What are Interfaces in golang ?

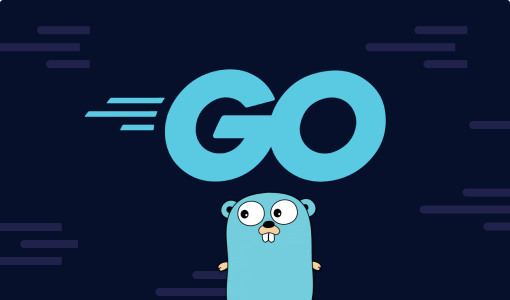
I had a hard time understanding Interfaces in go because I had never wrote a Interface before in my life. Interfaces in go are very simple and easy to understand they are slightly different from other programming language because go is not fully object oriented. The concept of interfaces in go is easy to work with
If we look at the go docs it simply states that An interface type is defined as a set of method signatures.
Lets take a real world example of interface type to make it easier to understand
In the below code i am implementing a server from std lib in go. Lets start by initialising the project
go mod init Server
Now write the package and import the libraries
package main
import (
"context"
"log"
"net/http"
"os"
)
we are going to use a Interface which is in the http lib. the http.Handler is a interface.
type Handler interface {
ServeHTTP(ResponseWriter, *Request)
}
this Handler has a ServerHTTP method which a type must implement. In the below code we define a type called TextHandler
, which is essentially a string. By adding a method named ServeHTTP
to TextHandler
, we implicitly satisfy the http.Handler
interface. This interface requires any type with a ServeHTTP
method to handle HTTP requests.
The main
function demonstrates the usage of TextHandler
as the handler for an HTTP server. When the server receives a request, it invokes the ServeHTTP
method of TextHandler
, which simply writes the string content to the response.
Same with TextHandlerSecond if this is used as a Handler in Server then the TextHandlerSecond method will be executed because it matches the method signature of Handler.
package main
import (
"context"
"log"
"net/http"
"os"
)
type TextHandler string
type TextHandlerSecond string
func main() {
server := http.Server{
Addr: ":8080",
Handler: TextHandler("hii its Nakul"),
}
go server.ListenAndServe()
req, _ := http.NewRequestWithContext(context.TODO(), "GET", "http://localhost:8080", nil)
resp, err := new(http.Client).Do(req)
if err != nil {
log.Fatal("err in requesting..")
}
defer resp.Body.Close()
resp.Write(os.Stdout)
}
func (t TextHandler) ServeHTTP(w http.ResponseWriter, r *http.Request) {
w.Write(([]byte(t)))
}
func (t TextHandlerSecond) ServeHTTP(w http.ResponseWriter, r *http.Request) {
w.Write([]byte(t))
}
This example showcases how Go interfaces allow different types, in this case, a string type, to be treated uniformly when they fulfill the expected interface methods. Understanding interfaces is essential for writing flexible and modular Go code, promoting code reuse and maintainability.
Subscribe to my newsletter
Read articles from Nakul Bhardwaj directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
