First-class Functions
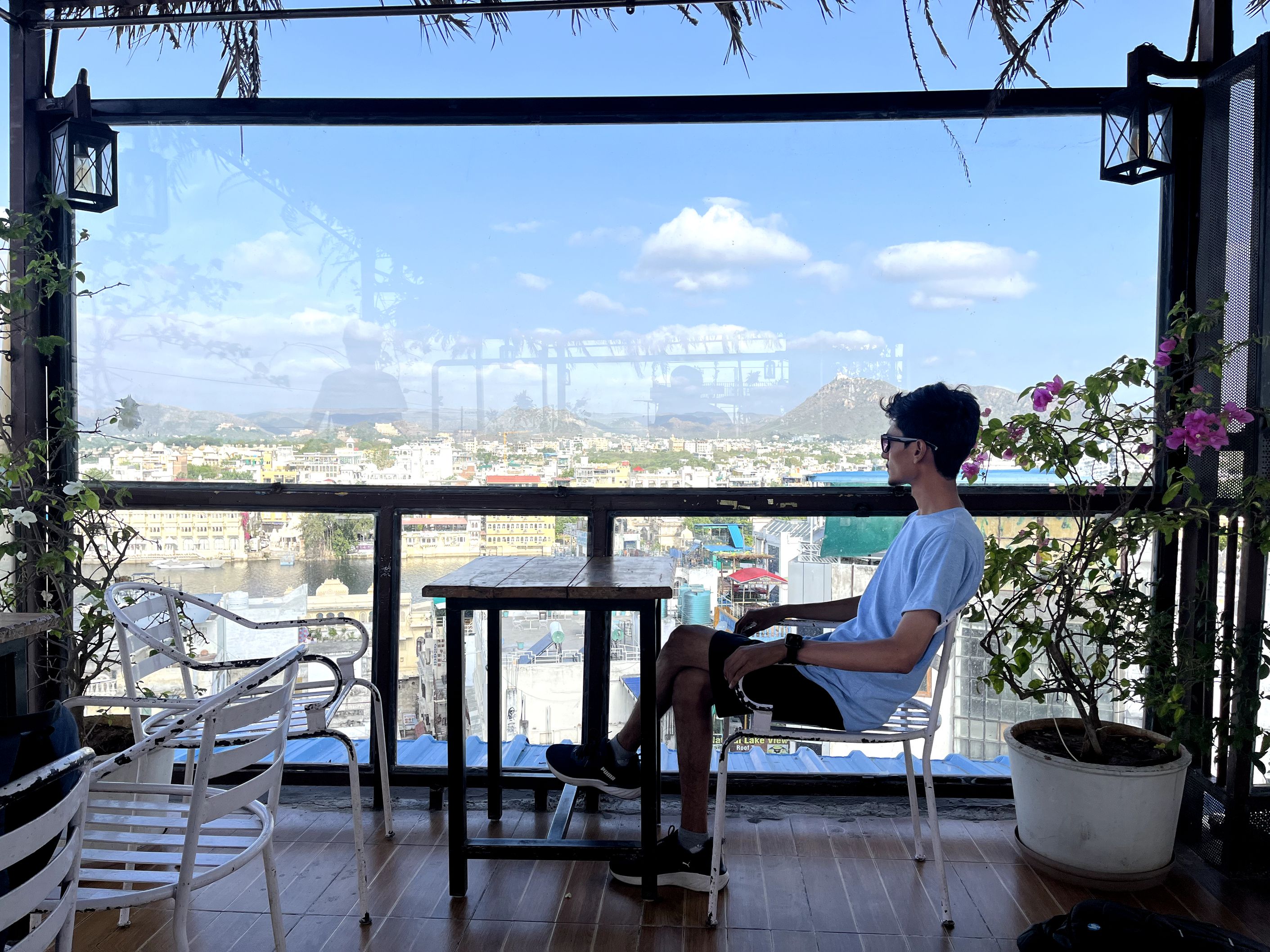
Expert-Level Explanation
In JavaScript, first-class functions mean that functions are treated as values. This means that functions can be assigned to variables, passed as arguments to other functions, and returned from other functions, just like any other value or object.
Creative Explanation
Imagine functioning as VIP guests (first-class citizens) in a country called JavaScript. They can travel anywhere, be stored in variables like homes, get on flights as arguments to other functions, and even invite other VIP guests to the country (return other functions).
