Strategic Roadmap for Coding a Gojek Clone App
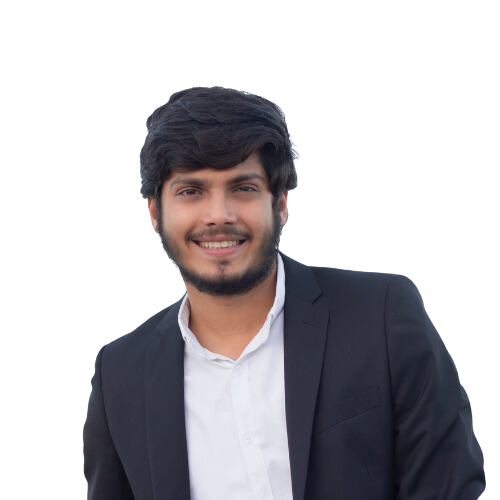
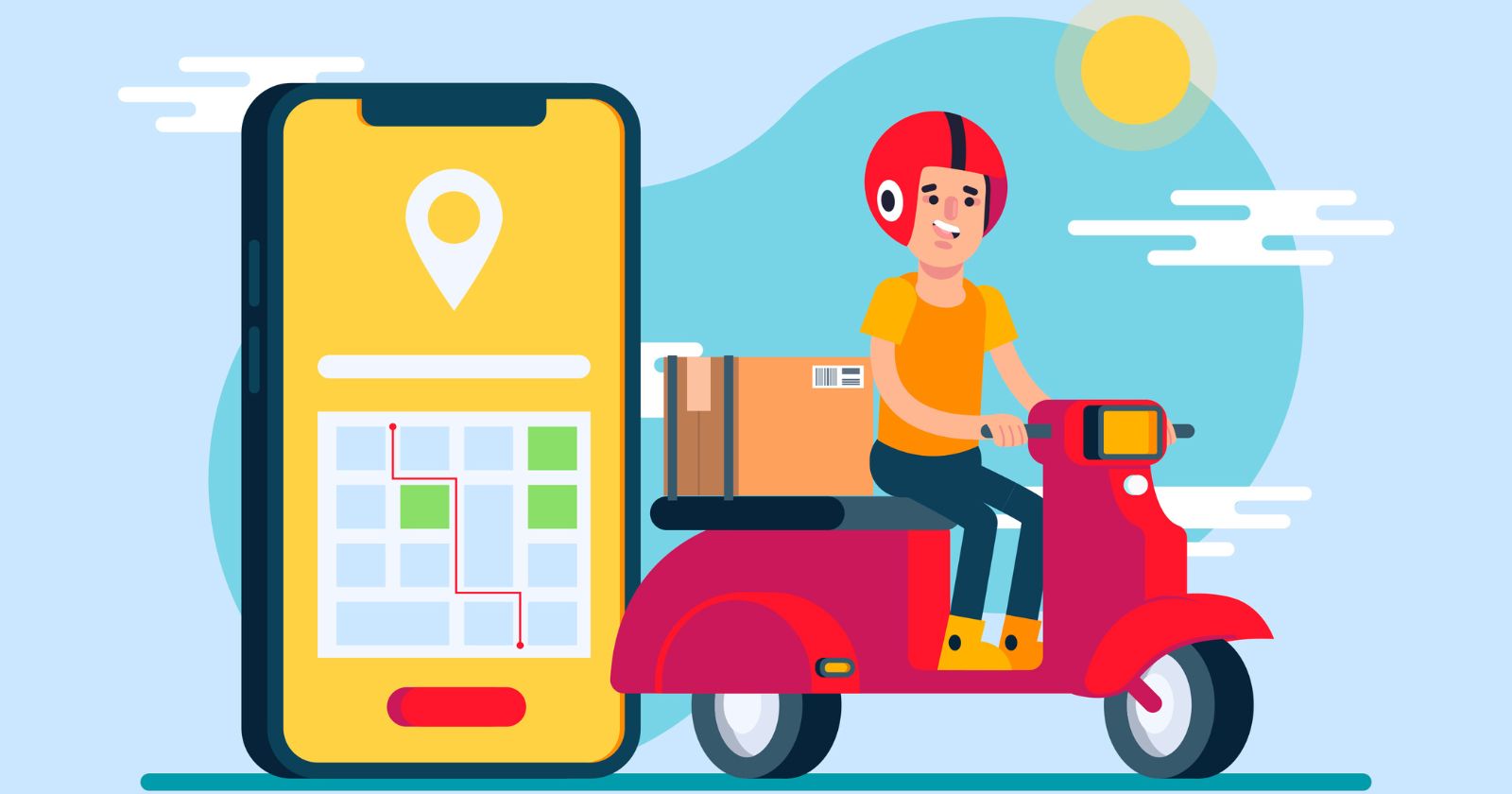
Gojek is a popular on-demand multi-service platform based in Indonesia that allows users to book transportation, food delivery, payments and various on-demand services through a mobile app. Some of Gojek's key features include ride-hailing, food delivery, payments and other on-demand services.
