Optimizing with shouldComponentUpdate and React.memo
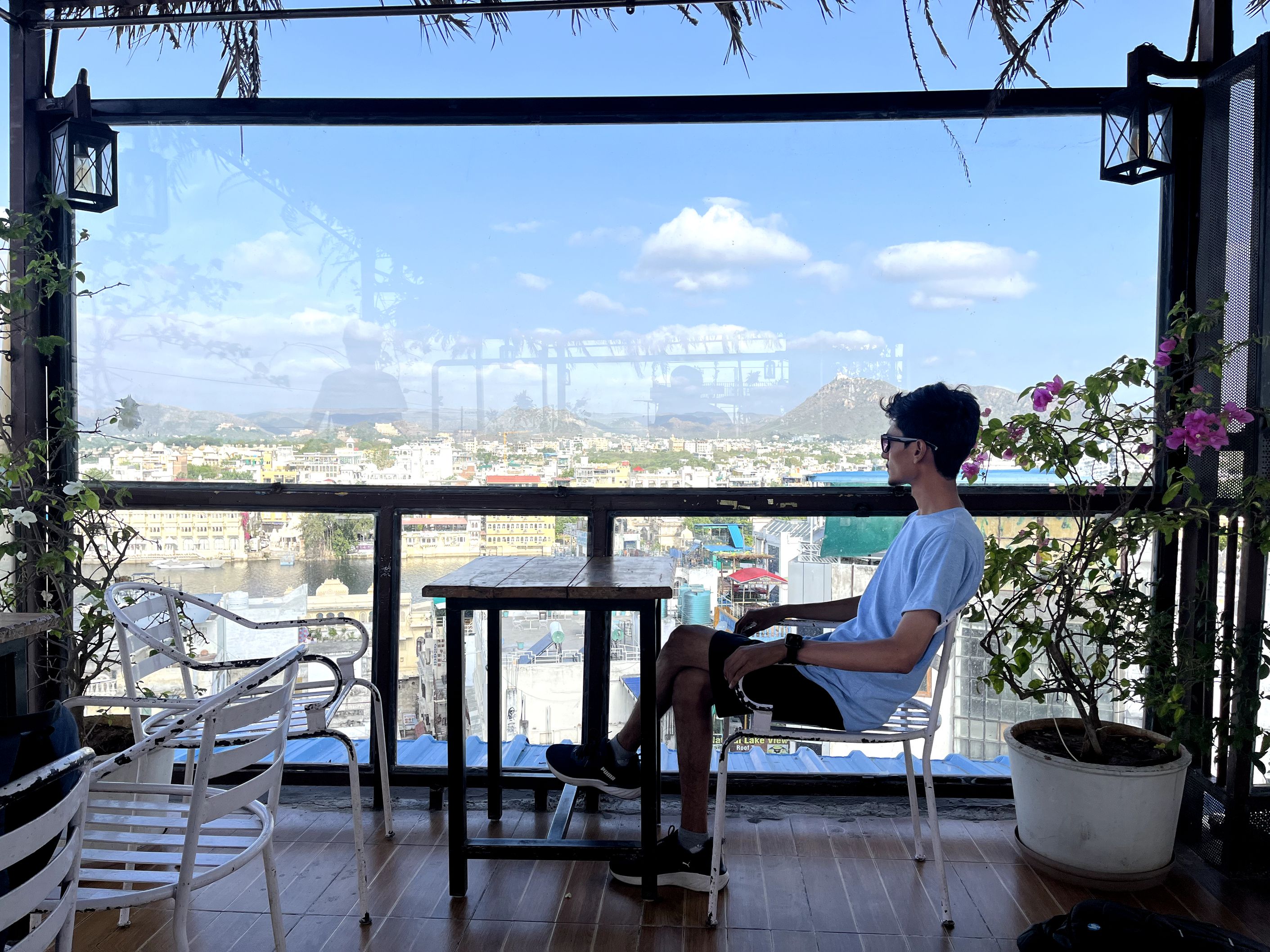
Expert-Level Explanation
shouldComponentUpdate
is a lifecycle method in class components that determines whether a component should re-render in response to a change in state or props. Returning false
from this method prevents the re-render. Functional components, React.memo
provides a similar optimisation. It performs a shallow comparison of the current and new props and re-renders the component only if they differ. Both are used to optimise performance by avoiding unnecessary renders.
Creative Explanation
Consider shouldComponentUpdate
and React.memo
as quality control inspectors in a factory. They check each product (component) before it leaves the assembly line (re-renders). If a product hasn't changed since the last inspection (no change in state or props), it's not sent back through the line (prevented from re-rendering), saving time and resources.
