Optimize using useCallback and useMemo Hooks in React
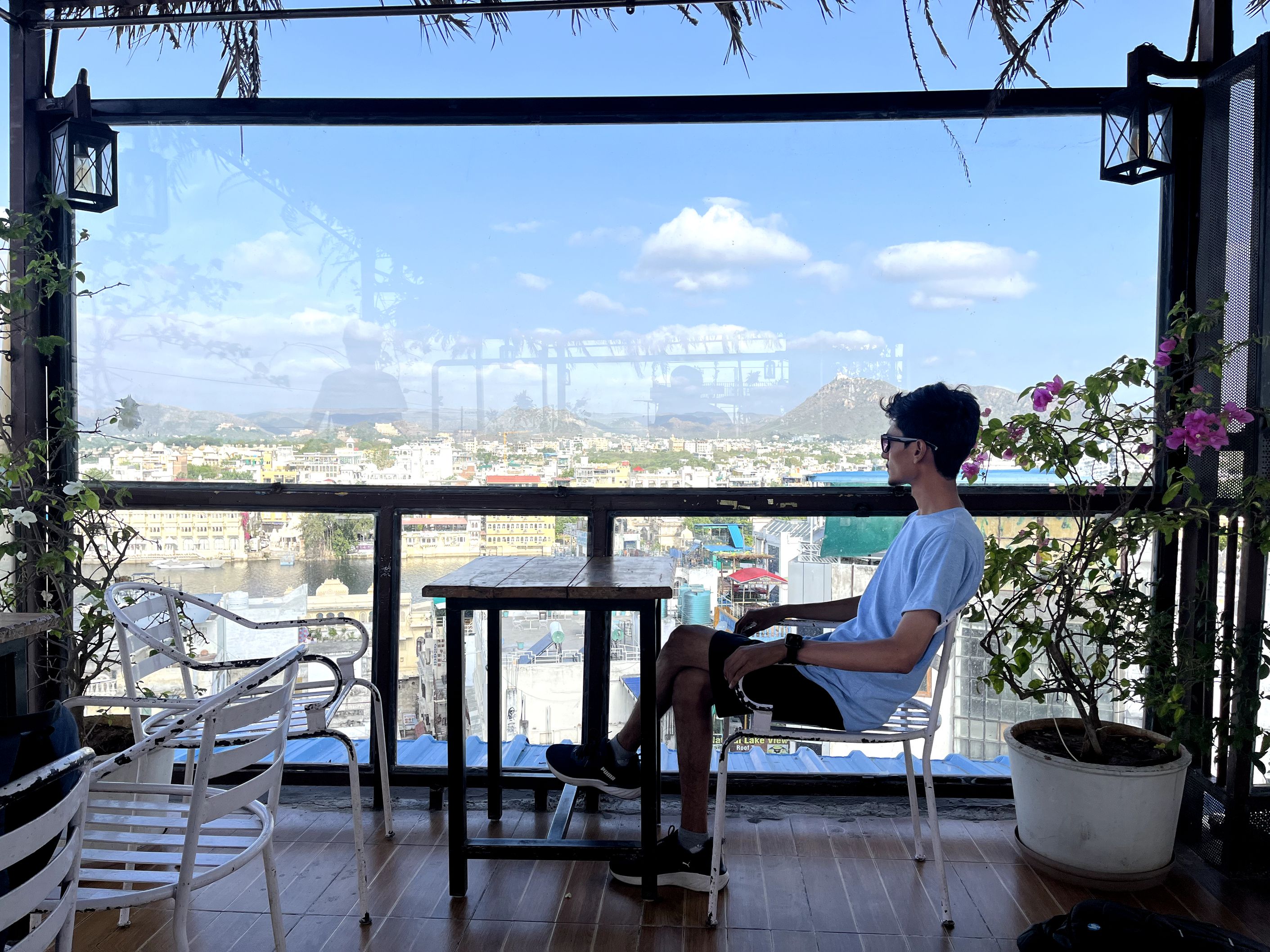
Expert-Level Explanation
useCallback
and useMemo
are hooks in React that help optimise performance. useCallback
returns a memoized version of a callback function that only changes if one of its dependencies has changed. This is useful to prevent unnecessary re-renders of child components that rely on reference equality for performance optimisations. useMemo
returns a memoized value. It recalculates the value only when one of its dependencies changes, preventing expensive recalculations on every render.
Creative Explanation
Imagine useCallback
as a smart assistant who remembers how you solved a specific problem (the callback function) and only rethinks the solution if the problem changes (dependencies change). useMemo
is like a chef who prepares a complex dish (expensive calculation) and keeps it in the fridge, reheating it (returning the memoized value) whenever you want to eat it again, unless the ingredients (dependencies) change.
