Django Decorators: An incomprehensive guide
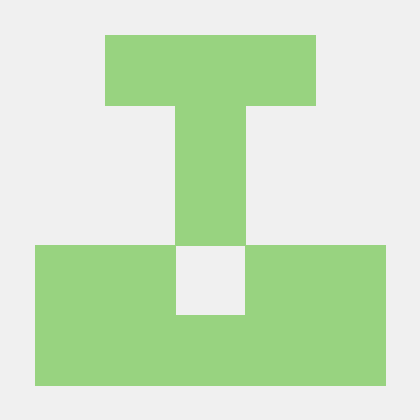
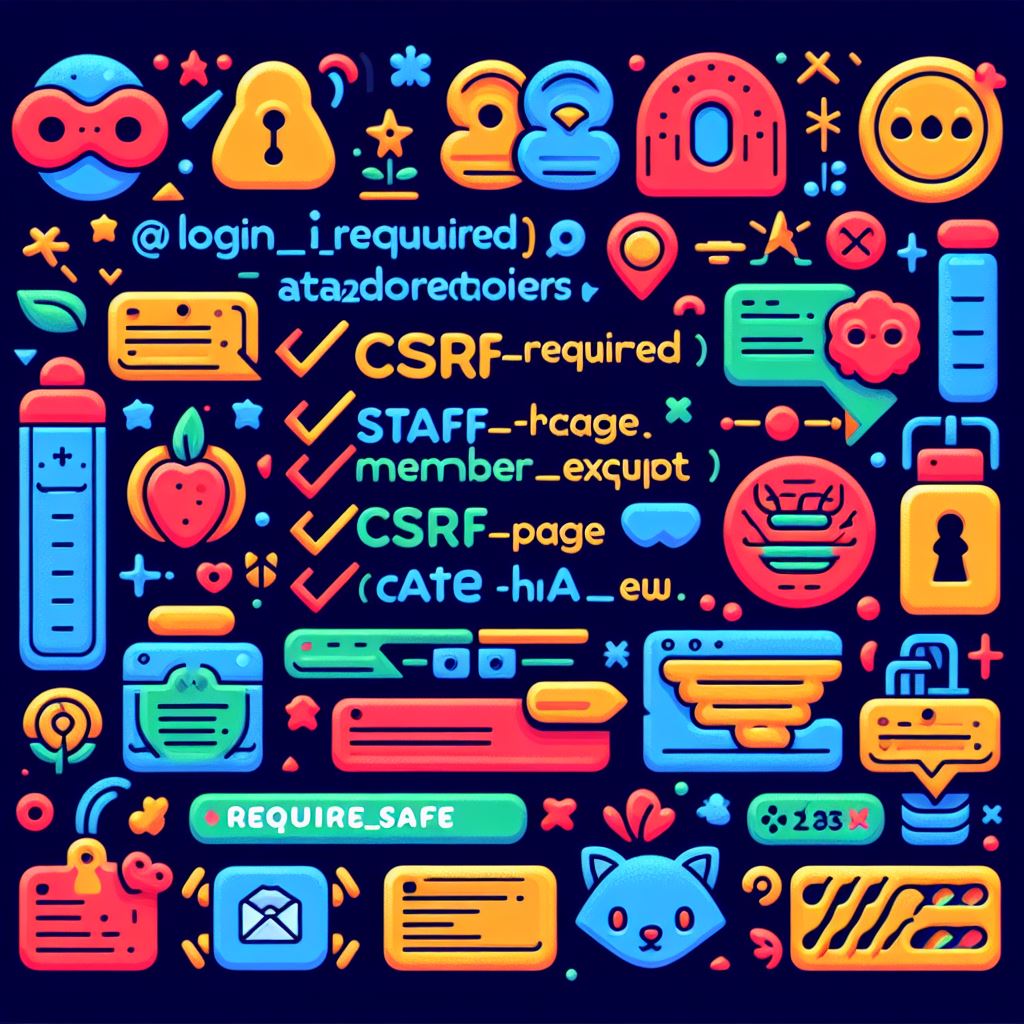
Mastering Django Decorators: A Deep Dive into Pythonic Web Development
Django, a web framework written in Python, is celebrated for its simplicity and flexibility. One of the powerful features that contribute to this reputation is decorators. Decorators in Django allow developers to modify or extend the behavior of functions or methods effortlessly. In this blog post, we'll embark on a journey to master Django decorators, exploring their basics, common use cases, and advanced patterns.
Learn Python & Django at django-tutorial.dev
Understanding Python Decorators
Before diving into Django-specific decorators, it's essential to understand Python decorators in general. In Python, a decorator is a function that takes another function and extends or modifies its behavior. Decorators are denoted with the @
symbol and are placed above the function they decorate.
# A simple decorator
def my_decorator(func):
def wrapper():
print("Something is happening before the function is called.")
func()
print("Something is happening after the function is called.")
return wrapper
@my_decorator
def say_hello():
print("Hello!")
say_hello()
"""
The output will be:
Something is happening before the function is called.
Hello!
Something is happening after the function is called.
