Class Syntax (constructor, extends, super, get, set)
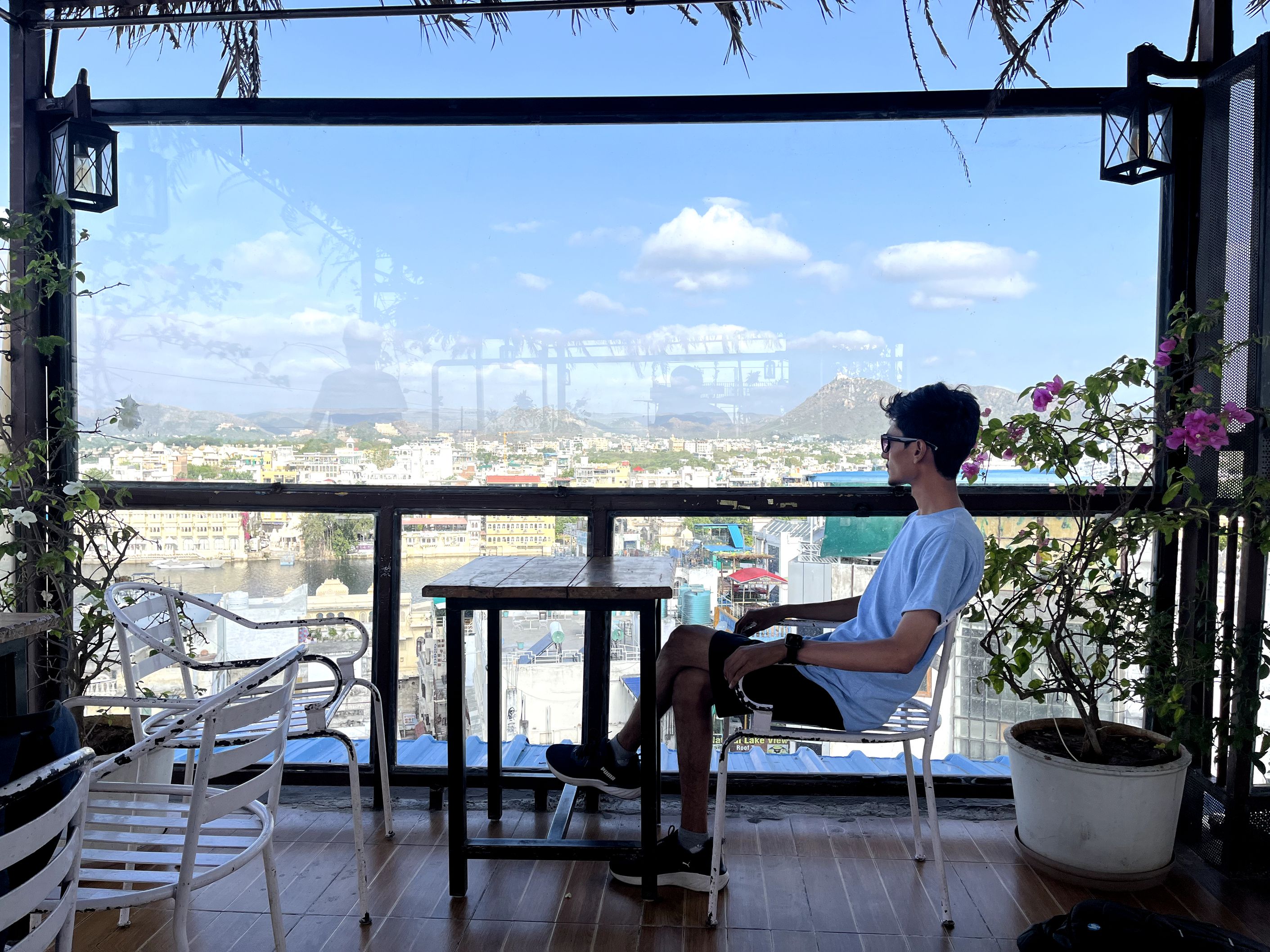
Expert-Level Explanation
In JavaScript, class syntax provides a more clear and concise way to create objects and deal with inheritance. A class can include a constructor (for initialising new objects), methods to define behaviours, and can extend other classes. The extends
keyword is used for inheritance,super
for calling the parent's constructor, and get
/set
for accessing properties in a controlled way.
Creative Explanation
Think of a class as a blueprint for building a house. The constructor is like the ground-breaking ceremony where you lay down the foundation. extends
is like designing your house based on an existing model but with your own modifications. super
is like referencing the original blueprint when making these modifications. get
andset
are like having security guards at the gate who control who enters and exits.
