Introduction to Python
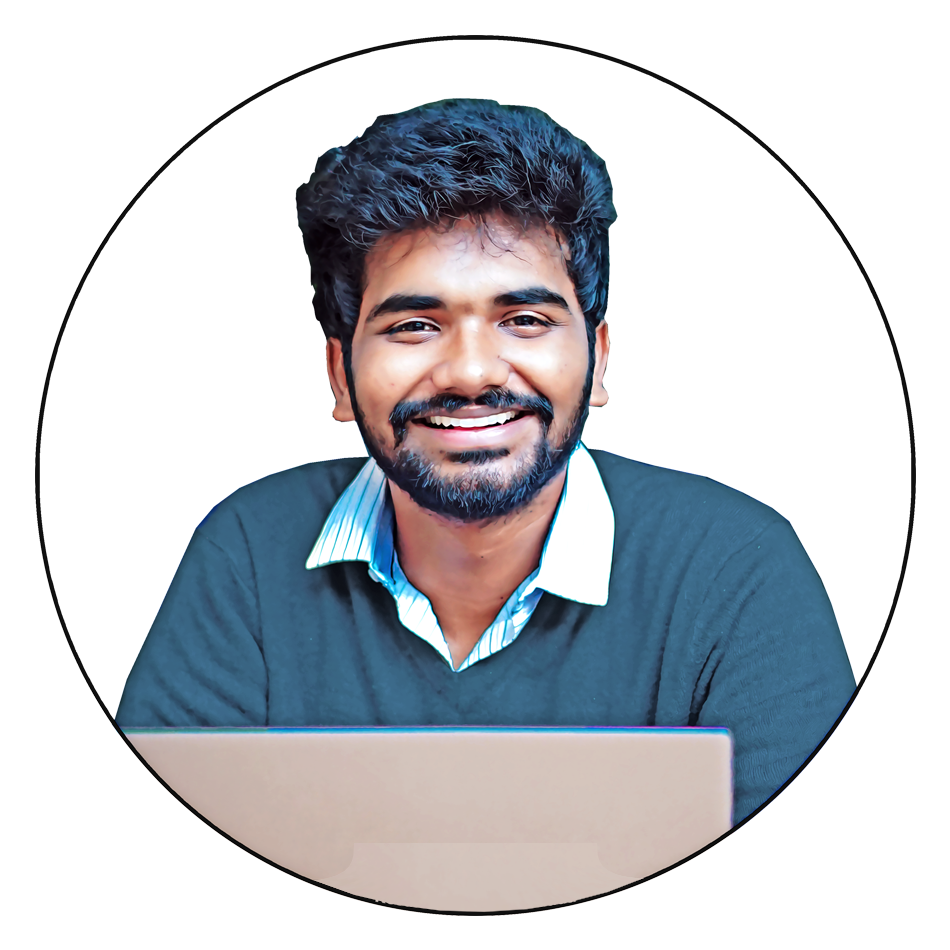
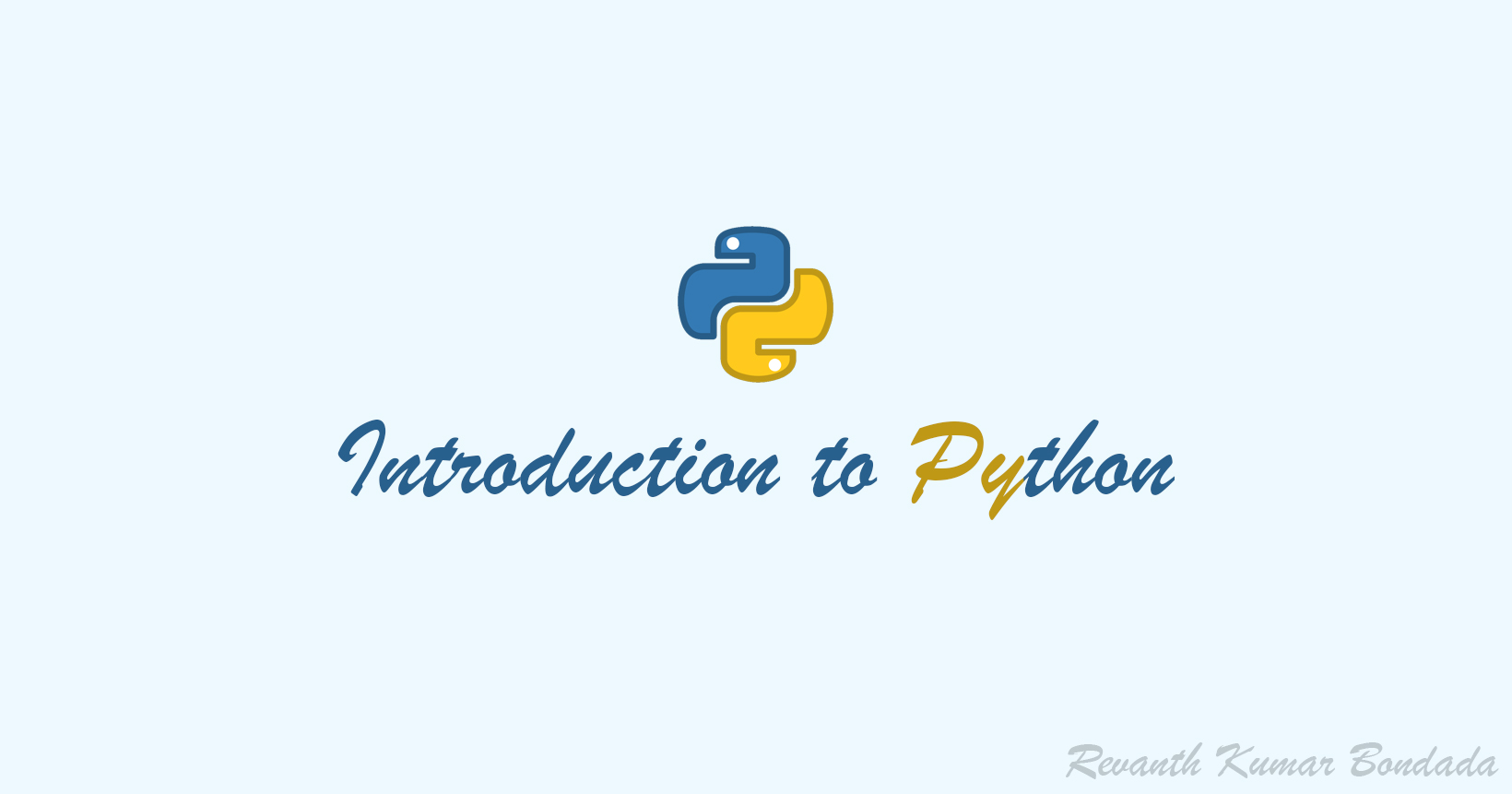
Download Python: Visit the official Python website and download the latest version of Python for your operating system (Windows, macOS, or Linux).
Installation:
Windows: Run the installer, select "Add Python to PATH", and follow the prompts.
macOS/Linux: Often comes pre-installed. If not, use the package manager (e.g., Homebrew for macOS, apt for Ubuntu) to install Python.
Verify Installation: Open a command prompt or terminal and type python --version
. You should see the Python version number.
Set Up a Text Editor or IDE: You can start with a simple text editor like Notepad or advanced IDEs like PyCharm, VS Code, or Jupyter Notebooks for writing Python code.
Variables:
Used to store data.
Python is dynamically typed, so you don't need to declare a variable's data type.
Example: x = 5, name = "RevanthKumarBondada"
Arithmetic Operators
Arithmetic operators are used to perform mathematical calculations in Python.
+ (Addition): Adds two values.
Example: 5 + 3 equals 8
- (Subtraction): Subtracts the right value from the left.
Example: 5 - 3 equals 2.
* (Multiplication): Multiplies two values.
Example: 5 * 3 equals 15.
/ (Division): Divides the left value by the right value and returns a floating-point result.
Example: 5 / 2 equals 2.5.
% (Modulus): Divides the left value by the right value and returns the remainder.
Example: 5 % 3 equals 2.
** (Exponentiation): Raises the left value to the power of the right value.
Example: 5 ** 3 equals 125 (which is 5^3).
// (Floor Division): Divides the left value by the right value and returns the largest integer less than or equal to the result.
Example: 5 // 2 equals 2.
Comparison Operators
Comparison operators are used to compare two values.
(==) (Equal to): Checks if the values on either side are equal.
Example: 5 == 5 is True
.!= (Not Equal to): Checks if the values are not equal.
Example: 5 != 3 is True.
> (Greater than): Checks if the left value is greater than the right value.
Example: 5 > 3 is True.
< (Less than): Checks if the left value is less than the right value.
Example: 3 < 5 is True.
>= (Greater than or Equal to): Checks if the left value is greater than or equal to the right value.
Example: 5 >= 5 is True.
<= (Less than or Equal to): Checks if the left value is less than or equal to the right value.
Example: 3 <= 5 is True.
Logical Operators
Logical operators are used to combine conditional statements.
and: Returns True if both statements are true.
