Error Boundaries in React
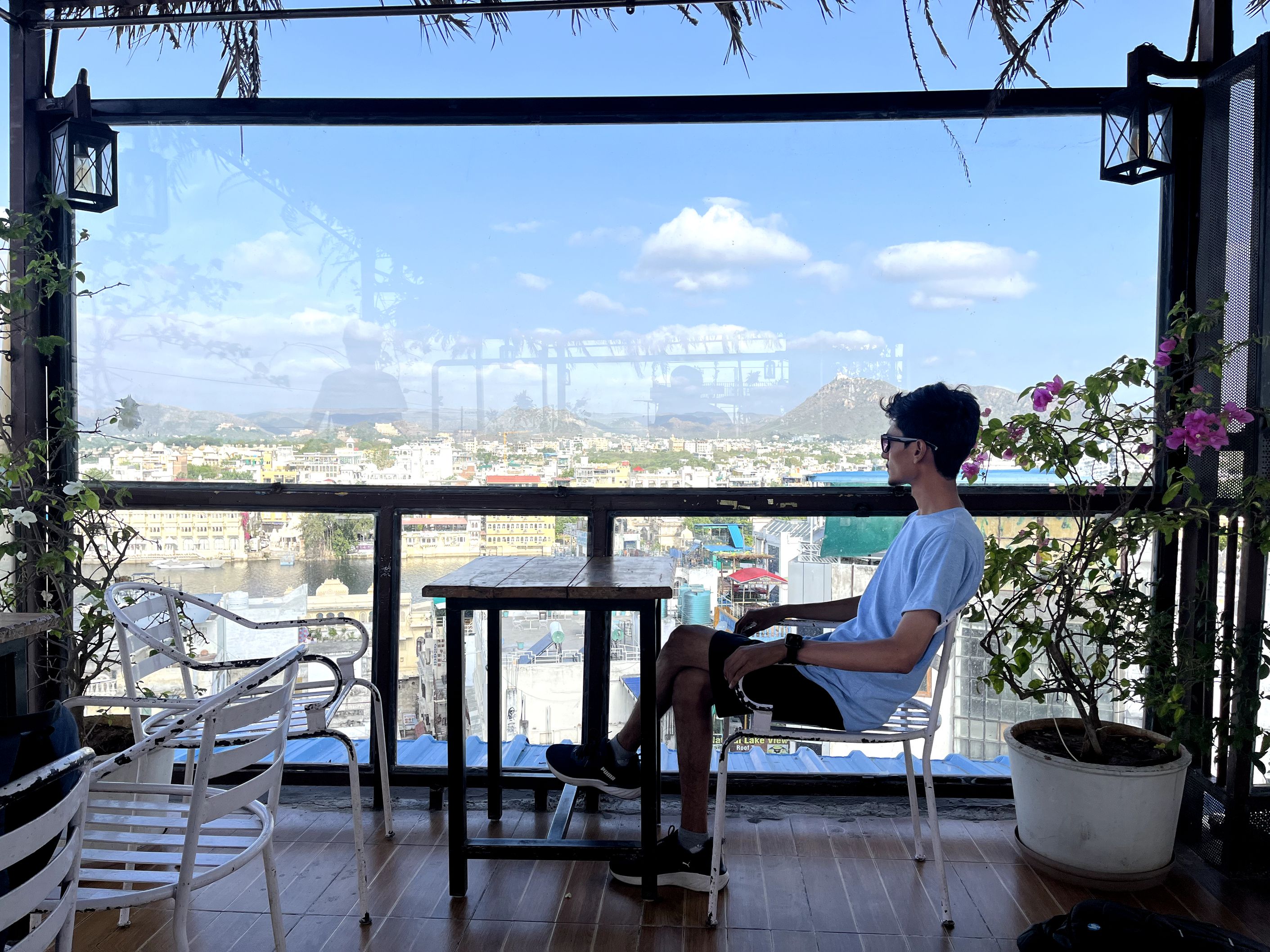
Expert-Level Explanation
Error boundaries in React are components that catch JavaScript errors in their child component tree, log those errors, and display a fallback UI instead of the component tree that crashed. They are a way to gracefully handle errors in a React application. Error boundaries catch errors during rendering, in lifecycle methods, and in the constructors of the whole tree below them.
Creative Explanation
Think of an error boundary as a safety net in a circus. When acrobats (components) perform high in the air (rendering), the safety net (error boundary) is in place to catch anyone who falls (errors in components). This ensures that despite the fall, the show (application) can go on, possibly with an alternative act (fallback UI), and the audience (users) doesn't experience the full impact of the accident.