Practical Explanation with Code
class Animal {
constructor(name) {
this.name = name;
}
speak() {
console.log(`${this.name} makes a sound.`);
}
}
class Dog extends Animal {
constructor(name, breed) {
super(name); // Call the super class constructor
this.breed = breed;
}
speak() {
console.log(`${this.name} barks.`);
}
}
let dog = new Dog("Rex", "Golden Retriever");
dog.speak(); // Rex barks.
Real-world Example
Imagine a class like a recipe for a specific type of cake. You can create many cakes (objects) from this recipe, and if you want a different flavour (subclass), you can modify the original recipe (inheritance) while keeping the basic steps (super). The get
and set
methods are like having specific instructions on how to add or remove ingredients.
Subscribe to my newsletter
Read articles from Akash Thoriya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
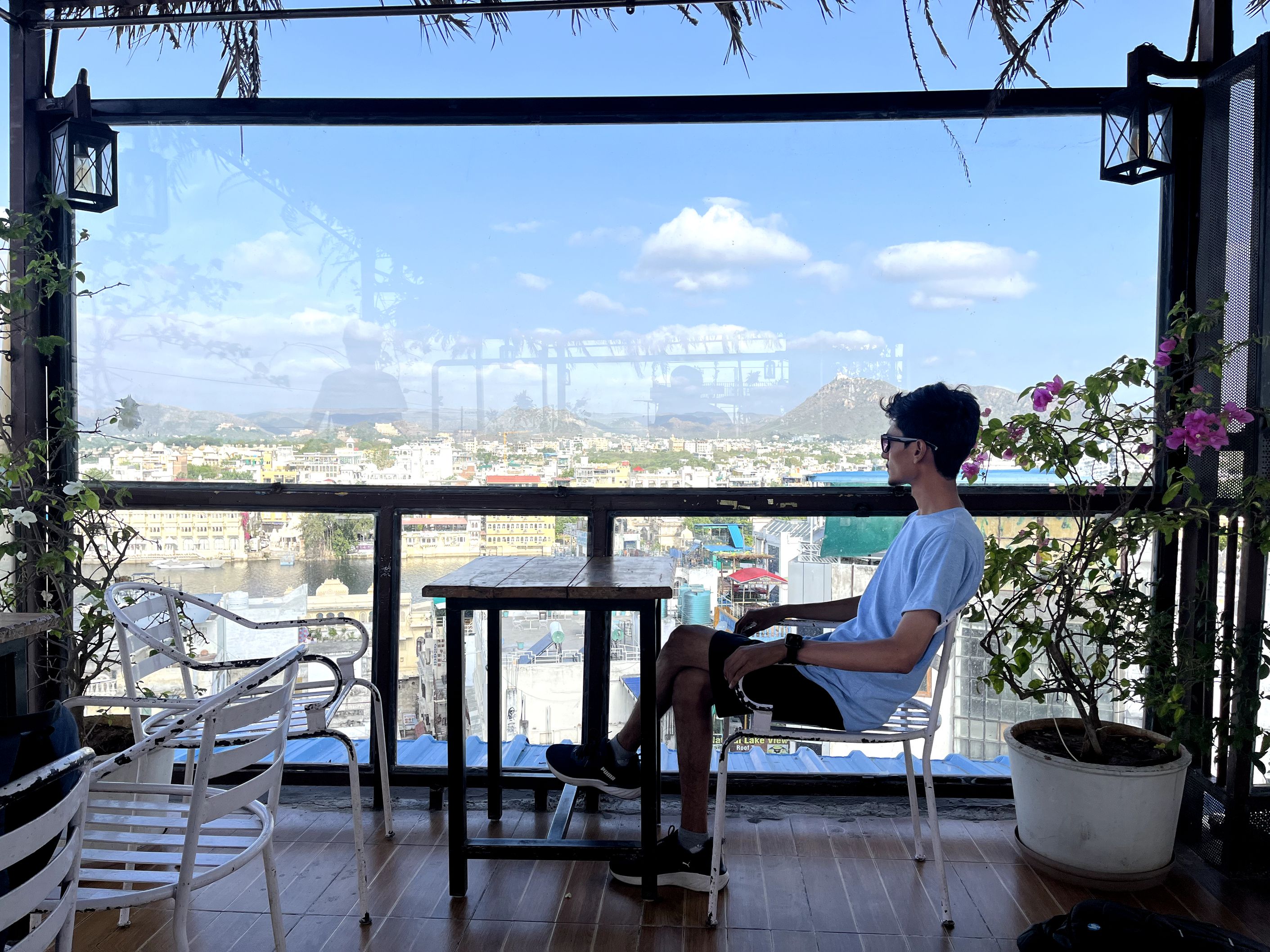
Akash Thoriya
Akash Thoriya
As a senior full-stack developer, I am passionate about creating efficient and scalable web applications that enhance the user experience. My expertise in React, Redux, NodeJS, and Laravel has enabled me to lead cross-functional teams and deliver projects that consistently exceed client expectations.