Dart Concurrency : Isolates

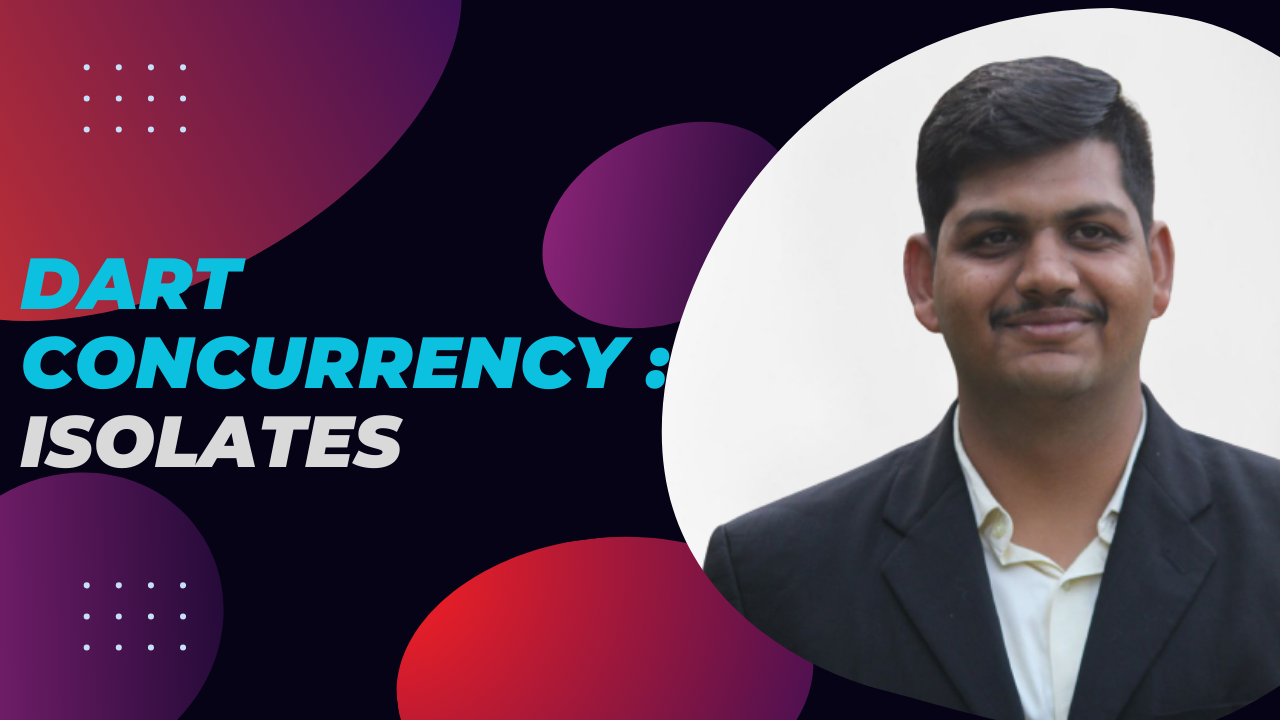
Concurrency is a crucial aspect of modern software development, enabling applications to perform multiple tasks simultaneously, improving performance, and enhancing user experiences. Dart, a versatile programming language developed by Google, provides a powerful concurrency model called "Isolates." In this blog post, we'll explore the concept of isolates in Dart and how they can be harnessed to write highly concurrent and responsive applications.
What are Isolates?
Isolates are independent, lightweight threads of execution in Dart that run concurrently. Unlike traditional multithreading, isolates do not share memory, reducing the risk of data corruption and simplifying concurrent programming. Each isolate has its own memory heap, allowing it to execute code independently of other isolates.
Creating Isolates: In Dart, creating isolates is a straightforward process. The Isolate.spawn function is used to start a new isolate and execute a given function within it. This function can be a top-level function, a static method, or a closure. Here's a simple example:
void isolateFunction(message) {
print('Isolate Received: $message');
}
void main() {
Isolate.spawn(isolateFunction, 'Hello from the main isolate!');
}
Communication between Isolates: Since isolates do not share memory, communication between them is achieved through message passing. Dart provides a mechanism for sending and receiving messages between isolates using the SendPort and ReceivePort classes. The Isolate.spawn function returns a SendPort that can be used to send messages to the spawned isolate.
void isolateFunction(SendPort sendPort) {
sendPort.send('Hello from the spawned isolate!');
}
void main() {
ReceivePort receivePort = ReceivePort();
Isolate.spawn(isolateFunction, receivePort.sendPort);
receivePort.listen((message) {
print('Main Isolate Received: $message');
});
}
Shared and Immutable Data: While isolates do not share memory, there are scenarios where data needs to be shared between them. Dart provides mechanisms like the Isolate.spawnUri function, which allows isolates to share code through a shared URI.
// SharedCode.dart
void sharedFunction(message) {
print('Shared Isolate Received: $message');
}
// main.dart
void main() {
Isolate.spawnUri(Uri.file('path/to/SharedCode.dart'), [], 'Hello from shared isolate!');
}
Error Handling and Termination: Isolates run independently, and an unhandled error in one isolate won't affect others. Dart provides ways to catch errors and exceptions in isolates to prevent crashes. Additionally, isolates can be terminated explicitly using the Isolate.kill method.
Conclusion: Dart isolates offer a powerful and efficient way to introduce concurrency into your applications. By leveraging isolates, developers can build responsive and high-performance Dart applications that can handle multiple tasks concurrently. As you delve deeper into Dart concurrency, you'll find isolates to be a valuable tool in your programming arsenal.
Subscribe to my newsletter
Read articles from Vinit Mepani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vinit Mepani
Vinit Mepani
"Hello World, I'm Vinit Mepani, a coding virtuoso driven by passion, fueled by curiosity, and always poised to conquer challenges. Picture me as a digital explorer, navigating through the vast realms of code, forever in pursuit of innovation. In the enchanting kingdom of algorithms and syntax, I wield my keyboard as a magical wand, casting spells of logic and crafting solutions to digital enigmas. With each line of code, I embark on an odyssey of learning, embracing the ever-evolving landscape of technology. Eager to decode the secrets of the programming universe, I see challenges not as obstacles but as thrilling quests, opportunities to push boundaries and uncover new dimensions in the realm of possibilities. In this symphony of zeros and ones, I am Vinit Mepani, a coder by passion, an adventurer in the digital wilderness, and a seeker of knowledge in the enchanting world of code. Join me on this quest, and let's create digital wonders together!"