Map, Filter & Reduce Function
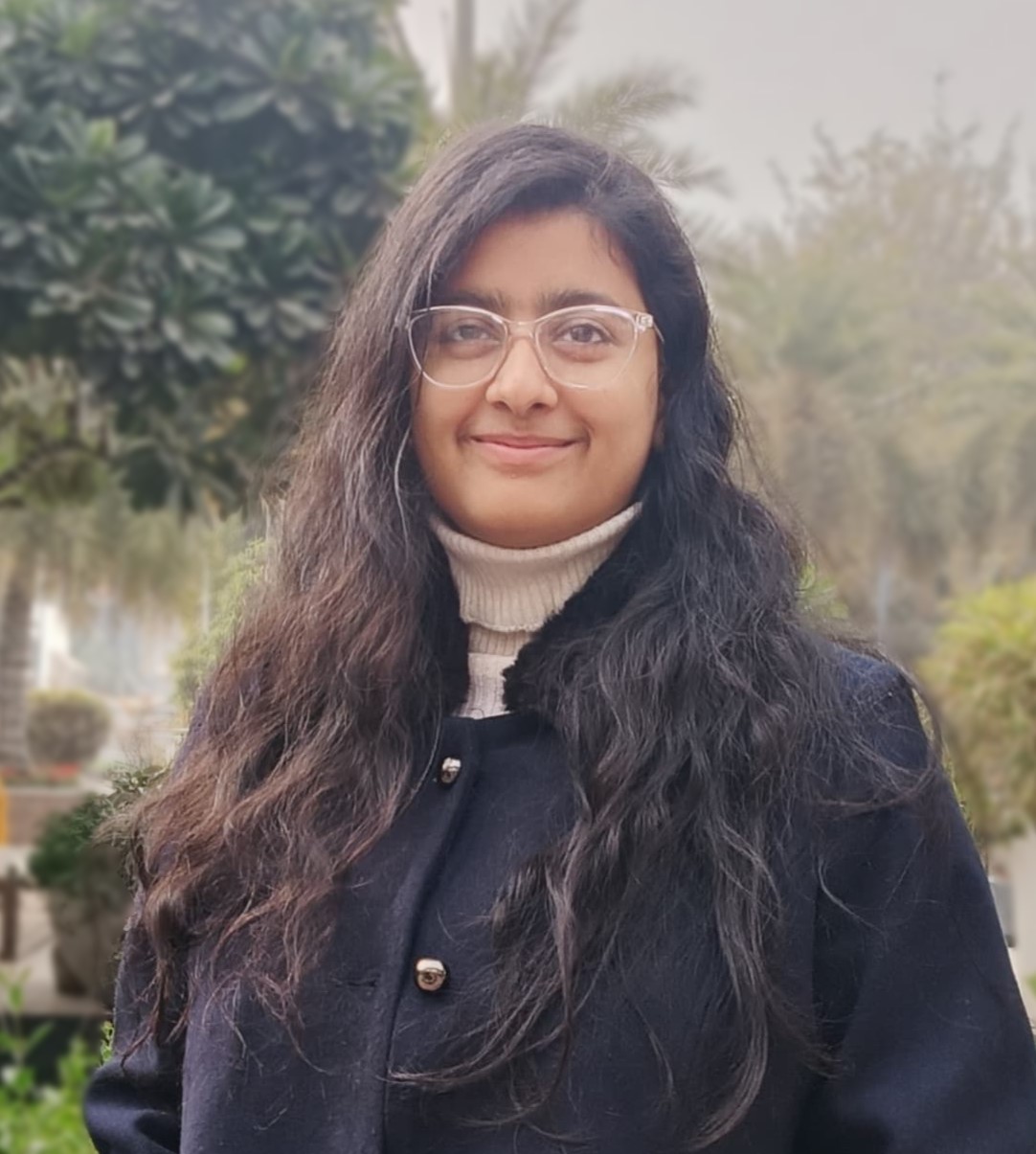
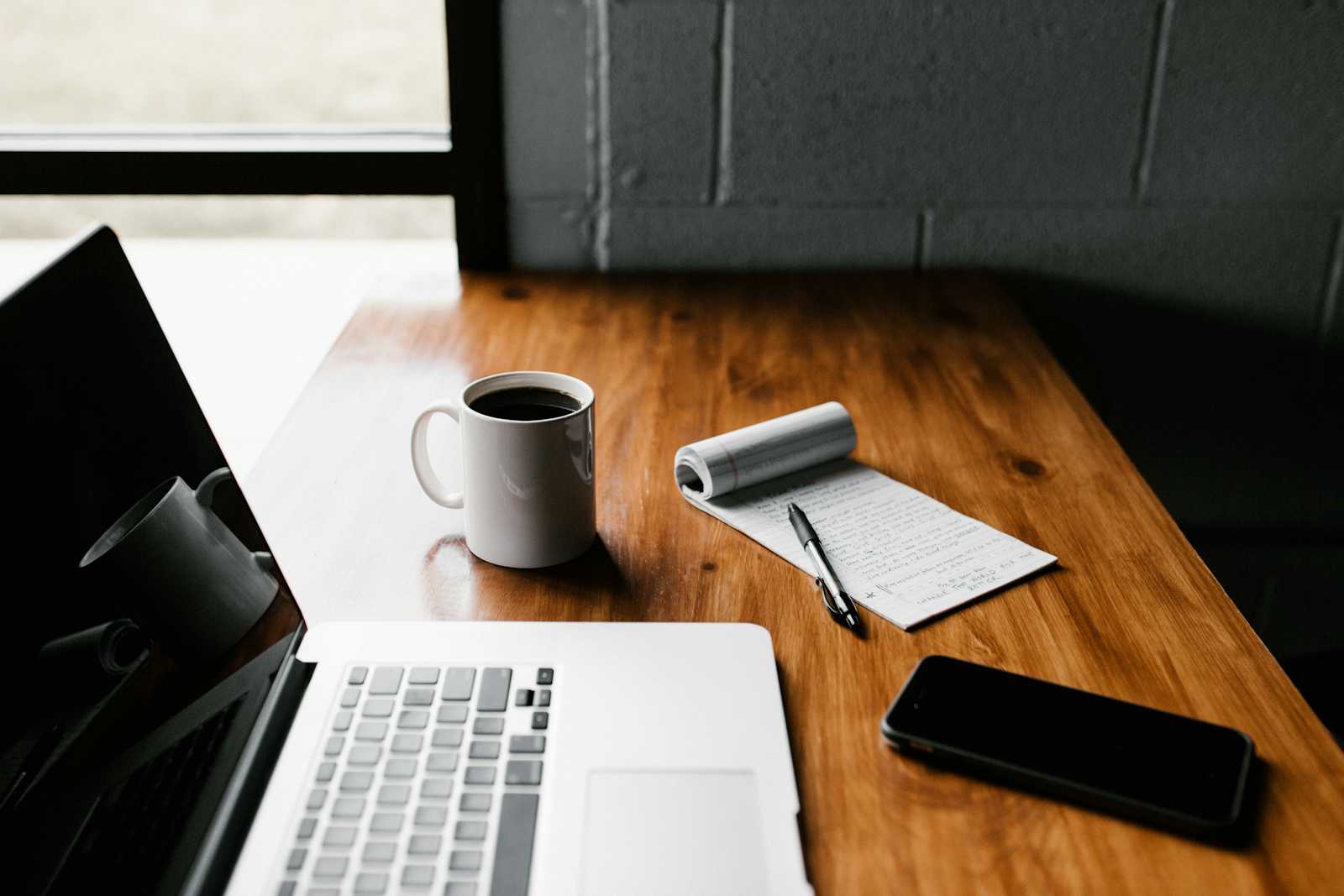
map()
The map()
function applies a given function to each item of an iterable (like a list) and returns a map object (an iterator).
Syntax:
map(function, iterable)
Example:
# Doubling the numbers in a list
numbers = [1, 2, 3, 4, 5]
doubled = list(map(lambda x: x * 2, numbers))
print(doubled) # Output: [2, 4, 6, 8, 10]
filter()
The filter()
function filters elements from an iterable based on a function that returns True
or False
.
Syntax:
filter(function, iterable)
Example:
# Filtering even numbers from a list
numbers = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
even_numbers = list(filter(lambda x: x % 2 == 0, numbers))
print(even_numbers) # Output: [2, 4, 6, 8, 10]
reduce()
The reduce()
function applies a rolling computation to sequential pairs of elements in an iterable, reducing them to a single value.
Note: In Python 3, reduce()
is part of the functools
module.
