Unraveling the Mysteries of Sorting and Searching in Java Collections
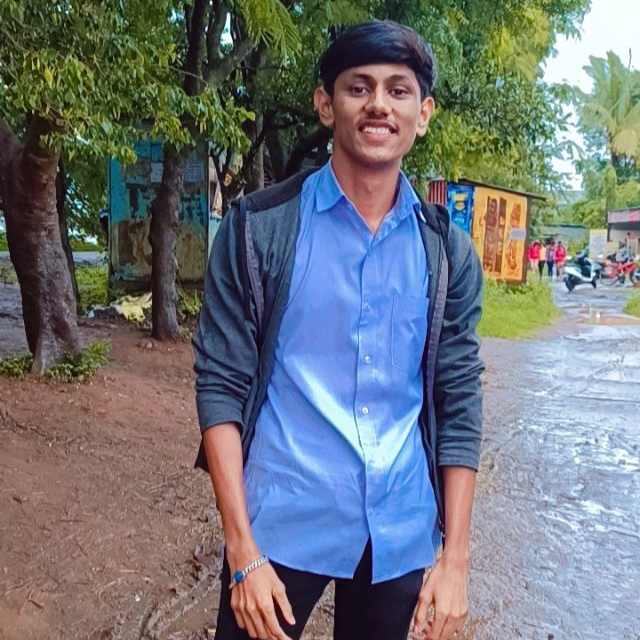
Table of contents
- #CodeMagicLaughs🦸♂️
- 1. Introduction:
- 2. Understanding Sorting:
- 3. There are 2 Interfaces used for Sorting in the Collections framework, those are:
- 4. Sorting in Java Collections:
- 5. Best Practices and Performance Considerations:
- 6. Searching in Java Collections:
- 7. Explore additional methods used in Searching:
- 8. Exploring Searching Algorithms:
- 9. Best Practices and Performance Considerations:
- Conclusion:
- #CodeMagicLaughs🦸♂️
- Happy coding! 🚀✨
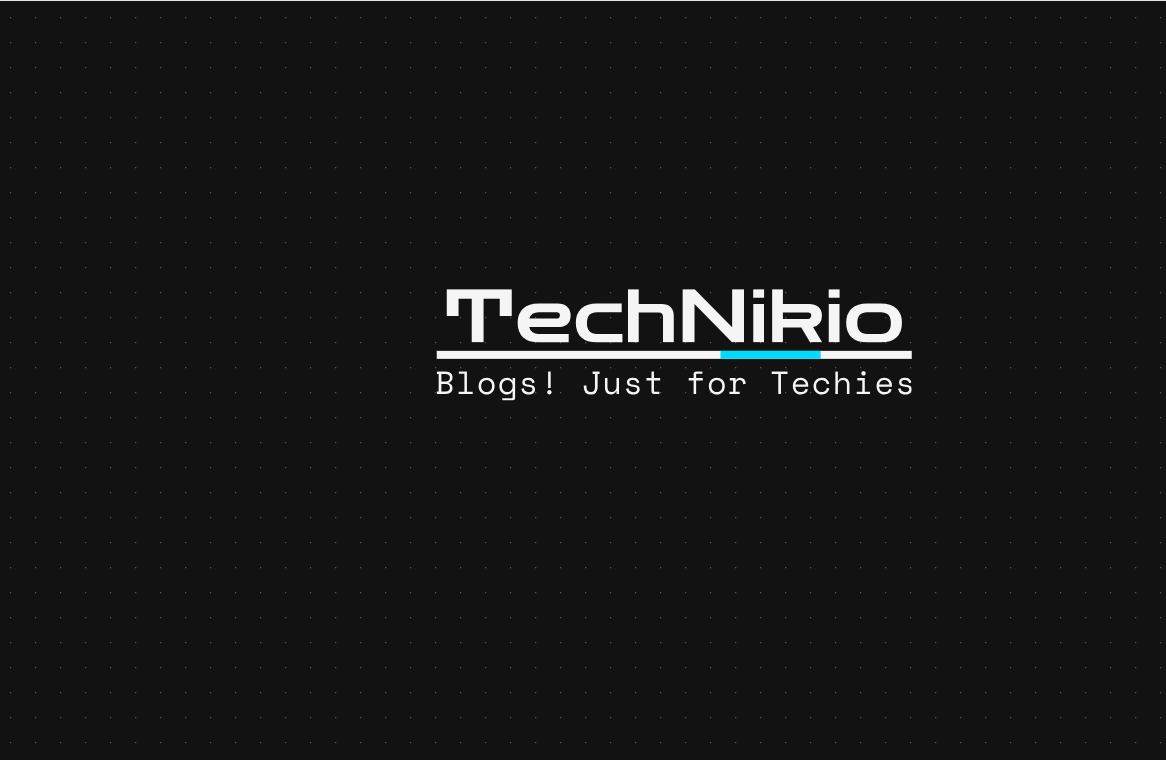
#CodeMagicLaughs🦸♂️
1. Introduction:
Welcome, fellow developers, to an exhilarating expedition through the realm of sorting and searching in the Java Collection Framework!
Join us as we delve deep into the heart of data manipulation, uncovering the secrets of sorting algorithms and searching techniques hidden within Java collections.
2. Understanding Sorting:
Sorting, the art of arranging elements in a specific order, lies at the heart of data organization and manipulation.
From the depths of arrays to the heights of collections, sorting algorithms wield the power to transform chaos into order with breathtaking precision.
Sorting are fundamental operations when working with colelctions in java.
The java collections fraemwork provides various mechanisms and classes to facilitate these operations efficiently.
3. There are 2 Interfaces used for Sorting in the Collections framework, those are:
Comparable Interface
Comparator Interface
- Comparable Interface:
Many Collection classes support sorting based on the natural order of elements,which is defined thorugh the 'Comparable' interface.
Elements in the collection must implement the 'Comparable Interface' to use this feature.
Enter the realm of natural ordering with the Comparable interface! This noble interface empowers classes to define their inherent sorting logic.
Behold the simplicity of compareTo() method, the cornerstone of natural ordering:
