What is asynchronous JavaScript? Event loop with an example and diagram in depth
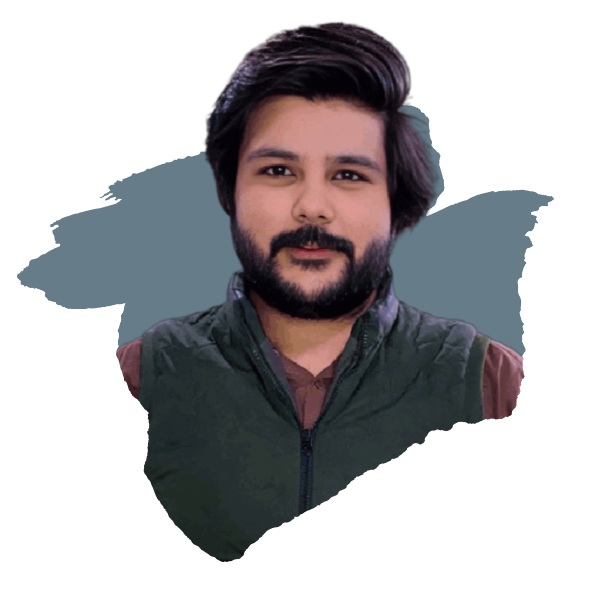
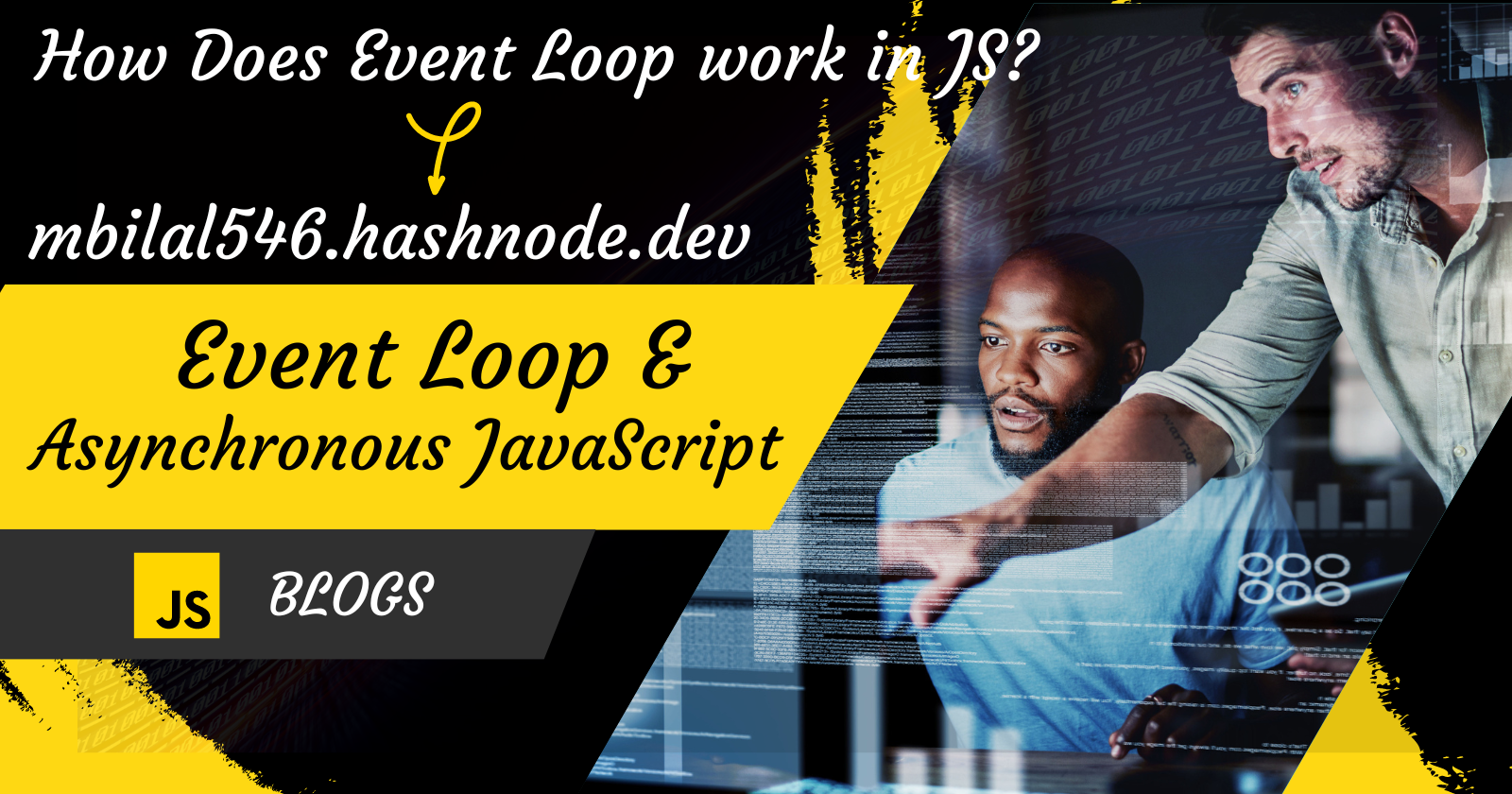
What is synchronous JavaScript?
