File Path System: Foundation
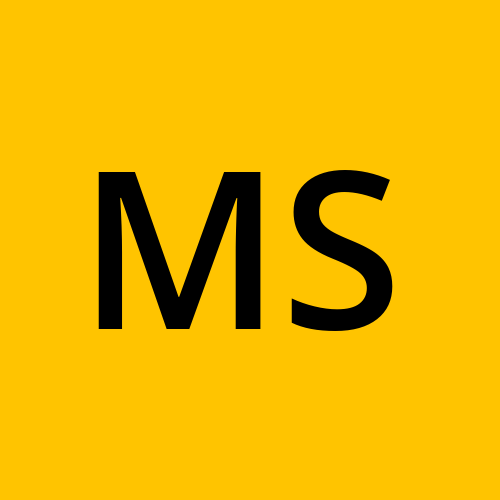
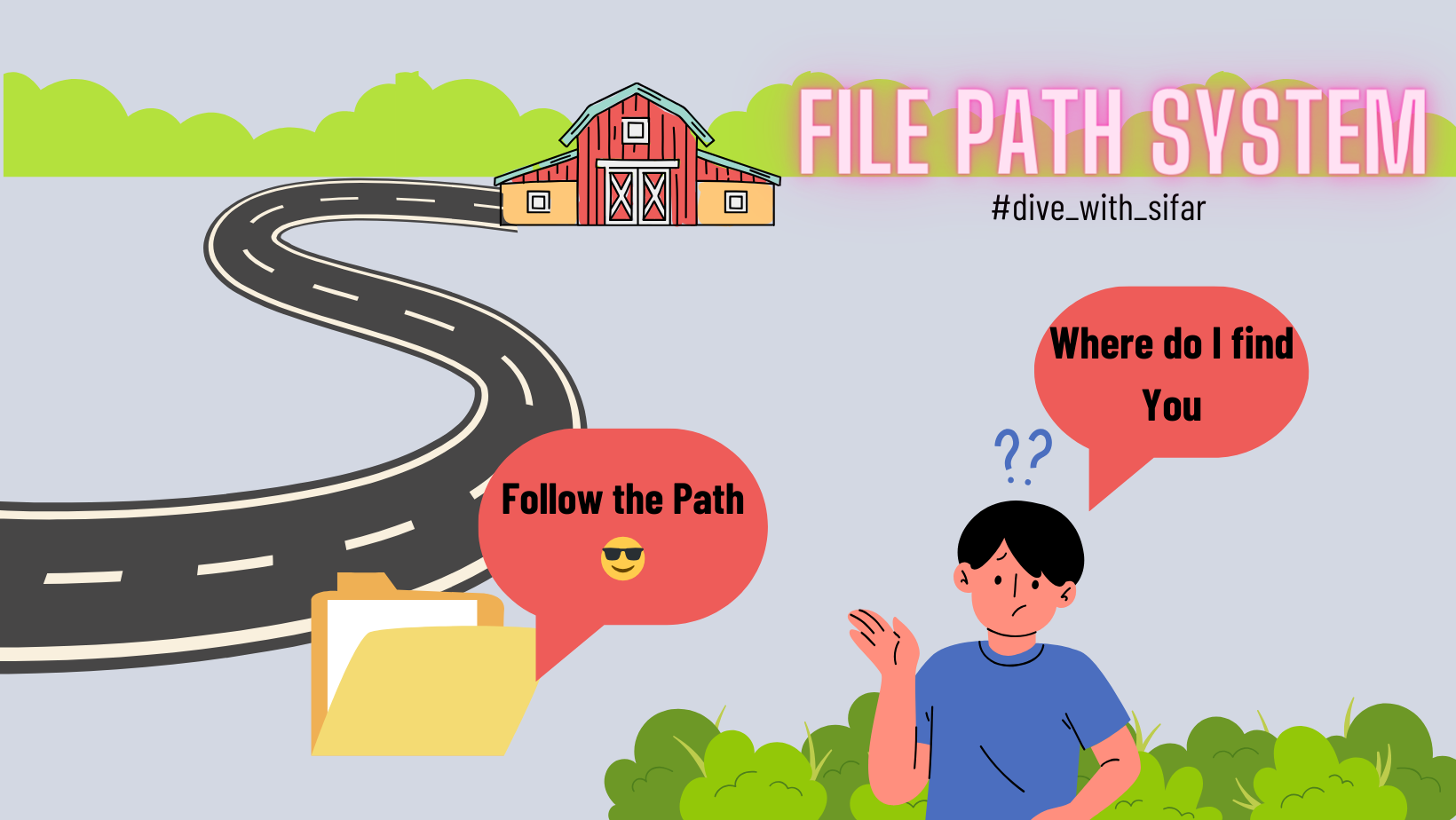
The file path system structure is the backbone of how data is organized, stored, and accessed on a computer. For beginners entering the world of computing, understanding the fundamentals of file systems is a key step towards becoming proficient users. In this blog post, we'll delve into the basics of file system structure, its importance, and the various use cases where this knowledge proves invaluable.
