Mastering User Notifications with React Toastify
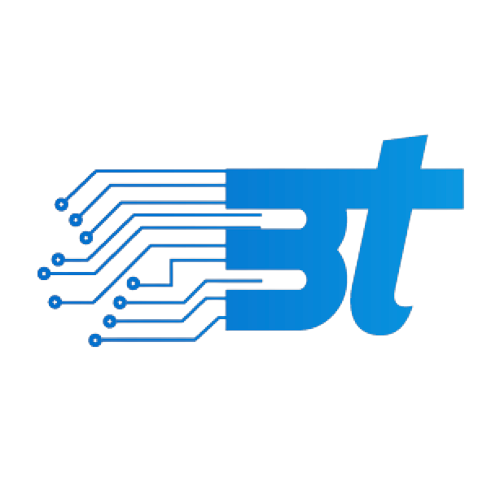
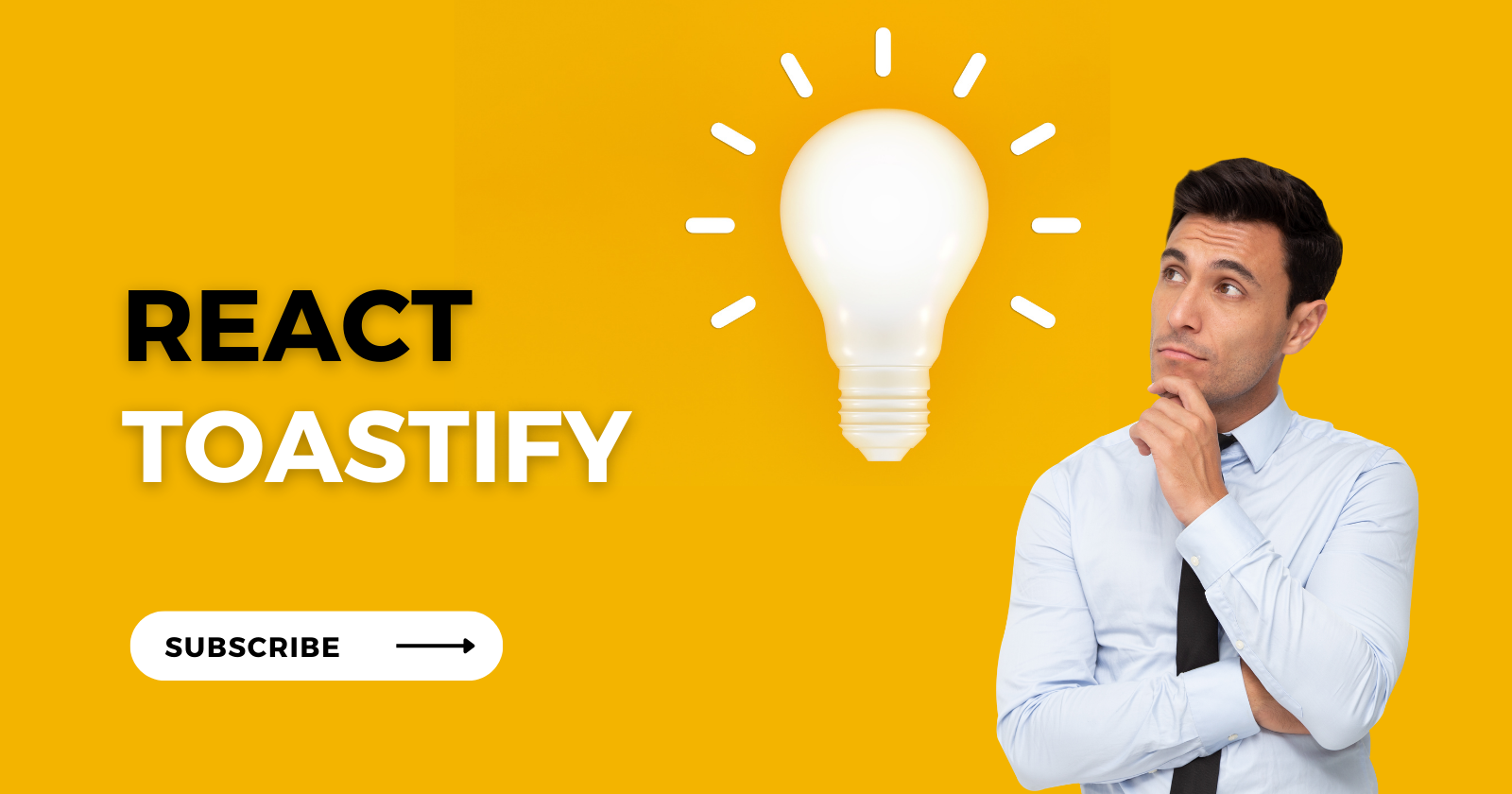
In the world of web development, creating a seamless and user-friendly experience is paramount. One crucial aspect of this is providing effective and unobtrusive notifications to users. React Toastify is a powerful library that allows developers to easily implement and customize notifications in their React applications. In this blog post, we'll explore the basics of React Toastify and how you can leverage its advanced features to master user notifications.