This blog aims to provide a strategic roadmap for developing a Gojek clone app by breaking down the required steps into manageable phases. We will analyze Gojek's business model, define key technical requirements, design the app's infrastructure and integration points as well as strategies for launch and growth.
By following this roadmap, aspiring entrepreneurs and developers can build a sustainable Gojek-like platform for their target market.
Market Research
The first step is to analyze the on-demand transportation and food delivery industry landscape in the target market. Some aspects to research include:
Size and growth projections of the ride-hailing/food delivery market
Key players operating in the space and their USPs
Prevailing economic and infrastructure conditions
Demand patterns and price points
Potential target customer segments
Research Gojek's business model to understand what made them successful. Key aspects include their multiple service verticals, heavy investment in subsidies and promotions to scale rapidly. Visit: https://zipprr.com/gojek-clone/
Conduct surveys to validate ideas and gauge customer demand. Some questions could be:
Which on-demand services would be most useful?
Willingness to pay various price points
Preferred payment methods
Pain points with existing transportation options
# Sample Python code to analyze survey responses
import pandas as pd
survey = pd.read_csv('survey_responses.csv')
print(survey.groupby(['service_preference'])['responses'].count())
The findings will help customize the product-market fit and monetization strategies.
Technology Stack
For the core ride-hailing/booking functionality, a real-time transportation platform like Grab/Uber is recommended. Some suitable frameworks are:
Android: Java or Kotlin
iOS: Swift or Objective-C
Backend: Node.js, Python Django or Flask
Database: PostgreSQL, MySQL or MongoDB
Maps: Google Maps or Mapbox APIs
Payments: Braintree, Stripe or PayPal APIs
Push: Firebase Cloud Messaging
Analytics: Google Analytics
// Sample Node.js/Express API
const express = require('express');
const app = express();
app.get('/rides', (req, res) => {
Ride.find()
.then(rides => res.json(rides))
.catch(err => res.status(400).json('Error: ' + err));
});
app.listen(5000, () => console.log('Server started on port 5000'));
These enable smooth driver-rider matching, real-time tracking, efficient mapping, seamless payments and analytics.
App Requirements and Wireframes
The app must allow users to:
Register/Login securely
Book rides and deliveries
Track bookings in real-time
Make and receive payments
Save favorite addresses
View transaction history
Rate drivers/orders
Some sample wireframes:
![App Wireframes][]
Break development into phases - Registration/Login (MVP), Ride Booking, Driver Matching, Payments, Delivery Management etc.
This allows swift product-market fit validation and gradual enhancements.
Database Design
The main entities to model in the database are:
Users (name, email, location, wallet)
Vehicles (make, model, plate, driver)
Rides/Orders (origin, destination, fare, status)
Drivers (name, vehicle, rating)
Payments (user, amount, status)
A sample schema could be:```sql
CREATE TABLE users ( id SERIAL PRIMARY KEY, name VARCHAR(100), email VARCHAR(255), location VARCHAR(255)
);
CREATE TABLE vehicles ( id SERIAL PRIMARY KEY, driver_id INTEGER REFERENCES drivers(id), make VARCHAR(50), model VARCHAR(25), plate VARCHAR(10)
);```
Indexes should be added on frequent search columns. Tables may be denormalized for performance. Start schema can evolve based on usage patterns.
Map Integration
Integrate the Google Maps JavaScript API to:
Display real-time vehicle and order locations
Calculate driving directions and estimated fares
Autocomplete places for pickup/drop
Optimize driver-order matching
Sample code:```js
// Display map
function initMap() { map = new google.maps.Map(document.getElementById('map'), { center: {lat: -34.397, lng: 150.644}, zoom: 8 });
}
// Show vehicle location
map.addMarker({ position: vehicle.location, map: map
});```
Proper error handling and authentication must be implemented. Regularly stay up-to-date with API changes.
Payment Gateway Integration
Integrate leading payment gateways based on the target market:
Braintree for credit/debit cards
Razorpay for UPI/wallets in India
Stripe for global coverage
PayPal for international transfers
Sample PHP code to make a payment:```php
// Make payment
$result = Braintree_Transaction::sale([ 'amount' => '10.00', 'paymentMethodNonce' => $nonce, 'options' => [ 'submitForSettlement' => true ]
]);
if ($result->success) { // payment successful
}```
Handle callbacks, refunds etc. Test integrations thoroughly and add error handling. Compliance with local payment regulations is important.