Key Concepts:
In computing, a file system is a way of organizing and storing data on a computer or storage device. It provides a structured way to manage files and directories. Here are some key concepts:
File: A file is a collection of data stored under a specific name. Files can contain text, images, programs, or any other type of information.
Directory (or Folder): A directory is a container for files and other directories. It's like a folder that can hold multiple files or subdirectories.
Path: The location of a file or directory in the file system is specified by its path. A path can be absolute (starting from the root of the file system) or relative (starting from the current working directory).
Absolute Path:
Specifies the exact location from the root of the file system, providing a complete and unambiguous address.
Examples:
/home/user/documents/file.txt
(Linux) orC:\Users\user\Documents\file.txt
(Windows).
Relative Path:
Specifies the location of a file or directory relative to the current working directory.
Examples:
file.txt
(assuming current directory is/home/user/documents/
) orfile.txt
(assuming current directory isC:\Users\user\Documents\
).
When using relative paths, it's important to be aware of the current working directory and navigate accordingly. Absolute paths, on the other hand, provide a full and independent address from the root.
Root Directory: The top-level directory in a file system. In Linux, it's denoted by '/', and in Windows, it's typically 'C:' or another drive letter.
Concept of Backslash(\) in Windows and Forward Slash(/) in Linux:
Windows:
- Use backslash (
\
) in file paths, likeC:\Users\YourUsername\Documents\example.txt
.
- Use backslash (
Linux:
- Use forward slash (
/
) in file paths, like/home/yourusername/Documents/example.txt
.
- Use forward slash (
Parent Directory: The directory that contains a given directory or file. It's represented by two dots ('..'). For instance, if you want to access a file located in the parent directory, you might specify the path as
'../filename.txt'
Current Working Directory: The directory in which a user is currently working. When you open a terminal or command prompt, you are usually in your home directory or another default location.
File Extension: A set of characters at the end of a file name that indicates the file's format or type. For example,
.txt
for text files,.jpg
for image files, etc.
Here's a simple example to illustrate these concepts:
#Linux
/
|-- home/
| |-- user/
| |-- documents/
| |-- file.txt
| |-- image.jpg
|
|-- var/
|-- log/
|-- system.log
#Windows
C:\
|-- Users\
| |-- user\
| |-- Documents\
| |-- file.txt
| |-- image.jpg
|
|-- Program Files\
|-- Common Files\
|-- System\
|-- system.log
In this example:
/
represents the root directory in Linux.C:\
represents the root directory on the C: drive in Windows.The user's home directory is
/home/user/
in Linux andC:\Users\user\
in Windows.The directory structure under the
Documents
directory is the same in both Linux and Windows.The
system.log
file is located in thelog
directory inside thevar
directory in Linux and in theSystem
directory inside theCommon Files
directory on the C: drive in Windows.
Understanding the file system structure is essential for navigating your computer and organizing your files efficiently. Different operating systems (such as Windows, Linux, and macOS) have their own file system structures, but the basic concepts are similar.
Common Mistakes:
Let's delve into these common pitfalls with a reflective perspective and explore solutions to guide us through the labyrinth of file systems.
Certainly! Here's the modified text with brief descriptions added for each point:
1. Incorrect Slash Direction:
Mistake Example:
# Unix-like systems (Linux, macOS)
incorrect_path = 'home/user/documents/file.txt'
# Windows
incorrect_path = 'C:\Users\User\Documents\file.txt'
Solution: Be mindful of the slash direction, aligning it with the conventions of your operating system.
# Unix-like systems
correct_path = 'home/user/documents/file.txt'
# Windows
correct_path = 'C:\\Users\\User\\Documents\\file.txt' # or 'C:/Users/User/Documents/file.txt'
2. Absolute vs. Relative Path Confusion:
Mistake Example:
# Using a relative path instead of an absolute path
relative_path = 'documents/file.txt'
Solution: Clarify the context and use the appropriate path type.
# Absolute path
absolute_path = '/home/user/documents/file.txt'
3. Spaces in Filenames and Directories:
Mistake Example:
# Space in filename without proper quoting
file_path = 'home/user/documents/my file.txt'
Solution: Use quotes or replace spaces with underscores for clarity.
# Using quotes
file_path = 'home/user/documents/my file.txt'
# Or using underscores
file_path = 'home/user/documents/my_file.txt'
4. Case Sensitivity:
Mistake: Ignoring case sensitivity.
