Dart Isolate : Unresponsive Applications

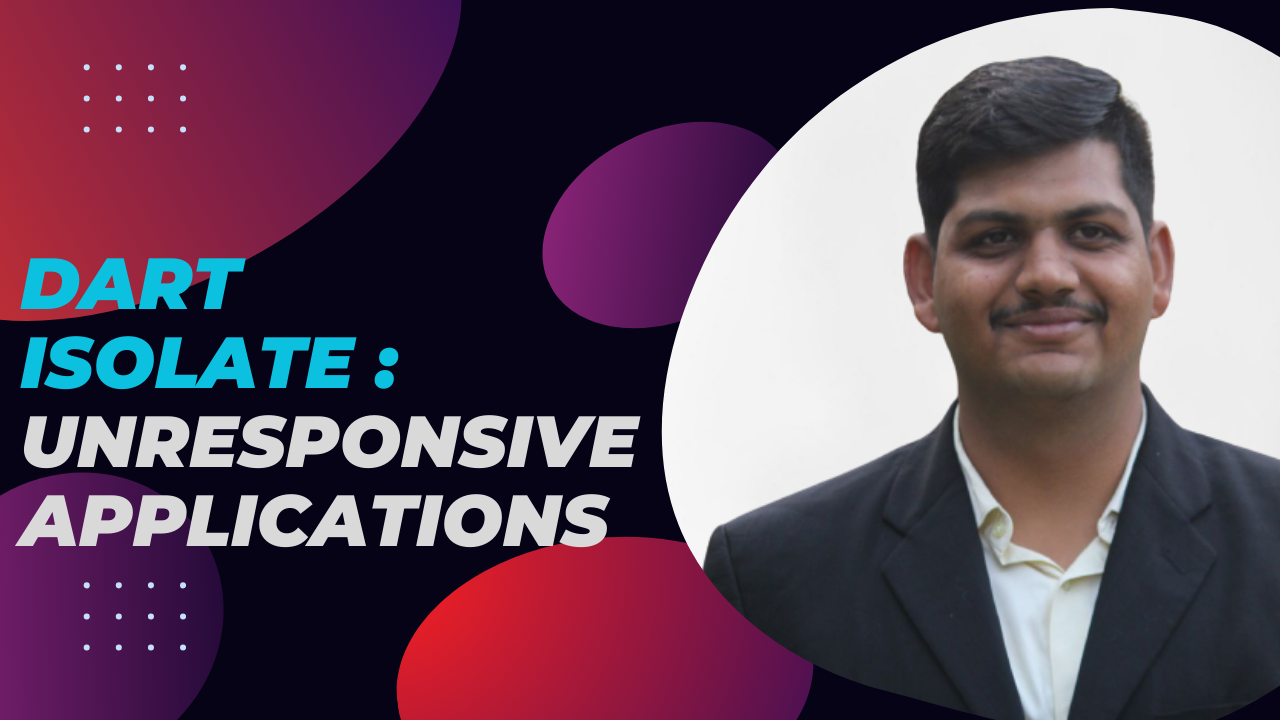
Introduction
Dart, a versatile and modern programming language, introduces the concept of isolates for concurrent programming. Isolates play a pivotal role in managing concurrency, but certain challenges may arise, such as unresponsive applications and the need for effective communication between isolates. In this blog post, we'll explore these challenges and present solutions for addressing unresponsiveness, as well as implementing one-way and two-way communication between Dart isolates.
Dart Isolate Unresponsiveness
One of the primary concerns in concurrent programming is the potential for unresponsive applications due to long-running tasks in isolates. Dart mitigates this issue by allowing isolates to yield control back to the event loop periodically. Developers can use the Future.microtask or Future to introduce breaks in execution, ensuring responsiveness.
void longRunningTask() {
for (int i = 0; i < 1000000000; i++) {
// Perform computation
if (i % 1000000 == 0) {
// Yield control to the event loop
Future.microtask(() {});
}
}
}
void main() {
longRunningTask();
print('Task completed!');
}
By employing Future.microtask, Dart can process other tasks in the event loop, preventing the application from becoming unresponsive during lengthy computations.
One-Way Isolate Communication
Isolates communicate through message passing, and Dart facilitates one-way communication through SendPort and ReceivePort. The parent isolate can send messages to the spawned isolate, and vice versa.
void isolateFunction(SendPort sendPort) {
sendPort.send('Hello from the spawned isolate!');
}
void main() {
ReceivePort receivePort = ReceivePort();
// Spawn isolate and pass the send port
Isolate.spawn(isolateFunction, receivePort.sendPort);
// Listen for messages from the spawned isolate
receivePort.listen((message) {
print('Main Isolate Received: $message');
});
}
Here, the Isolate.spawn function sends the SendPort to the spawned isolate, enabling communication in one direction. This one-way communication pattern is useful for scenarios where data flows predominantly from one isolate to another.
Two-Way Isolate Communication
Achieving two-way communication between isolates involves setting up two sets of SendPort and ReceivePort instances, allowing messages to flow bidirectionally.
void isolateFunction(SendPort mainToIsolate, ReceivePort isolateToMain) {
// Listen for messages from the main isolate
isolateToMain.listen((message) {
print('Spawned Isolate Received: $message');
});
// Send a message to the main isolate
mainToIsolate.send('Hello from the spawned isolate!');
}
void main() {
// Set up ports for communication in both directions
ReceivePort receivePortFromIsolate = ReceivePort();
SendPort sendPortToIsolate = receivePortFromIsolate.sendPort;
ReceivePort receivePortToIsolate = ReceivePort();
SendPort sendPortFromIsolate = receivePortToIsolate.sendPort;
// Spawn isolate and pass the ports for bidirectional communication
Isolate.spawn(isolateFunction, [sendPortToIsolate, receivePortFromIsolate]);
// Listen for messages from the spawned isolate
receivePortToIsolate.listen((message) {
print('Main Isolate Received: $message');
});
// Send a message to the spawned isolate
sendPortFromIsolate.send('Hello from the main isolate!');
}
This approach establishes a two-way communication channel, enabling both isolates to send and receive messages. It's suitable for scenarios where inter-isolate collaboration is essential.
Subscribe to my newsletter
Read articles from Vinit Mepani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vinit Mepani
Vinit Mepani
"Hello World, I'm Vinit Mepani, a coding virtuoso driven by passion, fueled by curiosity, and always poised to conquer challenges. Picture me as a digital explorer, navigating through the vast realms of code, forever in pursuit of innovation. In the enchanting kingdom of algorithms and syntax, I wield my keyboard as a magical wand, casting spells of logic and crafting solutions to digital enigmas. With each line of code, I embark on an odyssey of learning, embracing the ever-evolving landscape of technology. Eager to decode the secrets of the programming universe, I see challenges not as obstacles but as thrilling quests, opportunities to push boundaries and uncover new dimensions in the realm of possibilities. In this symphony of zeros and ones, I am Vinit Mepani, a coder by passion, an adventurer in the digital wilderness, and a seeker of knowledge in the enchanting world of code. Join me on this quest, and let's create digital wonders together!"