Size of Datatypes in Java!

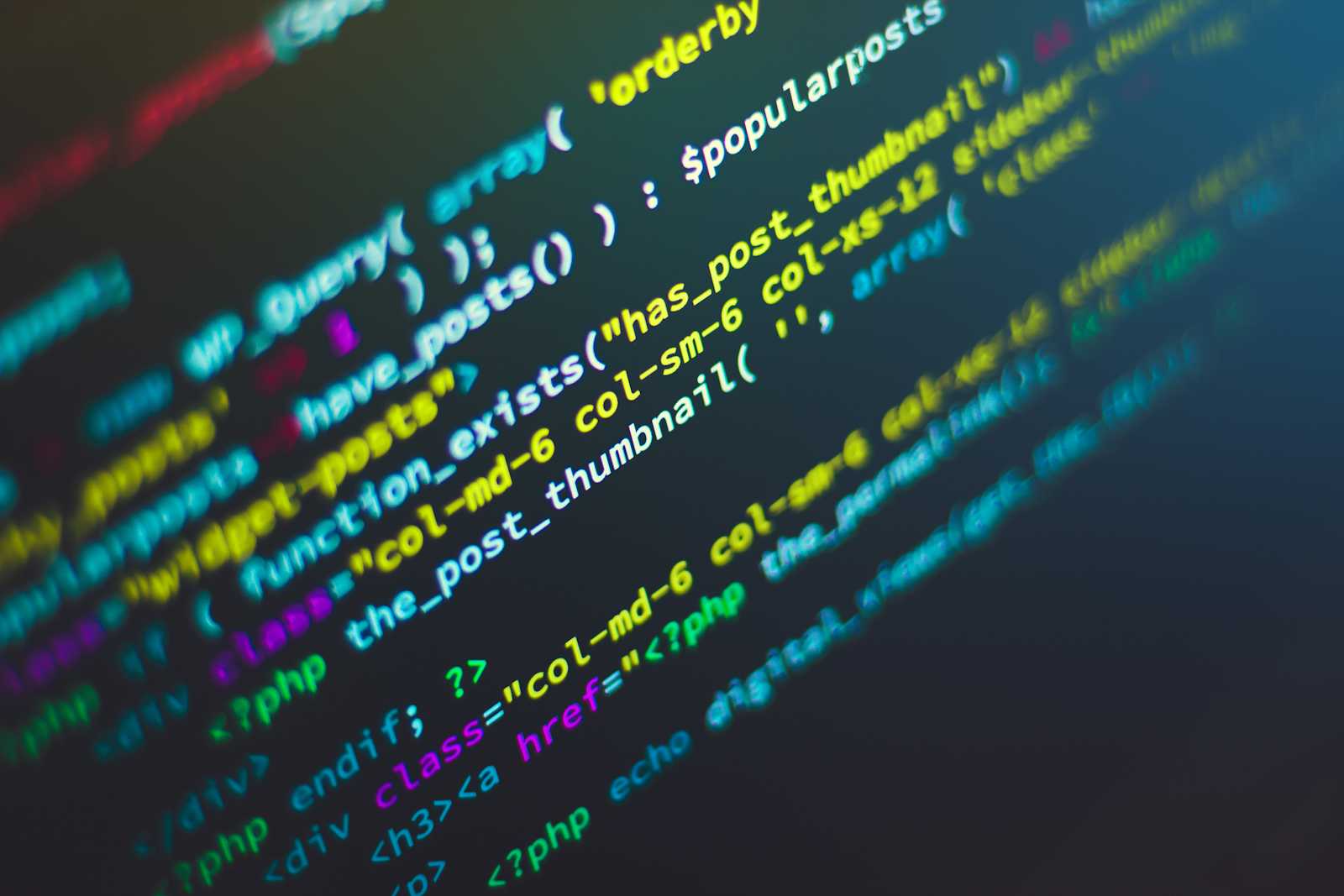
Java has been one of the most popular programming languages. Each data type is predefined withing the programming language which makes Java a statically and strongly typed language. Variables and constants must be specified with one of the predefined Java data types. Two categories of data types are available in Java based on how they store and manage data in a programming language.
Primitive Data Type: The basic data types which are predefined and are directly supported by the language. These are stored in the stack memory.
Non-Primitive Data Type: These data types are not predefined by the programming language. These are created by the programmers. These are stored in the heap memory.
Below are the primitive and non-primitive data types available in Java.
There is no sizeof() operator in Java like in C++ to know the size of the data types in the code. However, in order to achieve this we need to use the wrapper class using datatype.SIZE. The answer will be in the bits so we can divide it by 8 to convert it into bytes format. [ 1 byte = 8 bits]
Syntax for finding size of primtive data types using wrapperlass:
public class Main{
public static void main(String[] args){
System.out.println("Size of float is " + (Float.SIZE/8) + " bytes");
System.out.println("Size of double is " + (Double.SIZE/8) + " bytes");
System.out.println("Size of int is " + (Integer.SIZE/8) + " bytes");
System.out.println("Size of short is " + (Short.SIZE/8) + " bytes");
System.out.println("Size of byte is " + (Byte.SIZE/8) + " bytes");
System.out.println("Size of long is " + (Long.SIZE/8) + " bytes");
System.out.println("Size of character is " + (Character.SIZE/8) + " bytes");
}
}
Note: Finding the size of a boolean in Java is a bit trickier due to the fact that Java doesn't specify a fixed size for a boolean. While the typical understanding is that a boolean takes up 1 bit of space, Java does not allocate memory at the bit level; it allocates memory in units of bytes. Moreover, Java uses Unicode standard for representing characters. It allows for the representation of a much larger set of characters compared to ASCII. This is the reason the size of character is 2 bytes in Java.
Subscribe to my newsletter
Read articles from Sanya Verma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sanya Verma
Sanya Verma
A coding enthusiast on the exciting journey of becoming a skilled developer.