Day 21 Task: Docker Important Interview Questions
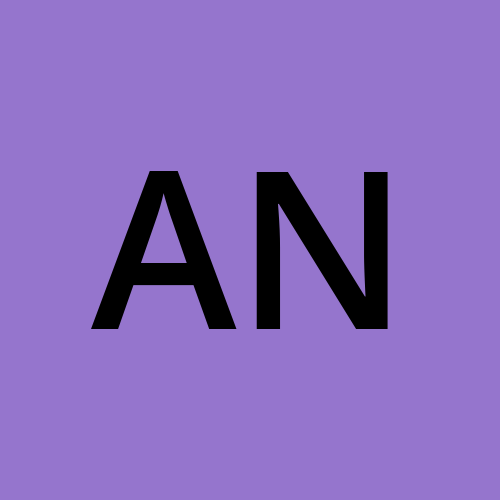
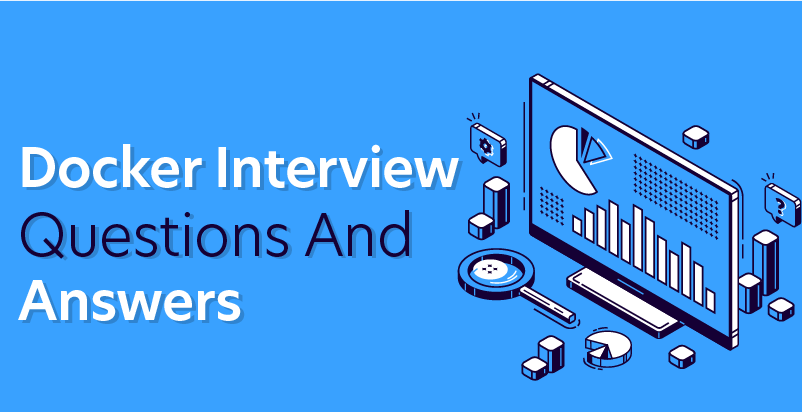
🎯What is the difference between an Image, Container and Engine?
An image is like a complete set of instructions. It's a detailed guide that tells the computer exactly how to set up and run a particular program.
A container is a self-contained package. It takes those instructions from the image and wraps them up along with all the necessary tools and resources the program needs to run.
The engine is the runtime environment. It's the software that takes the packaged program (container) and makes sure it runs smoothly, handling tasks like starting, stopping, and managing resources.
🎯What is the difference between the Docker command COPY vs ADD?
Use COPY for straightforward copying of local files and directories from the host to the container.
Use ADD if you need additional features, like copying from URLs or automatically extracting compressed archives.
🎯What is the difference between the Docker command CMD vs RUN?
Use RUN to execute commands during the Docker image build.
Use CMD to specify the default command to run when a Docker container starts.
RUN is for building the image, and CMD is for defining the default runtime behavior of the container. i.e RUN is for build-time actions, and CMD is for run-time defaults.
🎯How will you reduce the size of the Docker image?
To make Docker image smaller need to follow below steps:
Use a lightweight base image like Alpine Linux.
Use multi-stage builds to separate build and runtime.
Minimize layers and use the
&&
operator to combine commands.Clean up unnecessary files and dependencies.
Use a .dockerignore file to exclude unnecessary files.
Compress files before copying into the image.
Choose efficient and lightweight libraries and packages.
🎯Why and when to use Docker?
Docker is a platform that enables developers to package applications and their dependencies into isolated, portable containers. It addresses challenges like inconsistent environments, simplifies deployment through containerization, ensures consistency across different systems, and facilitates scalability. Docker allows for easy experimentation with tools without impacting the host system, streamlines deployment processes, and optimizes resource utilization. It's particularly valuable in scenarios where maintaining consistency, isolating environments, and achieving efficient scaling are crucial factors in application development and deployment.
🎯Explain the Docker components and how they interact with each other.
Docker includes several components that work together to create and manage containers.
