Asynchronous Programming in JavaScript
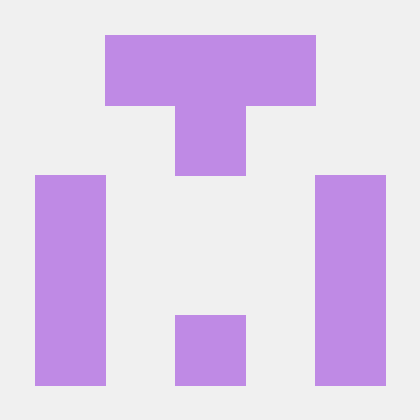
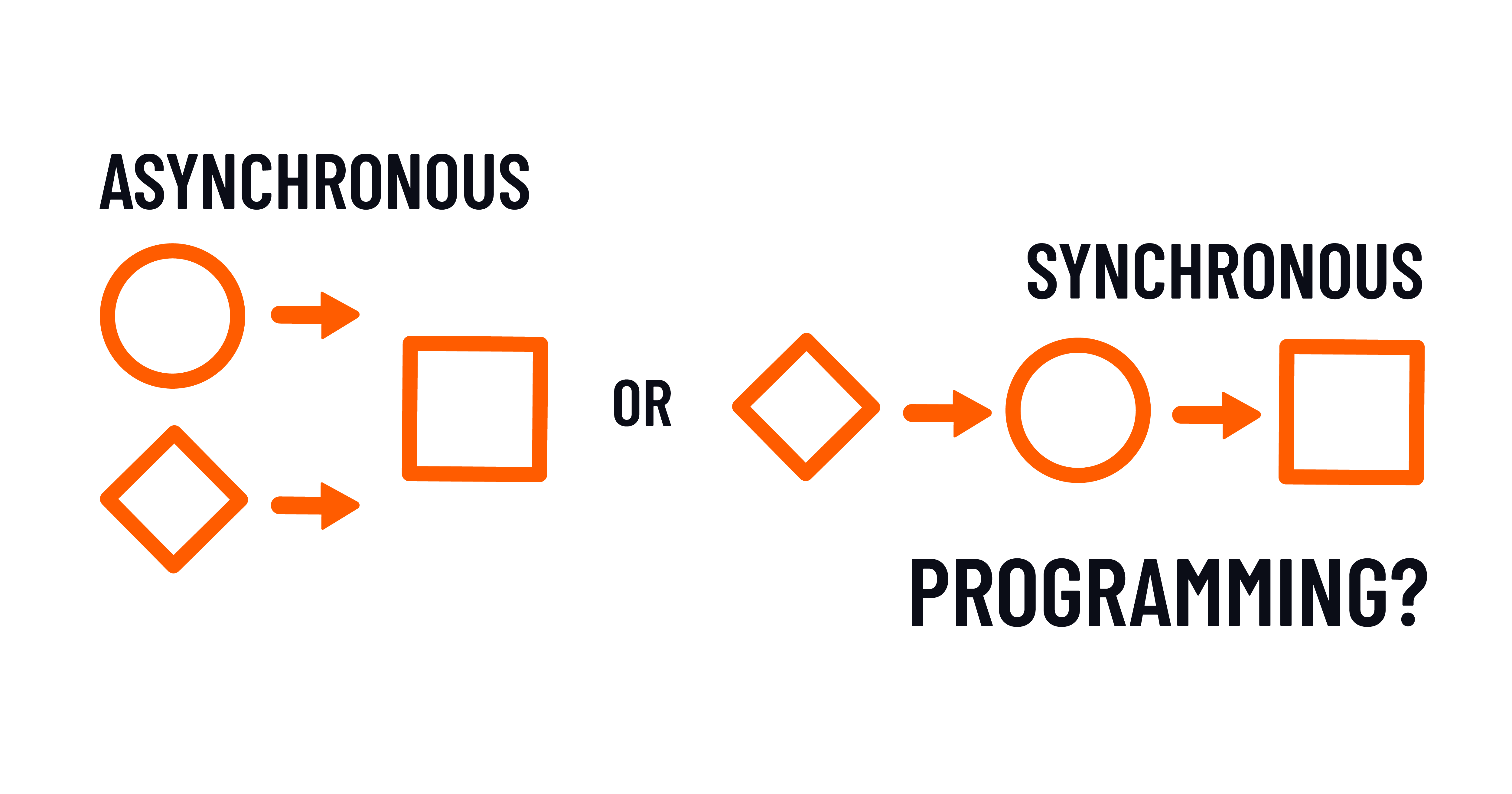
Introduction: let's dive into the world of asynchronous programming in JavaScript. Don't worry if it sounds a bit techy; we're going to break it down in simple terms. By the end, you'll be a pro at making your code run smoothly and efficiently.