Syntax:
from functools import reduce
reduce(function, iterable)
Example:
from functools import reduce
# Adding all numbers in a list
numbers = [1, 2, 3, 4, 5]
sum_numbers = reduce(lambda x, y: x + y, numbers)
print(sum_numbers) # Output: 15 (1 + 2 + 3 + 4 + 5 = 15)
These functions are powerful when used appropriately. They enhance code readability and efficiency, especially when dealing with transformations, filtering, or aggregations on iterable data structures.
Subscribe to my newsletter
Read articles from Anugya Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
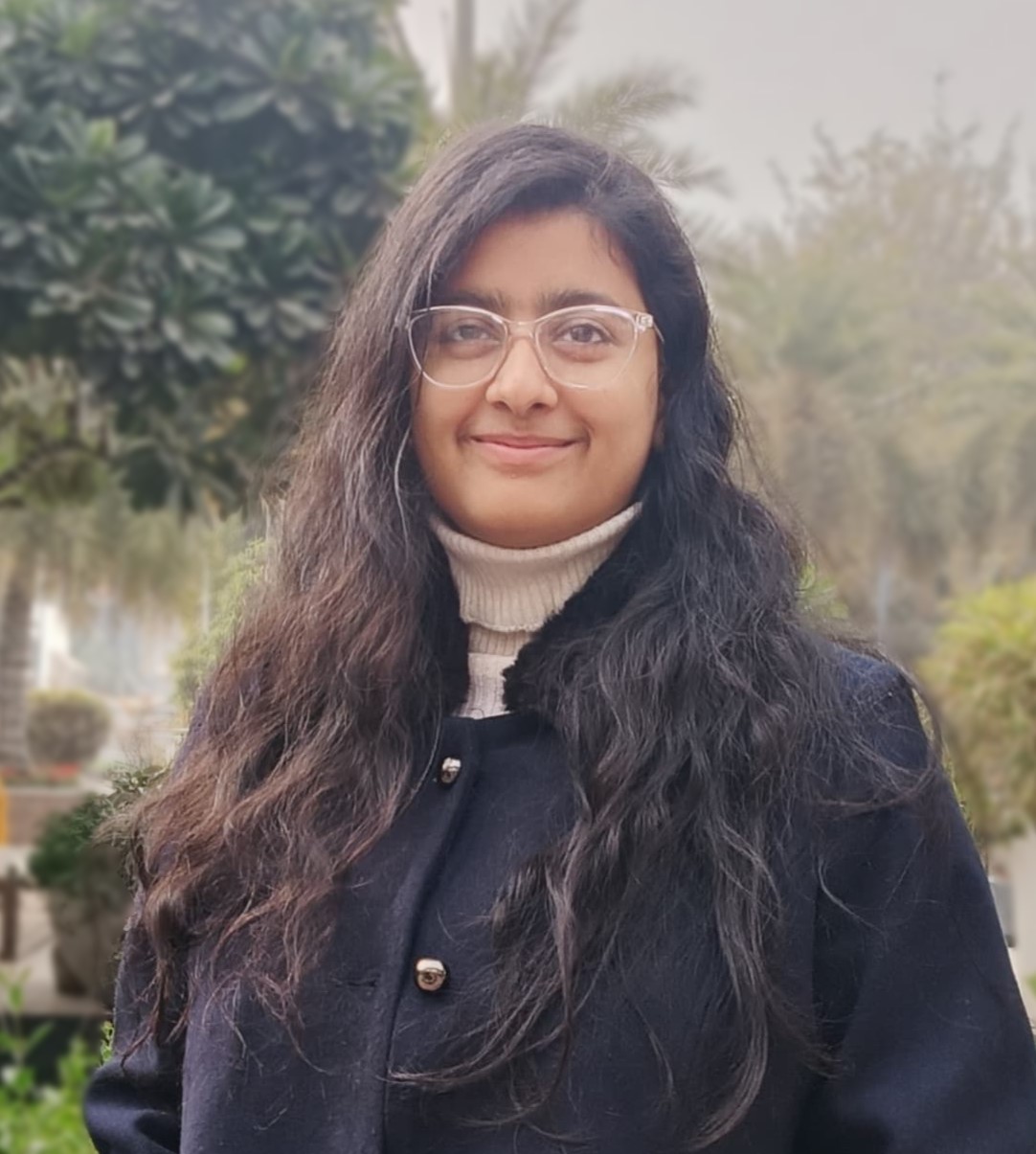
Anugya Gupta
Anugya Gupta
Hi! I'm an aspiring techie with a passion for translating complex concepts into simple, engaging content. Eager learner and detail-oriented individual committed to delivering high-quality articles.