Render Props in React
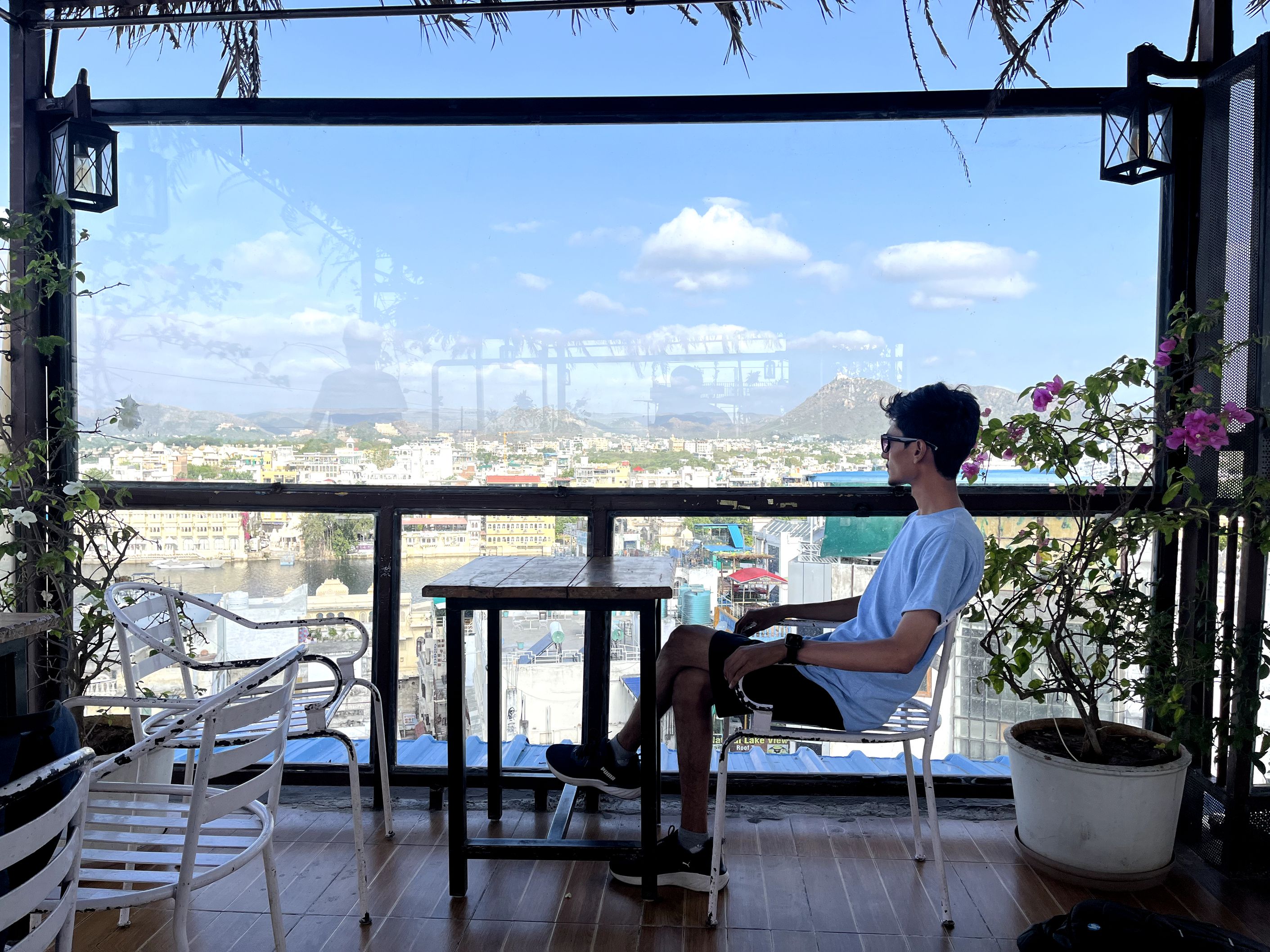
Expert-Level Explanation
Render props is a technique in React for sharing code between components using a prop whose value is a function. A component with a render prop takes a function that returns a React element and calls it instead of implementing its own render logic. This pattern is used to share behaviour across different components.
Creative Explanation
Imagine render props as a recipe book (the render prop function) given to different chefs (components). Each chef uses the same book to cook a variety of dishes (rendering different UIs). The recipe book provides the method (shared logic), but each chef can choose different ingredients (props) to create a unique dish (UI).
Practical Explanation with Code
Using Render Props
class MouseTracker extends React.Component {
state = { x: 0, y: 0 };
handleMouseMove = (event) => {
this.setState({
x: event.clientX,
y: event.clientY
});
};
render() {
return (
<div style={{ height: '100vh' }} onMouseMove={this.handleMouseMove}>
{this.props.render(this.state)}
</div>
);
}
}
// Usage
<MouseTracker render={({ x, y }) => (
<h1>The mouse position is ({x}, {y})</h1>
)} />
In this example, MouseTracker
is a component that tracks the mouse position and uses a render prop to render the UI.