Synchronous JavaScript refers to the execution of code in a blocking manner, where one operation must be completed before the next one starts. In a synchronous environment, each line of code is executed one after the other, and the program waits for each operation to finish before moving on to the next.
For example:
function synchronousExample() {
console.log("Start");
// Synchronous operation
const result1 = operation1();
console.log(result1);
// Synchronous operation
const result2 = operation2(result1);
console.log(result2);
console.log("End");
}
function operation1() {
console.log("Operation 1 started");
// Simulating a time-consuming task
for (let i = 0; i < 1000000000; i++) {
// Some heavy computation
}
console.log("Operation 1 completed");
return "Result from operation 1";
}
function operation2(input) {
console.log("Operation 2 started with input:", input);
// Simulating another time-consuming task
for (let i = 0; i < 500000000; i++) {
// Some heavy computation
}
console.log("Operation 2 completed");
return "Result from operation 2";
}
synchronousExample();
Output:
Start
Operation 1 started
Operation 1 completed
Result from operation 1
Operation 2 started with input: Result from operation 1
Operation 2 completed
Result from operation 2
End
It's important to note that synchronous code can lead to performance issues, especially in web development, where it can result in a slow and unresponsive user interface. To tackle this, asynchronous programming techniques such as allbackspromises
andasync/await
, are often used in JavaScript to allow non-blocking execution.
What is asynchronous JavaScript?
Asynchronous JavaScript refers to a programming pattern or paradigm that allows code to execute non-blocking operations. In synchronous programming, code is executed sequentially, and each operation must be completed before the next one starts. In contrast, asynchronous programming enables certain tasks to be initiated and executed independently of the main program flow.
JavaScript, being a single-threaded language, sequentially executes code by default. However, it also provides mechanisms for handling asynchronous operations. The primary tool for achieving asynchrony in JavaScript is the event loop.
How does the event loop work?
The event loop is a fundamental concept in JavaScript, and it plays a crucial role in handling asynchronous operations. JavaScript is a single-threaded language, meaning it has only one execution thread. However, it often needs to deal with asynchronous tasks, such as fetching data from a server or handling user input.
Here's a simplified explanation of how the event loop works in JavaScript:
Execution Context (Call Stack):
When your JavaScript code is executed, it gets pushed onto the call stack.
The call stack is a data structure that keeps track of the currently executing function or statement.
We studied the call stack in our previous blog in the execution context.
Web APIs:
- Web APIs (application programming interfaces) in JavaScript provide a way for your code to interact with external functionalities provided by web browsers. These APIs enable developers to access various features of a web browser or other web-related services. Here are some common types of web APIs in JavaScript, like DOM APIs, Fetch APIs, etc.
Task Queue:
JavaScript runtime environments, like browsers or Node.js, have a task queue (also known as the message queue).
When asynchronous operations are complete or events occur (e.g., user clicks, complete HTTP requests), corresponding tasks are placed in the task queue.
High Priority Queue (Promise Queue)
JavaScript runtime environments, like browsers or Node.js, also have a high-priority queue (for promises).
When any
Promise
are created, corresponding tasks are placed in the priority queue.
Event Loop:
The event loop constantly checks the call stack and the task queue.
If the call stack is empty, it takes the first task from the task queue and pushes it onto the call stack, starting the execution of that task.
