Lazy Loading with React.lazy and Suspense
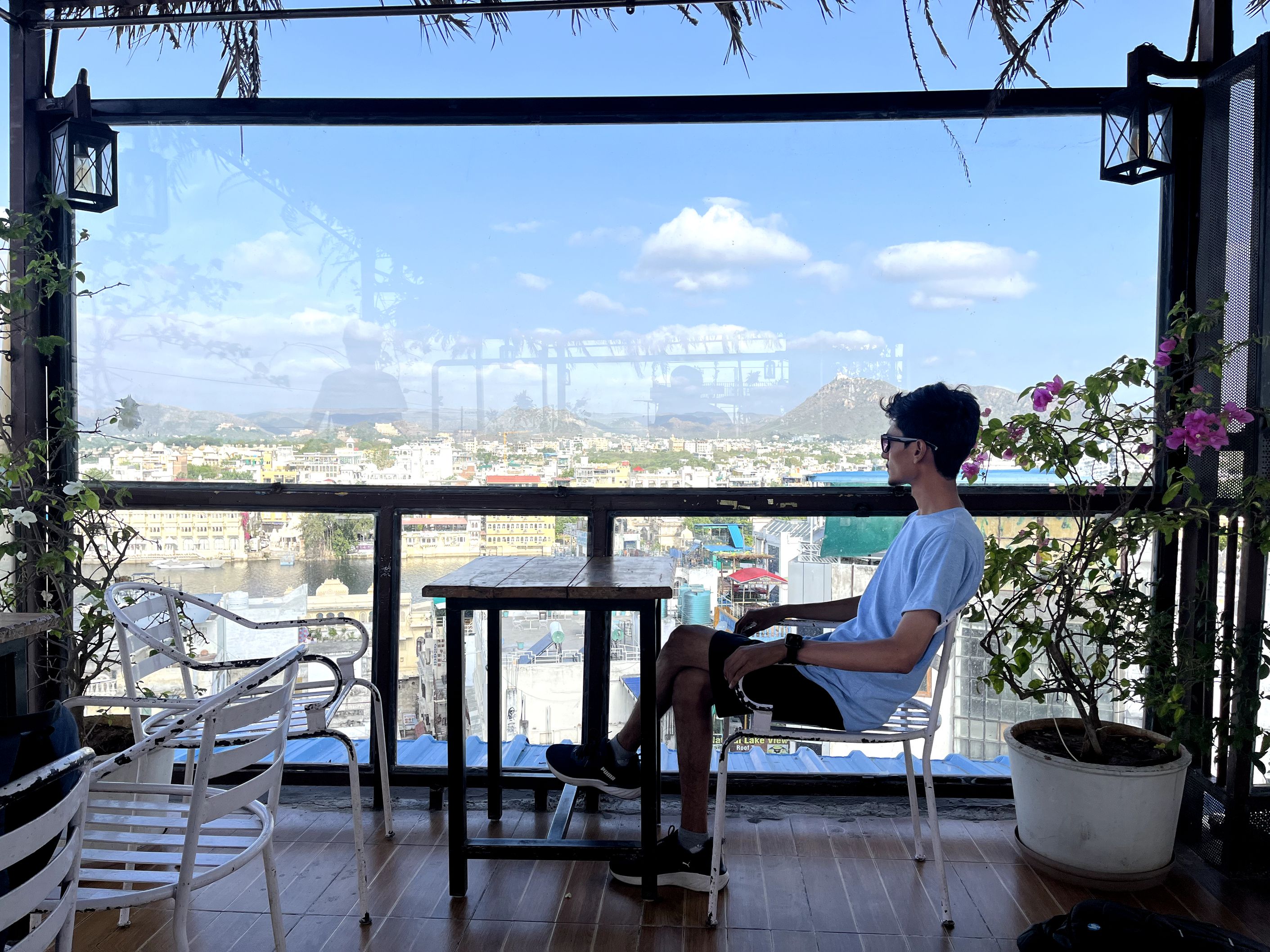
Expert-Level Explanation
React.lazy
and Suspense
in React 18 are used for lazy loading components, a technique that defers the loading of non-critical resources at page load time. It allows you to split your component code into separate chunks and load them only when needed, resulting in faster initial page load times. React.lazy
is a function that enables you to render a dynamic import as a regular component and Suspense
lets you specify the loading state (like a spinner) while waiting for the lazy component to load.
Creative Explanation
Imagine your app as a theatre play. Not all actors (components) are needed on stage (in the browser) at the start. Some can wait backstage (not loaded yet) and only come on stage (load) when it's their turn in the scene (when the user navigates to that part of the app). React.lazy
andSuspense
manage this process, ensuring smooth transitions and an engaging performance (user experience) without overwhelming the audience (browser) right at the beginning.
