#BuckshotRoulette Python Dealer AI
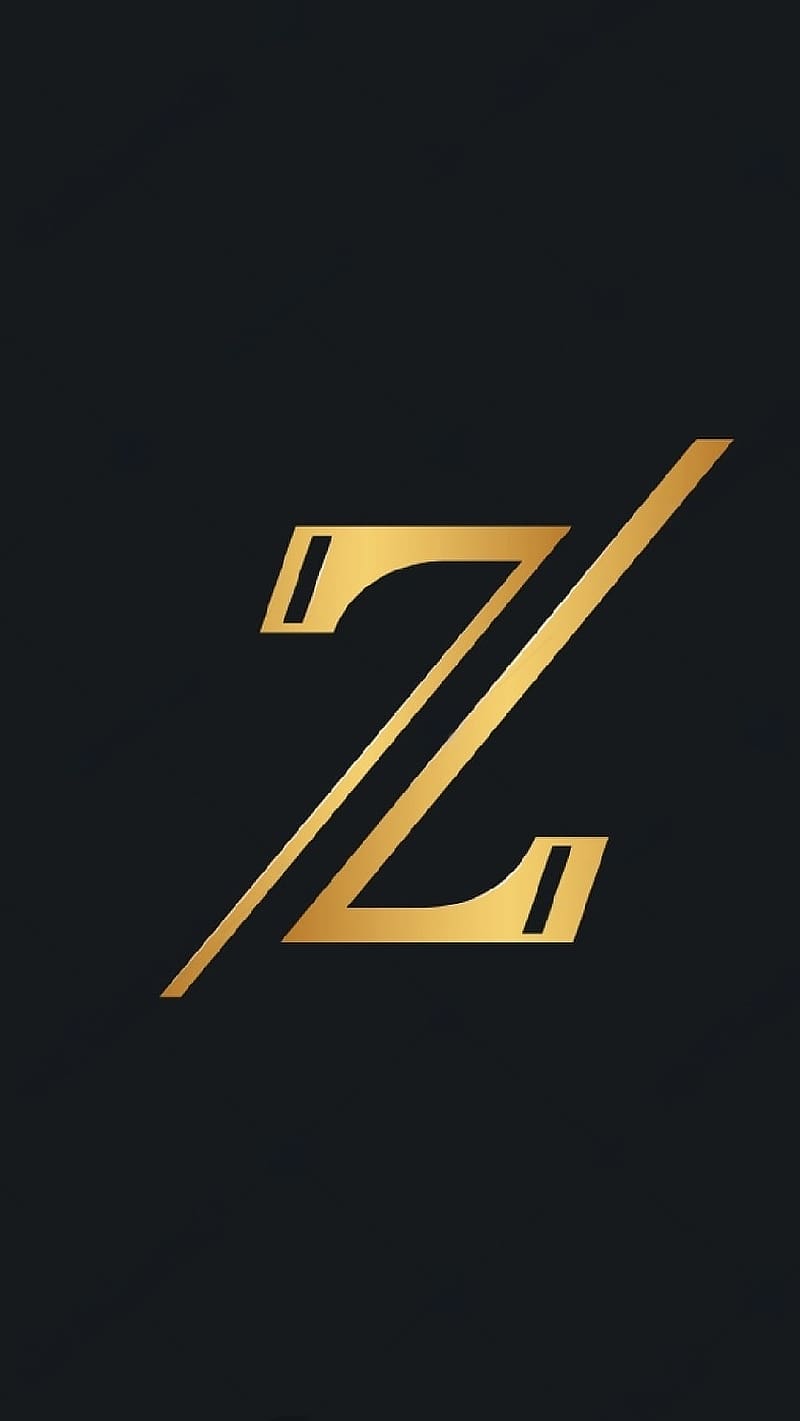
Table of contents
Hi students! Today I'm gonna show you how can you make a Dealer AI in python.
Requirements:
Python3
Tutorial:
Firstly , we are going to import "random library" with we will use for making choices.
import random
Afterwards , we will make the variables for our code. We will also select the actual_round with the random library.
# Library
import random # Comes with python3, using for random number generation
# Variables
items = [] # Add "Magnifiying_Glass" if you want the dealer to see the bullet.
actual_round = None # Actual Round
round = None # Round that dealer knows
# Code
rng2 = random.randint(0,1) # Random number : gives us 1 or 0 randomly.
if rng2 == 0: # If the random number is 0
actual_round = "Shoot" # Make the actual round "Shoot" (Live)
elif rng2 == 1: # If the random number is 1
actual_round = "Blank" # Make the actual round Blank
Now that we have simple things set-up, we are going to make the dealer loop through the items table so that he can check if there is magnifying glass in it and use it.