Practical Explanation with Code
// Assigning a function to a variable
const greet = function() { console.log("Hello World!"); };
greet(); // Calls the function
// Passing a function as an argument
function sayHello(fn) { fn(); }
sayHello(greet); // Passing greet as an argument
// Returning a function
function greeter() {
return function() { console.log("Hello again!"); };
}
const greetAgain = greeter();
greetAgain(); // Calls the returned function
Real-world Example
A first-class function is like a digital file. You can email it (pass as an argument), store it on a USB drive (assign to a variable), or copy it into another file (return from another function).
Subscribe to my newsletter
Read articles from Akash Thoriya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
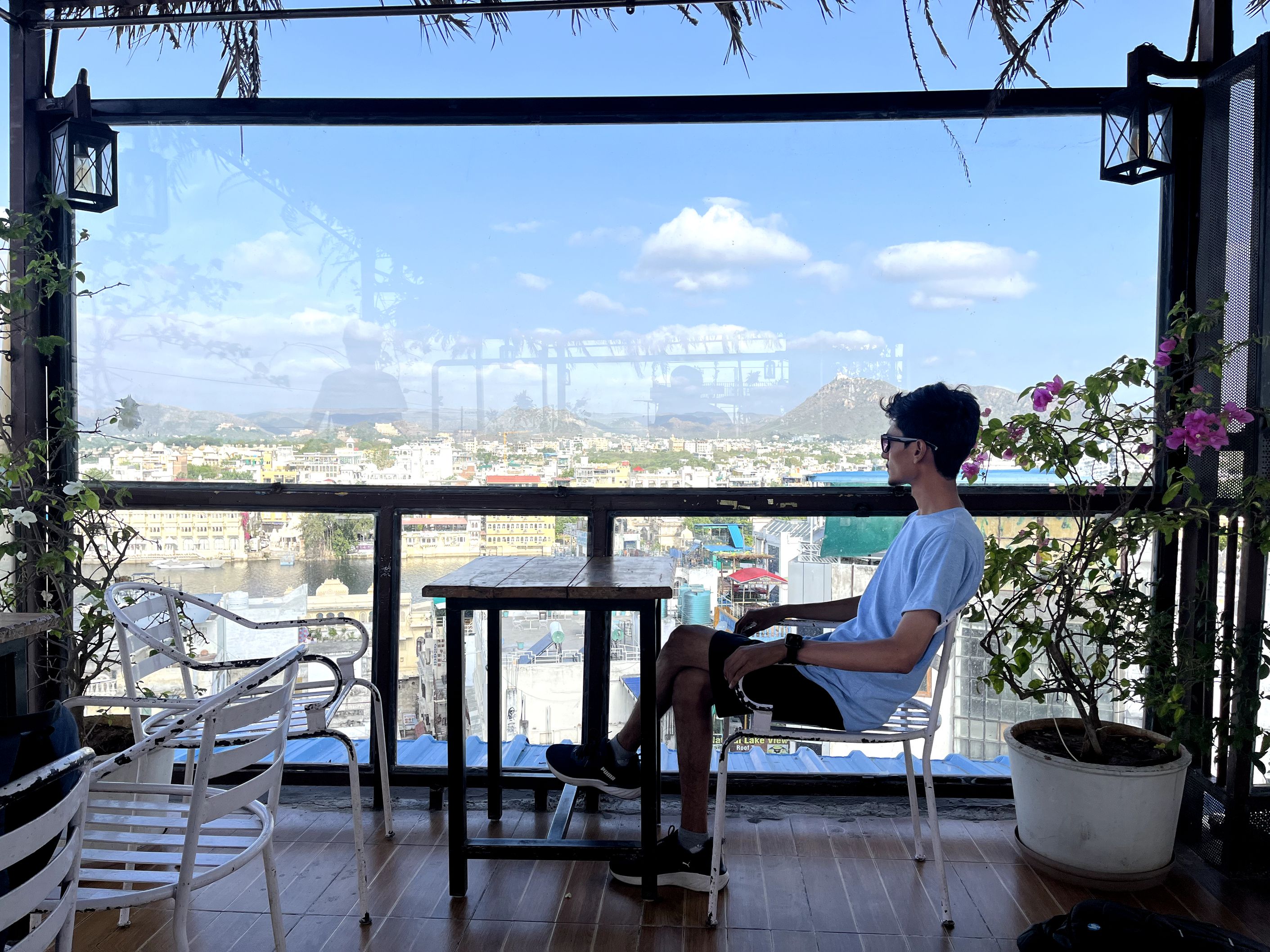
Akash Thoriya
Akash Thoriya
As a senior full-stack developer, I am passionate about creating efficient and scalable web applications that enhance the user experience. My expertise in React, Redux, NodeJS, and Laravel has enabled me to lead cross-functional teams and deliver projects that consistently exceed client expectations.