Practical Explanation with Code
Using React.lazy
and Suspense
:
import React, { Suspense } from 'react';
const LazyComponent = React.lazy(() => import('./LazyComponent'));
function MyComponent() {
return (
<Suspense fallback={<div>Loading...</div>}>
<LazyComponent />
</Suspense>
);
}
In this example, LazyComponent
is loaded only when MyComponent
renders, and a loading message is shown until it's loaded.
Real-World Example
On a large e-commerce site, product review sections can be loaded lazily. As a user scrolls down the product details page, the review section, which is a separate chunk, is loaded only when needed, improving the page load performance.
Subscribe to my newsletter
Read articles from Akash Thoriya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
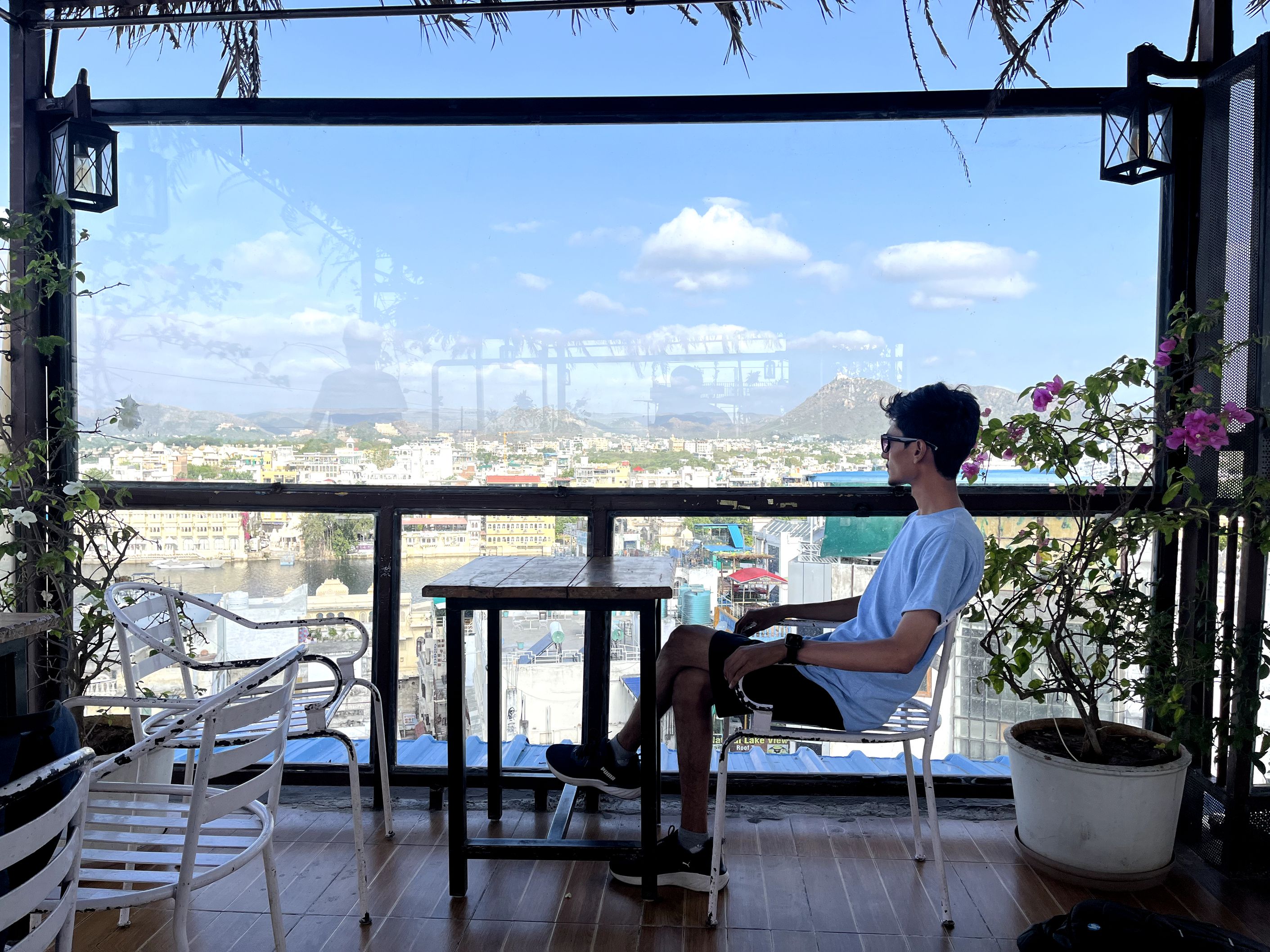
Akash Thoriya
Akash Thoriya
As a senior full-stack developer, I am passionate about creating efficient and scalable web applications that enhance the user experience. My expertise in React, Redux, NodeJS, and Laravel has enabled me to lead cross-functional teams and deliver projects that consistently exceed client expectations.