#Rest of the code here
for item in items: # Looping through the items
if item == "Magnifiying_Glass": # Is the item a magnifiying glass?
print("Seen") # Yes, the item is magnifiying glass.
round = actual_round # Makes me know the actual_round.
if round == "Shoot": # If the round is shoot (live)
print("I am gonna shoot! Using Handsaw") # I shoot him
# Use handsaw code here.
Now that dealer has look through his inventory and made his choice if he has a magnifying glass , lets make some decisions even when we don't have a magnifying glass!
Firstly , we will make functions for shooting ourselves and the opponent.
# Library
import random # Comes with python3, using for random number generation
# Variables
items = [] # Add "Magnifiying_Glass" if you want the dealer to see the bullet.
actual_round = None # Actual Round
round = None # Round that dealer knows
# Code
rng2 = random.randint(0,1) # Random number : gives us 1 or 0 randomly.
if rng2 == 0: # If the random number is 0
actual_round = "Shoot" # Make the actual round "Shoot" (Live)
elif rng2 == 1: # If the random number is 1
actual_round = "Blank" # Make the actual round Blank
def shoot_itself(): # Gonna shoot myself
print("Shot itself") # Shot myself
if actual_round == "Blank": # Is the round blank?
print("I dodged a bullet") # Yes, I survive.
else:
print("Ouch") # Ouchies , I shot myself.
return None
def shoot_opponent(): # Gonna shoot him
print("Shot him") # Shot him
if actual_round == "Shoot": # Is the round live?
print("Time to smoke a pack") # Yes I shot him.
else:
print("I wasted it.") # No it was blank , I wasted it.
return None
for item in items: # Looping through the items
if item == "Magnifiying_Glass": # Is the item a magnifiying glass?
print("Seen") # Yes, the item is magnifiying glass.
round = actual_round # Makes me know the actual_round.
if round == "Shoot": # If the round is shoot (live)
print("I am gonna shoot! Using Handsaw") # I shoot him
# Use handsaw code here.
Now that we have made functions for shooting , we are going to decide with one to do!
if not round == None: # If we know the round
if round == "Blank": # If the round we know is blank
shoot_itself() # We shoot ourselfs
elif round == "Shoot": # If the round we know is shoot (live)
shoot_opponent() # We shoot him/her.
elif round == None: # If we dont know the round
rng3 = random.randint(0,1) # Random number ( 0 or 1)
if rng3 == 1: # If the random number is 1
shoot_opponent() # I shoot him/her.
if rng3 == 0: # If the random number is 0
shoot_itself() # I shoot myself.
There you go folks! You just made the dealer AI. Even thou we don't have code for using the other items it's just a loop through the items. Now for the full code:
# Library
import random # Comes with python3, using for random number generation
# Variables
items = [] # Add "Magnifiying_Glass" if you want the dealer to see the bullet.
actual_round = None # Actual Round
round = None # Round that dealer knows
# Code
rng2 = random.randint(0,1) # Random number : gives us 1 or 0 randomly.
if rng2 == 0: # If the random number is 0
actual_round = "Shoot" # Make the actual round "Shoot" (Live)
elif rng2 == 1: # If the random number is 1
actual_round = "Blank" # Make the actual round Blank
def shoot_itself(): # Gonna shoot myself
print("Shot itself") # Shot myself
if actual_round == "Blank": # Is the round blank?
print("I dodged a bullet") # Yes, I survive.
else:
print("Ouch") # Ouchies , I shot myself.
return None
def shoot_opponent(): # Gonna shoot him
print("Shot him") # Shot him
if actual_round == "Shoot": # Is the round live?
print("Time to smoke a pack") # Yes I shot him.
else:
print("I wasted it.") # No it was blank , I wasted it.
return None
for item in items: # Looping through the items
if item == "Magnifiying_Glass": # Is the item a magnifiying glass?
print("Seen") # Yes, the item is magnifiying glass.
round = actual_round # Makes me know the actual_round.
if round == "Shoot": # If the round is shoot (live)
print("I am gonna shoot! Using Handsaw") # I shoot him
# Use handsaw code here.
if not round == None: # If we know the round
if round == "Blank": # If the round we know is blank
shoot_itself() # We shoot ourselfs
elif round == "Shoot": # If the round we know is shoot (live)
shoot_opponent() # We shoot him/her.
elif round == None: # If we dont know the round
rng3 = random.randint(0,1) # Random number ( 0 or 1)
if rng3 == 1: # If the random number is 1
shoot_opponent() # I shoot him/her.
if rng3 == 0: # If the random number is 0
shoot_itself() # I shoot myself.