Docker Daemon:
The Docker daemon (dockerd) is a background process that manages Docker containers on a host system.
It listens for Docker API requests and manages container lifecycles, networking, and storage.
Docker Client:
The Docker client (docker) is a command-line tool or API that allows users to interact with the Docker daemon.
Users issue commands to the Docker client, which then communicates with the Docker daemon to execute those commands.
Docker Images:
Docker images are lightweight, standalone, and executable packages that include application code, libraries, dependencies, and system tools.
Images are used as the basis for creating Docker containers.
Docker Containers:
Containers are instances of Docker images. They run applications in isolated environments with their own file systems, processes, and networking.
Containers are portable and can run consistently across different environments.
Docker Registry:
Docker Registry is a centralized repository for storing and sharing Docker images.
Docker Hub is a public registry, and organizations often set up private registries to manage their images.
Docker Compose:
Docker Compose is a tool for defining and running multi-container Docker applications.
It uses a YAML file to specify the services, networks, and volumes, making it easier to manage complex applications.
Developers use the Docker client to build images, create containers, and interact with the Docker daemon. Images are stored in Docker Registries, and containers run isolated instances of these images. Docker Compose or orchestration tools help manage complex applications composed of multiple containers. Understanding how these components work together is essential for efficiently developing, deploying, and managing containerized applications using Docker.
🎯Explain the terminology: Docker Compose, Docker File, Docker Image, Docker Container?
Docker Compose helps manage multi-container applications by defining their structure in a YAML file.
Dockerfile is a script containing instructions for building a Docker image, specifying dependencies, configurations, etc.
Docker Image is a packaged, executable, and lightweight snapshot that includes everything needed to run an application.
Docker Container is a running instance of a Docker image, providing isolation and consistency for applications.
🎯In what real scenarios have you used Docker
I've utilized Docker for:
Microservices Scaling: Efficient deployment and management.
CI/CDintegration: Ensuring consistent software delivery
Multi-Cloud Probability: Seamless deployment across various cloud providers.
Automated Testing: Streamlined and reliable testing processes.
On-Premises consistency: Uniform deployment in on-premises setups.
Resource Efficiency: Maximizing server resources through containerization.
🎯Docker vs Hypervisor?
Docker is like a compact and efficient toolbox, bundling applications and their needs together for easy sharing and movement. It optimizes resource usage by sharing the host system's kernel, ensuring a lightweight and portable environment.
Hypervisors create separate, heavier workspaces for each task, resulting in more overhead and reduced portability.
Docker's approach is minimalist, promoting collaboration and flexibility, while hypervisors offer isolated, self-contained environments, suitable for standalone tasks with higher resource requirements.
🎯What are the advantages and disadvantages of using docker?
Advantages of Docker:
Portability: Easily run applications across different environments.
Isolation: Prevents "it works on my machine" issues by keeping containers independent.
Efficiency: Optimizes resource usage, running quickly and using fewer resources.
Scalability: Simple scaling with multiple containers to handle increased load.
DevOps Friendly: Integrates well into DevOps workflows, streamlining development and deployment.
Disadvantages of Docker:
Learning Complexity: Takes time to get used to Docker commands and concepts.
Security Concerns: Shared OS kernel can pose security risks.
Resource Overhead: Some resource usage overhead, impacting system performance.
Persistence Challenges: Containers are typically stateless, making persistent data storage challenging.
Compatibility Issues: Potential compatibility problems between Docker, host OS, and applications.
🎯What is a Docker namespace?
Docker namespaces are like partitions or shields for different aspects of a container's operation. Each namespace provides isolation for a specific resource, ensuring that what happens in one container doesn't interfere with others or the host system. It's a way to keep things neatly separated and avoid any unintended clashes or disruptions between containers.
🎯What is a Docker registry?
A Docker registry is a centralized repository for storing and sharing Docker container images. It serves as a hub for versioning, access control, and efficient distribution of images, either publicly (like Docker Hub) or privately within organizations.
