Concurrent Mode in React
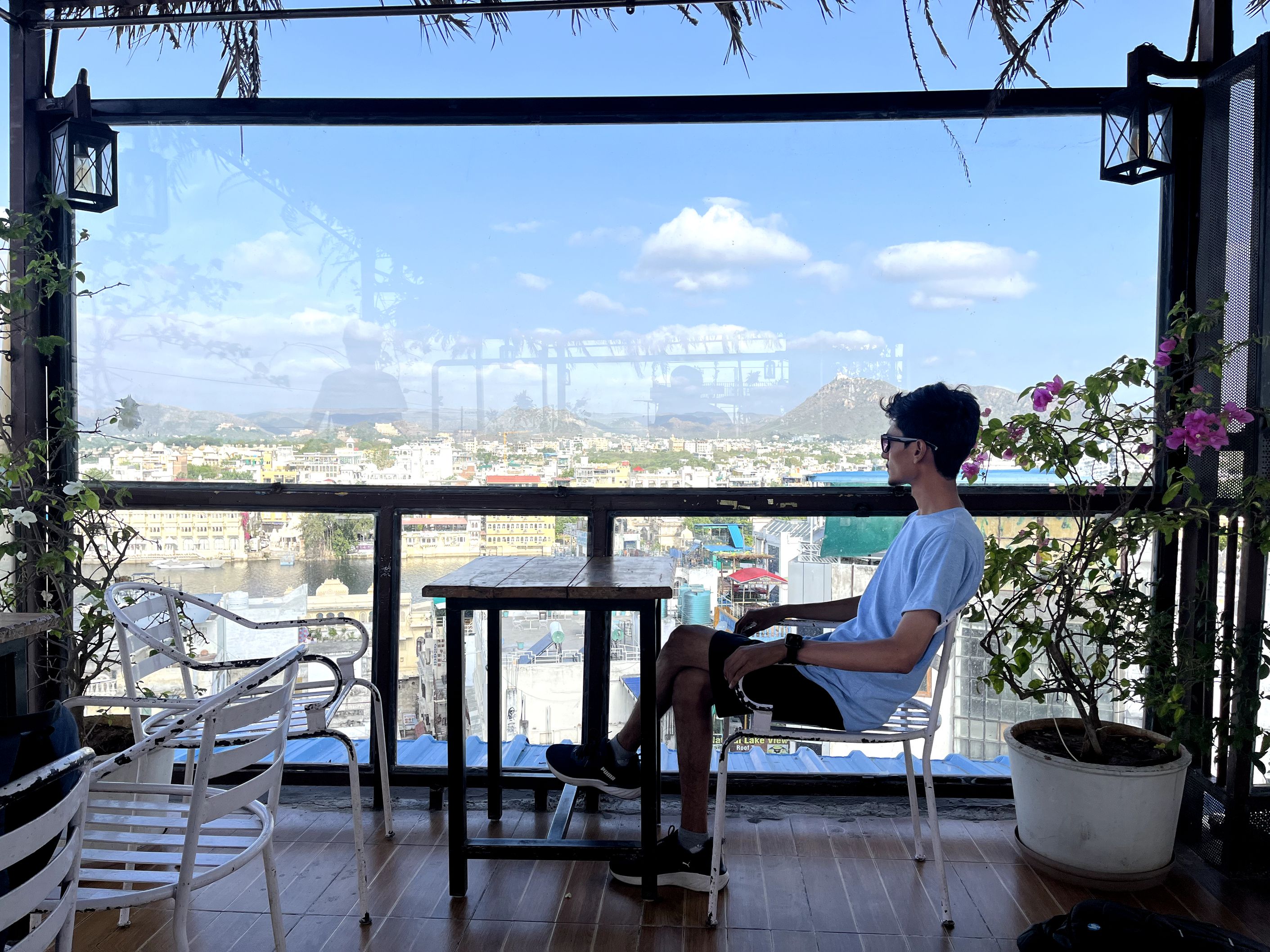
Expert-Level Explanation
Concurrent Mode is an advanced feature in React 18 that enables improved user experiences by making rendering interruptible. This mode allows React to prepare multiple versions of the UI at the same time, prioritising rendering based on user interaction and device capabilities. It helps keep the app responsive even when there is a heavy load by breaking down the UI rendering into smaller, non-blocking tasks. This is particularly useful for rendering large lists, complex animations, or fetching data.
Creative Explanation
Imagine Concurrent Mode as a skilled juggler (React) who is juggling several balls (UI updates). While juggling, if a new ball (a high-priority update like a user click) is thrown into the mix, the juggler can catch it and integrate it into the routine smoothly without dropping any balls. This keeps the performance smooth and responsive, ensuring that critical updates are handled first.
