Reconciliation Algorithm in React
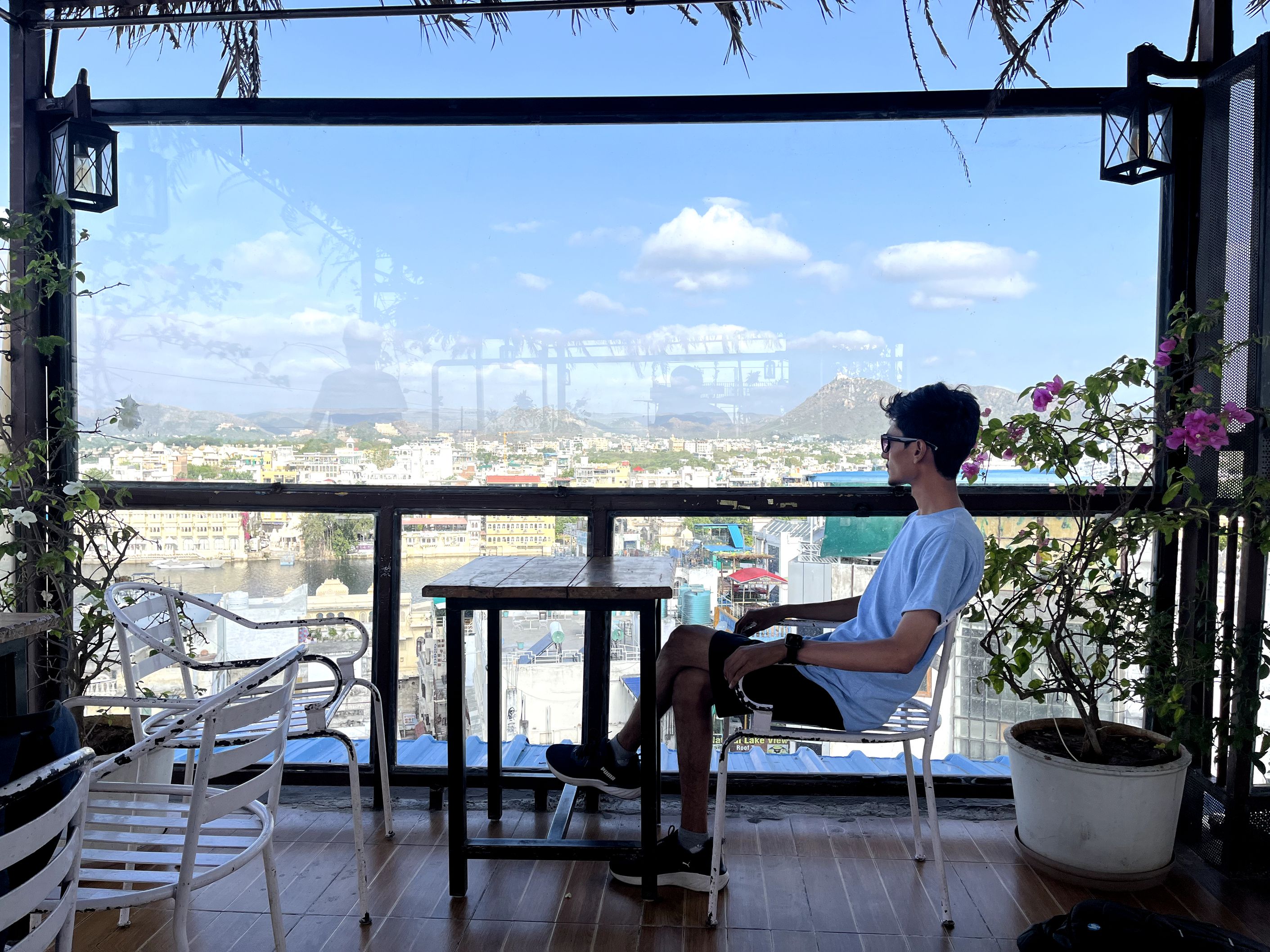
Expert-Level Explanation
The reconciliation algorithm in React is the process through which React updates the DOM. When a component’s state changes, React needs to determine whether an actual DOM update is necessary. The algorithm compares the rendered element (virtual DOM) with the previous one and calculates the minimal set of changes needed to update the DOM. This process is efficient and minimises DOM manipulation, leading to better performance.
Creative Explanation
Imagine you have a blueprint (virtual DOM) of a building (UI), and you make some changes to the design. Instead of tearing down the building and rebuilding it, a smart architect (reconciliation algorithm) compares the new blueprint with the old one and only makes the necessary changes to the existing structure. This is much faster and more efficient than reconstructing everything from scratch.