Driver Onboarding
Design an intuitive driver app allowing them to:
Easily register with minimal details
View ride requests on a live map
Navigate to passenger and start trips
Accept cash and digital payments
View earnings and incentives
Some incentives could be:
Sign up bonus for completing 'X' rides
Peak hour surge pricing
Rewards for maintaining high ratings
Sample driver registration page:![Driver Registration][]
Prioritize quick KYC and verification to get drivers active fast. Smooth on-trip experience will boost retention.
User Onboarding
Make the user onboarding flow simple yet secure:
Intuitive registration form collecting minimal necessary details
One-click social logins using Google/Facebook
Email verification for contact details
Allow guest booking for trial
Onboarding walking users through key features
Sample registration form:```html<form method="POST"> <input type="text" name="name" placeholder="Full Name"> <input type="email" name="email" placeholder="Email Address"> <input type="password" name="password" placeholder="Password"> <button type="submit">Register</button>
</form>```
Login and forgot password flows should also be seamless. Consider 2-factor authentication for safety.
App Distribution and Launch
With the core app developed, focus on:
Alpha and beta testing by drivers, users and friends/family
Gradually expanding the testing base
Iteratively fixing bugs based on feedback
App Store/Play Store optimization and listing
Marketing - Influencers, organic SEM/SEO, referral programs
Pricing - Commission models for drivers, subscription for premium features
Gradual geographical expansion
Some sample marketing tactics:
Vlogger promotions - Giving Vloggers free rides
Social media ads - Targeting local communities
Offers on food/other apps - Partnerships for cross promotion
PR - Pitching local tech publications about the new app
Go live step-by-step based on early signs of product-market fit. Continued marketing will help scale userbase.
Post Launch Growth
After initial launch, focus on:
Analysis of early usage patterns from Analytics
Feedback surveys to understand pain points
Expanding to new service categories based on demand
Driver growth programs - Fleet partnerships, leasing schemes
Performance optimization to handle scale
Progressive web app version for lower bandwidth areas
Internationalization - Adapting UI and payment systems
Consider Africa and Southeast Asian Expansion
Here is an example of weekly usage dashboard:![Usage Dashboard][]
Use data insight to refine strategies for maximum engagement and monetization over time. Listen closely to the evolving needs of drivers as well as users. Continuous iteration based on metrics is key to sustained growth.
Conclusion
By following this strategic roadmap, developers can efficiently code and launch a Gojek clone app tailored for their target market. Regular research, testing, feedback analysis and iterations based on data-driven insights will be crucial for the startup's success.
The foundational phases around market analysis, technology choices and infrastructure lay the groundwork for a strong product. Gradual roll-out of key features ensures timely validation of assumptions.
Driver and user onboarding experiences set the tone for future engagement levels. Integrations with maps and payments enable core product functionalities smoothly.
The app launch should be complemented by optimized distribution and an engaging marketing strategy. Continuous observations from analytics dashboards guide further improvements.
Internationalization in phases opens new avenues for growth. Additional services can be rolled out organically based on emerging needs. Partnerships and an engaged community strengthen the platform.
With diligent implementation of each step, budding entrepreneurs have a shot at building the 'Gojek of their market'. Regular innovation and adaptation as per evolving landscapes will determine long term gains. Overall, this roadmap presents a systematic approach to coding and scaling a sustainable multi-service platform.
Subscribe to my newsletter
Read articles from prasad venkatachalam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
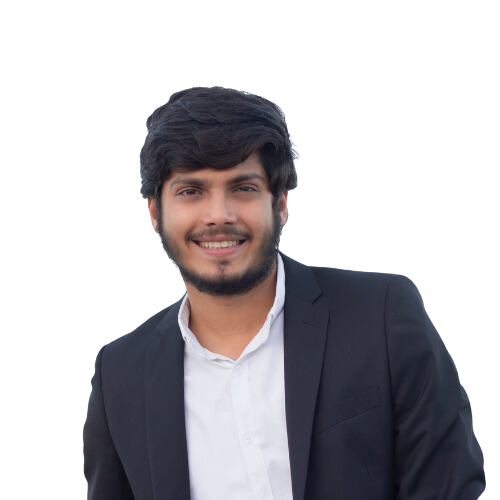
prasad venkatachalam
prasad venkatachalam
With over 10 years in web and app development, I'm more interested in writing about clone solutions of popular brands.