"""
In this example, my_decorator
is a simple decorator that adds behavior before and after the say_hello
function is called.
Django View Decorators
In Django, decorators are widely used to enhance the functionality of views. Let's explore some common view decorators and their applications.
@login_required
The @login_required
decorator ensures that a view can only be accessed by authenticated users. If a user is not logged in, they are redirected to the login page.
from django.contrib.auth.decorators import login_required
@login_required
def my_secure_view(request):
# Your view logic here
return render(request, 'secure_page.html')
This decorator simplifies the process of securing views that require user authentication.
@permission_required
Similar to @login_required
, the @permission_required
decorator checks if a user has specific permissions to access a view.
from django.contrib.auth.decorators import permission_required
@permission_required('myapp.can_view_data')
def restricted_data_view(request):
# Your view logic here
return render(request, 'restricted_data.html')
This decorator is useful when you need to control access based on custom permissions defined in your Django app aka Roles of User. This will be handy when you are creating a platform where there are more than 1 types of users like admin, management, employee & Clients.
Custom Decorators
While Django provides useful built-in decorators, you'll often find the need to create your own custom decorators to encapsulate reusable functionality.
Creating an Underrated Decorator
I don't want any supporter of UML( political party) to access my site. I can create a custom decorator to Respond to UML supporters with a message while giving anyone else complete access to my web app. I am going to just respond the text.
"YOU ARE THE PROBLEM"
# app/decorators.py
from django.http import HttpResponse
def get_help(view_func):
def wrapper(request,*args,**kwargs):
if request.user.name in uml_supporter_list: #this is already documented list
return HttpResponse("YOU ARE THE PROBLEM")
else:
view_func(request,*args,**kwargs)
return wrapper
Now, I can use @get_help
to decline any UML supporters of my Web Service
from app.decorators import get_help
from django.shortcuts import render
@get_help
def index(request):
return render(request,'app/index.html')
Creating a Logging Decorator
Let's say you want to log information about the arguments and return value of a view. You can create a custom logging decorator for this purpose. Below is a code for File based logging using python's built in module called 'logging'. For Django decorators the wrapper needs to take request, *args and **kwargs as parameter in most cases.
# app/decorators.py
import logging
# Configure the logging settings (add this to your settings.py or another configuration file)
logging.basicConfig(filename='views.log', level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s')
def log_view(func):
def wrapper(request, *args, **kwargs):
# Log information before calling the view
log_message = f"{request.user} is calling {func.__name__} with arguments: {args}, {kwargs}"
logging.info(log_message)
result = func(request, *args, **kwargs)
# Log information after calling the view
log_message = f"{func.__name__} returned: {result}"
logging.info(log_message)
return result
return wrapper
Now, you can use @log_view
to add logging to any view.
from app.decorators import log_view
@log_view
def my_logged_view(request):
# Your view logic here
return render(request, 'logged_page.html')
Handling Function Arguments in Decorators
Sometimes, you might need decorators that accept arguments. This can be achieved by adding an extra layer of nested functions.
# app/decorators.py
def custom_decorator(arg1, arg2):
def decorator(func):
def wrapper(*args, **kwargs):
# Custom logic using arg1 and arg2
result = func(*args, **kwargs)
# Additional logic if needed
return result
return wrapper
return decorator
Now, when applying the decorator, you provide arguments like so:
from app.decorators import custom_decorator
@custom_decorator(arg1='value1', arg2='value2')
def my_decorated_function():
# Your function logic here
pass
This pattern allows decorators to be flexible and adaptable to various scenarios.
Advanced Decorator Patterns
As you delve deeper into Django development, you may encounter advanced decorator patterns that leverage class-based views or mixins. For example, the @method_decorator
allows applying decorators to specific HTTP methods.
from django.utils.decorators import method_decorator
from django.views import View
@method_decorator(login_required, name='dispatch')
class MySecureView(View):
# Your view logic here
This ensures that the login_required
decorator is only applied to the dispatch
method of the class-based view.
Conclusion
Mastering Django decorators opens the door to elegant and modular code. Whether you're securing views, logging information, or creating custom functionality, decorators provide a clean and Pythonic way to enhance your web development experience. As you continue your Django journey, experimenting with and understanding decorators will undoubtedly contribute to the efficiency and maintainability of your codebase. Suffer decorating!
This is your 6/900 Steps in becoming a Django Master jr.
Subscribe to my newsletter
Read articles from Nischal lamichhane directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
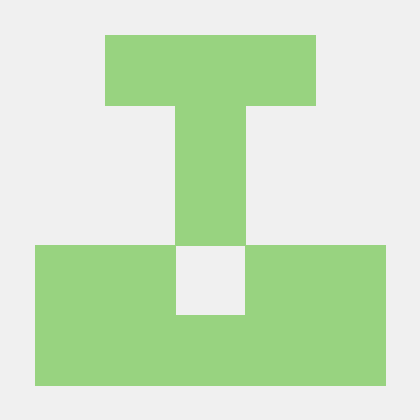
Nischal lamichhane
Nischal lamichhane
There are always 2 ways to do something in Django. They are Django Master's WAY WRONG WAY