Practical Explanation with Code
The reconciliation algorithm works behind the scenes, so it doesn't directly affect how you write React code.
function MyComponent({ items }) {
return (
<ul>
{items.map(item => <li key={item.id}>{item.name}</li>)}
</ul>
);
}
In this example, when items
changes, React uses the reconciliation algorithm to update only the changed items in the DOM instead of re-rendering the entire list.
Real-World Example
In a chat application, when a new message arrives, the reconciliation algorithm allows React to update the chat UI efficiently. It adds the new message to the chat window without re-rendering the entire list of messages, resulting in a smooth and responsive user experience.
Subscribe to my newsletter
Read articles from Akash Thoriya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
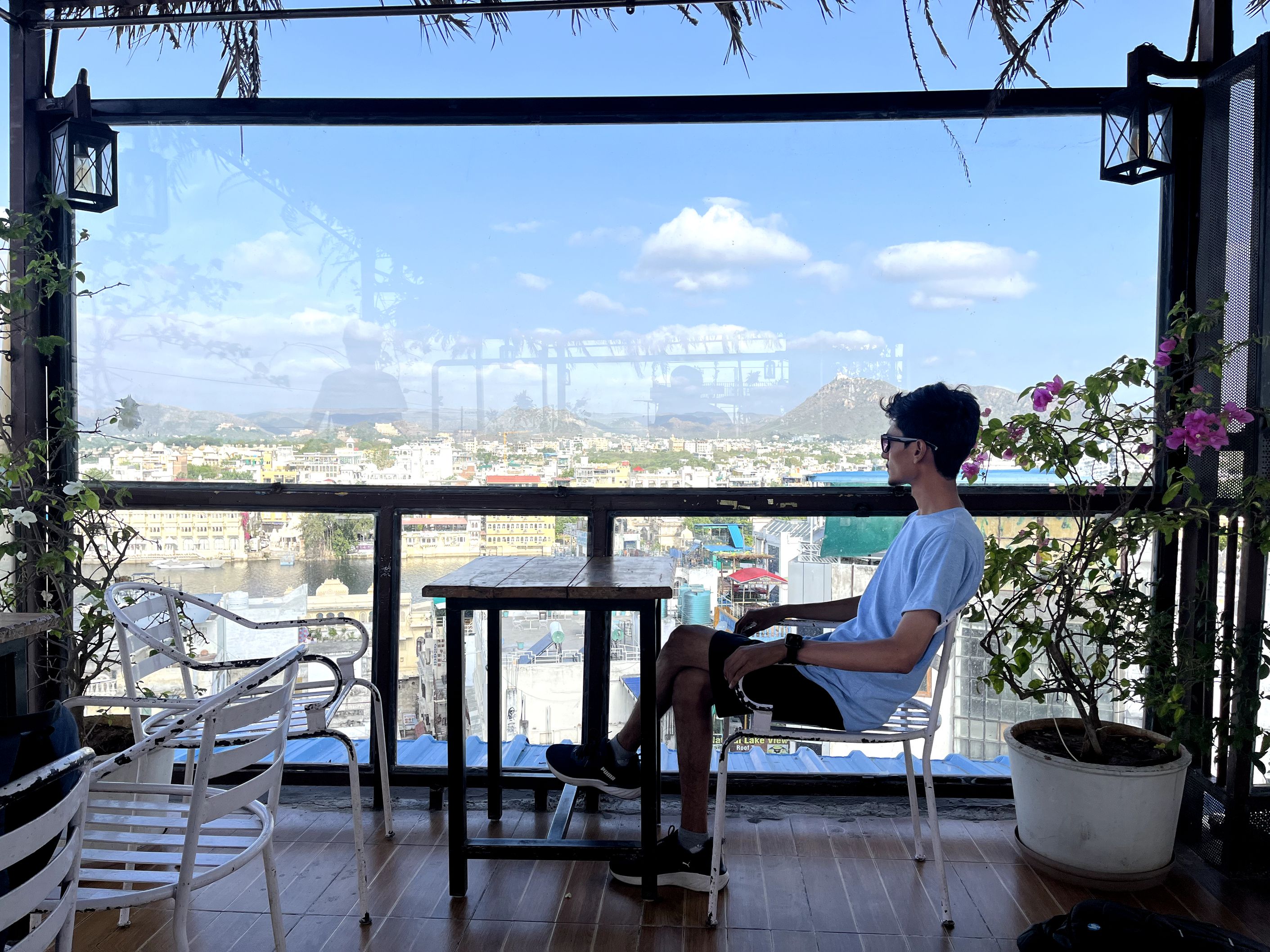
Akash Thoriya
Akash Thoriya
As a senior full-stack developer, I am passionate about creating efficient and scalable web applications that enhance the user experience. My expertise in React, Redux, NodeJS, and Laravel has enabled me to lead cross-functional teams and deliver projects that consistently exceed client expectations.