JavaScript DOM Manipulation Series (Part - IV)

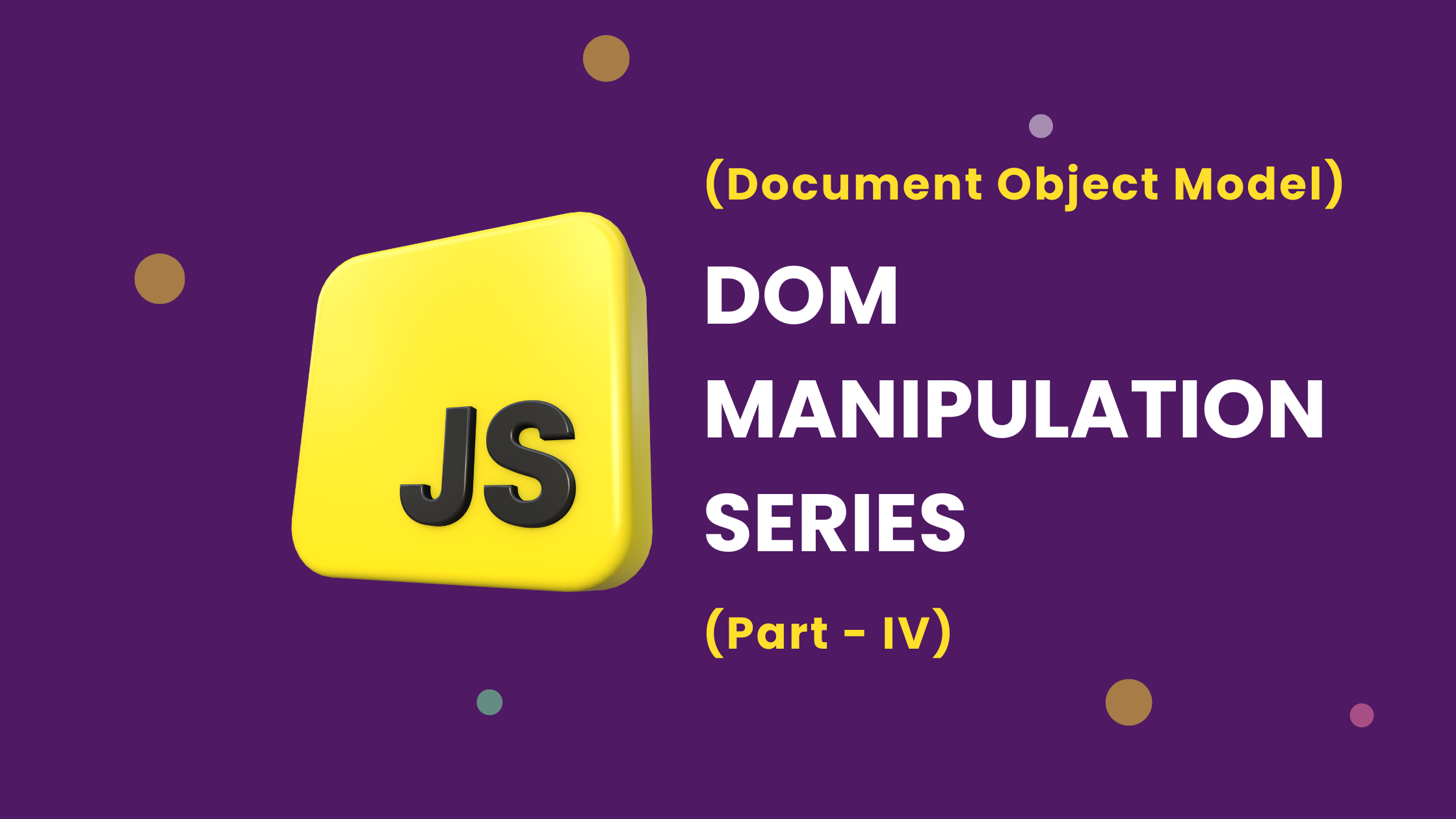
Introduction
In the previous three installments of this blog post series, we've traversed the world of JavaScript DOM manipulation, mastering the techniques for selecting, modifying, and creating elements within the HTML document. Now, we step into the realm of event handling, the art of making web pages respond to user interactions.
Event Handling in JavaScript:
Event handling is the lifeblood of interactive web applications. It enables us to capture user actions, such as mouse clicks, mouse over, keyboard presses, and form submissions, and trigger corresponding JavaScript code. This dynamic interaction transforms static web pages into engaging and responsive experiences.
1. Click Event:
The click event is one of the most fundamental and widely used events in web development. It occurs when a user interacts with an HTML element by clicking on it, whether it's a button, a link, an image, or any other clickable element. This event is a cornerstone for creating interactive and responsive user interfaces.
Basic Example:
Consider a simple example where we have an HTML button, and we want to display an alert when the button is clicked.
