A Guide to Modern JavaScript Array Methods

Table of contents
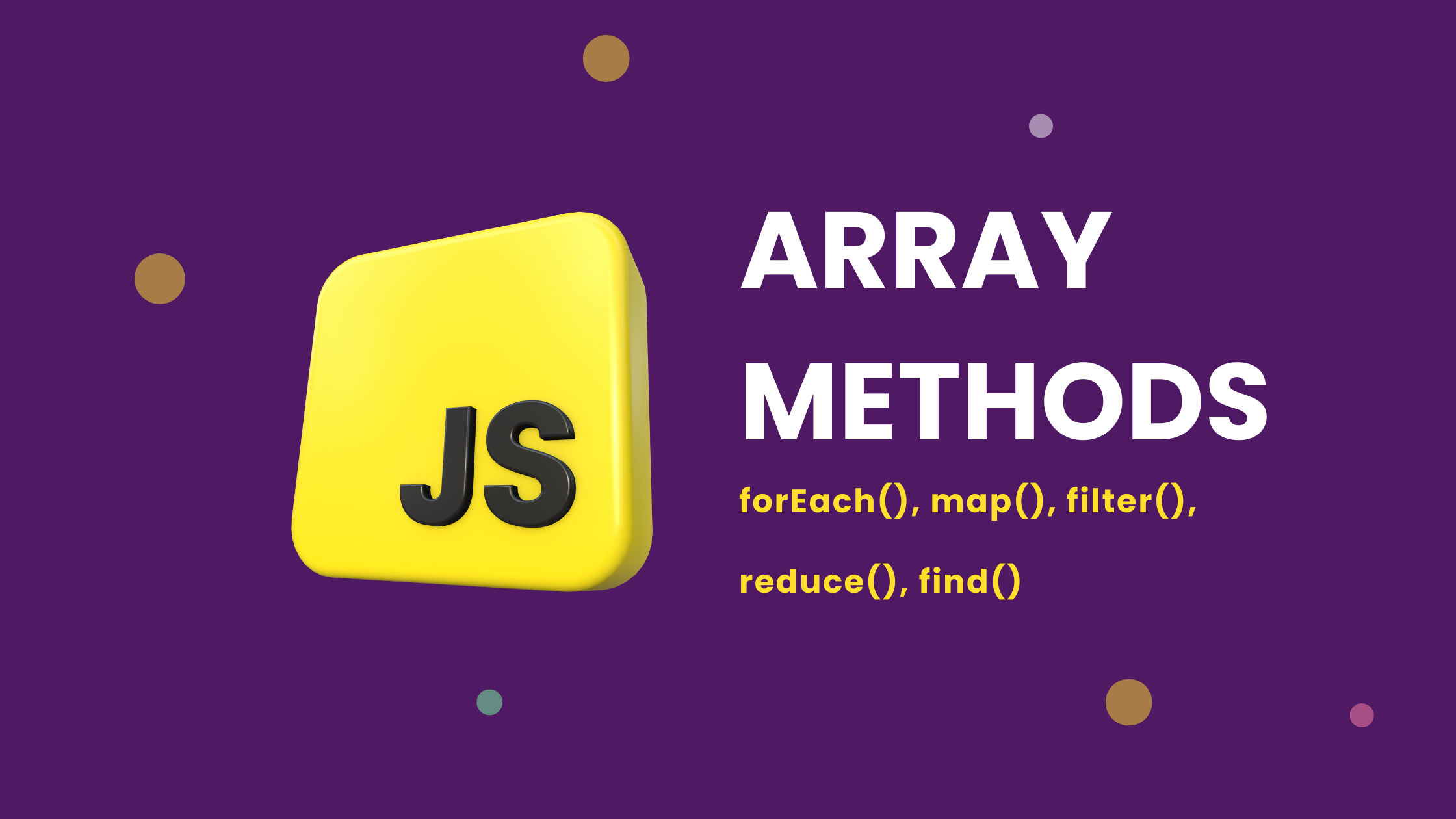
Introduction
JavaScript is a versatile programming language that continues to evolve, offering developers a wide range of tools and techniques to enhance their coding skills.
In this blog post, we will dive deep into one of the most powerful aspects of JavaScript Array Methods.
Specifically, we will explore the modern array methods that have been introduced in recent years. By understanding and mastering these methods, you will be able to write more efficient and concise code while leveraging the full potential of JavaScript.
forEach():
The forEach()
method allows you to iterate over each element in an array and execute a provided function for each element. It is an elegant alternative to traditional for loops.
