Controlled and Uncontrolled Components in React
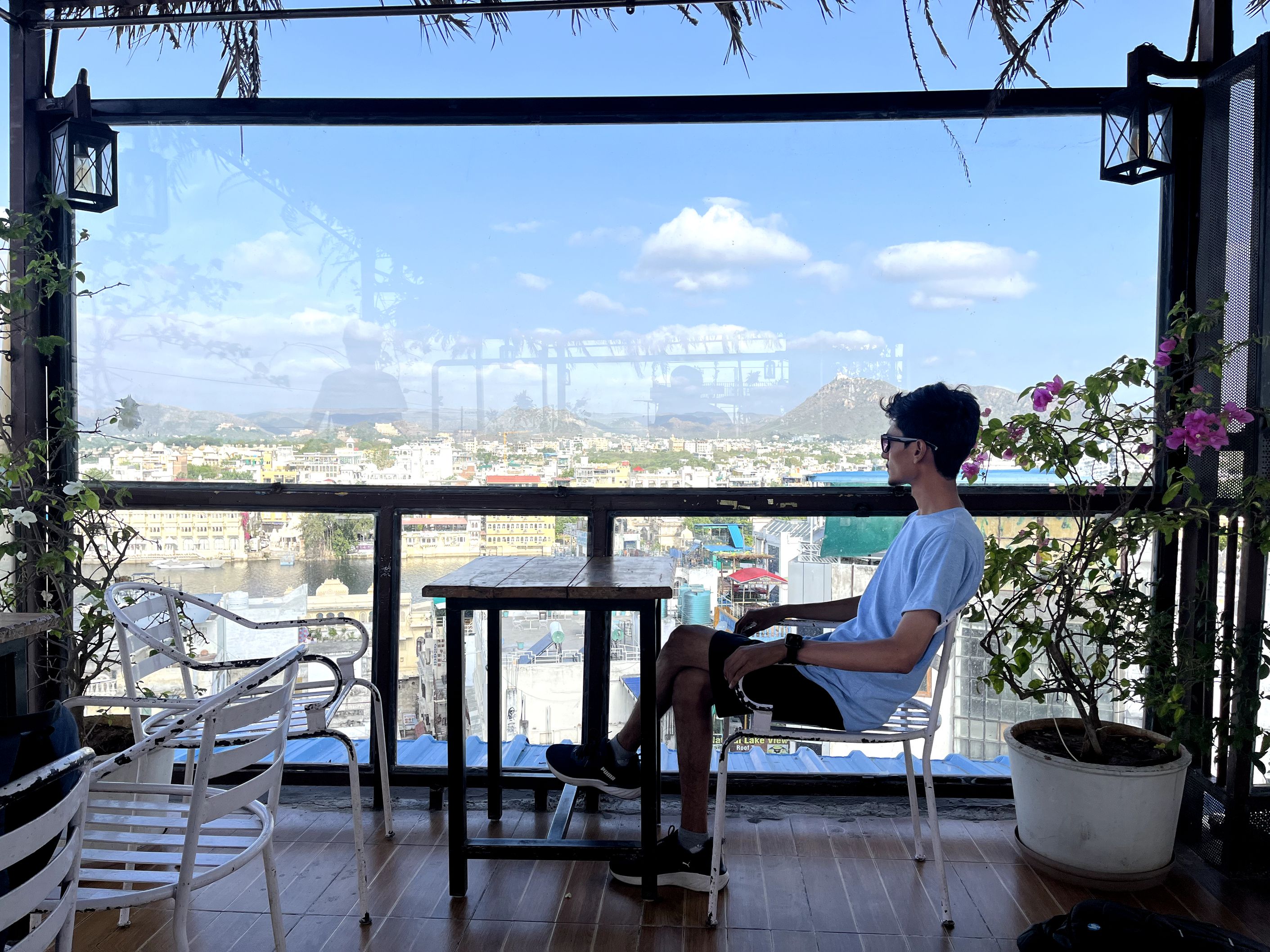
Expert-Level Explanation
Controlled components in React are components that derive their state from React state. The state of these components is controlled by React, typically using useState
or useReducer
. Uncontrolled components, on the other hand, maintain their own state internally, and React does not directly control their state. They are similar to traditional HTML form elements and can be accessed using references.
Creative Explanation
Controlled components are like remote-controlled toy cars. You have complete control over them via a remote (react state). Uncontrolled components are like wind-up toy cars. Once you wind them up and let them go, they operate on their own without your direct control, but you can still interact with them when needed (using references).
