Unveiling the Dynamic World of Iterators: Your Guide to Traversing Java Collections
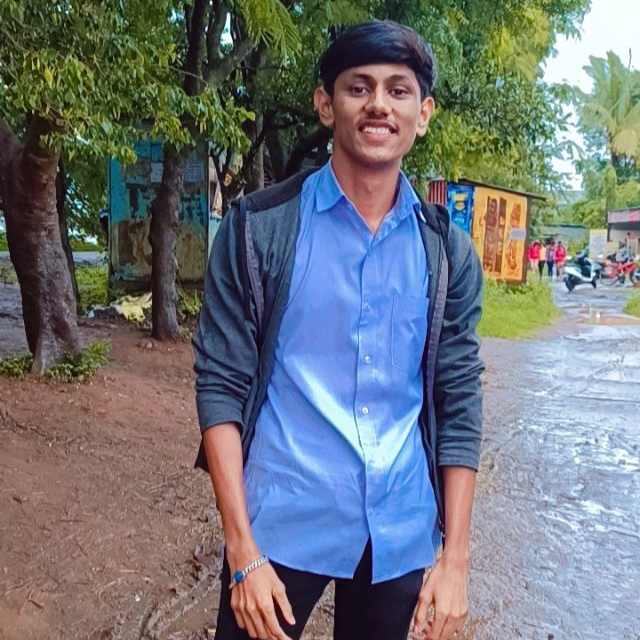
Table of contents
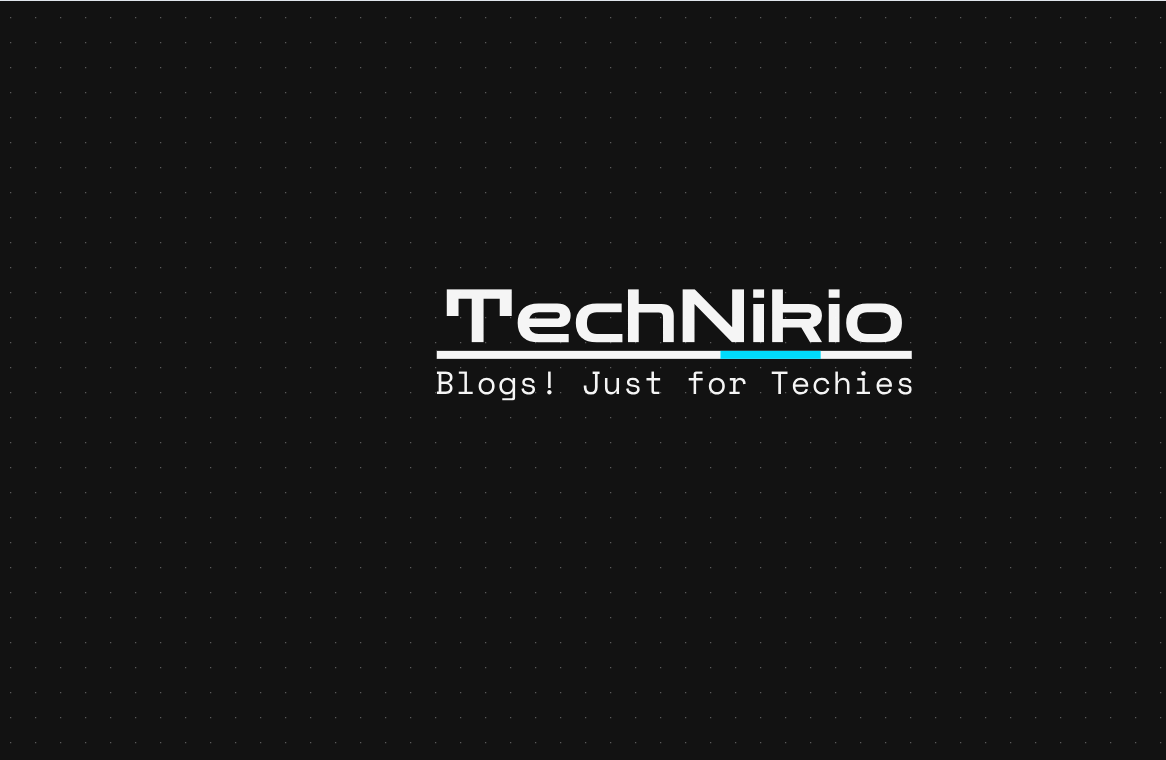
#CodeMagicLaughs🦸♂️
1. Introduction:
In the vibrant universe of Java programming, one of the most exhilarating journeys lies within the realm of iterators. As we delve into the heart of collections, we unearth a treasure trove of iterators, each with its unique charm and functionality. Join us as we embark on an exhilarating adventure through the labyrinth of Java collections, guided by the versatile iterators at our disposal.
