Higher-Order Components in React
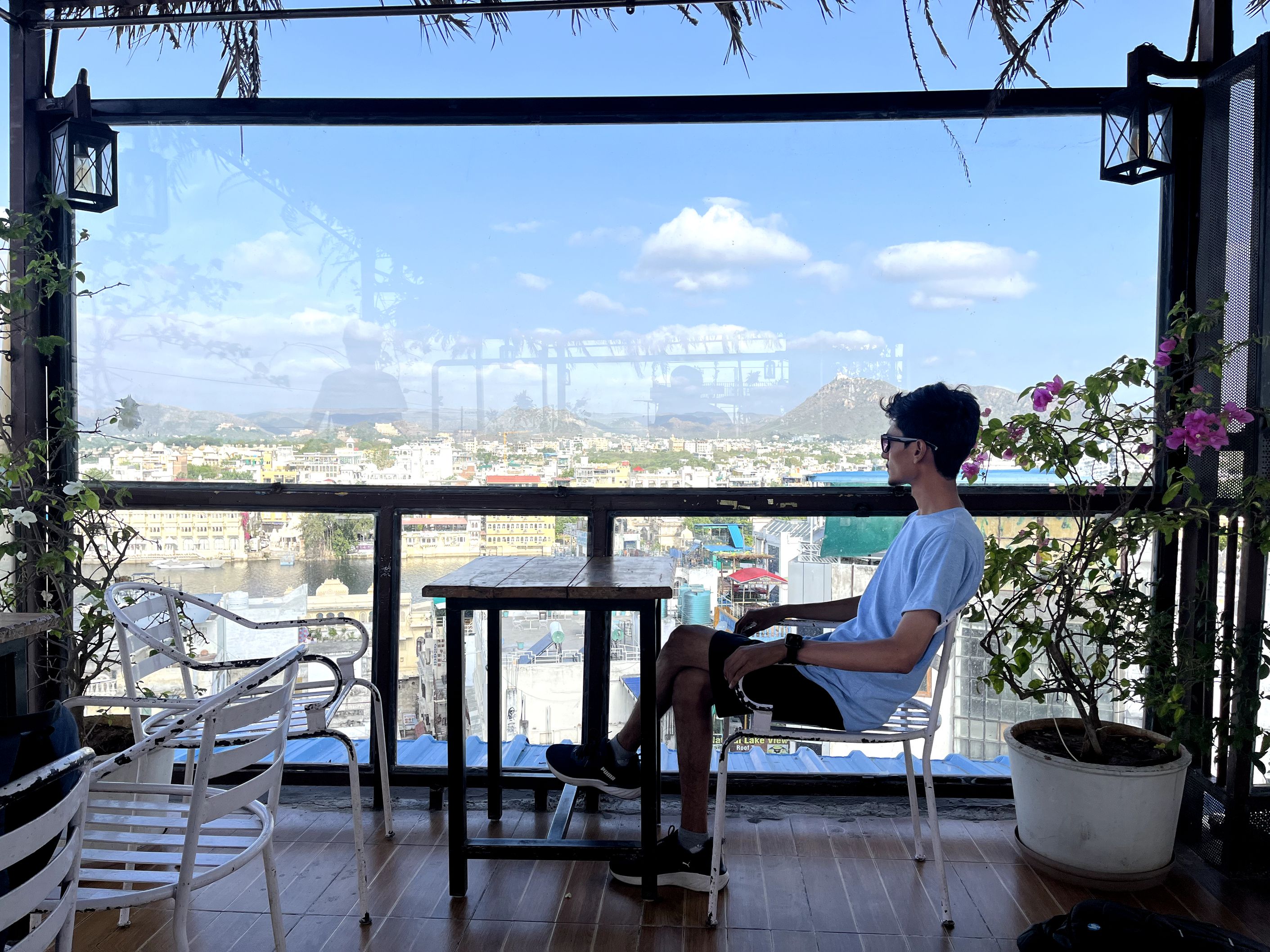
Expert-Level Explanation
Higher-Order Components (HOCs) in React are a pattern used to reuse component logic. An HOC is a function that takes a component and returns a new component with extended functionalities. They're similar to higher-order functions in JavaScript, which take functions as arguments or return a function. HOCs are useful for cross-cutting concerns, like enhancing components with additional data fetching capabilities, access control, or logging.
Creative Explanation
Imagine you run a car modification shop. Customers bring in their standard cars (components), and you enhance them with additional features like a turbo engine or advanced navigation systems (extra functionalities). Similarly, in React, you have a basic component, and an HOC adds additional features or enhancements to it, resulting in a new, more powerful component.