// Example: Implementing Comparable interface for a custom class public class Person implements Comparable<Person> { private String name; private int age; // Constructor, getters, and setters @Override public int compareTo(Person otherPerson) { return this.name.compareTo(otherPerson.getName()); } }
- Comparator Interface:
Alternatively, you can provide a custom sorting order by implementing the 'Comparator Interface' and passing it to the 'Collections.sort()' method for lists or to the constructor of sorted collections like TreeSet or TreeMap.
Explore the realm of custom sorting with the Comparator interface! This versatile interface allows developers to define their own sorting criteria.
Behold the power of compare() method, the beacon of custom ordering:
// Example: Implementing Comparator interface for custom sorting logic public class AgeComparator implements Comparator<Person> { @Override public int compare(Person person1, Person person2) { return person1.getAge() - person2.getAge(); } }
4. Sorting in Java Collections:
Witness the magic of sorting methods like Collections.sort() and Arrays.sort() as they bring order to arrays, lists, sets, and maps:
// Example: Sorting a list of Person objects using Comparable List<Person> personList = new ArrayList<>(); Collections.sort(personList); // Example: Sorting a list of Person objects using Comparator List<Person> personList = new ArrayList<>(); Collections.sort(personList, new AgeComparator());
5. Best Practices and Performance Considerations:
Arm yourselves with best practices for optimal sorting performance in Java collections.
Navigate the treacherous waters of algorithmic trade-offs and performance considerations, emerging victorious with newfound knowledge and prowess.
6. Searching in Java Collections:
Searching involves finding a specific element or elements in a collection.
The Java Collection Framework provides methods for searching and some of the most commonly used approaches include.
Navigate the maze of searching algorithms, from linear and binary to interpolation search techniques.