2. Ways to Traverse the Collection:
Iterator
ListIterator
Enumerator
SplitIterator
Method Reference
For loop
For each loop
foreach() method
3. Purpose:
1. Iterator:
Definition: Iterator is an interface in Java that allows sequential access to elements in a collection.
The standard iterator used to traverse most collections that implement the iterable interface it allows you to move through the elements of a collection in a forward direction.
Usage: It is commonly used with classes implementing the Collection interface (e.g., ArrayList, LinkedList, HashSet, TreeSet) and the Map interface (e.g., HashMap, TreeMap).
Syntax
Iterator<T> iterator = collection.iterator();
Example:
List<String> list = new ArrayList<>(); list.add("Java"); list.add("Python"); list.add("JavaScript"); Iterator<String> iterator = list.iterator(); while(iterator.hasNext()) { String element = iterator.next(); System.out.println(element); }
2. ListIterator:
Definition: ListIterator extends the Iterator interface and allows bidirectional traversal through a list, along with element modification.
Specifically used for lists. It extends the Iterator interface and allows you to traverse the list bidirectionally.
Usage: It's specifically designed for classes that implement the List interface (e.g., ArrayList, LinkedList, Vector).
Syntax:
ListIterator<T> li = list.listIterator();
Example:
List<String> list = new ArrayList<>(); list.add("Apple"); list.add("Banana"); list.add("Orange"); ListIterator<String> listIterator = list.listIterator(); // TO TRAVERSE NEXT ELEMENT while(listIterator.hasNext()) { String element = listIterator.next(); System.out.println(element); } // TO TRAVERSE PREVIOUS ELEMENT while(listIterator.hasPrevious()) { String element = listIterator.previous(); System.out.println(element); }
3. Enumerator (Legacy):
Definition: Enumerator is an interface in Java used to iterate through elements of a collection, primarily legacy collection classes like Vector and Hashtable.
An older iterator used with legacy collections like vector and Hashtable.
Its less commonly used in modern Java.
Usage: It's used with legacy collection classes such as Vector and Hashtable.
Syntax:
Vector<String> vector = new Vector<>(); Enumeration<T> enums = vector.elements();
Example:
Vector<String> vector = new Vector<>(); vector.add("Java"); vector.add("Python"); vector.add("JavaScript"); Enumeration<String> enumeration = vector.elements(); while(enumeration.hasMoreElements()) { String element = enumeration.nextElement(); System.out.println(element); }
4. SplitIterator (Java 8):
Definition: Introduced in Java 8, SplitIterator is an interface used for iterating elements sequentially and potentially in parallel.
Introduced in Java8, SplitIterator is designed for parallel traversal and splitting of data in certain collections, such as streams and parallel streams.
Usage: It's primarily used for parallel processing of elements in collections and streams.
Syntax:
SplitIterator<T> si = collection.splitIterator();
Example:
List<String> words = Arrays.asList("Java", "Python", "JavaScript", "C++"); Spliterator<String> spliterator = words.spliterator(); spliterator.forEachRemaining(System.out::println);
5. Method reference (Java 8):
Definition: Method reference is a shorthand syntax introduced in Java 8 for invoking a method by referring to it with the help of double colon (::) notation.
Usage: It's used in conjunction with functional interfaces, including those defined in Java's Collection framework.
Syntax:
Method references provide a shorthand syntax for invoking a method by referring to it with the help of the double colon (::) notation. The syntax of method reference varies slightly depending on the context in which it's used:
Reference to a Static Method:
arduinoCopy codeClassName::staticMethodName
Reference to an Instance Method of an Object:
phpCopy codeobjectReference::instanceMethodName
Reference to an Instance Method of a Containing Object:
arduinoCopy codeContainingClass::instanceMethodName
Reference to a Constructor:
arduinoCopy codeClassName::new
Here's a breakdown of each syntax:
ClassName: Refers to the name of the class containing the method.
staticMethodName: Refers to the name of the static method being referenced.
objectReference: Refers to an instance of a class.
instanceMethodName: Refers to the name of the instance method being referenced.
