Provider Pattern in React
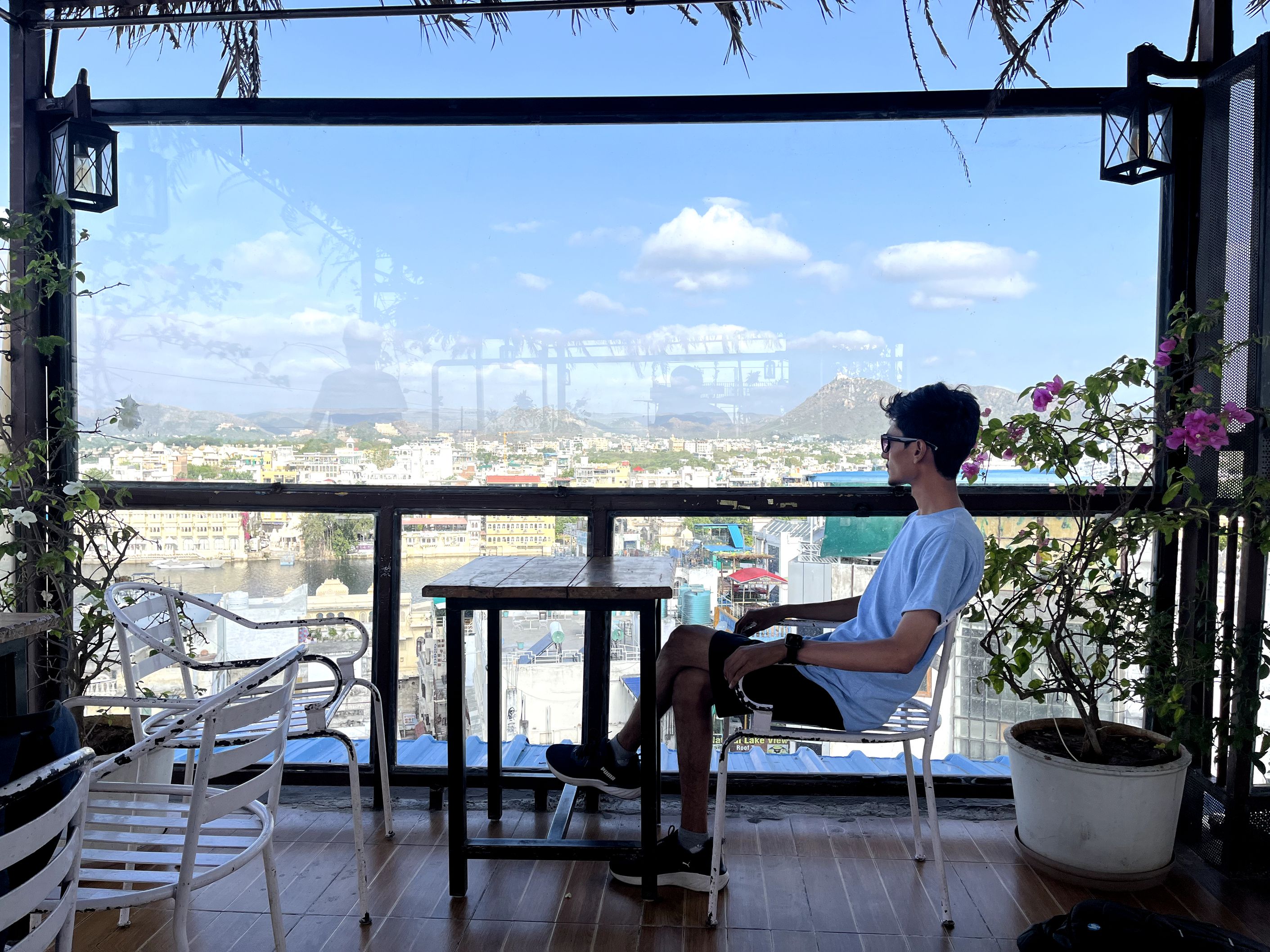
Expert-Level Explanation
The Provider Pattern in React leverages the Context API to pass data deeply through the component tree without explicitly passing props at every level. This pattern involves two main parts: the holding component Provider
, which holds the state and provides it to its children, and the consuming component, which accesses the state. This pattern is particularly useful for sharing global data like themes, user settings, or authentication status across many components.
Creative Explanation
Imagine a large family living in a house with several floors. Rather than sending messages up and down the stairs every time (passing props through every component), they install an intercom system (the Provider Pattern). This way, anyone in the house can communicate and access shared information (like dinner time or the WiFi password) directly, no matter where they are in the house.
