Simplifying Phone Number Verification using OTP in Java & Spring Boot
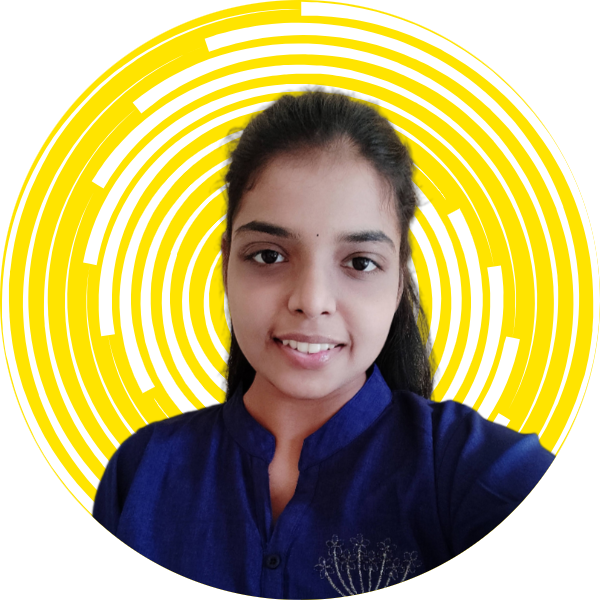
Table of contents
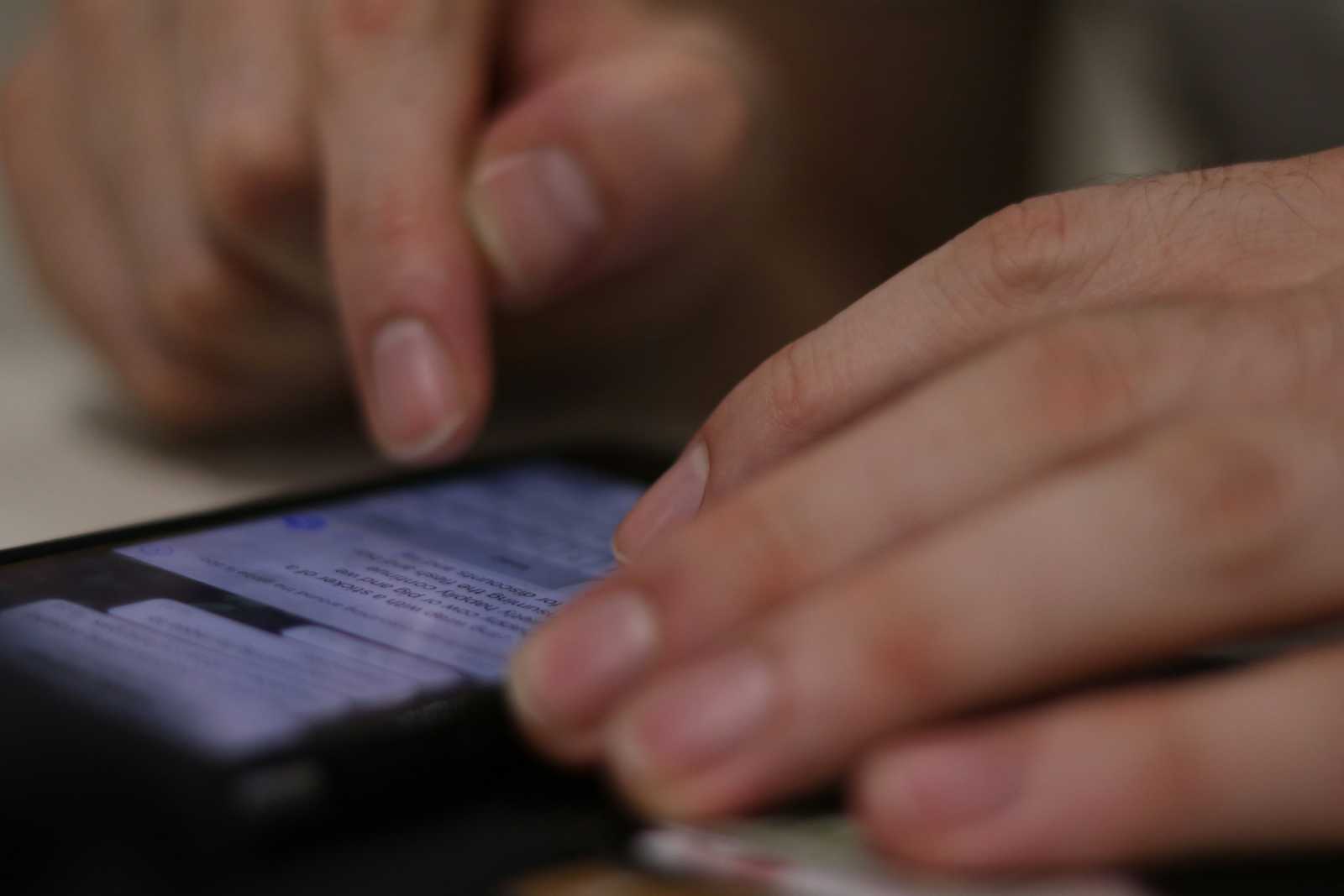
Introduction:
In today's digital era, securing user information is of utmost importance. One common method of enhancing security is by verifying user phone numbers through One-Time Passwords (OTPs). In this blog post, we'll explore how to implement phone number verification using Java, Twilio, and OTPs. Twilio is a popular cloud communications platform that allows developers to integrate messaging and voice capabilities into their applications.