🎯What is an entry point?
The ENTRYPOINT in a Dockerfile is like the starting point for your containerized application. It's the command or script that defines what happens first when your container is fired up. You specify the ENTRYPOINT in the Dockerfile, telling Docker how to kick off your application within the container. It's essentially the initial action, setting the stage for what goes on inside your container.
🎯How to implement CI/CD in Docker?
Continuous Integration (CI):
Connect code to platforms like Git.
Automate builds and tests with CI tools like Jenkins or GitLab or Circle CI.
Store Docker images in registries like Docker Hub or AWS ECR.
Continuous Deployment (CD):
Define deployment with tools like Docker Compose or Kubernetes.
Automate deployment using orchestration tools.
Optionally use tools like Spinnaker for deployment approval.
Plan for rollbacks in case of issues.
Monitor with tools like Prometheus or ELK Stack.
🎯Will data on the container be lost when the docker container exits?
Yes, by default anything you save inside a Docker container disappears when you're done using it – like a temporary workspace. But, Docker provides ways to keep our data even after the container finishes its job. We can use special techniques called "volumes" or "bind mounts" to make sure your data stays around, kind of like having a permanent storage spot for our stuff.
🎯What is a Docker swarm?
Docker Swarm is like a coordinator for Docker containers. It allows you to manage and organize groups of containers as a single unit. This makes it easier to scale your applications, handle failures, and simplify the management of multiple containers working together. Essentially, it's a tool that helps orchestrate and control the behavior of your Dockerized applications.
🎯What are the docker commands for the following:
view running containers
docker ps
command to run the container under a specific name
docker run -d --name <container_name> <docker_image>
command to export a docker
docker export [OPTIONS] CONTAINER
command to import an already existing docker image
docker import [OPTIONS] file|URL|- [REPOSITORY[:TAG]]
commands to delete a container
docker rm <container_name_or_id>
command to remove all stopped containers, unused networks, build caches, and dangling images?
docker system prune -a
🎯What are the common docker practices to reduce the size of Docker Image?
Choose a Slim Base Image, Use a smaller base image like alphine
Combine related commands to minimize layers
Limit Dependencies, Install only necessary dependencies
Clean Up After Each Step, Remove unnecessary files within the same RUN command
Combine Commands to minimize layers
Use Multi-Stage Builds, Discard unnecessary build artifacts in a multi-stage build
Only install essential tools
Day 20 - Docker Cheatsheet posted on my Linkedin
🙌 Thank you for taking the time to explore this blog!📚 I hope you found the information both helpful and insightful.✨
🚀 Enjoy your learning journey, and don't hesitate to reach out if you have any feedback. 🤓 Happy exploring!
Subscribe to my newsletter
Read articles from Anusha Nayak directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
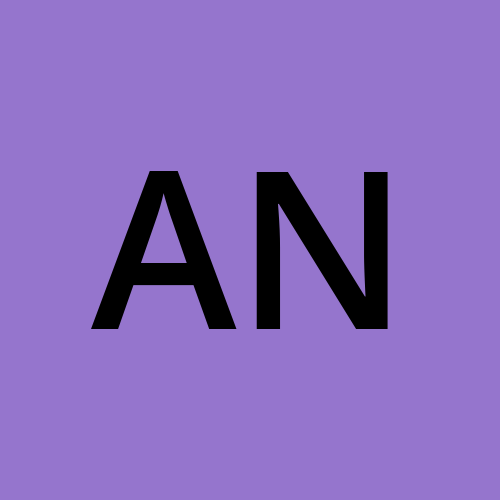
Anusha Nayak
Anusha Nayak
Hi everyone, I am Anusha Nayak. 🚀I am passionate about bridging the realms of development and operations to propel efficient and seamless software delivery! 💻 As a DevOps professional, I'm all about making things run smoother, like cutting out unnecessary steps, setting up automatic software installations, and making sure everything flows together nicely👨💻. I want to help teams create great software quickly every single time.