Non-blocking Execution:
Asynchronous operations (e.g., setTimeout, AJAX requests, Promises) allow JavaScript to execute code without waiting for the operation to finish.
Instead of blocking the entire program, these operations use callbacks
Promises
to handle the result when it becomes available.
Here we will understand with the example of setTimeout().
setTimeout()
setTimeout()
is a function in JavaScript that is used to schedule the execution of a function after a specified delay. It is commonly used to introduce a delay in the execution of a particular piece of code.
For Example:
console.log("Start");
setTimeout(function() {
console.log("This will print after 2 seconds");
}, 2000); // This time is in milliseconds
console.log("End");
Output:
Start
End
This will print after 2 seconds
Let's understand it through an event loop.
When setTimeout()
created in the JavaScript Engine global execution context and function execution context, everything is created in the call stack that we discussed in the previous blog.
setTimeout()
is an asynchronous action, so the JavaScript engine considered it a web API call. Call Sack sends a call to the browser where the callback is registered, and that register sends this task into the task queue. When its time is up, this callback is added to the call stack for execution. This is how it works.
New APIs
There are some relatively newly added APIs in JavaScript, likeFetch()
, that work on the concept of promises, which say to tell about the result in the future, whether it is completed or not. For that, the task queue is expanded, or, you can say, a new task queue is added, which is known as a high-priority queue. Everything in this happens like a task queue, but the difference is that it has a high priority.
You can understand this event loop flow with the help of a diagram.
We will discuss callbacks, promises, and async/await in the next blog.
Subscribe to my newsletter
Read articles from Muhammad Bilal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
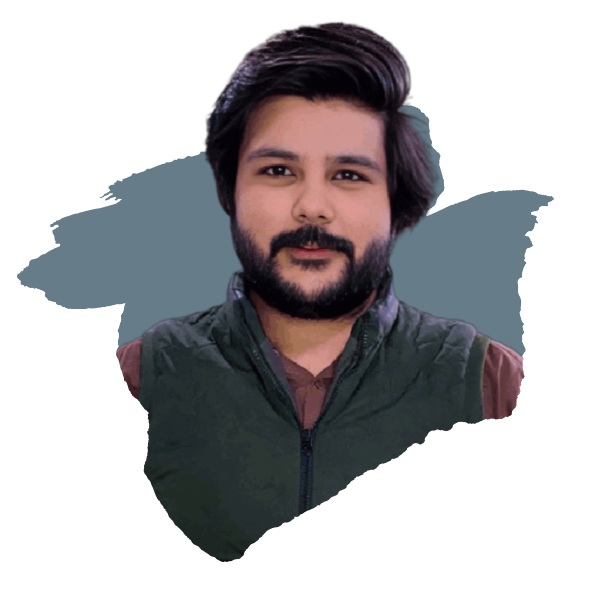
Muhammad Bilal
Muhammad Bilal
With a passion for creating seamless and visually appealing web experiences, I am Muhammad Bilal, a dedicated front-end developer committed to transforming innovative ideas into user-friendly digital solutions. With a solid foundation in HTML, CSS, and JavaScript, I specialize in crafting responsive and intuitive websites that captivate users and elevate brands. ๐ Professional Experience: In my two years of experience in front-end development, I have had the privilege of collaborating with diverse teams and clients, translating their visions into engaging online platforms. ๐ Skills: Technologies: HTML5, CSS3, JavaScript, WordPress Responsive Design: Bootstrap, Tailwind CSS, Flexbox, and CSS Grid Version Control: Git, GitHub ๐ฅ Projects: I have successfully contributed to projects where my focus on performance optimization and attention to detail played a crucial role in delivering high-quality user interfaces. โจ Continuous Learning: In the fast-paced world of technology, I am committed to staying ahead of the curve. Regularly exploring new tools and methodologies, I am dedicated to enhancing my skills and embracing emerging trends to provide the best possible user experience. ๐ Education: I hold a BSc degree in Software Engineering from Virtual University, where I gained both technical skills and knowledge. ๐ฅ Let's Connect: I am always open to new opportunities, collaborations, and discussions about the exciting possibilities in front-end development. Feel free to reach out via LinkedIn, Instagram, Facebook, Twitter, or professional email.