Practical Explanation with Code
To use error boundaries, you typically define a new class component with the lifecycle methods static getDerivedStateFromError
or componentDidCatch
.
static getDerivedStateFromError
class ErrorBoundary extends React.Component {
state = { hasError: false };
static getDerivedStateFromError(error) {
// Update state so the next render will show the fallback UI.
return { hasError: true };
}
render() {
if (this.state.hasError) {
return <h1>Something went wrong.</h1>;
}
return this.props.children;
}
}
componentDidCatch
class ErrorBoundary extends React.Component {
state = { hasError: false, errorInfo: null };
componentDidCatch(error, errorInfo) {
// You can also log the error to an error reporting service
this.setState({
hasError: true,
errorInfo: errorInfo
});
}
render() {
if (this.state.hasError) {
// You can render any custom fallback UI
return <h1>Something went wrong.</h1>;
}
return this.props.children;
}
}
Real-World Example
In a large e-commerce website, an error boundary can be used around a product listing component. If an error occurs in any part of this component (like an API call failing to fetch product details), the error boundary can catch this error and display a user-friendly message like "Oops! Something went wrong. Please try again later." This prevents the entire app from crashing and provides a better user experience.
Subscribe to my newsletter
Read articles from Akash Thoriya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
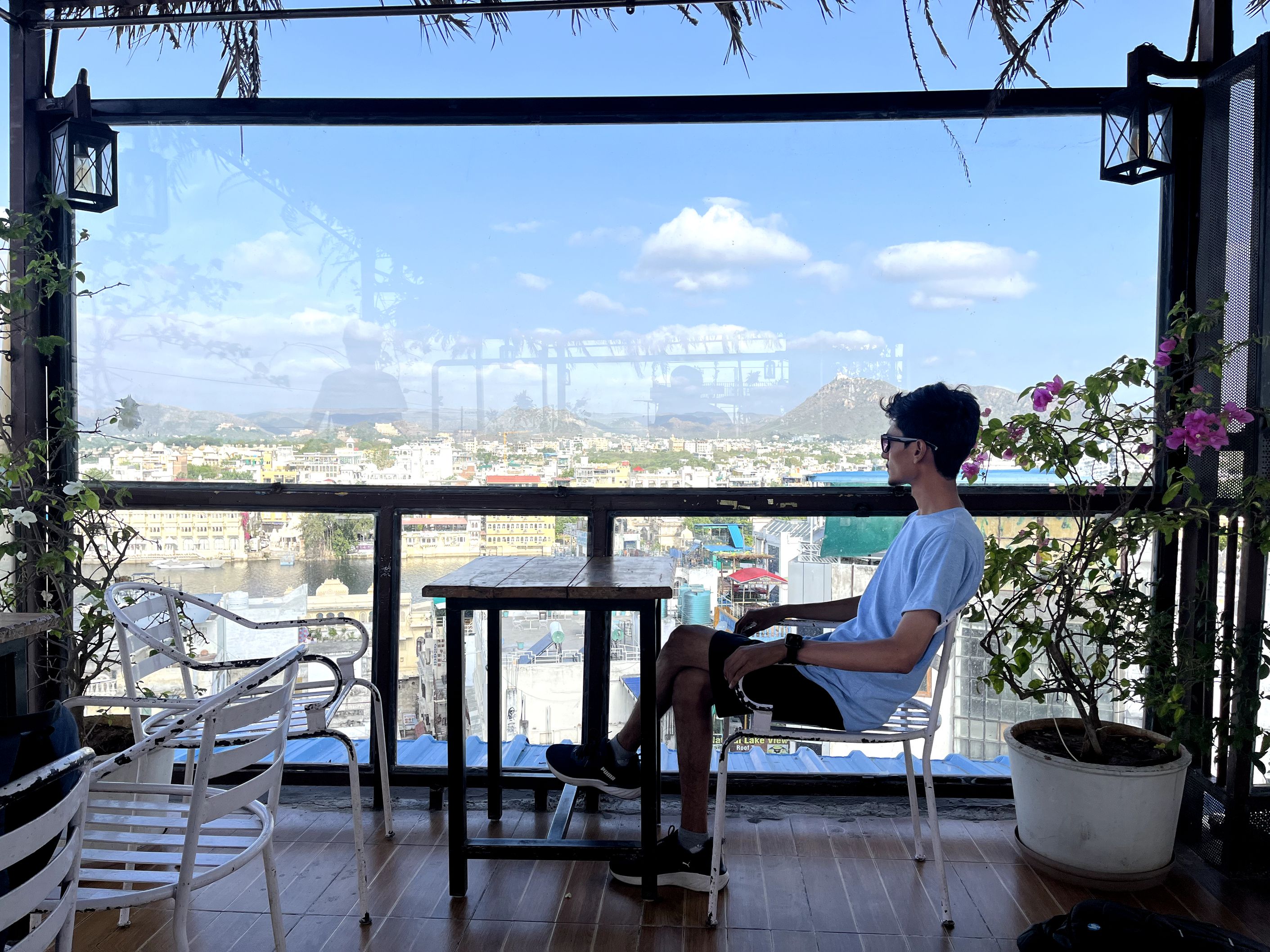
Akash Thoriya
Akash Thoriya
As a senior full-stack developer, I am passionate about creating efficient and scalable web applications that enhance the user experience. My expertise in React, Redux, NodeJS, and Laravel has enabled me to lead cross-functional teams and deliver projects that consistently exceed client expectations.