Practical Explanation with Code
Implementing the Provider Pattern:
import React, { createContext, useState } from 'react';
// Create a Context
const MyContext = createContext();
// Provider Component
export const MyProvider = ({ children }) => {
const [value, setValue] = useState('Initial Value');
return (
<MyContext.Provider value={{ value, setValue }}>
{children}
</MyContext.Provider>
);
};
// Consuming Component
const MyComponent = () => {
const { value, setValue } = useContext(MyContext);
return (
<div>
<p>{value}</p>
<button onClick={() => setValue('Updated Value')}>Update</button>
</div>
);
};
Real-World Example
In an online store, the Provider Pattern can manage user authentication status. A top-levelAuthProvider
component wraps the entire application, providing all child components with access to the user's login state and functions to log in or out without manually passing these down through props.
Subscribe to my newsletter
Read articles from Akash Thoriya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
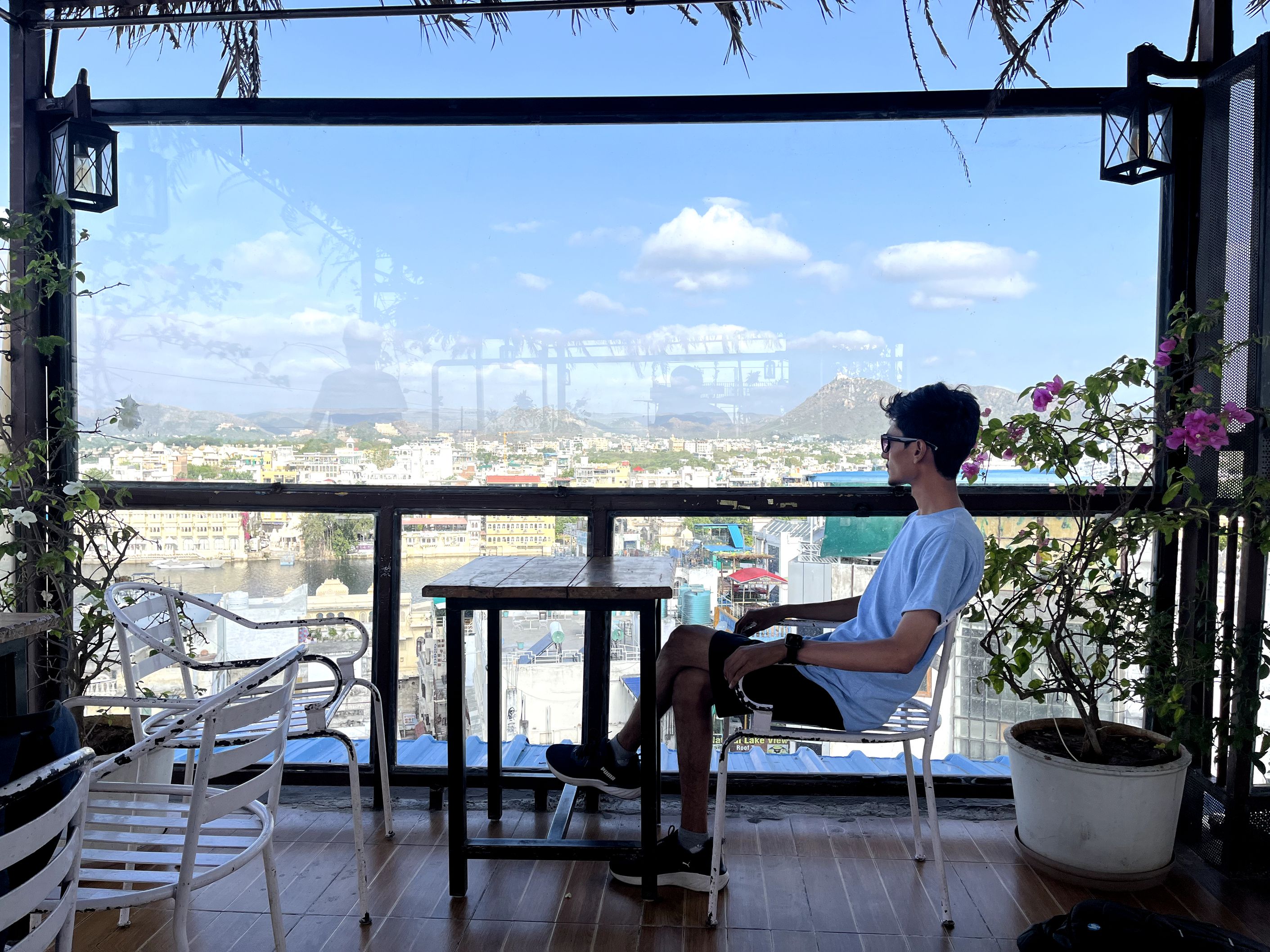
Akash Thoriya
Akash Thoriya
As a senior full-stack developer, I am passionate about creating efficient and scalable web applications that enhance the user experience. My expertise in React, Redux, NodeJS, and Laravel has enabled me to lead cross-functional teams and deliver projects that consistently exceed client expectations.