Practical Explanation with Code
Using useCallback
:
import React, { useCallback } from 'react';
function MyComponent({ onButtonClick }) {
const memoizedCallback = useCallback(
() => {
onButtonClick('A parameter');
},
[onButtonClick],
);
return <button onClick={memoizedCallback}>Click me</button>;
}
Using useMemo
:
import React, { useMemo } from 'react';
function MyComponent({ list }) {
const sortedList = useMemo(() => {
return list.sort((a, b) => a - b);
}, [list]);
return <div>{sortedList.map(item => <p key={item}>{item}</p>)}</div>;
}
Real-World Example
In a contacts application with a search feature, useCallback
can be used to memoize the search query handler to avoid unnecessary re-renders of search results. useMemo
can be used to store a sorted contacts list, which is only recalculated when the contacts list or the sort order changes.
Subscribe to my newsletter
Read articles from Akash Thoriya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
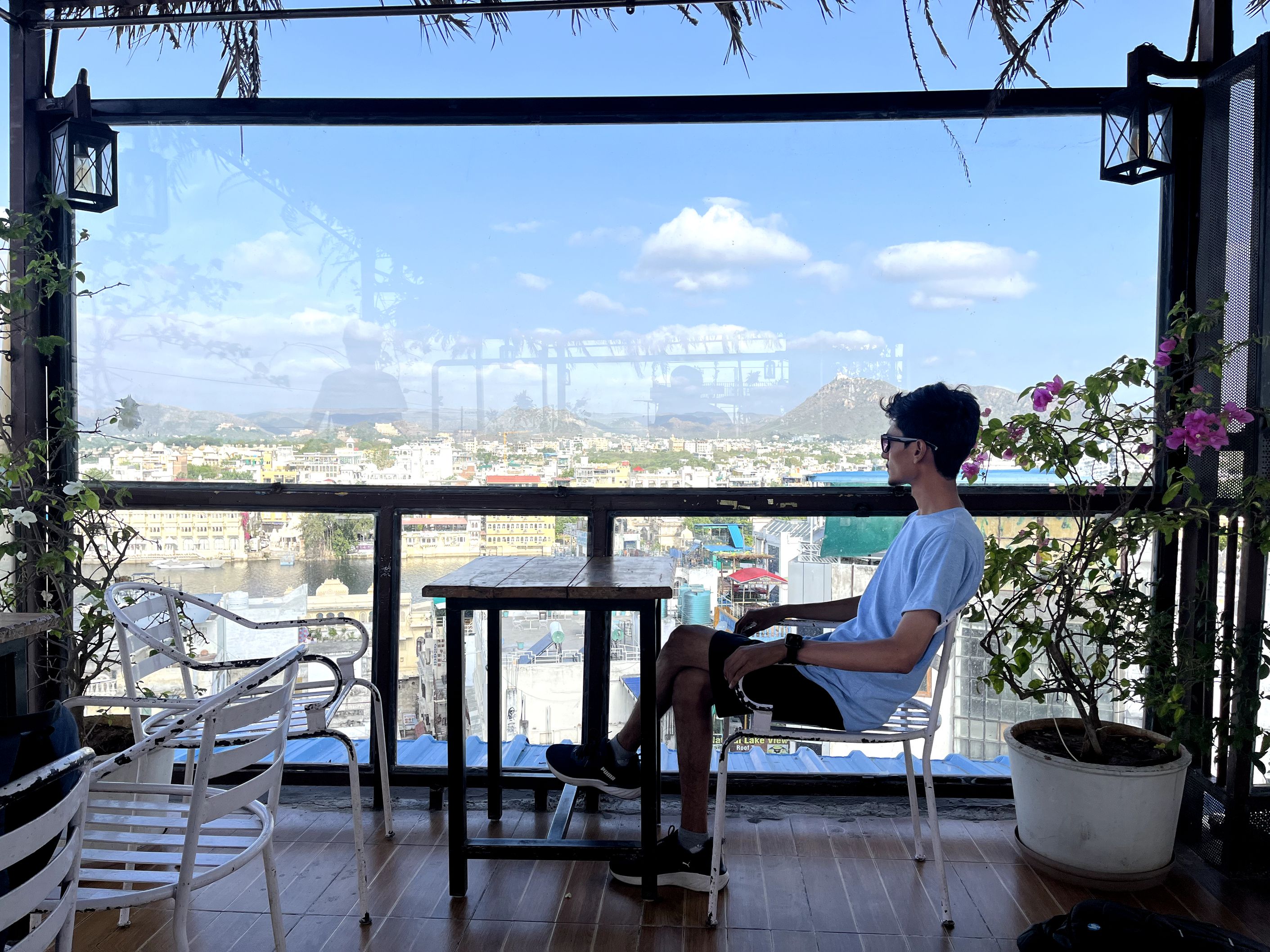
Akash Thoriya
Akash Thoriya
As a senior full-stack developer, I am passionate about creating efficient and scalable web applications that enhance the user experience. My expertise in React, Redux, NodeJS, and Laravel has enabled me to lead cross-functional teams and deliver projects that consistently exceed client expectations.