Unlock the power of binarySearch() method in Java collections, guiding us through sorted arrays and collections
// Example: Searching in a sorted array int[] numbers = {1, 2, 3, 4, 5, 6, 7, 8, 9}; int index = Arrays.binarySearch(numbers, 5); System.out.println("Index of 5: " + index); // Output: 4
7. Explore additional methods used in Searching:
additional methods for sorted collections:
subSet()
headSet()
tailSet()
first()
last()
additional methods for unsorted collections:
add()
remove()
contains()
isEmpty()
size()
clear()
iterator()
toArray()
8. Exploring Searching Algorithms:
Navigate the maze of searching algorithms, from linear and binary to interpolation search techniques.
Unlock the power of binarySearch() method in Java collections, guiding us through sorted arrays and collections:
// Example: Searching in a sorted array int[] numbers = {1, 2, 3, 4, 5, 6, 7, 8, 9}; int index = Arrays.binarySearch(numbers, 5); System.out.println("Index of 5: " + index); // Output: 4
9. Best Practices and Performance Considerations:
Arm yourselves with best practices for optimal sorting and searching performance in Java collections.
Navigate the treacherous waters of algorithmic trade-offs and performance considerations, emerging victorious with newfound knowledge and prowess.
Conclusion:
Conclusion: As we wrap up our voyage through sorting, searching, and the Java Collection Framework, let's savor the victories and excitement of our exploration. But hold onto your hats! The adventure doesn't end here. Stay tuned for an adrenaline-packed dive into utility classes within the Collection Framework. The thrill continues! 🚀🔍✨
With swords sharpened and minds enlightened, let us embark on this thrilling adventure through the world of sorting and searching in Java collections! 🚀📊🔍
The countdown begins—don't miss the thrill! 🚀💻 #JavaCollectionMagic #NextChapterUnleashed 🌈✨
#CodeMagicLaughs🦸♂️
Happy coding! 🚀✨
Subscribe to my newsletter
Read articles from Nikhil Abhiman Jadhav directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
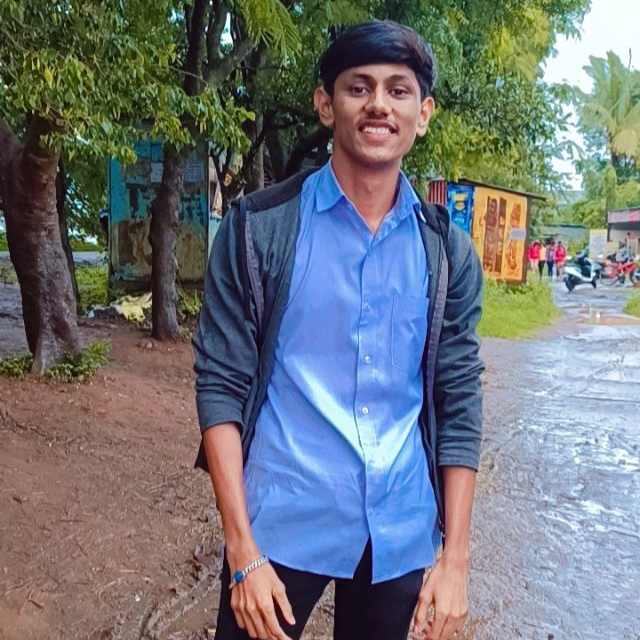
Nikhil Abhiman Jadhav
Nikhil Abhiman Jadhav
Hey there 👋🏻, I'm Nikhil Jadhav, a passionate tech enthusiast and aspiring writer on a mission to demystify the complexities of coding and technology. By day, I'm immersed in lines of code, and by night, I'm weaving words to make tech more accessible and enjoyable for everyone. 📬 Get in touch Twitter: https://twitter.com/Technikio LinkedIn:linkedin.com/in/nikhil-7571nik GitHub:github.com/jadhavnikhil2624