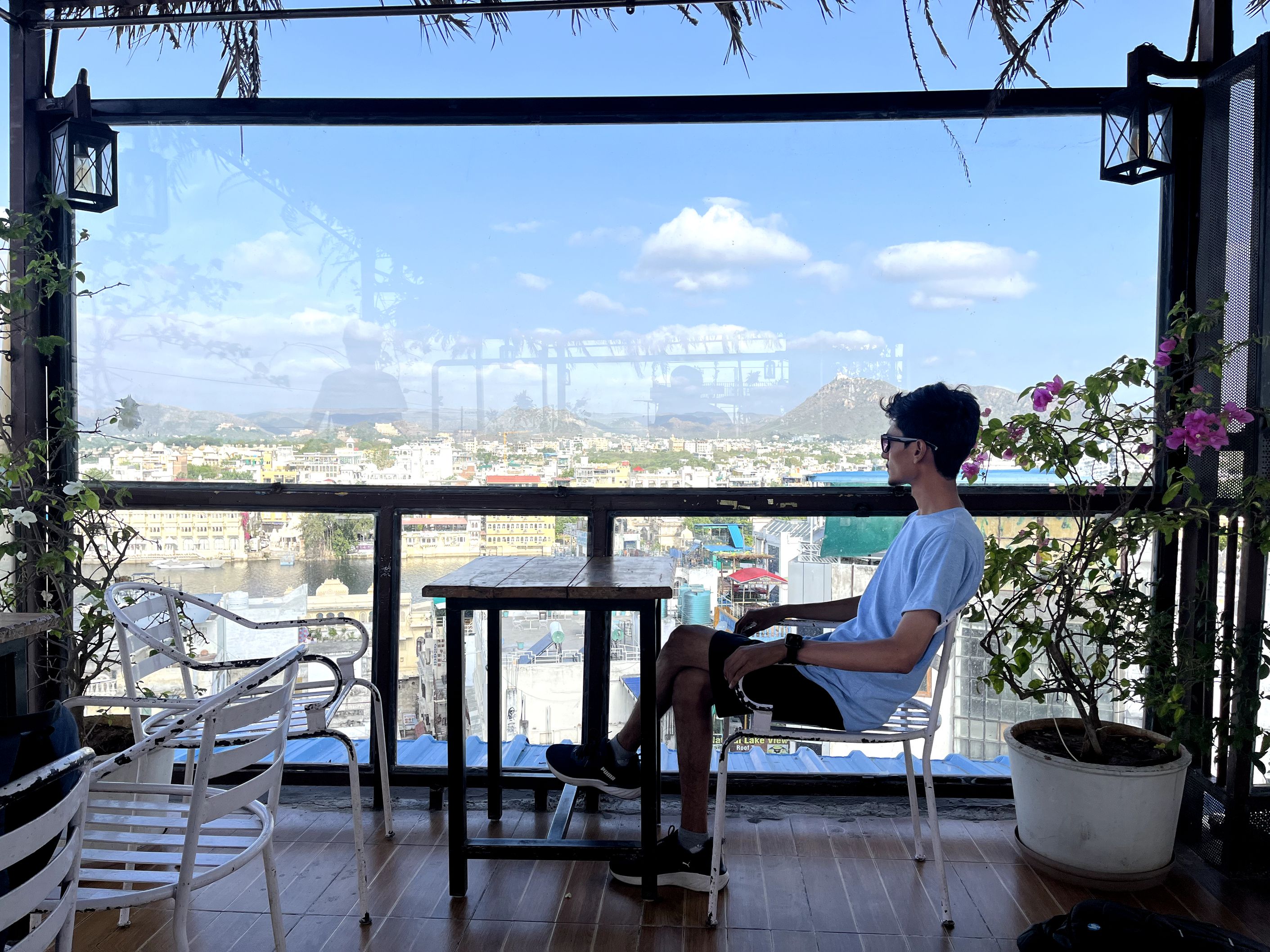
Akash Thoriya
Akash Thoriya
As a senior full-stack developer, I am passionate about creating efficient and scalable web applications that enhance the user experience. My expertise in React, Redux, NodeJS, and Laravel has enabled me to lead cross-functional teams and deliver projects that consistently exceed client expectations.