GitHub Action Runtime Adhoc Commands on PR
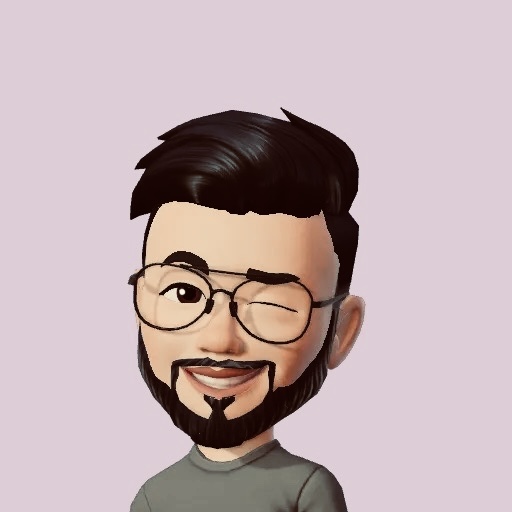
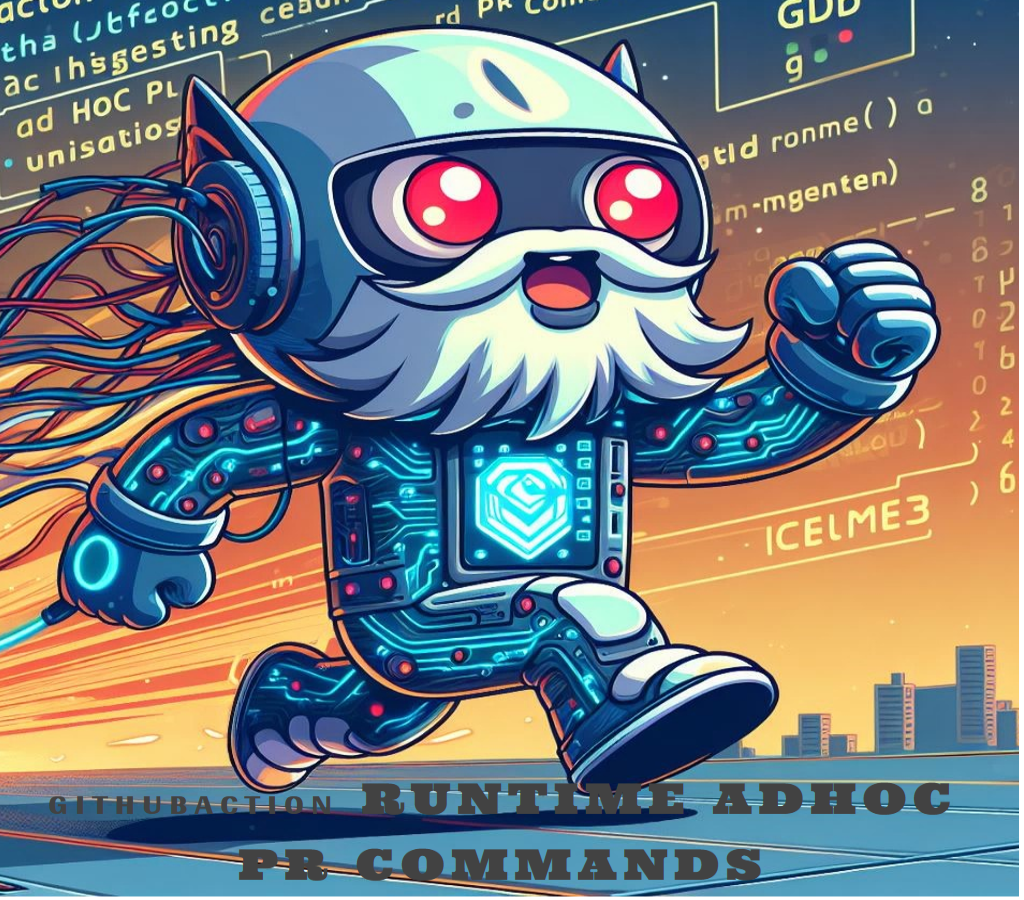
GitHub Actions provides a powerful automation platform that allows you to build, test, and deploy your code directly from your GitHub repository. One of the exciting features of GitHub Actions is the ability to run custom scripts and commands during the workflow.