Real-World Example
In an e-commerce website, a render prop could be used for a tooltip component. The tooltip component handles logic like positioning and visibility, while the render prop allows for custom content to be displayed within the tooltip. This way, the tooltip component can be reused with different content across the website.
Subscribe to my newsletter
Read articles from Akash Thoriya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
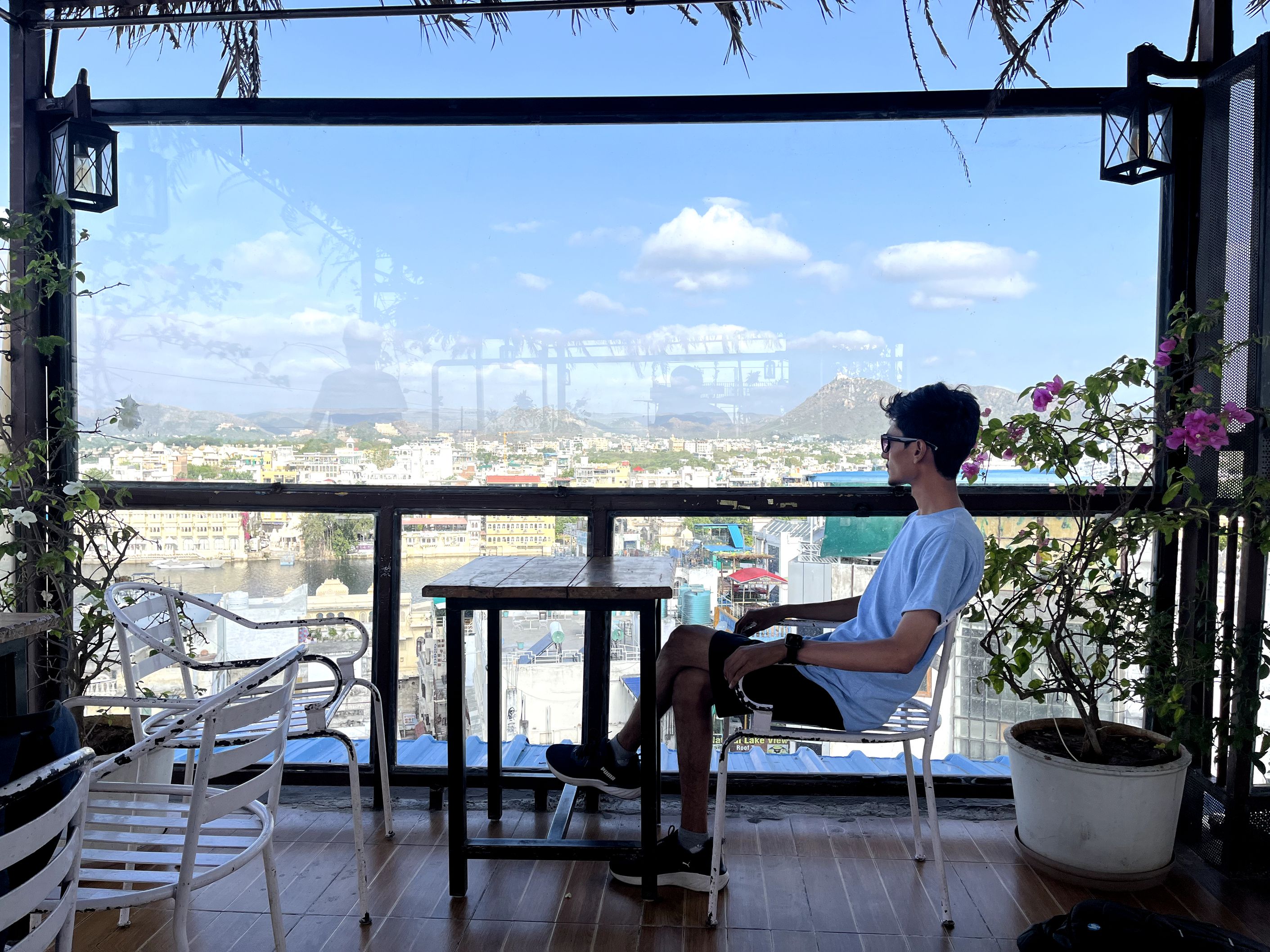
Akash Thoriya
Akash Thoriya
As a senior full-stack developer, I am passionate about creating efficient and scalable web applications that enhance the user experience. My expertise in React, Redux, NodeJS, and Laravel has enabled me to lead cross-functional teams and deliver projects that consistently exceed client expectations.