# Incorrect
file_path = '/home/user/Documents/File.txt'
Solution:
# Correct
file_path = '/home/user/documents/file.txt'
5. Failure to Escape Special Characters:
Mistake Example:
# Forgetting to escape parentheses
file_path = 'home/user/documents/(special).txt'
Solution: Escape special characters to ensure proper interpretation.
# Escaping parentheses
file_path = 'home/user/documents/\(special\).txt'
6. Hardcoding Paths:
Mistake Example:
# Hardcoding an absolute path
file_path = '/home/user/documents/file.txt'
Solution: Enhance code portability by using relative paths or environment variables.
# Using a relative path
file_path = 'documents/file.txt'
7. Assuming a Fixed Directory Structure:
Mistake Example:
# Assuming a fixed directory structure
config_path = '/etc/application/config.ini'
Solution: Foster flexibility by dynamically determining paths or allowing user configuration.
# Dynamic determination
config_path = get_config_path()
8. Ignoring Error Handling:
Mistake Example:
# Lack of error handling
try:
with open(file_path, 'r') as file:
data = file.read()
except Exception as e:
print(f"An error occurred: {e}")
Solution: Implement robust error handling to catch issues and provide meaningful feedback.
# Error handling
try:
with open(file_path, 'r') as file:
data = file.read()
except FileNotFoundError:
print(f"File not found: {file_path}")
except Exception as e:
print(f"An unexpected error occurred: {e}")
9. Not Checking for Existence:
Mistake Example:
# Not checking if a file exists before opening it
with open(file_path, 'r') as file:
data = file.read()
Solution: Always check for the existence of a file before attempting to access it.
# Checking for existence
if os.path.exists(file_path):
with open(file_path, 'r') as file:
data = file.read()
else:
print(f"File not found: {file_path}")
10. Using Hardcoded Drive Letters:
Mistake Example:
# Hardcoding a Windows drive letter
file_path = 'C:/Users/User/Documents/file.txt'
Solution: Avoid hardcoded drive letters for better cross-compatibility.
# Using a relative path
file_path = 'Documents/file.txt'
11. Include File Extensions:
# Missing file extension
file_path = 'documents/myfile'
Solution:
# Including file extension
file_path = 'documents/myfile.txt'
12. Document Path Conventions:
Best Practice:
- Maintain documentation to outline conventions for file and directory naming, helping team members understand and follow consistent practices.
13. Regularly Test File Operations:
Best Practice:
- Regularly conduct testing for file operations to ensure that file creation, modification, and deletion work as expected, minimizing the risk of errors in production.
By reflecting on these common mistakes and embracing best practices, we can navigate the complexities with greater ease and ensure our code stands resilient across various environments.
Use Cases and Importance:
Organizing and Managing Data: A well-structured file system aids in efficient data organization.
Navigation and Access: Users need to navigate through the file system to locate and open files.
File and Data Security: File permissions and ownership ensure the security of files and data.
Backup and Recovery: File system structure is essential for effective backup and recovery strategies.
Software Installation and Configuration: Correct installation and configuration of software rely on understanding the file system structure.
Interchangeability of (\) and (/) in Python:
Windows:
In Python, you can use either backslashes (
\\
) or forward slashes (/
). For example:path = "C:\\Users\\YourUsername\\Documents\\example.txt" # double backslash is there because in python to write / we need to write //
or
path = "C:/Users/YourUsername/Documents/example.txt"
Linux:
In Python on Linux, use forward slashes (
/
). For example:path = "/home/yourusername/Documents/example.txt"
Tip:
- For simplicity and cross-platform compatibility in Python, it's common to use forward slashes or use
os.path.join()
.
This way, you can use backslashes or forward slashes in Python on Windows, and forward slashes on Linux. The os.path.join()
function in Python can be handy for writing code that works on both operating systems.
Subscribe to my newsletter
Read articles from Md. Musfikur Rahman Sifar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
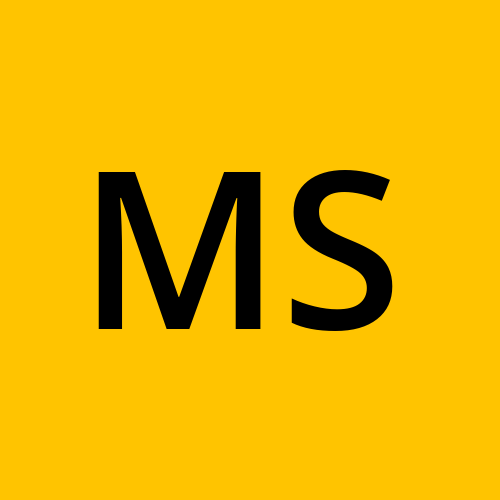