ContainingClass: Refers to the class containing the instance method being referenced.
new: Refers to the constructor of a class.
Example:
Static Method Reference:
javaCopy codeFunction<Integer, String> converter = Integer::toString;
Instance Method Reference:
javaCopy codeList<String> names = Arrays.asList("Alice", "Bob", "Charlie"); names.forEach(System.out::println);
Reference to an Instance Method of a Containing Object:
javaCopy codeclass MyClass { void print(String message) { System.out.println(message); } } MyClass myObject = new MyClass(); Consumer<String> consumer = myObject::print; consumer.accept("Hello, world!");
Constructor Reference:
javaCopy codeSupplier<List<String>> listSupplier = ArrayList::new;
6. For Loop:
Definition: The traditional for loop is used for iterating over a collection or an array with a defined start, end, and increment/decrement.
Usage: It can be used with arrays or any class that implements the Iterable interface (which includes most collection classes in Java).
Syntax:
for(initialization;condition;increment){ // operation }
Example:
int[] array = {1, 2, 3, 4, 5}; for(int i = 0; i < array.length; i++) { System.out.println(array[i]); }
7. for-each Loop:
Definition: Also known as the enhanced for loop, it provides a concise way to iterate over elements in an array or a collection.
Usage: It's used with arrays, or any class that implements the Iterable interface, including most collection classes in Java.
Syntax:
for(datatype variable:list){ // operations }
Example:
List<String> list = new ArrayList<>(); list.add("Apple"); list.add("Banana"); list.add("Orange"); for(String fruit : list) { System.out.println(fruit); }
8. forEach() method (Java 8):
Definition: Introduced in Java 8, the forEach() method is part of the Iterable interface and allows executing a given action for each element in the collection.
Usage: It's used with any class implementing the Iterable interface, which includes collection classes in Java.
Example:
List<String> list = new ArrayList<>(); list.add("Apple"); list.add("Banana"); list.add("Orange"); list.forEach(System.out::println);
Conclusion:
In our exploration of Java collection traversal, we've journeyed through various methods like iterators, loops, and streams, mastering the art of navigating collections with ease. As we conclude this chapter, we invite you to stay tuned for our next adventure: sorting and searching within the Collection framework. Get ready to unravel the mysteries of efficient data manipulation in Java. Stay tuned for more exciting insights! 🚀👨💻
The countdown begins—don't miss the thrill! 🚀💻 #JavaCollectionMagic #NextChapterUnleashed 🌈✨
#CodeMagicLaughs🦸♂️
Happy coding! 🚀✨
Subscribe to my newsletter
Read articles from Nikhil Abhiman Jadhav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
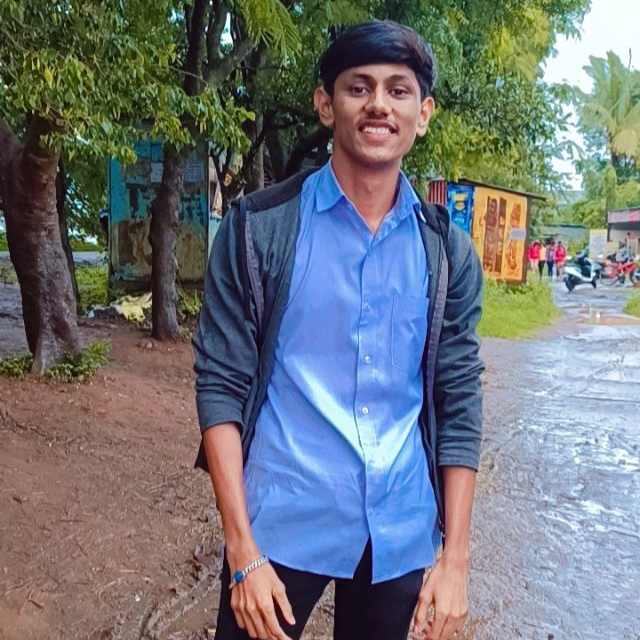
Nikhil Abhiman Jadhav
Nikhil Abhiman Jadhav
Hey there 👋🏻, I'm Nikhil Jadhav, a passionate tech enthusiast and aspiring writer on a mission to demystify the complexities of coding and technology. By day, I'm immersed in lines of code, and by night, I'm weaving words to make tech more accessible and enjoyable for everyone. 📬 Get in touch Twitter: https://twitter.com/Technikio LinkedIn:linkedin.com/in/nikhil-7571nik GitHub:github.com/jadhavnikhil2624