Here's a simple example:
const numbers = [1, 2, 3, 4, 5];
numbers.forEach((number) => {
console.log(number * 2);
});
/*
Output:
2
4
6
8
10
*/
Real World Example:
Imagine, You are a manager at a retail store, and you need to calculate the total cost of all the items in a customer's shopping cart before they checkout.
const cartItems = [
{ name: 'Shirt', price: 20 },
{ name: 'Pants', price: 30 },
{ name: 'Shoes', price: 40 },
{ name: 'Jacket', price: 50 }
];
let totalCost = 0; // Initialize totalCost
cartItems.forEach(item => {
totalCost += item.price;
});
console.log('Total Cost: $' + totalCost);
// Output: Total Cost: $140
Explanation:
The
forEach
method takes a callback function as an argument, which is called once for each item in the array. In this case, the callback function is anonymous and takes one argument,item
.Inside the callback function, we access the
price
property of the currentitem
and add it to thetotalCost
variable.Since we don't need to return anything from the callback function, we don't include a return statement. Instead, we simply update the
totalCost
variable directly.Once the
forEach
method finishes iterating over all the items in the array, it returns undefined.
Benefits of Using:
The primary advantage of implementing
forEach
is that it allows us to change external conditions without changing the original data structure. In this example, we update thetotalCost
variable without changing the originalcartItems
array.Another benefit is that
forEach
makes code easy to read and understand, since it clearly states the intention of iterating over every item in an array and performing a specific action.
When to Use:
Use
forEach
when you need to perform an operation on each item in an array or object and don't need to return anything from the callback function.Avoid using
forEach
when you need to return a value from the callback function, since it doesn't support returning values. In such cases, consider usingmap
,filter
, or a traditional loop instead.
map():
The map()
method creates a new array by applying a provided function to each element in the original array. It is commonly used to transform or manipulate array elements.
Consider the following example:
const numbers = [1, 2, 3, 4, 5];
const doubledNumbers = numbers.map((number) => {
return number * 2;
});
console.log(doubledNumbers);
// Output: [2, 4, 6, 8, 10]
Real World Example:
Suppose, You are a developer at a travel company, and you need to display a list of destinations on your website. Each destination has a name, description, and price. You want to display only the names and prices of the destinations, but not the descriptions.
const destinations = [
{ name: 'Paris', description: 'The City of Light', price: 500 },
{ name: 'London', description: 'The British Capital', price: 400 },
{ name: 'Tokyo', description: 'A Bustling Metropolis', price: 600 },
{ name: 'New York', description: 'The Big Apple', price: 700 }
];
const namesAndPrices = destinations.map(destination => (
{
name: destination.name,
price: destination.price
}
));
console.log(namesAndPrices);
/*
Output:
[
{ name: 'Paris', price: 500 },
{ name: 'London', price: 400 },
{ name: 'Tokyo', price: 600 },
{ name: 'New York', price: 700 }
]
*/
Explanation:
The
map
method takes a callback function as an argument, which is called once for each item in the array. In this case, the callback function is anonymous and takes one argument,destination
.Inside the callback function, we create a new object with the desired properties and return it.
Since the
map
method returns a new array containing the transformed values, we assign the result to a new variable callednamesAndPrices
.Once the
map
method finishes iterating over all the items in the array, it returns the newly formed array of transformed objects.
Benefits of Using:
One advantage of using
map
is that it allows us to transform or manipulate an array of data without modifying the original array. This helps keep our data clean and maintainable.Another benefit is that
map
makes code easy to read and understand, since it clearly states the intention of transforming each item in the array into something else.
When to Use:
Use
map
when you need to transform or manipulate an array of data in some way, while keeping the original data intact.Avoid using
map
when you want to filter out certain elements from the array based on a condition. In such cases, consider using thefilter
method instead.
filter():
The filter()
method creates a new array with all elements that pass a provided condition. It is often used to extract specific elements from an array.
Let's look at an example:
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = numbers.filter((number) => {
return number % 2 === 0;
});
console.log(evenNumbers);
// Output: [2, 4]
Real World Example:
Suppose, You are a developer at an e-commerce website, and you need to display a list of products that are priced above $100.
const products = [
{ name: 'Phone Case', price: 30 },
{ name: 'Headphones', price: 55 },
{ name: 'Laptop', price: 700 },
{ name: 'Speakers', price: 200 },
{ name: 'Smartwatch', price: 65 },
{ name: 'Gaming Console', price: 400 }
];
const expensiveProducts = products.filter(product => product.price > 100);
console.log(expensiveProducts);
/*
Output:
[
{ name: 'Laptop', price: 700 },
{ name: 'Speakers', price: 200 },
{ name: 'Gaming Console', price: 400 }
]
*/
Explanation:
The
filter
method creates a new array that includes only the items for which the provided callback function returnstrue
.The callback function can return any value, but it's typically a boolean value indicating whether the item should be included in the resulting array.
The
filter
method can also be used with a third argument, calledthisArg
, which specifies the context object for the callback function.
Benefits of Using:
One advantage of using
filter
is that it allows us to narrow down an array of data based on a specific criterion, making it easier to work with the data we need.Another benefit is that
filter
makes code concise and expressive, since it clearly communicates the intent to filter the array based on a particular condition.