Practical Explanation with Code
Using concurrent mode requires no specific coding practices. It's more about enabling it in your React application.
import ReactDOM from 'react-dom';
import App from './App';
// Enable Concurrent Mode
ReactDOM.createRoot(document.getElementById('root')).render(<App />);
This code snippet shows how to enable concurrent mode by using ReactDOM.createRoot
instead ofReactDOM.render
.
Real-World Example
In a news feed application, as new articles are fetched and rendered, the UI might become sluggish. With Concurrent Mode, React can render these new articles in the background, ensuring the user's interactions (like clicking a link or button) are prioritised and the UI remains responsive.
Subscribe to my newsletter
Read articles from Akash Thoriya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
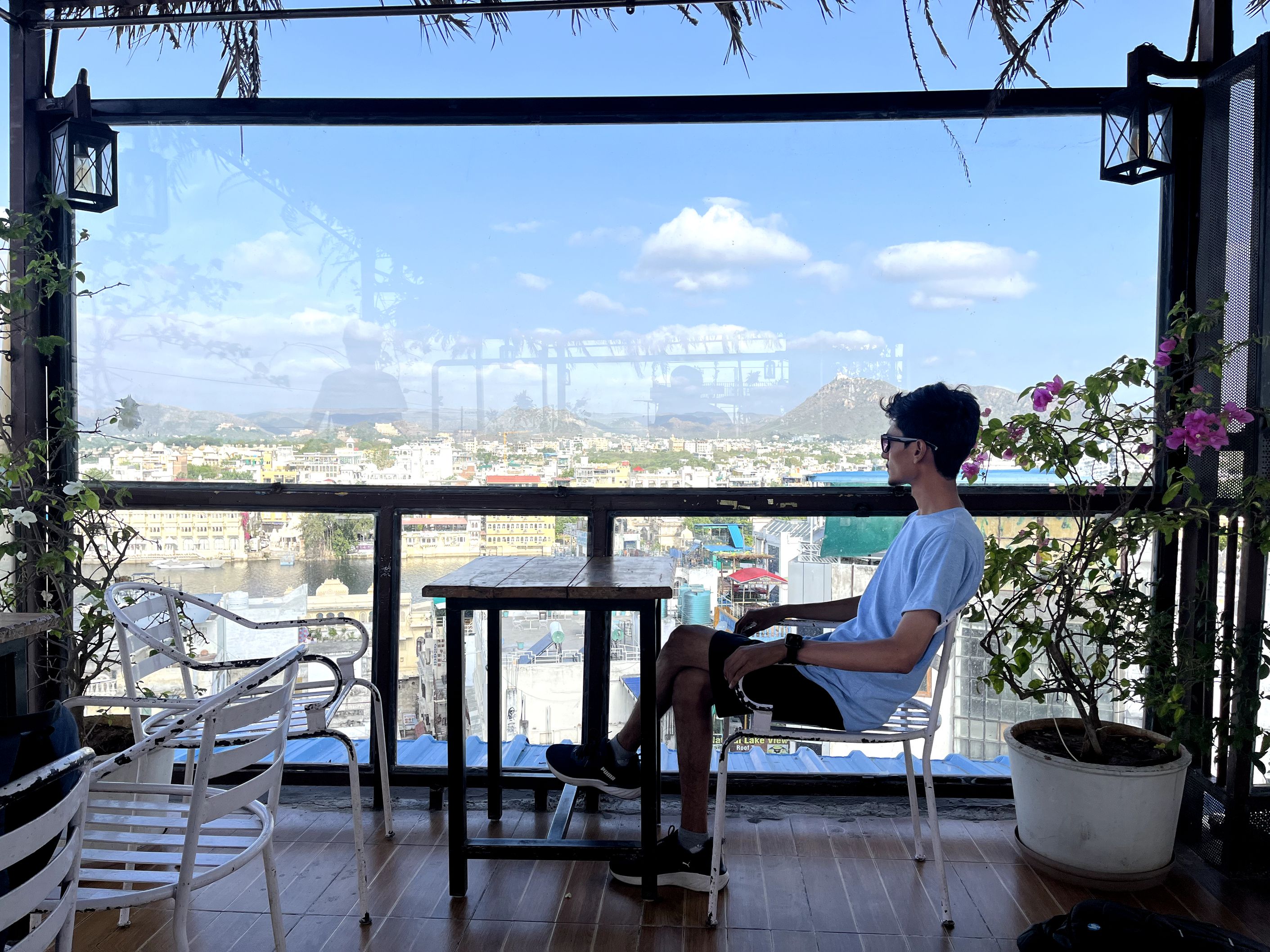
Akash Thoriya
Akash Thoriya
As a senior full-stack developer, I am passionate about creating efficient and scalable web applications that enhance the user experience. My expertise in React, Redux, NodeJS, and Laravel has enabled me to lead cross-functional teams and deliver projects that consistently exceed client expectations.