Here's a guide from beginner to pro for Android development:
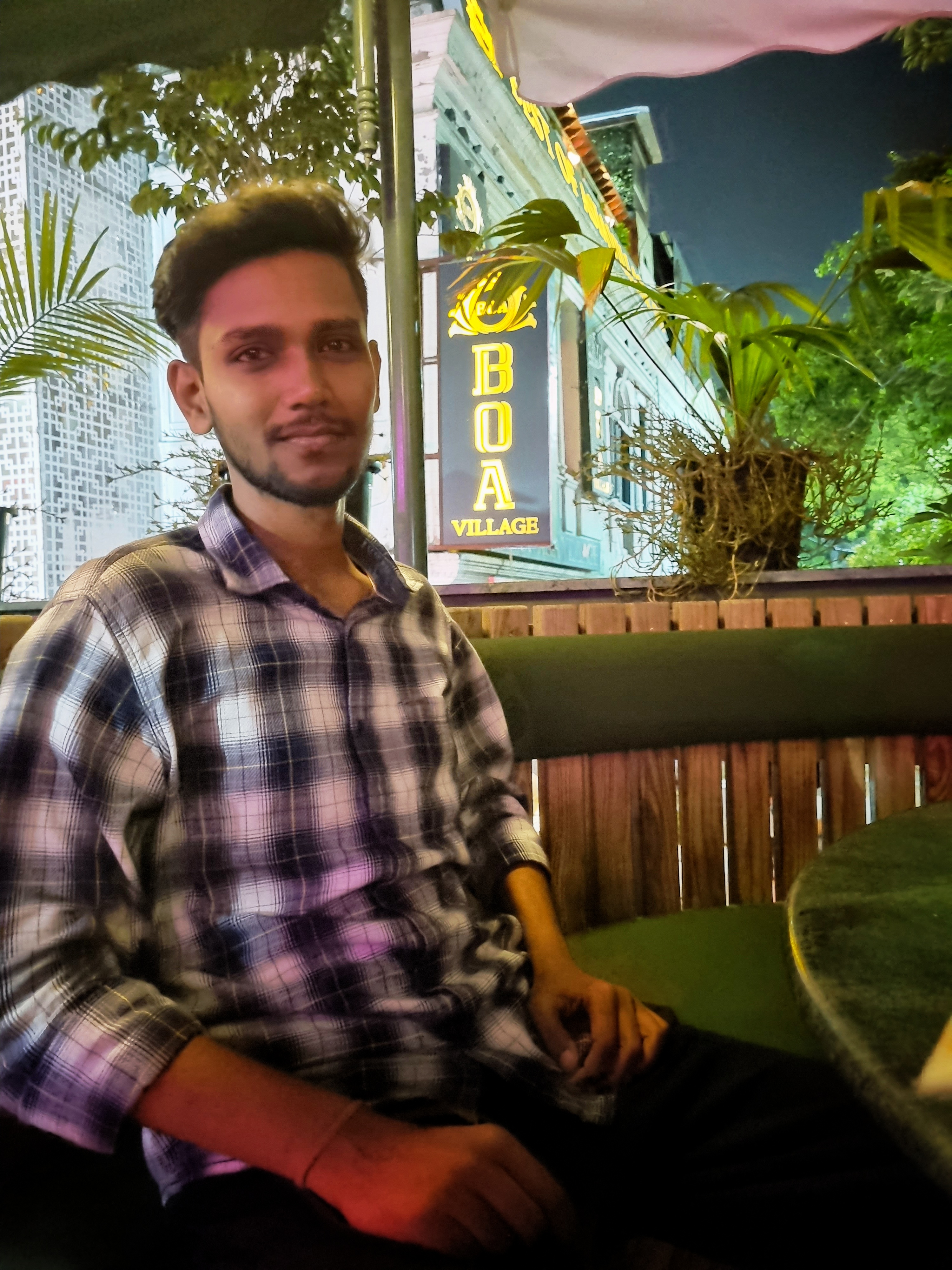
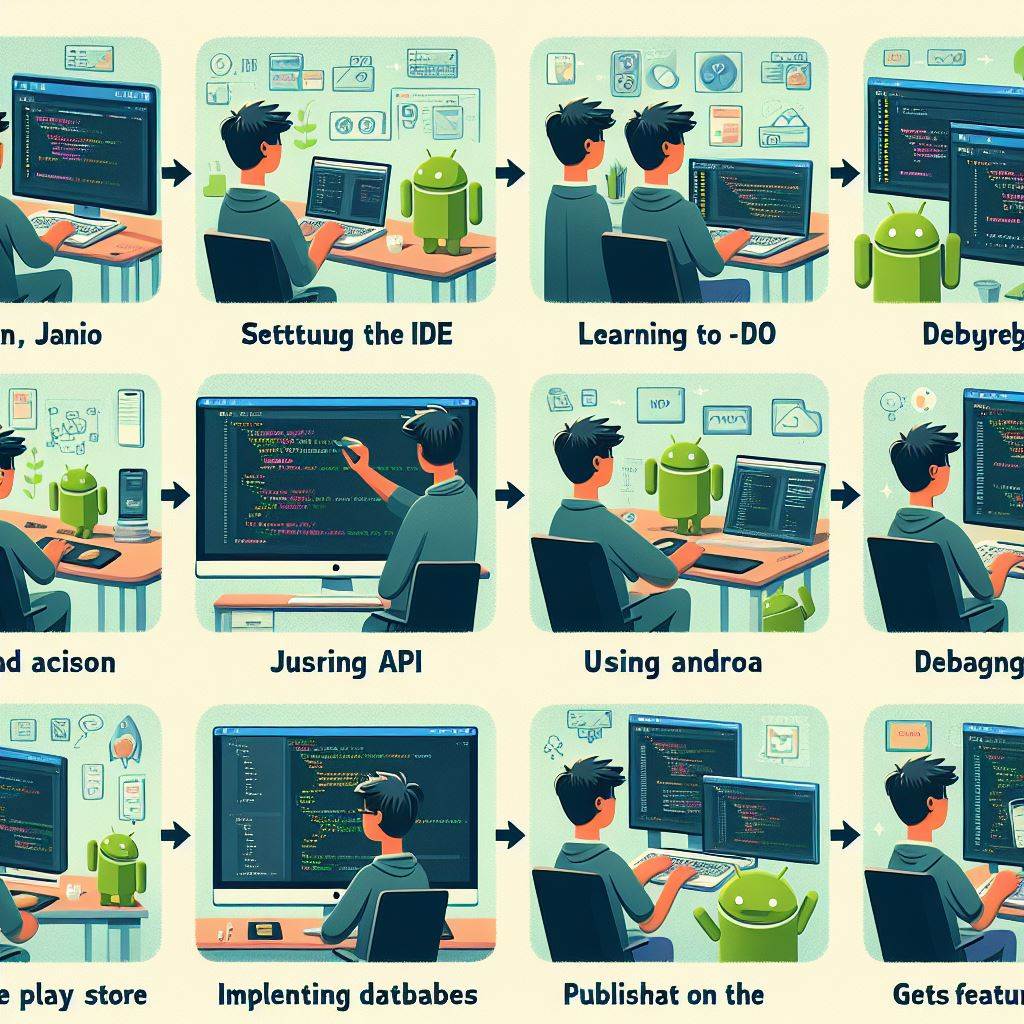
Beginner Level:
Learn the Basics of Java/Kotlin:
Understand fundamental concepts like variables, data types, loops, and conditionals.
Explore object-oriented programming (OOP) principles.
Set Up Android Studio:
- Install and set up Android Studio, the official IDE for Android development.
Hello World App:
- Create a simple Android app to understand the project structure and basic app components.
User Interface (UI) Fundamentals:
Learn XML for designing layouts.
Explore common UI components like buttons, text views, and image views.
Activity Lifecycle:
- Understand the lifecycle of an Android Activity and how to manage it.
Intent and Navigation:
- Learn how to navigate between activities using Intents.
Intermediate Level:
Fragment Basics:
- Understand fragments and their role in creating flexible UIs.
RecyclerView:
- Implement dynamic lists using RecyclerView for efficient handling of large datasets.
Data Persistence:
- Explore SQLite databases and SharedPreferences for data storage.
Networking:
- Use Retrofit or Volley to make API calls and handle JSON responses.
Permissions and Security:
- Understand Android's permission system and implement security best practices.
Material Design:
- Implement Google's Material Design principles for a modern and consistent UI.
Advanced Level:
MVVM Architecture:
- Learn and implement Model-View-ViewModel architecture for clean and maintainable code.
Dependency Injection:
- Use Dagger or Koin for dependency injection to improve code scalability.
Testing:
- Explore unit testing and UI testing with JUnit and Espresso.
Background Processing:
- Implement services, AsyncTask, or WorkManager for background tasks.
Firebase Integration:
- Integrate Firebase for features like authentication, real-time database, and cloud messaging.
Advanced UI/UX Concepts:
- Implement advanced UI animations, custom views, and responsive design.
Pro Level:
Jetpack Compose:
- Master the declarative UI toolkit for building native Android applications.
Kotlin Coroutines:
- Dive deep into asynchronous programming using Kotlin Coroutines.
AR/VR Development:
- Explore Augmented Reality (AR) and Virtual Reality (VR) development for immersive experiences.