Overview
In modern software development workflows, efficient collaboration and automation play a crucial role. The "GithubAction Runtime Adhoc PR Commands" is a GitHub Action designed to streamline and automate specific tasks on pull requests, enhancing the overall development process.
The primary purpose of this GitHub Action is to enable the execution of ad-hoc commands dynamically in response to user comments on pull requests. Ad-hoc commands refer to custom, user-defined instructions that can be triggered to perform specific actions. These commands can be particularly useful for tasks such as rebasing, auto squashing, or any other custom workflow steps that developers may need during the pull request review process.
Problem Statement
Imagine a scenario where you want to execute specific commands on a pull request dynamically. GitHub Actions offer a great way to automate workflows, but sometimes you need more flexibility. Maybe you want to trigger certain tasks only when a specific comment is added to a PR. This is where ad-hoc commands come into play.
Key Features
Automation of Custom Commands: The GitHub Action automates the execution of custom commands specified in pull request comments. This eliminates the need for manual intervention, making the development process more efficient.
Dynamic Response to User Interactions: The Action responds dynamically to user comments, allowing developers to trigger specific tasks based on their needs. This flexibility is valuable in scenarios where different actions may be required for different pull requests.
Enhanced Workflow Flexibility: By incorporating this GitHub Action into your workflow, you gain a high degree of flexibility. Developers can interact with the pull request by simply adding specific commands in their comments, and the automation takes care of the rest.
Easy Integration with GitHub Actions: The Action seamlessly integrates with GitHub Actions, utilizing the power of workflows to orchestrate the execution of ad-hoc commands. This integration ensures a smooth and cohesive experience within the GitHub ecosystem.
Use Cases
Rebasing: Developers can trigger a rebase operation on their pull requests by adding the
/rebase
command in a comment. This is particularly useful for keeping the codebase up-to-date with the latest changes from the main branch.Autosquashing: The
/autosquash
command allows developers to automate the process of squashing commits in their pull requests, creating a cleaner commit history.Custom Workflow Steps: Beyond rebase and autosquash, developers can define and execute any custom workflow steps by incorporating specific commands tailored to their project requirements.
Usage:
Add below action to your workflow YAML
Specify your custom commands on the PR comments
watch the magic happen as your PRs get supercharged
name: PR Adhoc commands
on:
issue_comment:
types: [created]
jobs:
build:
name: PR-Adhoc-Commands
runs-on: ubuntu-latest
if: >-
github.event.issue.pull_request != '' &&
(
contains(github.event.comment.body, '/rebase') ||
contains(github.event.comment.body, '/autosquash') ||
contains(github.event.comment.body, '/rebase-autosquash')
)
steps:
- name: Checkout the latest code
uses: actions/checkout@v3
with:
token: ${{ secrets.GITHUB_TOKEN }}
fetch-depth: 0
- name: Running Adhoc commands
uses: GirishCodeAlchemy/githubaction-runtime-adhoc-pr-commands@v1.0
env:
GITHUB_TOKEN: ${{ secrets.GITHUB_TOKEN }}
Command Usage :
Solution Overview
To address this challenge, we'll create a GitHub Action that responds to comments on a pull request and executes custom commands. The key components of the solution include:
GitHub Action Workflow: Define a workflow that triggers on pull request comments.
Custom Action Script: Write a script that interprets the comment content and executes the desired commands.
GitHub API Integration: Use the GitHub API to fetch information about the pull request and perform necessary actions.
Let's break down the implementation step by step.