Understanding Asynchronous JavaScript: Picture JavaScript as a normal human that can do one thing at a time. Asynchronous programming is like giving this human a superpower to do multiple things at once without waiting for one to finish before starting another. It's what makes our websites and apps responsive and quick.
Callbacks: The Building Blocks: Callbacks are like secret notes you pass to JavaScript, telling it what to do when it's done with a task. While they work, using too many can get messy and confusing.
A callback is a function passed as an argument to another function, which is then invoked after the completion of an asynchronous operation. It allows for the continuation of program execution while waiting for tasks like data retrieval, I/O operations, or event handling to complete.
javaScript is single-threaded and non-blocking. Asynchronous operations ensure that the program doesn't halt while waiting for time-consuming tasks to finish. Instead, the callback is executed once the asynchronous operation concludes.
Callback Hell: A Challenge to Readability: Callback Hell, or the Pyramid of Doom, is a common issue when dealing with nested callbacks. This occurs when multiple asynchronous operations are performed sequentially, leading to deeply nested callback functions. The resulting code becomes challenging to read, maintain, and debug.
function fetchdata(callback) {
setTimeout(() => {
console.log("Data fetched successfully");
callback();
}, 1000);
}
fetchdata(() => {
console.log("Callback executed");
});
Solution to Callback Hell: Promises :
Promises are a powerful abstraction in JavaScript that provide a cleaner and more structured way to handle asynchronous operations. Introduced with ECMAScript 6 (ES6), Promises offer a more readable alternative to traditional callback patterns. Let's delve into the technical intricacies of Promises and understand how they contribute to asynchronous control flow.
Promise Basics: A Promise is an object representing the eventual completion or failure of an asynchronous operation. It is in one of three states:
Pending: The initial state, neither fulfilled nor rejected.
Fulfilled: The operation completed successfully, resulting in a resolved value.
Rejected: The operation encountered an error, resulting in a reason for rejection.
Creating a Promise: The Promise
constructor takes a single argument, a function known as the executor. This executor function receives two functions, resolve
and reject
, as parameters. Developers use these functions to indicate the success or failure of the asynchronous operation.
Async/Await in JavaScript: Built on top of promises:
Async/Await, built on top of Promises, designed to make asynchronous code more readable, concise, and approachable. It offers a more synchronous style of writing asynchronous code, addressing some of the challenges posed by raw Promise chaining. Let's explore the Async/Await and understand how it enhances the developer experience compared to traditional Promises.
Async Functions: The Foundation of Async/Await: An async
function is a function that always returns a Promise. The keyword async
is placed before the function declaration. Inside an async function, the await
keyword is used to pause execution until the Promise is resolved or rejected.
async function fetchData() {
return new Promise((resolve) => {
setTimeout(() => {
console.log("Data fetched successfully");
resolve("Data");
}, 1000);
});
}
Awaiting Promises: A Synchronous Touch: The await
keyword is used inside an async function to pause its execution until the Promise settles. This allows for more sequential and readable code, resembling synchronous programming.
async function fetchDataAndProcess() {
try {
const result = await fetchData();
console.log(result);
const processedResult = await processResult(result);
console.log(processedResult);
} catch (error) {
console.error("Error:", error.message);
}
}
fetchDataAndProcess();
The sequential execution style offered by Async/Await simplifies the writing and understanding of asynchronous code, allowing developers to reason about it in a manner reminiscent of traditional synchronous programming. This is particularly beneficial when dealing with tasks that need to be performed in a step-by-step fashion.
In summary, callbacks paved the way for handling asynchronous tasks but brought challenges in code readability. Promises emerged to address these issues, introducing a more structured approach. Finally, Async/Await builds on Promises, offering a synchronous-style syntax that combines the simplicity of callbacks with the structured nature of Promises. This trio represents the evolution of asynchronous programming in JavaScript, providing developers with increasingly powerful tools to navigate the complexities of non-blocking code execution.
Let me know your thoughts in the comments below.
#Explore. Code. Evolve.
Happy coding! ๐
Subscribe to my newsletter
Read articles from Amod directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
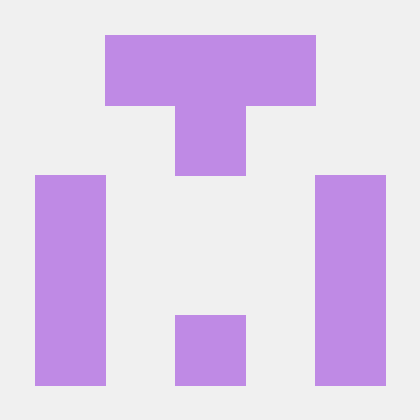