Dart: Concurrency | Performance and Isolate group

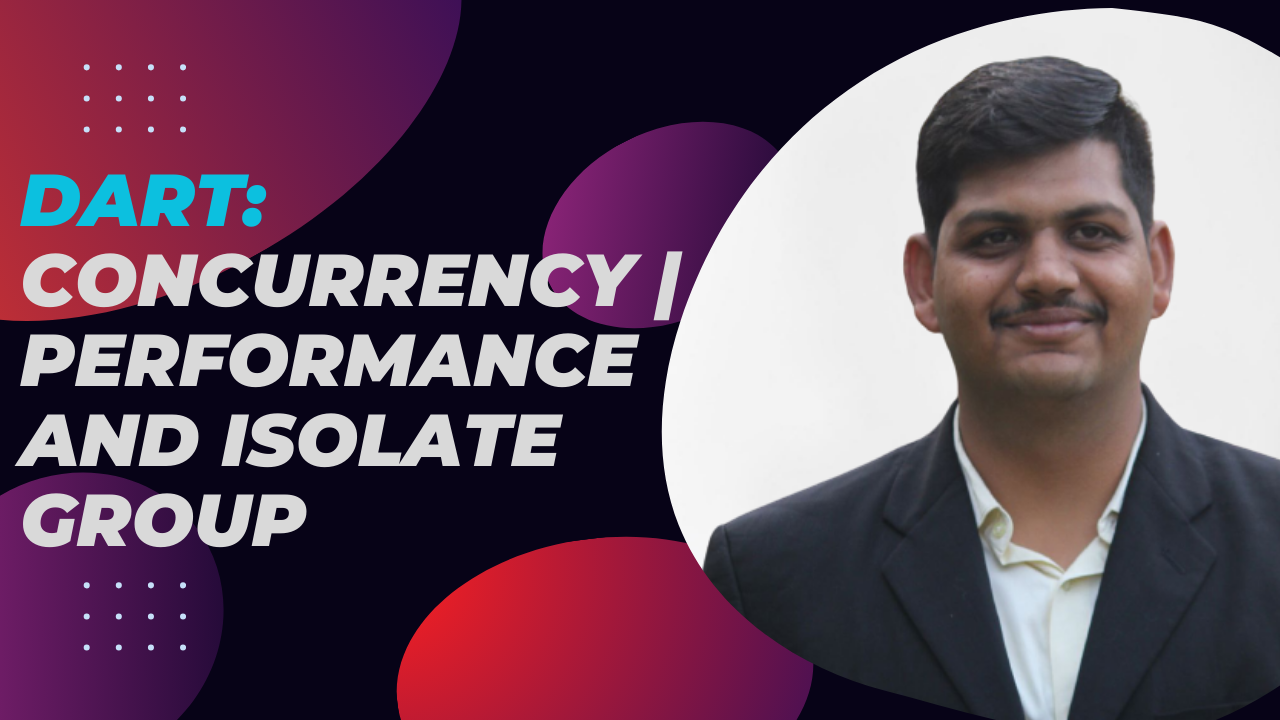
Introduction: In the dynamic landscape of Dart concurrency, developers often seek ways to optimize performance by efficiently managing isolates. Enter the concept of Isolate Groups. In this blog post, we'll explore how Isolate Groups can be harnessed to enhance performance, parallelize tasks, and unlock the full potential of concurrent Dart applications.
Understanding Isolate Groups: Isolate Groups in Dart provide a higher-level abstraction for managing and coordinating multiple isolates. This abstraction is particularly useful when dealing with a large number of isolates, offering enhanced control and organization for better performance.
Example: Basic Usage of Isolate Groups
import 'dart:isolate';
void main() async {
print('Main isolate starts');
// Creating an IsolateGroup with two isolates
final IsolateGroup isolateGroup = IsolateGroup(2);
// Submitting tasks to the isolate group
isolateGroup.spawn(isolateFunction, 'Task 1');
isolateGroup.spawn(isolateFunction, 'Task 2');
// Performing tasks in the main isolate
for (int i = 1; i <= 3; i++) {
print('Main isolate task $i');
await Future.delayed(Duration(seconds: 1));
}
// Closing the isolate group
await isolateGroup.close();
}
void isolateFunction(String task) {
print('Isolate task "$task" started');
// Simulating a task in the isolate
for (int i = 1; i <= 3; i++) {
print('Isolate task "$task": Step $i');
sleep(Duration(seconds: 1));
}
print('Isolate task "$task" ends');
}
Explanation:
The main function creates an IsolateGroup with two isolates.
Tasks are submitted to the isolate group using isolateGroup.spawn.
The main isolate and isolates in the group execute tasks concurrently.
Fine-Tuning Performance with Isolate Groups: Isolate Groups provide additional features to fine-tune performance and control isolate execution. These features include managing isolate priority, handling errors, and dynamically adjusting the number of isolates in the group.
Example: Fine-Tuning Performance with Isolate Groups
import 'dart:isolate';
void main() async {
print('Main isolate starts');
// Creating an IsolateGroup with two isolates
final IsolateGroup isolateGroup = IsolateGroup(2);
// Setting the isolate group's priority
isolateGroup.setPriority(Isolate.immediate);
// Submitting tasks to the isolate group
isolateGroup.spawn(isolateFunction, 'Task 1');
isolateGroup.spawn(isolateFunction, 'Task 2');
// Performing tasks in the main isolate
for (int i = 1; i <= 3; i++) {
print('Main isolate task $i');
await Future.delayed(Duration(seconds: 1));
}
// Dynamically adjusting the number of isolates in the group
isolateGroup.resize(3);
// Closing the isolate group
await isolateGroup.close();
}
void isolateFunction(String task) {
print('Isolate task "$task" started');
// Simulating a task in the isolate
for (int i = 1; i <= 3; i++) {
print('Isolate task "$task": Step $i');
sleep(Duration(seconds: 1));
}
print('Isolate task "$task" ends');
}
Subscribe to my newsletter
Read articles from Vinit Mepani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Vinit Mepani
Vinit Mepani
"Hello World, I'm Vinit Mepani, a coding virtuoso driven by passion, fueled by curiosity, and always poised to conquer challenges. Picture me as a digital explorer, navigating through the vast realms of code, forever in pursuit of innovation. In the enchanting kingdom of algorithms and syntax, I wield my keyboard as a magical wand, casting spells of logic and crafting solutions to digital enigmas. With each line of code, I embark on an odyssey of learning, embracing the ever-evolving landscape of technology. Eager to decode the secrets of the programming universe, I see challenges not as obstacles but as thrilling quests, opportunities to push boundaries and uncover new dimensions in the realm of possibilities. In this symphony of zeros and ones, I am Vinit Mepani, a coder by passion, an adventurer in the digital wilderness, and a seeker of knowledge in the enchanting world of code. Join me on this quest, and let's create digital wonders together!"