Getting Started with React Toastify
Installation
To begin, you need to install React Toastify in your project. You can do this using npm or yarn:
npm install react-toastify
# or
yarn add react-toastify
Basic Usage
Once installed, you can import and use React Toastify in your React components. Here's a basic example:
import React from 'react';
import { ToastContainer, toast } from 'react-toastify';
import 'react-toastify/dist/ReactToastify.css';
const MyComponent = () => {
const notify = () => toast.success('Hello, world!');
return (
<div>
<button onClick={notify}>Show Notification</button>
<ToastContainer />
</div>
);
};
export default MyComponent;
This simple example demonstrates how easy it is to display a notification using React Toastify. The ToastContainer
component is responsible for managing and rendering the toasts, while the toast
object provides methods to show different types of notifications.
Customizing Notifications
React Toastify provides a wide range of customization options to tailor notifications to your application's design. You can customize the appearance, position, animation, and more.
Toast Types
React Toastify supports various toast types out of the box, including success
, error
, warning
, and info
. You can use these types to indicate different kinds of messages to your users:
toast.success('Success!');
toast.error('Error!');
toast.warning('Warning!');
toast.info('Informational message.');
Custom Styling
To style your toasts, you can use the className
and style
props. Additionally, you can provide a custom toastClassName
to style the entire toast container:
toast.success('Custom styled toast', {
className: 'custom-toast',
bodyClassName: 'custom-body-toast',
progressClassName: 'custom-progress-toast',
});
Positioning
You can control the position of your toasts by setting the position
prop in the ToastContainer
. Options include top-right
, top-center
, top-left
, bottom-right
, bottom-center
, and bottom-left
.
<ToastContainer position="bottom-right" />
Advanced Features
React Toastify goes beyond the basics with advanced features that empower developers to create sophisticated notification systems.
Toast Lifecycle Events
React Toastify provides lifecycle events that allow you to hook into various stages of a toast's lifecycle, such as onOpen
, onClose
, and onExit
. This enables you to execute custom logic when a toast is displayed, closed, or fully exited:
toast.success('Custom lifecycle events', {
onOpen: () => console.log('Toast opened'),
onClose: () => console.log('Toast closed'),
onExit: () => console.log('Toast fully exited'),
});
Toast Configuration
You can configure the behaviour of your toasts globally using the toast.configure
method. This is particularly useful for setting default options for all toasts in your application:
import { toast } from 'react-toastify';
toast.configure({
autoClose: 3000,
draggable: false,
// other global options...
});
Async Operations
React Toastify supports asynchronous operations, allowing you to wait for a toast to be fully closed before executing additional logic:
const notify = async () => {
await toast.promise(promiseFunction(), {
pending: 'Pending operation...',
success: 'Operation succeeded!',
error: 'Operation failed!',
});
};
Conclusion
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
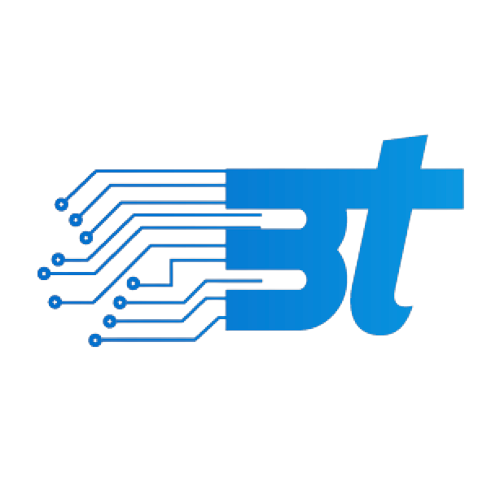
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.