Here's how you might implement it:
<!-- HTML -->
<button id="myButton">Click me</button>
<!-- JavaScript -->
<script>
// Adding a click event listener to the button
const myButton = document.getElementById('myButton');
myButton.addEventListener('click', function() {
alert('Button clicked!');
});
</script>
Explanation:
There is a simple HTML button with the id "myButton."
Now, We use JavaScript to select the button element by its id.
document.getElementById('myButton')
An event listener is added to the button for the "click" event using
addEventListener
.The event listener contains a function that displays an alert saying "Button clicked!" when the button is clicked.
This example illustrates a basic use case where a click event is used to initiate a simple action (displaying an alert). In practical scenarios, click events are commonly employed for more complex interactions, such as form submissions, navigation, or toggling visibility.
2. Mouseover Event:
The mouseover event is triggered when the mouse cursor enters the area occupied by an HTML element. This event is valuable for creating interactive and dynamic user interfaces by allowing developers to respond to the user's movement over specific elements.
Basic Example:
Consider a simple example where we have an HTML div, and we want to change its background color when the mouse cursor hovers over it:
<!-- HTML -->
<div id="myDiv"></div>
<!-- CSS -->
<style>
#myDiv {
width: 100px;
height: 100px;
background-color: lightblue;
}
</style>
<!-- JavaScript -->
<script>
// Adding a mouseover event listener to the div
const myDiv = document.getElementById('myDiv');
myDiv.addEventListener('mouseover', function() {
myDiv.style.backgroundColor = 'lightcoral';
});
// Adding a mouseout event listener to revert the color when the mouse leaves
myDiv.addEventListener('mouseout', function() {
myDiv.style.backgroundColor = 'lightblue';
});
</script>
Explanation:
There is a simple HTML div with the id "myDiv."
The div has initial styling, setting its width, height, and background color to "lightblue."
We use JavaScript to select the div element by its id
document.getElementById('myDiv')
.An event listener is added to the div for the
mouseover
event usingaddEventListener
.Inside the
mouseover
event listener, the background color of the div is changed to "lightcoral."Another event listener is added for the
mouseout
event, and inside this listener, the background color is reverted to "lightblue" when the mouse leaves the div.
This example illustrates a common use case for the mouseover event, enhancing the visual feedback for users interacting with specific elements on a webpage. Such interactive effects are frequently employed in buttons, links, and other UI components to create a more engaging user experience.
3. Keyboard Event:
The keyboard events in JavaScript are events triggered by user interactions with the keyboard. These events allow developers to capture and respond to various keyboard actions such as key presses, key releases, and key holds. By handling keyboard events, you can create more dynamic and user-friendly web applications.
Basic Example:
Consider a simple example where we capture and display the key pressed by the user in an HTML input field:
<!-- HTML -->
<input type="text" id="myInput" placeholder="Type something...">
<!-- JavaScript -->
<script>
const myInput = document.getElementById('myInput');
// Adding a keydown event listener to the input field
myInput.addEventListener('keydown', function(event) {
const pressedKey = event.key;
alert('Key pressed: ' + pressedKey);
});
</script>
Explanation:
There is a simple HTML input field with the id "myInput" and a placeholder instructing the user to type something.
We use JavaScript to select the input field by its id
document.getElementById('myInput')
.An event listener is added to the input field for the
keydown
event usingaddEventListener
.The event handling function is triggered when a key is pressed down.
It retrieves the pressed key from the event object using
event.key
.An alert is displayed with the message "Key pressed: [pressed key]".
This example illustrates a basic use case for capturing keyboard input. In a real-world scenario, you might use keyboard events to perform actions like form validation, navigating through a menu, or controlling elements in a game.
Conclusion:
Congratulations on completing this blog post series on DOM manipulation in JavaScript! Throughout this comprehensive series, we've embarked on a journey into the realm of JavaScript DOM manipulation, uncovering the tools and techniques that empower us to transform static web pages into dynamic and interactive masterpieces.
From selecting and modifying elements to creating and appending new ones, we've delved into the intricacies of altering the very structure of HTML documents using JavaScript's powerful DOM manipulation methods. We've explored the nuances of styling elements to enhance their visual appeal and introduced the concept of event handling, enabling our web applications to respond to user interactions with seamless reactivity.
As you continue your journey in web development, remember to practice these concepts in real-world projects. Experiment with different scenarios, explore advanced techniques, and stay up-to-date with the latest developments in JavaScript and the DOM.
Thank you for joining us on this exploration of DOM manipulation. We hope this series has equipped you with the knowledge and skills needed to create engaging and interactive web applications.
Check out previous blogs of this series for complete details aboutDOM Manipulation in JavaScript!โ๏ธ
~HappyCoding!๐
Don't forget to visit us on these platform!
Instagram/thecodingco
YouTube/thecodingc0
Website/thecodingco
Telegram/thecodingco
Subscribe to my newsletter
Read articles from TheCodingCo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