Practical Explanation with Code
Using shouldComponentUpdate
:
class MyComponent extends React.Component {
shouldComponentUpdate(nextProps, nextState) {
// return true or false based on some condition
return nextProps.id !== this.props.id;
}
render() {
// render logic
}
}
Using React.memo
:
const MyComponent = React.memo(function MyComponent(props) {
// render logic
});
Real-World Example
In an application with a list of users, suppose each user component has a profile picture and status. If only the status changes frequently, you could use shouldComponentUpdate
or React.memo
to avoid re-rendering the profile picture, which improves performance, especially when there are many users listed.
Subscribe to my newsletter
Read articles from Akash Thoriya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
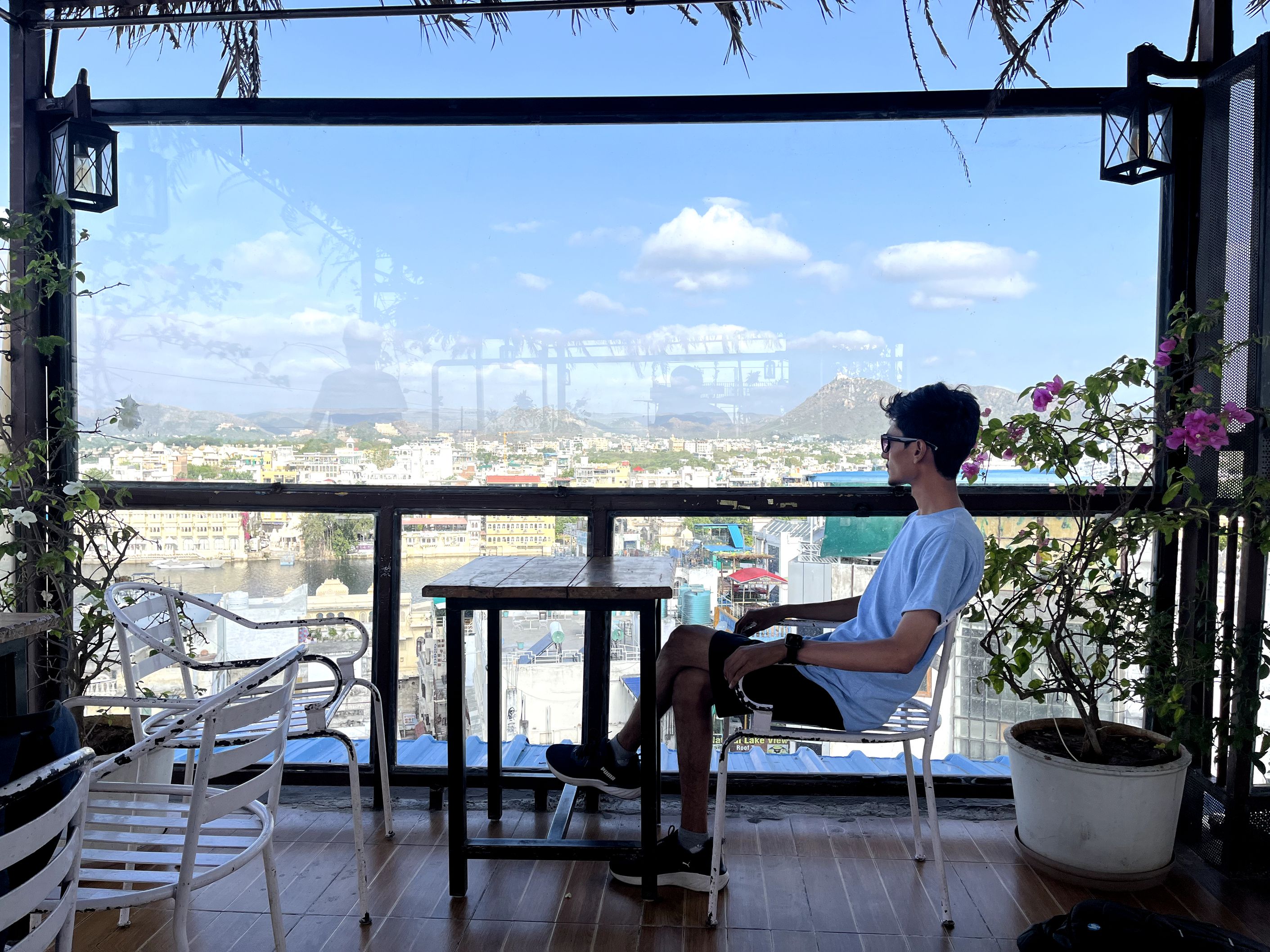
Akash Thoriya
Akash Thoriya
As a senior full-stack developer, I am passionate about creating efficient and scalable web applications that enhance the user experience. My expertise in React, Redux, NodeJS, and Laravel has enabled me to lead cross-functional teams and deliver projects that consistently exceed client expectations.