Don't forget to follow me on twitter :) : https://twitter.com/Zogu_X
If you have any questions feel free to contact me.
Subscribe to my newsletter
Read articles from Oğuzhan Kırbaş directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
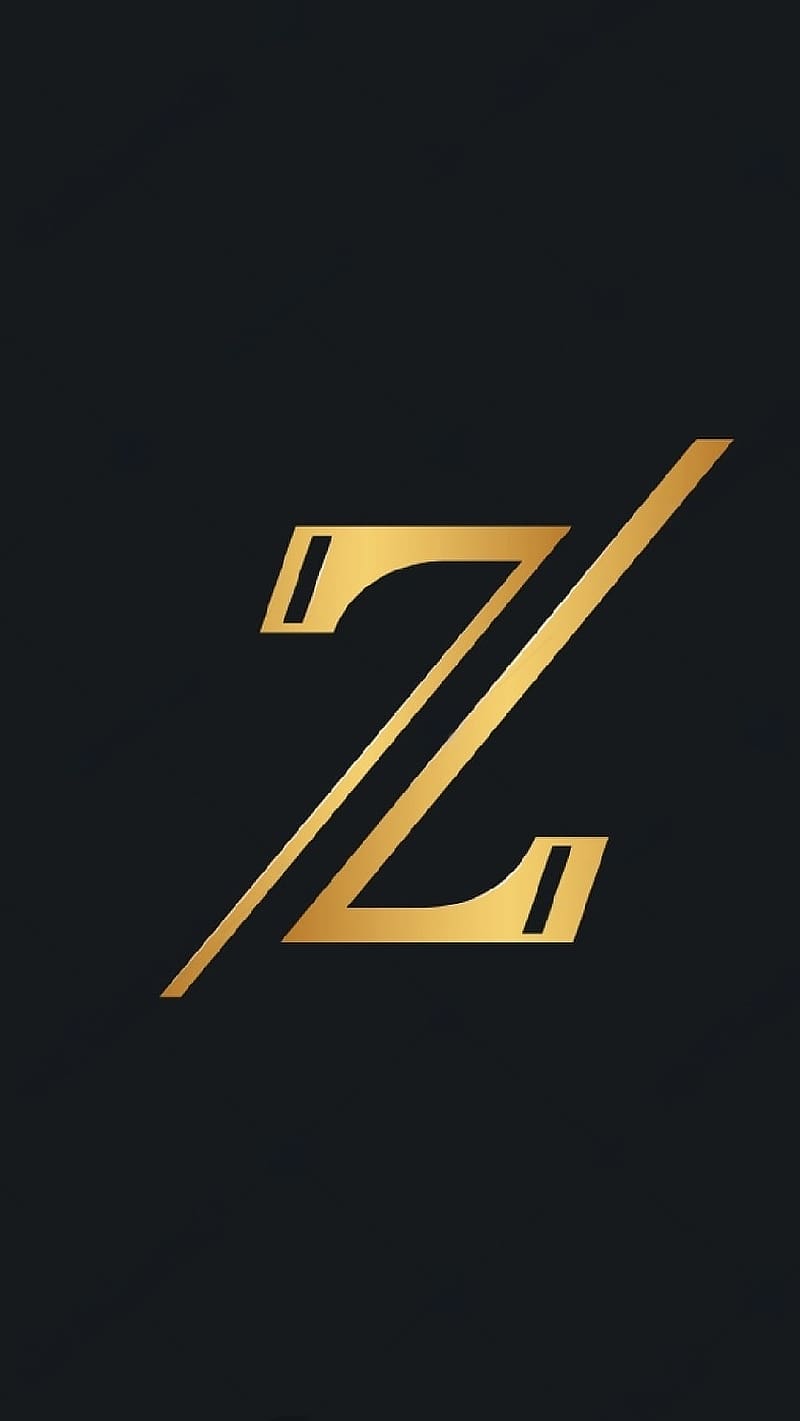