Practical Explanation with Code
Building an HOC
function withExtraInfo(WrappedComponent) {
return class extends React.Component {
render() {
return <WrappedComponent extraInfo="This is extra info" {...this.props} />;
}
};
}
// Usage
function MyComponent(props) {
return <div>{props.extraInfo}</div>;
}
const EnhancedComponent = withExtraInfo(MyComponent);
In this example, withExtraInfo
is an HOC that adds extraInfo
as a prop to any component passed to it.
Real-World Example
In a real-world application like a blogging platform, an HOC could be used to add user authentication checks to various components. For example, an withAuthentication
HOC could wrap around components that require the user to be logged in, automatically redirecting to a login page if the user is not authenticated.
Subscribe to my newsletter
Read articles from Akash Thoriya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
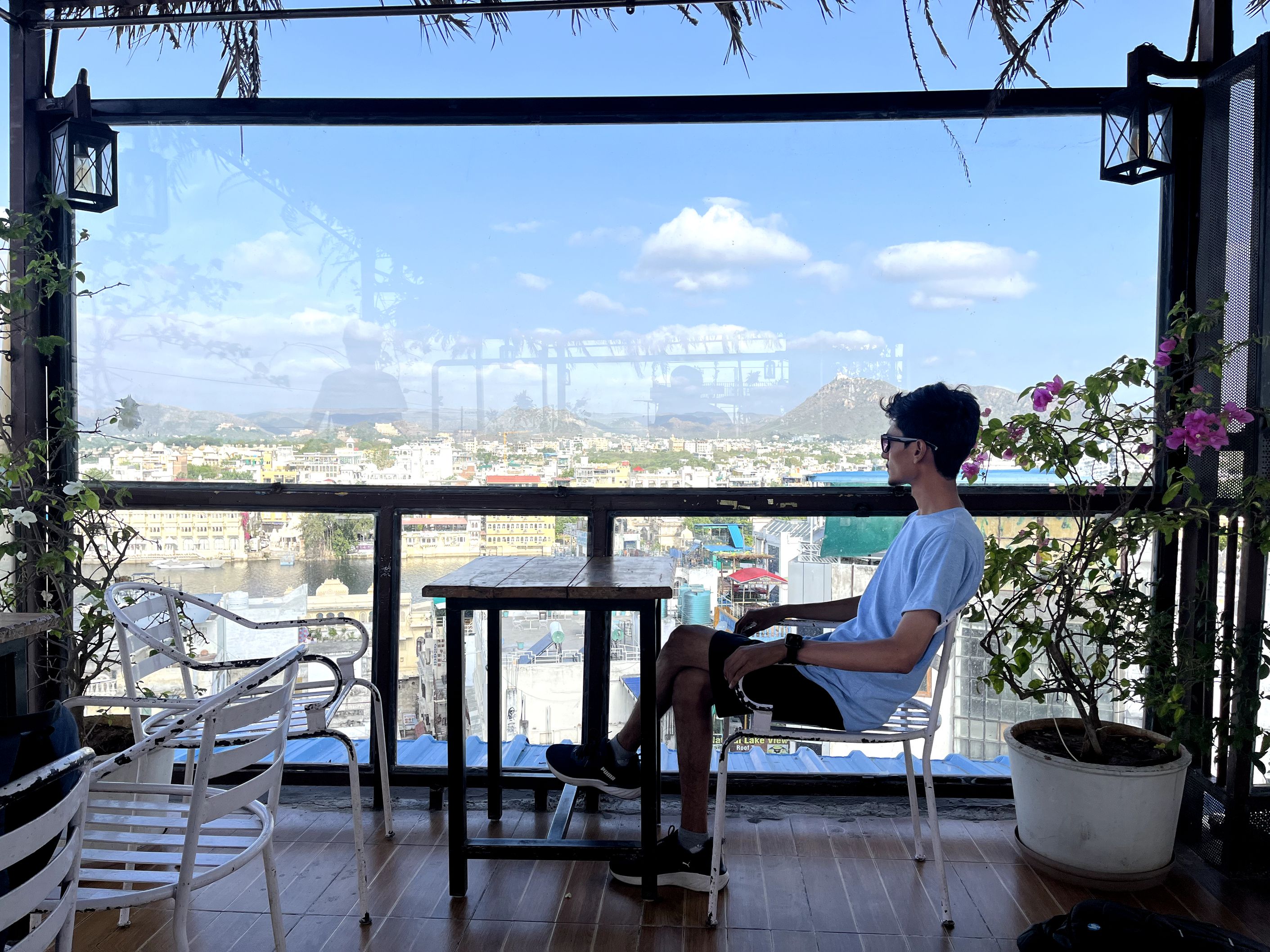
Akash Thoriya
Akash Thoriya
As a senior full-stack developer, I am passionate about creating efficient and scalable web applications that enhance the user experience. My expertise in React, Redux, NodeJS, and Laravel has enabled me to lead cross-functional teams and deliver projects that consistently exceed client expectations.