Practical Explanation with Code
Controlled Component Example:
import React, { useState } from 'react';
function ControlledComponent() {
const [value, setValue] = useState('');
return (
<input
type="text"
value={value}
onChange={(e) => setValue(e.target.value)}
/>
);
}
Uncontrolled Component Example:
import React, { useRef } from 'react';
function UncontrolledComponent() {
const inputRef = useRef();
const handleSubmit = () => {
alert(`Input Value: ${inputRef.current.value}`);
};
return (
<>
<input type="text" ref={inputRef} />
<button onClick={handleSubmit}>Submit</button>
</>
);
}
Real-World Example
In a login form, a controlled component approach would be used for the username and password inputs, where their values are managed by React state, enabling features like instant validation. An uncontrolled component approach might be used for a file input, where the file is only needed when the form is submitted and there's no need to manage its state via React.
Subscribe to my newsletter
Read articles from Akash Thoriya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
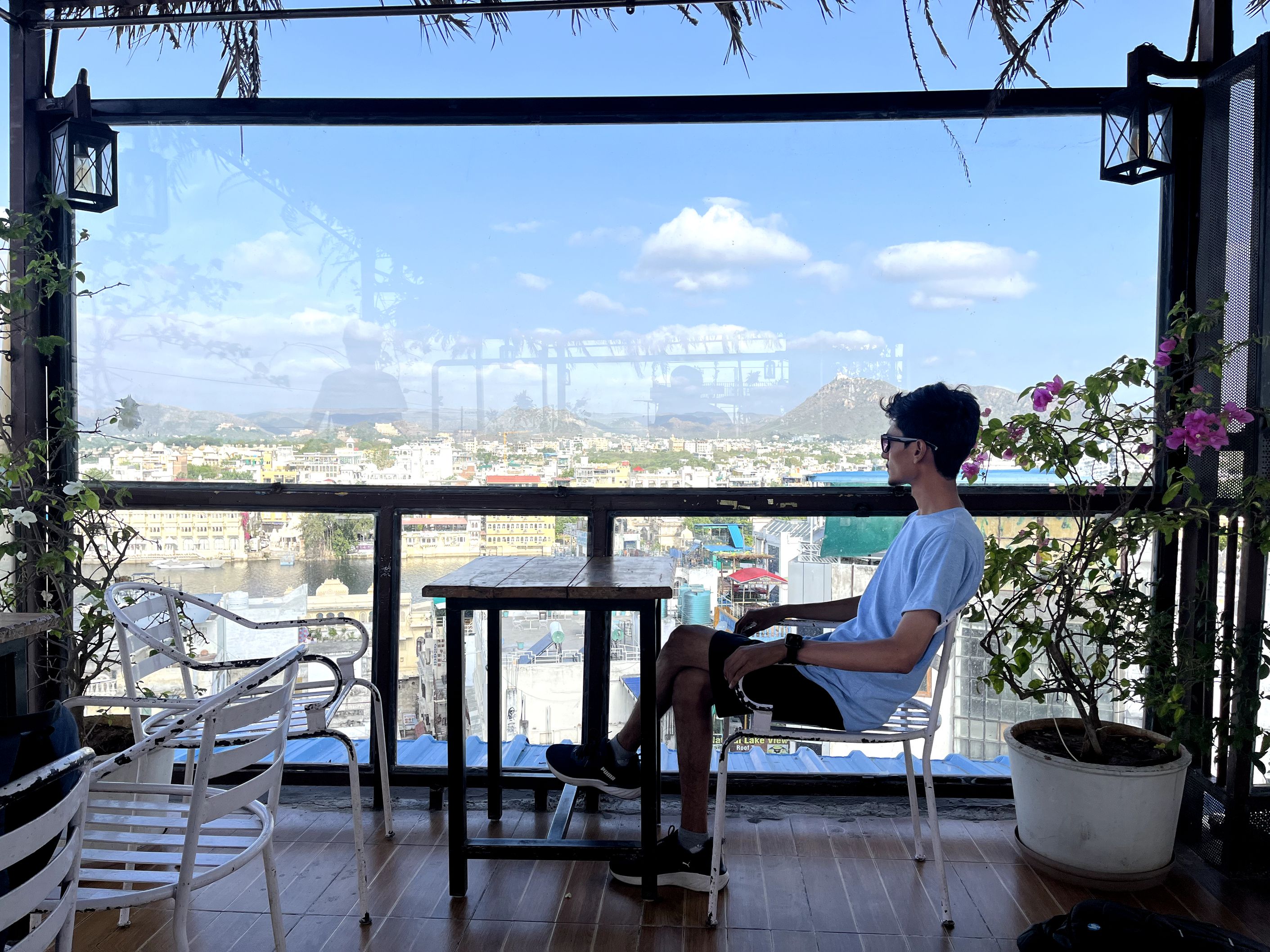
Akash Thoriya
Akash Thoriya
As a senior full-stack developer, I am passionate about creating efficient and scalable web applications that enhance the user experience. My expertise in React, Redux, NodeJS, and Laravel has enabled me to lead cross-functional teams and deliver projects that consistently exceed client expectations.