Performance Optimization:
- Optimize app performance using tools like Systrace and Profiler.
Publishing and Monetization:
- Understand the process of publishing apps on the Google Play Store and explore monetization strategies.
Open Source Contributions:
- Contribute to open-source Android projects to enhance your skills and give back to the community.
Continuous Learning:
- Stay updated with the latest Android technologies, attend conferences, and participate in online communities.
Subscribe to my newsletter
Read articles from Deepak M. directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
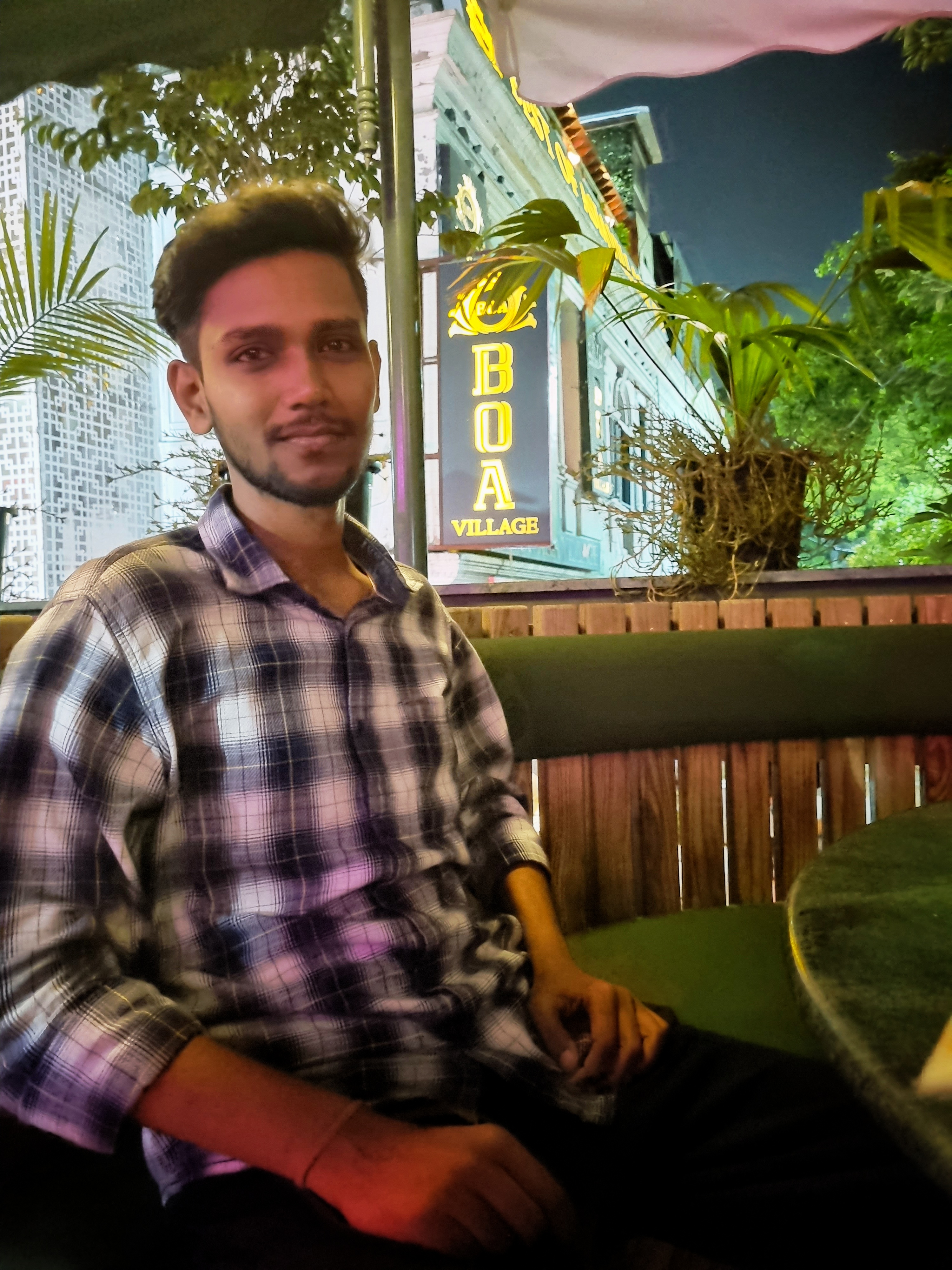
Deepak M.
Deepak M.
I am always eager to explore new challenges and contribute to meaningful projects that make a positive impact. I am confident that my skills and dedication will make me a valuable asset to any team. I am proficient in Android, Kotlin, Java, MVVM Architecture, Firebase, Retrofit, Rest Api, etc.