Prerequisites:
Before we dive into the code, make sure you have the following prerequisites:
Java Development Kit (JDK) installed on your machine.
A Twilio account and Twilio phone number. You can sign up for a free Twilio account at https://www.twilio.com.
Setting Up Your Twilio Account:
Sign in to your Twilio account and obtain your Account SID, Auth Token, and Twilio phone number.
Create a new Messaging Service on Twilio, which will be used to send OTPs.
Get Account SID, Auth Token, and Twilio phone number at https://console.twilio.com
Writing Java Code for Phone Number Verification:
import com.twilio.Twilio;
import com.twilio.rest.api.v2010.account.Message;
import com.twilio.type.PhoneNumber;
import java.util.Random;
public class PhoneVerification {
// Replace these values with your Twilio Account SID, Auth Token, and Twilio phone number
private static final String ACCOUNT_SID = "your_account_sid";
private static final String AUTH_TOKEN = "your_auth_token";
private static final String TWILIO_PHONE_NUMBER = "your_twilio_phone_number";
public static void main(String[] args) {
// Initialize Twilio
Twilio.init(ACCOUNT_SID, AUTH_TOKEN);
// Generate a random 6-digit OTP
String otp = generateOTP();
// Replace this with the recipient's phone number
String recipientPhoneNumber = "+1234567890";
// Send the OTP via Twilio
sendOtpViaTwilio(recipientPhoneNumber, otp);
// You can now use the received OTP for verification
System.out.println("OTP sent successfully!");
}
private static String generateOTP() {
Random random = new Random();
int otp = 100000 + random.nextInt(900000);
return String.valueOf(otp);
}
private static void sendOtpViaTwilio(String to, String otp) {
Message message = Message.creator(
new PhoneNumber(to),
new PhoneNumber(TWILIO_PHONE_NUMBER),
"Your OTP for phone verification is: " + otp)
.create();
System.out.println("OTP sent with SID: " + message.getSid());
}
}
Explanation:
Twilio Initialization: Replace
your_account_sid
,your_auth_token
, andyour_twilio_phone_number
with your Twilio credentials.Generating OTP: The
generateOTP()
method generates a random 6-digit OTP.Sending OTP via Twilio: The
sendOtpViaTwilio()
method uses Twilio'sMessage.creator
to send the OTP to the specified phone number.Main Method: Replace
+1234567890
with the recipient's phone number. After running the program, the OTP will be sent to the specified phone number.
Conclusion:
Implementing phone number verification using Java, Twilio, and OTPs is a straightforward process. This approach adds an extra layer of security to your applications by ensuring that users provide a valid phone number and confirming their identity through OTPs. Integrating Twilio's powerful messaging capabilities allows for a seamless and reliable user experience in the verification process.
Subscribe to my newsletter
Read articles from Pushpa Mali directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
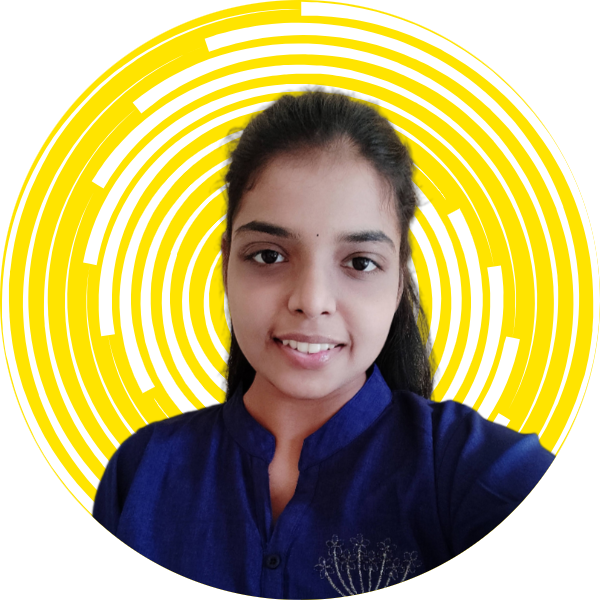
Pushpa Mali
Pushpa Mali
I am Pushpa Mali a Software Developer. My tech stacks are HTML, CSS, Javascript, Node JS , Java, Spring Boot, React Js, SQL, Figma.