Example: 5 > 3 and 5 < 10 is True because both conditions are true.
or: Returns True if one of the statements is true.
Example: 5 > 3 or 5 > 10 is True because the first condition is true.
not: Reverses the result, returns False if the result is true.
Example: not(5 > 3) is False because 5 > 3 is true, but not reverses it.
Data Types:
Integers: Whole numbers, e.g., 5, 100.
Floats: Decimal numbers, e.g., 3.14, 2.0.
Strings: Text, e.g., "Hello", "Python".
Booleans: True or False.
NoneType: Represents the absence of a value, None.
Control Structures: If, Else, and Loops (Used for decision-making)
Syntax:
if condition:
# code to execute if condition is true
elif another_condition:
# code to execute if another condition is true
else:
# code to execute if all conditions are false
Example:
if x > 0:
print("x is positive")
elif x == 0:
print("x is zero")
else:
print("x is negative")
Loops:
For Loop: Iterates over a sequence (like a list, tuple(like lists but Immutable), dictionary, set, or string).
Syntax:
for element in sequence:
# code to execute for each element
Example:
for i in range(5):
print(i)
While Loop: Repeats a block of code as long as a condition is true.
Syntax:
while condition:
# code to execute while the condition is true
Example:
count = 0
while count < 5:
print(count)
count += 1
Subscribe to my newsletter
Read articles from Revanth kumar Bondada directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
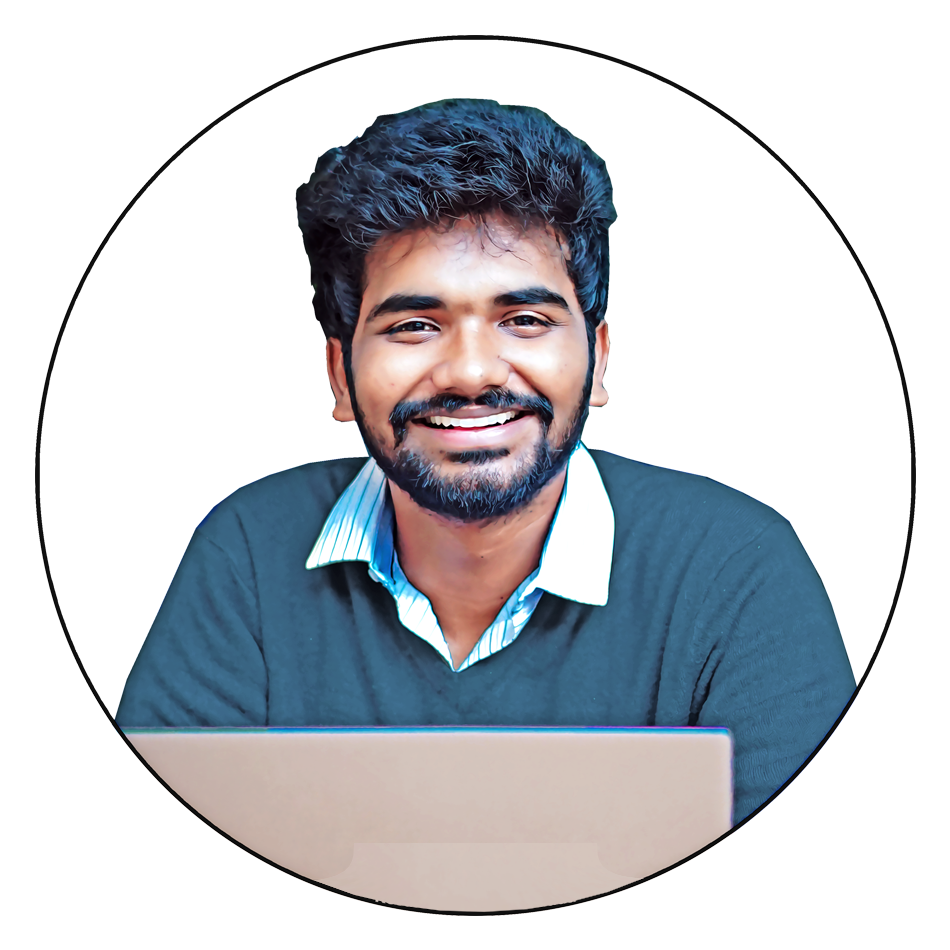
Revanth kumar Bondada
Revanth kumar Bondada
Graduate Student at SJSU with 3 years of experience in AI and Machine Learning application design, specializing in Cloud Data Engineering, Data Warehousing, ETL processes, Business Intelligence, and Reporting.