Python Data Structures List Methods Usage.

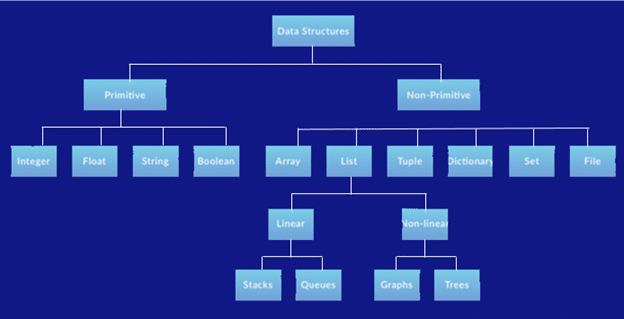
Python: list methods usage.
append()
- Adds an element to the end of the list.my_list = [1, 2, 3] my_list.append(4) print(my_list)
Output:
[1, 2, 3, 4]
clear()
- Removes all elements from the list.my_list = [1, 2, 3] my_list.clear() print(my_list)
Output:
[]
copy()
- Creates a shallow copy of the list.original_list = [1, 2, 3] copied_list = original_list.copy() print(copied_list)
Output:
[1, 2, 3]
count()
- Returns the number of occurrences of a specified element in the list.my_list = [1, 2, 2, 3, 2] count_of_2 = my_list.count(2) print(count_of_2)
Output:
3
extend()
- Appends the elements of another list to the end of the current list.list1 = [1, 2, 3] list2 = [4, 5, 6] list1.extend(list2) print(list1)
Output:
[1, 2, 3, 4, 5, 6]
index()
- Returns the index of the first occurrence of a specified element.my_list = [1, 2, 3, 2, 4] index_of_2 = my_list.index(2) print(index_of_2)
Output:
1
insert()
- Inserts an element at a specified position.my_list = [1, 2, 3] my_list.insert(1, 4) print(my_list)
Output:
[1, 4, 2, 3]
pop()
- Removes and returns the element at the specified position (default is the last element).my_list = [1, 2, 3] popped_element = my_list.pop(1) print(popped_element) print(my_list)
Output:
2 [1, 3]
remove()
- Removes the first occurrence of a specified element.my_list = [1, 2, 3, 2, 4] my_list.remove(2) print(my_list)
Output:
[1, 3, 2, 4]
reverse()
- Reverses the order of elements in the list.my_list = [1, 2, 3, 4] my_list.reverse() print(my_list)
Output:
[4, 3, 2, 1]
sort()
- Sorts the elements of the list in ascending order (or specified order).my_list = [4, 2, 1, 3] my_list.sort() print(my_list)
Output:
[1, 2, 3, 4]
These examples demonstrate the basic usage of each list method in Python.
Subscribe to my newsletter
Read articles from parmar pavan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