When to Use:
Use
filter
when you need to extract a subset of data from an array based on a specific condition or criteria.Avoid using
filter
when you need to transform or manipulate the data in addition to filtering it. In such cases, consider using themap
method instead.
reduce():
The reduce()
method applies a function against an accumulator and each element in the array, resulting in a single value. It is useful for tasks like calculating a sum or finding the maximum value in an array.
Here's an example:
const numbers = [1, 2, 3, 4, 5];
const sum = numbers.reduce((accumulator, number) => {
return accumulator + number;
}, 0);
console.log(sum);
// Output: 15
Real World Example:
Imagine, You are a developer at a bank, and you need to calculate the total amount of money spent by a customer across multiple transactions. The transactions are stored in an array of objects, where each object represents a transaction and has two properties: amount
and type
(either "deposit" or "withdrawal").
const transactions = [
{ amount: 100, type: "deposit" },
{ amount: 50, type: "withdrawal" },
{ amount: 200, type: "deposit" },
{ amount: 150, type: "withdrawal" },
{ amount: 300, type: "deposit" }
];
const totalAmount = transactions.reduce((accumulator, transaction) => {
if (transaction.type === "deposit") {
accumulator += transaction.amount;
} else {
accumulator -= transaction.amount;
}
return accumulator;
}, 0);
console.log(`Total Amount: ${totalAmount}`); // Total Amount: 400
Explanation:
The
reduce()
method works by reducing an array of values into a single value. It does this by calling a provided callback function for each item in the array, passing in the previous value and the current item.The callback function can return any value, but it must return a value that is passed to the next iteration of the function.
The
reduce()
method can also take an optional second argument, calledinitialValue
, which sets the starting value of the accumulator.
Benefits of Using:
One advantage of using
reduce()
is that it allows us to handle nested data structures and perform calculations on them.Another benefit is that
reduce()
makes code concise and expressive, since it clearly communicates the intent to combine values together.
When to Use:
Use
reduce()
when you need to process a collection of data and combine it into a single value.Use
reduce()
when you need to perform a calculation across multiple items in an array and produce a final result.Avoid using
reduce()
when the calculation is simple and can be accomplished with a straightforward loop.
find():
The find()
method returns the first element in an array that satisfies a provided condition. It is commonly used to search for a specific element.
Consider the following example:
const numbers = [1, 2, 3, 4, 5];
const foundNumber = numbers.find((number) => {
return number > 3;
});
console.log(foundNumber);
// Output: 4
Real World Example:
Suppose, You are building a website for a bookstore and you need to find a specific book in an array of books. Each book object in the array has properties such as title, author, and price.
const books = [
{
title: "JavaScript: The Good Parts",
author: "Douglas Crockford",
price: 20
},
{
title: "Eloquent JavaScript",
author: "Marijn Haverbeke",
price: 30
},
{
title: "JavaScript: The Definitive Guide",
author: "David Flanagan",
price: 40
},
{
title: "You Don't Know JS",
author: "Kyle Simpson",
price: 25
}
];
const bookToFind = "JavaScript: The Definitive Guide";
const foundBook = books.find(book => book.title === bookToFind);
console.log(foundBook);
/*
output:
{
title: "JavaScript: The Definitive Guide",
author: "David Flanagan",
price: 40
}
*/
Explanation:
The
find()
method works by iterating over each item in an array and executing a callback function for each item. It returns the first item in the array that satisfies the condition specified in the callback function.The callback function takes three parameters:
currentValue
(the current item being processed),index
(the index of the current item), andarray
(the array being traversed).The callback function should return a truthy value if the item satisfies the condition, and a falsy value otherwise. Once a truthy value is returned, the
find()
method stops iterating and returns the current item.
Benefits of Using:
The
find()
method provides a concise way to search for a specific item in an array based on a condition.It returns the first matching item, allowing you to quickly find the desired element without having to iterate through the entire array.
When to Use:
Use
find()
when you need to search for an item in an array based on a specific condition.Use
find()
when you only need to find the first matching item and don't require the entire array to be processed.
Conclusion
Modern JavaScript provides an array of powerful array methods that can significantly simplify your coding tasks. By mastering methods like forEach()
, map()
, filter()
, reduce()
, and find()
, you can manipulate arrays efficiently and elegantly. Experiment with these methods, explore their possibilities, and unlock the full potential of modern JavaScript. Happy coding!
Remember, this is just a glimpse into the world of modern JavaScript array methods. There are many more methods available, each with its unique features and use cases. Stay curious, keep learning, and let the array methods empower your JavaScript journey.
~HappyCoding!๐
Follow us: TheCodingCo!!โ๏ธ
Subscribe to my newsletter
Read articles from TheCodingCo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