Implementation
1. GitHub Action Workflow
name: Adhoc Commands
on:
issue_comment:
types:
- created
jobs:
adhoc_commands:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Run Adhoc Commands
uses: GirishCodeAlchemy/githubaction-runtime-adhoc-pr-commands@v1.0
env:
GITHUB_TOKEN: ${{ secrets.GITHUB_TOKEN }}
This workflow triggers on new comments (issue_comment
event) and uses a custom action (which we'll create next) to run the ad-hoc commands.
2. Custom Action Script
# alchemy.py
import subprocess
def execute_adhoc_commands(comment_body):
# Logic to parse and execute commands
# Example: Execute "echo Hello World" if the comment contains "/run-command"
if "/run-command" in comment_body:
subprocess.run(["echo", "Hello World"])
# Entry point
if __name__ == "__main__":
comment_body = input("Enter the comment body: ")
execute_adhoc_commands(comment_body)
In this script, we define a function execute_adhoc_commands
that interprets the comment content and executes specific commands. Customize this logic according to your requirements.
3. GitHub API Integration
Extend the script to fetch additional information about the pull request using the GitHub API. For example, you can retrieve the PR number, author, or any other relevant details.
# alchemy.py
import subprocess
import os
import requests
def get_pr_info():
github_token = os.getenv("GITHUB_TOKEN")
pr_number = os.getenv("PR_NUMBER")
repo = os.getenv("GITHUB_REPOSITORY")
headers = {
"Authorization": f"Bearer {github_token}",
"Accept": "application/vnd.github.v3+json"
}
response = requests.get(
f"https://api.github.com/repos/{repo}/pulls/{pr_number}",
headers=headers
)
return response.json()
def execute_adhoc_commands(comment_body):
pr_info = get_pr_info()
pr_author = pr_info["user"]["login"]
pr_number = pr_info["number"]
# Logic to parse and execute commands
# Example: Execute "echo Hello World" if the comment contains "/run-command"
if "/run-command" in comment_body:
subprocess.run(["echo", f"Hello World from PR {pr_number} by {pr_author}"])
# Entry point
if __name__ == "__main__":
comment_body = input("Enter the comment body: ")
execute_adhoc_commands(comment_body)
Here, we've added a get_pr_info
function to fetch PR information. Update the logic in execute_adhoc_commands
to utilize this information as needed.
Github Marketplace:
The action is now available on the public GitHub Actions Marketplace: PR Runtime Adhoc commands
Conclusion
With this GitHub Action setup, you have a flexible way to execute custom commands dynamically based on comments in your pull requests. Whether you need to trigger specific tests, deploy to staging, or perform any other task, this solution provides the extensibility you need.
๐ Stay tuned for more empowering insights into the world of GitHub Actions and PR runtime adhoc commands. Happy automating!! ๐
โ๏ธ Github: github.com/GirishCodeAlchemy
โ๏ธLinkedin:linkedin.com/in/vgirish10
Subscribe to my newsletter
Read articles from Girish V directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
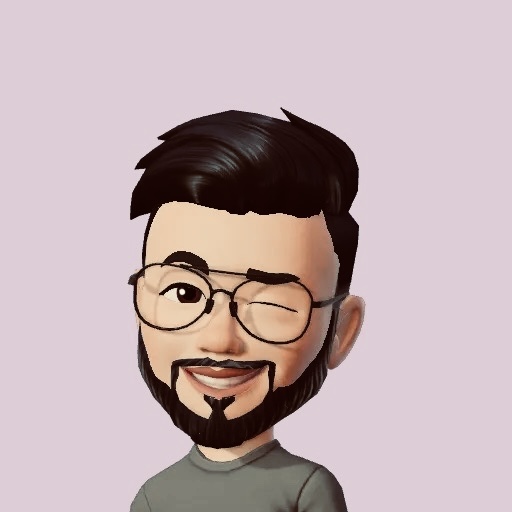